ORMs are typically a bridge between frontend and database backend. Object-Relational Mapping (ORM) is a programming technique used to convert data between incompatible type systems in object-oriented programming languages. This method creates a “virtual object database” that can be used from within the programming language. The primary goal of ORM is to bridge the gap between the relational database and the object-oriented models by automating the conversion of data. In this article, we will delve into the core concepts, advantages, challenges, and common implementations of ORM.
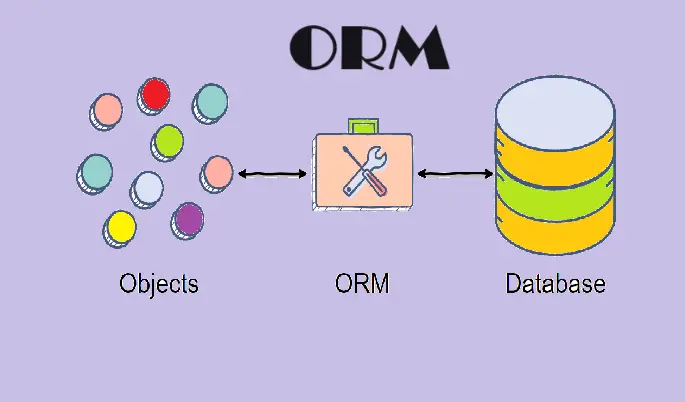
Core Concepts of ORM
Object-Relational Impedance Mismatch
The term “object-relational impedance mismatch” refers to the conceptual and technical difficulties that arise when a relational database system interacts with an object-oriented programming language. Relational databases are designed around the concept of tables, columns, and rows, whereas object-oriented programming languages are structured around objects, classes, and inheritance. These two paradigms are fundamentally different, leading to a mismatch that ORM aims to resolve.
Mapping Objects to Database Tables
At the heart of ORM is the concept of mapping objects in the code to database tables. Each class in the object-oriented model corresponds to a table in the database. Similarly, each instance of a class corresponds to a row in the table, and each attribute of the class maps to a column in the table. This mapping process allows developers to interact with the database using the object-oriented paradigm without writing extensive SQL queries.
CRUD Operations
CRUD operations, which stand for Create, Read, Update, and Delete, are the four basic functions of persistent storage. ORM tools simplify these operations by providing an abstraction layer. For example, to create a new record, a developer simply needs to create an instance of a class and save it, rather than writing an SQL INSERT
statement.
Advantages of ORM
Increased Productivity
ORM significantly boosts developer productivity. By automating the conversion between objects and database tables, ORM allows developers to work within the familiar environment of their programming language. This reduces the amount of boilerplate code required and allows developers to focus on the business logic of the application.
Code Maintainability
Since ORM abstracts the database interactions, it leads to more maintainable code. Changes in the database schema can often be managed with minimal changes to the codebase. This abstraction layer also makes it easier to refactor code, as the database interactions are encapsulated within the ORM framework.
Reduced SQL Dependency
With ORM, developers do not need to write complex SQL queries. The ORM framework generates the necessary SQL statements based on the operations performed on objects. This abstraction reduces the learning curve for developers who are not proficient in SQL and ensures that the SQL code is optimized and secure.
Database Agnosticism
ORM frameworks support multiple database systems, enabling applications to be more flexible in terms of the database technology used. Switching from one database to another becomes more straightforward, as the ORM handles the differences in SQL dialects and database functionalities.
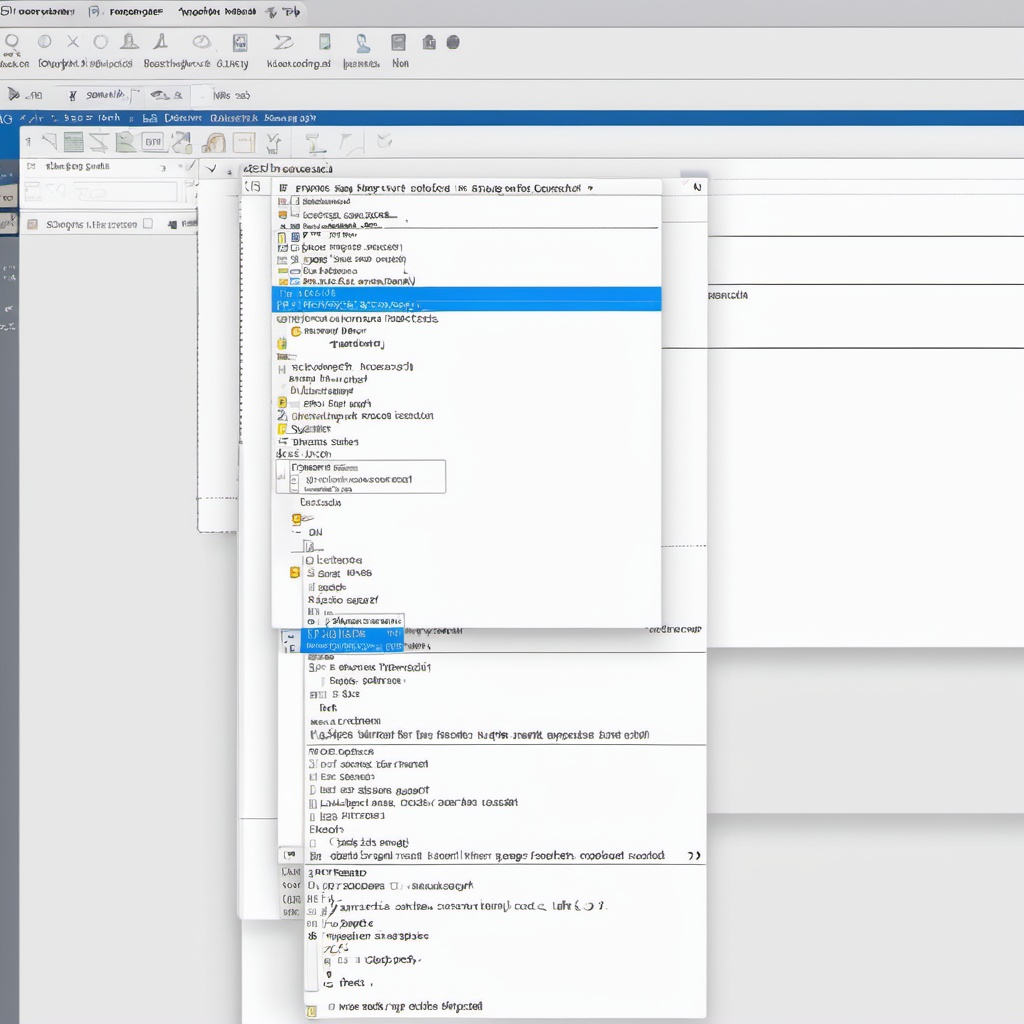
Challenges of ORM
Performance Overhead
While ORM simplifies database interactions, it can introduce performance overhead. The abstraction layer can generate suboptimal SQL queries, leading to slower performance compared to hand-written SQL. For applications with high performance requirements, careful optimization and sometimes manual SQL tuning are necessary.
Complexity in Advanced Queries
Simple CRUD operations are straightforward with ORM, but complex queries can become cumbersome. ORM frameworks provide mechanisms to handle advanced queries, but these can become complex and less intuitive than writing raw SQL. Developers might need to balance between using ORM for simplicity and writing custom SQL for performance and complexity.
Learning Curve
Despite its advantages, ORM has a learning curve. Developers need to understand the underlying principles of ORM and how to use the framework effectively. Misuse of ORM can lead to inefficient code and difficult-to-debug issues. Proper training and experience are essential to leverage the full potential of ORM.
Limited Flexibility
ORM frameworks impose certain constraints and conventions on how the database schema and object models should be designed. This can limit the flexibility for developers who have specific database design requirements. Sometimes, the ORM’s way of mapping objects to tables may not align perfectly with the desired database design.
Common ORM Implementations
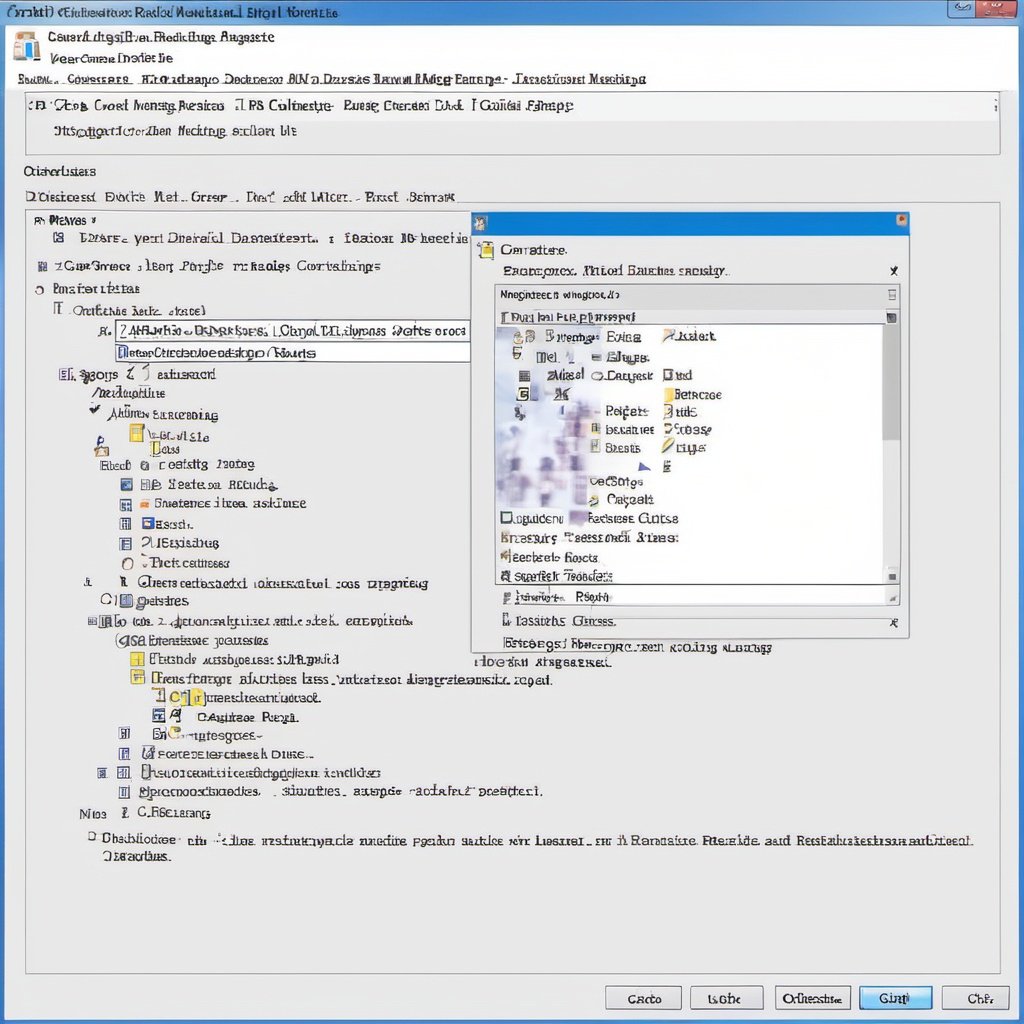
Hibernate (Java)
Hibernate is one of the most popular ORM frameworks for Java. It provides a comprehensive solution for mapping Java classes to database tables and supports various relational databases. Hibernate automates many of the repetitive tasks associated with database interactions and offers a robust query language, HQL (Hibernate Query Language), for complex queries.
Entity Framework (C#)
Entity Framework (EF) is a widely used ORM framework for .NET applications. It allows developers to work with data in the form of objects and properties, without having to focus on the underlying database tables and columns. EF supports both code-first and database-first approaches, making it flexible for different development workflows.
SQLAlchemy (Python)
SQLAlchemy is a powerful ORM for Python that provides both high-level ORM capabilities and low-level database access. It is known for its flexibility and support for a wide range of database backends. SQLAlchemy allows developers to use a declarative syntax for defining models and supports advanced querying capabilities through its SQL Expression Language.
Django ORM (Python)
Django, a high-level web framework for Python, comes with its own ORM. Django ORM provides a simple and intuitive way to interact with the database using Python classes and methods. It is tightly integrated with the Django framework, making it a popular choice for web development projects in Python.
Active Record (Ruby on Rails)
Active Record is the ORM layer supplied with the Ruby on Rails framework. It follows the Active Record design pattern, where classes are mapped to database tables and instances of classes are mapped to rows in the table. Active Record provides a rich set of features for database interactions and integrates seamlessly with the Rails framework.
Best Practices for Using ORM
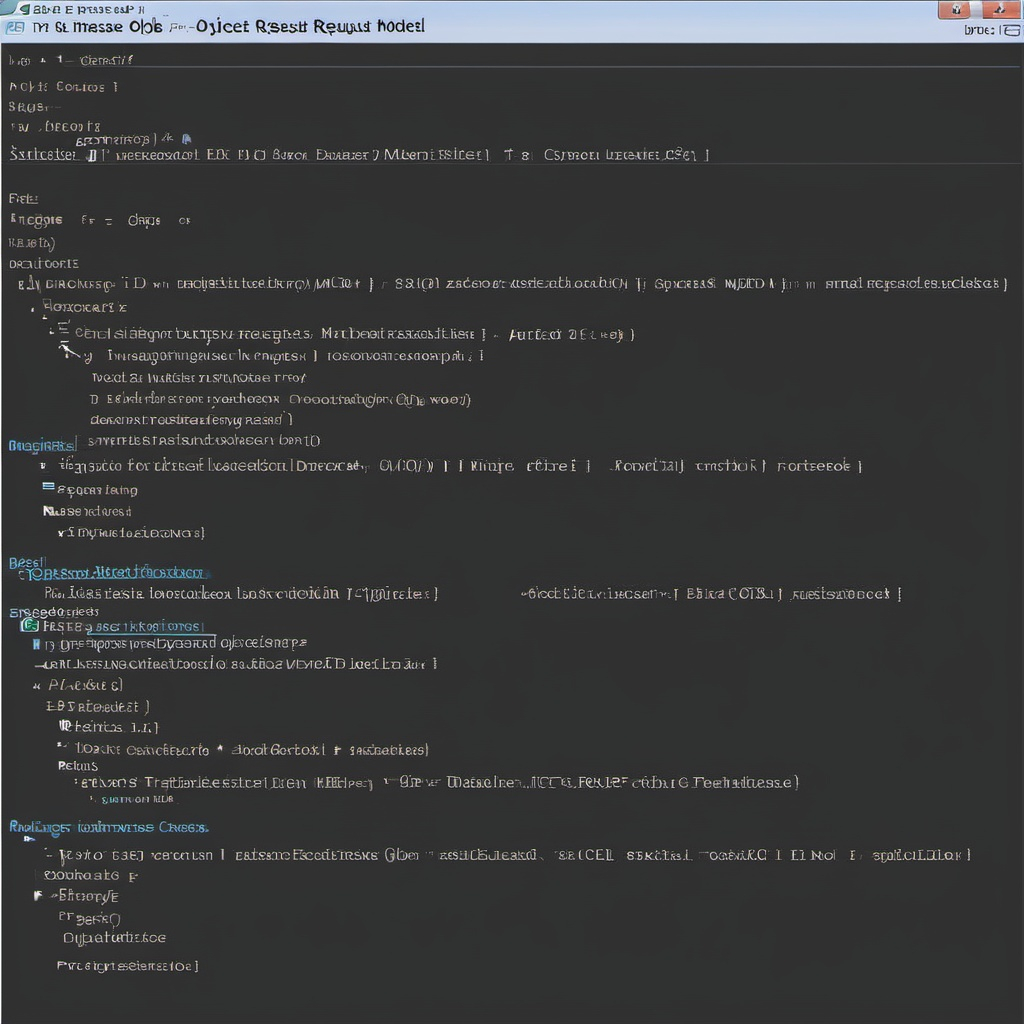
Understand the ORM Framework
Before using any ORM framework, it is crucial to understand its features, limitations, and best practices. Proper knowledge of the framework can help avoid common pitfalls and ensure efficient use of its capabilities.
Optimize Performance
To mitigate the performance overhead introduced by ORM, developers should profile and optimize their database interactions. This includes using lazy loading, optimizing queries, and leveraging the caching mechanisms provided by the ORM framework.
Use Raw SQL When Necessary
While ORM abstracts away SQL, there are situations where writing raw SQL is more efficient. For complex and performance-critical queries, developers should not hesitate to use raw SQL. Most ORM frameworks provide mechanisms to execute raw SQL when needed.
Keep Business Logic Separate
Business logic should be kept separate from the data access layer. This separation of concerns makes the code more modular and maintainable. ORM should be used to interact with the database, while business logic should reside in the service layer or domain model.
Regularly Review Database Schema
Regularly reviewing and optimizing the database schema is important, especially as the application evolves. This ensures that the database structure remains efficient and aligned with the application’s requirements.
Why These ORMs Are Popular
The most used ORM depends on the specific technology stack and the requirements of the project. Hibernate, Entity Framework, SQLAlchemy, Django ORM, and Active Record are among the most popular ORMs in their respective communities. Their popularity is driven by strong community support, comprehensive documentation, seamless integration with frameworks, and a balance of flexibility and performance. For any given project, the best ORM is the one that aligns well with the development team’s expertise, the project requirements, and the existing technology stack.
Community and Ecosystem Support
The popularity of these ORMs is often tied to the strength of their ecosystems. Hibernate and Entity Framework benefit from the robust Java and .NET communities, respectively. SQLAlchemy and Django ORM are bolstered by Python’s popularity, while Active Record thrives within the Rails community.
Comprehensive Documentation and Resources
These ORMs offer extensive documentation, tutorials, and community support, making it easier for developers to learn and troubleshoot issues. The availability of these resources contributes significantly to their widespread adoption.
Integration with Frameworks
Many of these ORMs are tightly integrated with popular web frameworks. For example, Django ORM is part of the Django framework, and Active Record is integral to Ruby on Rails. This seamless integration simplifies development and encourages adoption.
Flexibility and Performance
These ORMs provide a balance of flexibility and performance. They allow developers to perform simple CRUD operations easily while also offering tools for complex querying and optimization. This versatility makes them suitable for a wide range of applications, from small projects to large enterprise systems.
Enterprise Adoption
In enterprise environments, the choice of ORM is often driven by the technology stack in use. Hibernate and Entity Framework are commonly adopted in large organizations due to their robust feature sets and support for enterprise-grade applications.
Conclusion
Object Relational Mapping is a powerful technique that bridges the gap between object-oriented programming and relational databases. By automating the conversion between objects and database tables, ORM simplifies database interactions and enhances productivity. However, it comes with its own set of challenges, including performance overhead and complexity in advanced queries. Understanding these challenges and following best practices can help developers effectively leverage ORM in their applications. With popular implementations like Hibernate, Entity Framework, SQLAlchemy, and Django ORM, developers have a wide range of tools to choose from, each with its own strengths and use cases. By carefully selecting and using an ORM framework, developers can build robust and maintainable applications that efficiently manage data.
Díky moc!|Hej, jeg synes, dette er en fremragende blog. Jeg snublede over det;
Esta página tem definitivamente toda a informação que eu queria sobre este assunto e não sabia a quem perguntar. Este é o meu primeiro comentário aqui, então eu só queria dar um rápido