Building Reliable Message Queues and Monitoring Utilization
In the previous article we already saw what RabbitMQ was and how we plan to use it In this article we are going to see how to utilize RabbitMQ with C#, Python and create Triggers for accomplishing small tasks. If you are a Python developer, you may directly want to jump to Python section.
Contents
Building Reliable Message Queues and Monitoring Utilization
Creating triggers with RabbitMQ..
Step 1: Create a Producer That Triggers on an Event
Step 2: Create a Consumer That Reacts to the Trigger
RabbitMQ with Python message queue and utilization.
Step 3: Utilization and Monitoring.
RabbitMQ with C#
RabbitMQ is a widely used open-source message broker for asynchronous communication between services. You can interact with RabbitMQ using various libraries, with RabbitMQ.Client
being a popular choice for C#. Here’s a complete example demonstrating how to use RabbitMQ with C# to send and receive messages.
Prerequisites
- Install RabbitMQ and ensure it’s running.
- Set up a C# environment (like a .NET Core project).
- Install the RabbitMQ.Client package in your C# project (
dotnet add package RabbitMQ.Client
).
Example Overview
In this example, we’ll:
- Create a simple RabbitMQ producer that sends messages to a queue.
- Create a consumer that listens to the queue and processes messages.
Creating the Producer
Let’s create a producer that connects to RabbitMQ, creates a queue, and sends a message to that queue.
csharp
using RabbitMQ.Client;
using System;
using System.Text;
class Producer
{
public static void Main()
{
// Connect to the local RabbitMQ server
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
{
using (var channel = connection.CreateModel())
{
// Declare a queue (it will be created if it doesn't exist)
channel.QueueDeclare(queue: "my_queue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
// Create a message to send
string message = "Hello, RabbitMQ!";
var body = Encoding.UTF8.GetBytes(message);
// Publish the message to the queue
channel.BasicPublish(exchange: "",
routingKey: "my_queue",
basicProperties: null,
body: body);
Console.WriteLine($" [x] Sent '{message}'");
}
}
}
}
This code:
- Connects to RabbitMQ on the local machine.
- Declares a queue named “my_queue”.
- Publishes a simple “Hello, RabbitMQ!” message.
Creating the Consumer
Next, create a consumer that listens to the same queue and processes messages as they arrive.
using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System;
using System.Text;
class Consumer
{
public static void Main()
{
// Connect to the local RabbitMQ server
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
{
using (var channel = connection.CreateModel())
{
// Declare the same queue (should be the same as the producer)
channel.QueueDeclare(queue: "my_queue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
// Create a consumer event handler
var consumer = new EventingBasicConsumer(channel);
consumer.Received += (model, ea) =>
{
var body = ea.Body.ToArray();
var message = Encoding.UTF8.GetString(body);
Console.WriteLine($" [x] Received '{message}'");
};
// Start consuming messages
channel.BasicConsume(queue: "my_queue",
autoAck: true,
consumer: consumer);
Console.WriteLine(" Press [enter] to exit.");
Console.ReadLine(); // Keep the application running
}
}
}
}
This code:
- Connects to RabbitMQ on the local machine.
- Declares the same queue as the producer.
- Listens for new messages and prints them to the console.
Running the Example
To test the complete example:
- Run the producer code. It should connect to RabbitMQ and send a message.
- Run the consumer code. It should connect to RabbitMQ, start listening, and print the message when it arrives.
With this example, you’ve set up a simple producer-consumer pattern with RabbitMQ in C#. This can be extended to more complex messaging scenarios, such as using exchanges and different types of queues.
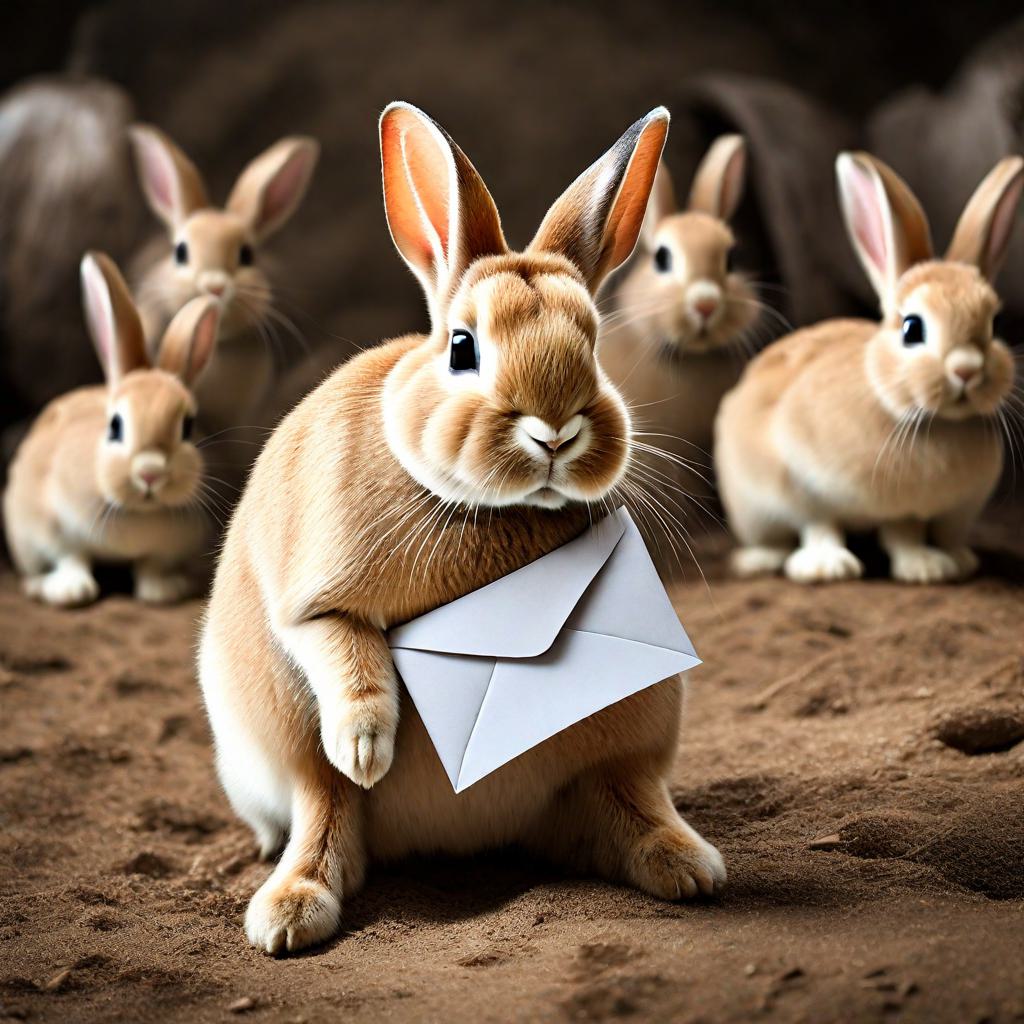
Creating triggers with RabbitMQ
RabbitMQ doesn’t directly support triggers like databases. However, you can achieve similar functionality by creating a system where certain events in one service trigger actions in another service through message passing.
Here is an outline of how you can create a trigger-like behavior with RabbitMQ:
- Define the Triggering Event: Determine the condition that will trigger the message sending. This could be an action in your application, a change in a database, a file system event, etc.
- Create a Producer to Send Trigger Messages: This producer will send a message to RabbitMQ when the triggering event occurs.
- Create a Consumer to React to Trigger Messages: This consumer will listen for messages and take appropriate action when it receives them.
- Choose an Exchange and Routing Strategy: Determine if you’re using direct routing, fanout (broadcast), or other types of exchange. This will depend on your use case and how many consumers you have.
Here’s a simple example where a producer sends a message when a specific condition is met, and a consumer reacts to that message.
Prerequisites
- Install RabbitMQ and ensure it’s running.
- Set up a C# environment (like a .NET Core project).
- Install the RabbitMQ.Client package in your C# project (
dotnet add package RabbitMQ.Client
).
Step 1: Create a Producer That Triggers on an Event
This producer sends a message when a specific condition is met, simulating a trigger-like behavior.
using RabbitMQ.Client;
using System;
using System.Text;
class TriggerProducer
{
public static void Main()
{
// Simulate a condition to trigger an action
bool condition = DateTime.Now.Second % 2 == 0; // Trigger when the current second is even
if (condition)
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
{
using (var channel = connection.CreateModel())
{
// Declare an exchange to route messages
channel.ExchangeDeclare(exchange: "trigger_exchange", type: "direct");
// Define a routing key for the message
string routingKey = "trigger_event";
// Create a message to send
string message = "Trigger event occurred";
var body = Encoding.UTF8.GetBytes(message);
// Publish the message to the exchange with the routing key
channel.BasicPublish(exchange: "trigger_exchange",
routingKey: routingKey,
basicProperties: null,
body: body);
Console.WriteLine($" [x] Triggered '{message}'");
}
}
}
else
{
Console.WriteLine("No trigger event");
}
}
}
This producer checks whether the current second is even and sends a message to a direct exchange if it is.
Step 2: Create a Consumer That Reacts to the Trigger
This consumer listens to a specific queue and processes messages when they arrive.
using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System;
using System.Text;
class TriggerConsumer
{
public static void Main()
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
{
using (var channel = connection.CreateModel())
{
// Declare an exchange and a queue to bind to it
channel.ExchangeDeclare(exchange: "trigger_exchange", type: "direct");
channel.QueueDeclare(queue: "trigger_queue",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
// Bind the queue to the exchange with the routing key
channel.QueueBind(queue: "trigger_queue",
exchange: "trigger_exchange",
routingKey: "trigger_event");
// Set up a consumer to listen to the queue
var consumer = new EventingBasicConsumer(channel);
consumer.Received += (model, ea) =>
{
var body = ea.Body.ToArray();
var message = Encoding.UTF8.GetString(body);
Console.WriteLine($" [x] Received trigger: '{message}'");
};
// Start consuming messages
channel.BasicConsume(queue: "trigger_queue",
autoAck: true,
consumer: consumer);
Console.WriteLine("Listening for trigger events. Press [enter] to exit.");
Console.ReadLine(); // Keep the application running
}
}
}
}
This consumer listens to a specific queue and processes messages with the routing key “trigger_event”.
Running the Example
To test this setup:
- Run the producer code. If the condition is met (current second is even), a message is sent to RabbitMQ.
- Run the consumer code. It will receive and process messages from the queue, indicating a trigger event.
By following this approach, you can create trigger-like behavior with RabbitMQ in a C# environment. The flexibility of RabbitMQ allows you to customize routing strategies, message formats, and other features to suit your needs.
RabbitMQ with Python message queue and utilization
RabbitMQ is a popular message broker that allows you to decouple different components in your system, enabling them to communicate asynchronously. Using RabbitMQ with Python involves setting up a message queue, sending messages from a producer, and processing those messages with a consumer. You can also monitor and manage RabbitMQ to ensure efficient utilization.
Here’s an overview of setting up RabbitMQ with Python, including creating a message queue, producing and consuming messages, and monitoring utilization.
Prerequisites
- Install RabbitMQ and ensure it’s running.
- Set up a Python environment.
- Install
pika
, a RabbitMQ client library for Python (pip install pika
).
Step 1: Create a Producer
Let’s create a producer that sends messages to RabbitMQ.
python code
import pika
# Connect to RabbitMQ server
connection = pika.BlockingConnection(pika.ConnectionParameters(
'localhost'))
channel = connection.channel()
# Declare a queue (create if doesn't exist)
channel.queue_declare(queue=
'my_queue')
# Publish a message to the queue
message =
channel.basic_publish(exchange=
'', routing_key=
'my_queue', body=message)
(
f" [x] Sent '{message}'")
# Close the connection
connection.close()
This code snippet:
- Connects to RabbitMQ on
localhost
. - Declares a queue named “my_queue”.
- Publishes a message to the queue.
- Closes the connection.
Step 2: Create a Consumer
Now, create a consumer that listens to the queue and processes messages.
python
import pika
# Connect to RabbitMQ server
connection = pika.BlockingConnection(pika.ConnectionParameters(
'localhost'))
channel = connection.channel()
# Declare the queue (same name as the producer)
channel.queue_declare(queue=
'my_queue')
# Define a callback function to process messages
defcallback(
ch, method, properties, body):
(
f" [x] Received '{body.decode('utf-8')}'")
# Start consuming messages
channel.basic_consume(queue=
'my_queue', on_message_callback=callback, auto_ack=
True)
(
" [*] Waiting for messages. To exit, press CTRL+C")
# Start the consuming loop
channel.start_consuming()
This code snippet:
- Connects to RabbitMQ on
localhost
. - Declares the same queue as the producer.
- Sets a callback function to process incoming messages.
- Starts consuming messages.
Step 3: Utilization and Monitoring
To ensure efficient utilization of RabbitMQ, consider the following:
- Queue Durability: Ensure queues are durable so they persist even if RabbitMQ restarts.
- Auto Acknowledge: If messages must be processed at least once, set
auto_ack=False
and manually acknowledge messages after processing. - Prefetch Count: To control how many messages are sent to a consumer before it’s overwhelmed, use
basic_qos
to set a prefetch count. - Monitoring: Use RabbitMQ’s management interface to monitor queue sizes, message rates, and more.
Prefetch Count
You can control how many messages a consumer gets before acknowledging with basic_qos
:
python
channel.basic_qos(prefetch_count=
1)
# Only send one unacknowledged message at a time
RabbitMQ Management Interface
RabbitMQ has a web-based management interface for monitoring:
- Enable the management plugin:
rabbitmq-plugins enable rabbitmq_management
- Open a browser and go to
http://localhost:15672/
(default username/password isguest
/guest
). - Monitor queues, exchanges, and overall server health.
Putting It All Together
To create a full workflow with RabbitMQ and Python:
- Set up RabbitMQ with Python producers and consumers as described above.
- Consider how to handle message retries, dead-letter queues, and consumer scaling.
- Use RabbitMQ management tools to monitor queues and server health.
- Use best practices like queue durability, manual acknowledgments, and prefetch controls to ensure efficient utilization and message delivery.
By following this approach, you can build a robust system that uses RabbitMQ for asynchronous messaging in Python, with a focus on reliability and monitoring to ensure smooth operations.
Related Article