Introduction
Selenium has become one of the most widely used automation testing tools for web applications due to its open-source nature, cross-browser compatibility, and support for multiple programming languages. It enables testers to automate repetitive test cases, ensuring faster and more accurate results compared to manual testing. Whether you are a beginner or an experienced tester, mastering Selenium is essential for securing a career in automation testing.
In today’s competitive job market, companies seek professionals with hands-on experience in Selenium and a strong understanding of its concepts. During Selenium interviews, candidates are often evaluated on their ability to write and execute automation scripts, handle dynamic web elements, and integrate Selenium with frameworks such as TestNG, Cucumber, and Jenkins. Employers also test knowledge of best practices in test automation, performance optimization, and debugging techniques.
This comprehensive list of the Top 50 Selenium Interview Questions and Answers covers fundamental and advanced topics, including WebDriver commands, locators, waits, handling pop-ups, executing JavaScript within Selenium, and automation framework concepts. These questions are designed to help job seekers prepare effectively and increase their chances of acing their Selenium interviews.
By studying these questions, candidates will not only build confidence but also develop a deeper understanding of real-world test automation scenarios. Whether you are applying for a QA Automation Engineer, Test Analyst, or SDET (Software Development Engineer in Test) role, this guide will serve as a valuable resource in your interview preparation.
Basic Selenium Questions
- What is Selenium?
Selenium is an open-source automated testing framework for web applications across different browsers and platforms. It provides support for multiple programming languages like Java, Python, and C#. - What are the main components of Selenium?
Selenium consists of four components: Selenium IDE, Selenium RC (deprecated), Selenium WebDriver, and Selenium Grid. - How does Selenium WebDriver work?
Selenium WebDriver interacts with web browsers using browser-specific drivers. It sends commands to the browser and retrieves results using the browser’s native automation support. - What are the limitations of Selenium?
Selenium cannot automate Windows-based applications, handle captcha, and perform mobile testing without third-party tools. - Which programming languages are supported by Selenium?
Selenium supports Java, Python, C#, Ruby, JavaScript, and Kotlin.
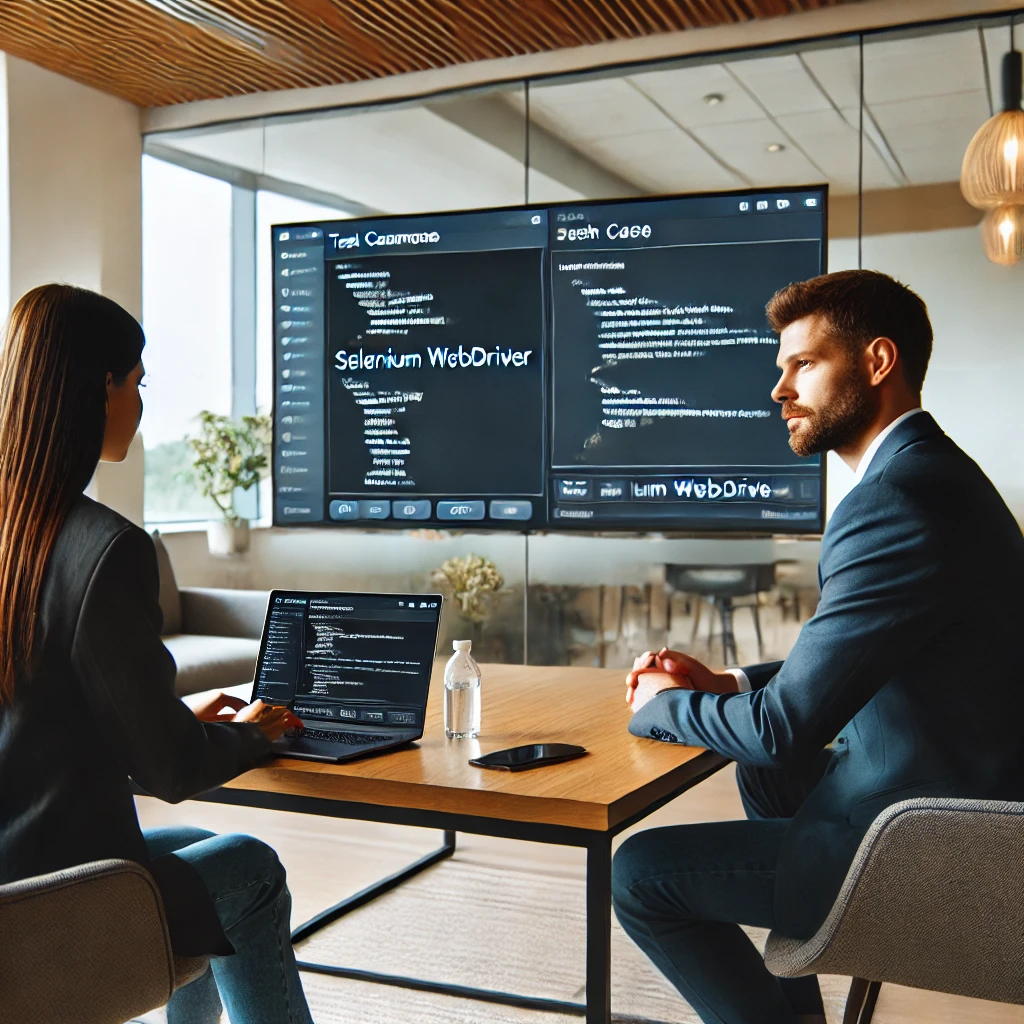
Locators and Web Elements
- What are Selenium locators?
Locators in Selenium are used to find web elements. Examples include ID, Name, Class Name, XPath, CSS Selector, Link Text, and Tag Name. - What is XPath in Selenium?
XPath is an XML path used to navigate through elements in an HTML document. It can be absolute or relative. - What is the difference between absolute and relative XPath?
Absolute XPath starts from the root element (/html/body/…), while relative XPath starts from anywhere (//tagname[@attribute=’value’]). - What is the difference between CSS Selector and XPath?
CSS Selectors are faster and more readable, while XPath is more powerful for navigating dynamic elements. - How do you handle dynamic elements in Selenium?
You can use dynamic XPath, CSS Selectors with contains (*), starts-with (^), or ends-with ($) functions.
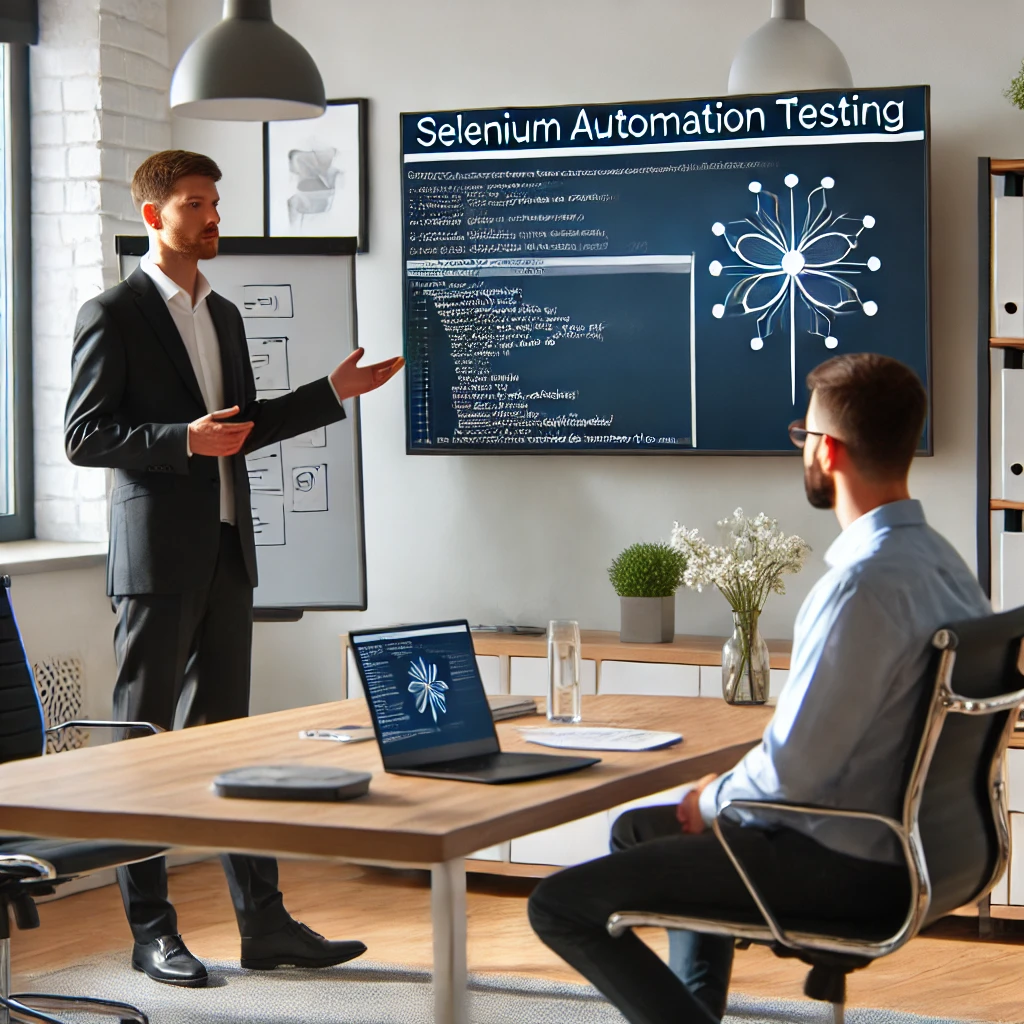
WebDriver Commands
- What is the difference between driver.get() and driver.navigate().to()?
driver.get() waits for the page to load, while driver.navigate().to() does not wait for page load completion. - How do you maximize a browser window in Selenium?
Use driver.manage().window().maximize(); in Java. - How do you refresh a webpage in Selenium?
You can use driver.navigate().refresh(); or driver.get(driver.getCurrentUrl());. - How do you handle alerts in Selenium?
Use driver.switchTo().alert() to handle JavaScript alerts and pop-ups. - How do you capture screenshots in Selenium?
Use TakesScreenshot interface and FileUtils.copyFile(src, new File(“destination path”)).
Synchronization and Waits
- What are the different types of waits in Selenium?
Selenium provides three types of waits: Implicit Wait, Explicit Wait, and Fluent Wait. - What is the difference between Implicit and Explicit Wait?
Implicit Wait applies to all elements globally, while Explicit Wait waits for a specific condition before proceeding. - How do you implement Explicit Wait in Selenium?
Use WebDriverWait along with ExpectedConditions. - What is Fluent Wait in Selenium?
Fluent Wait polls the DOM periodically until the expected condition is met. - How do you handle a page load timeout in Selenium?
Use driver.manage().timeouts().pageLoadTimeout(Duration.ofSeconds(30));.
Frames, Windows, and Pop-ups
- How do you switch between multiple windows in Selenium?
Use driver.switchTo().window(windowHandle);. - How do you handle frames in Selenium?
Use driver.switchTo().frame(index or name or WebElement);. - How do you close a specific browser window in Selenium?
Use driver.close(); for the current window and driver.quit(); for all windows. - How do you handle browser pop-ups in Selenium?
Use Alert alert = driver.switchTo().alert(); and alert.accept(); or alert.dismiss();. - How do you upload a file in Selenium?
Use sendKeys() method on file input element.
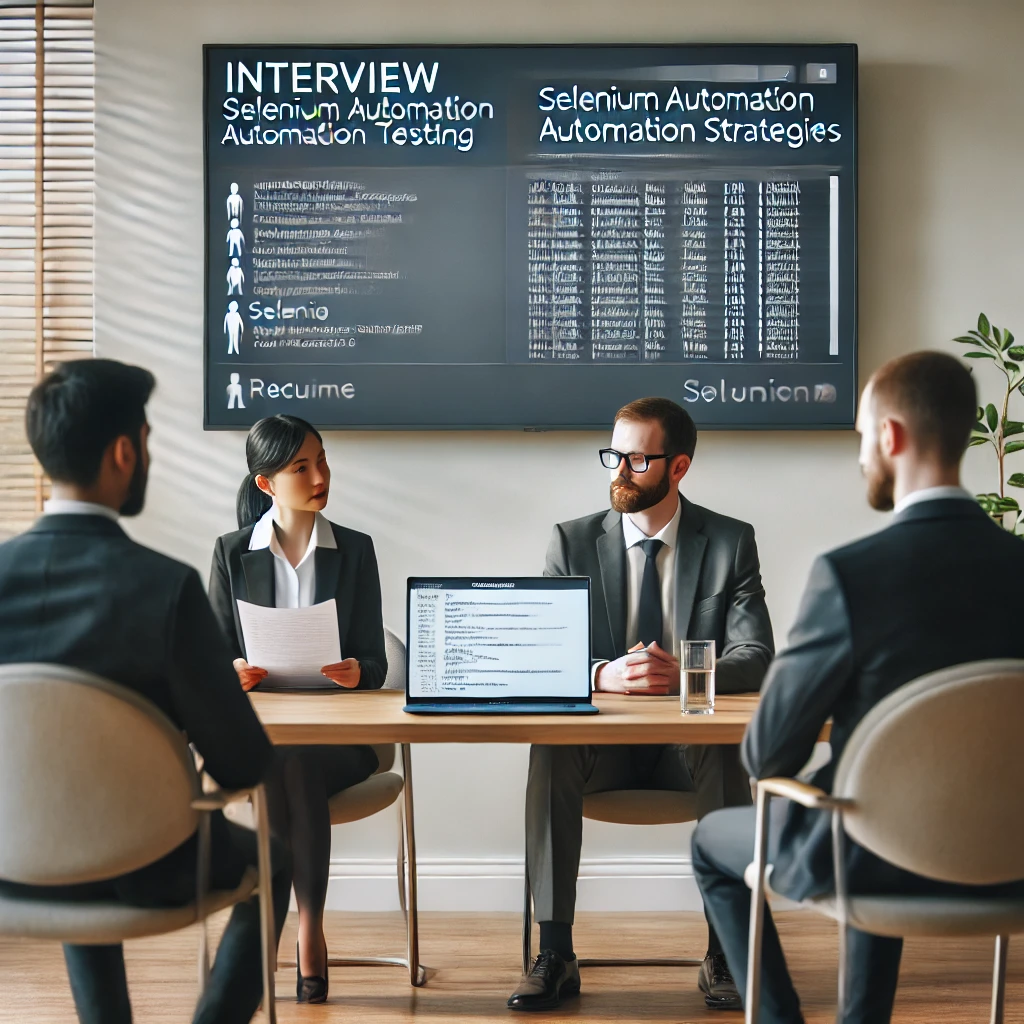
Advanced Selenium Questions
- How do you perform mouse hover in Selenium?
Use Actions class and moveToElement(element).perform();. - What is the use of JavaScript Executor in Selenium?
JavaScript Executor helps execute JavaScript code within Selenium using ((JavascriptExecutor) driver).executeScript(“script”);. - How do you perform right-click (context click) in Selenium?
Use Actions class with contextClick(element).perform();. - How do you drag and drop an element in Selenium?
Use Actions class with dragAndDrop(source, target).perform();. - What is headless browser testing in Selenium?
Headless browsers run without a UI, using tools like Chrome Headless, PhantomJS, or HtmlUnitDriver.
Selenium Frameworks
- What are the types of frameworks in Selenium?
Data-Driven, Keyword-Driven, Hybrid, and BDD (Behavior Driven Development). - What is Page Object Model (POM) in Selenium?
POM is a design pattern that creates an object repository for web elements to improve maintainability. - How do you implement a Page Factory in Selenium?
Use @FindBy annotation and PageFactory.initElements(driver, this);. - What is TestNG and how is it used with Selenium?
TestNG is a testing framework that provides annotations, parallel execution, and assertions. - How do you perform parallel testing in Selenium?
Use TestNG parallel attribute in the XML configuration file.
Miscellaneous Questions
- How do you handle SSL Certificate errors in Selenium?
Use ChromeOptions or FirefoxOptions with acceptInsecureCerts(true);. - How do you read data from Excel in Selenium?
Use Apache POI library. - How do you integrate Selenium with Jenkins?
Configure a Jenkins job and use Maven/TestNG for execution. - What are some alternatives to Selenium?
Cypress, Playwright, Puppeteer, and TestCafe. - What is BDD in Selenium?
BDD (Behavior-Driven Development) uses Cucumber, SpecFlow, and JBehave for writing test cases in natural language. - What are shadow DOM elements?
Elements inside a shadow root that require JavaScript Executor to access. - How do you execute failed test cases in TestNG?
Use testng-failed.xml to rerun failed tests. - What is Selenium Grid?
Selenium Grid enables parallel execution across multiple machines. - How do you test mobile applications with Selenium?
Use Appium. - What is Docker in Selenium?
Docker allows running Selenium tests in isolated containers. - What are the common exceptions in Selenium?
NoSuchElementException, StaleElementReferenceException, TimeoutException, etc. - How do you debug Selenium scripts?
Use breakpoints and debugging tools in IDEs. - What is Sauce Labs in Selenium?
It is a cloud-based testing platform for Selenium. - How do you handle authentication pop-ups in Selenium?
Use https://username:password@url.com. - What is the future of Selenium?
Selenium 4 introduces new features like improved grid, relative locators, and better debugging.
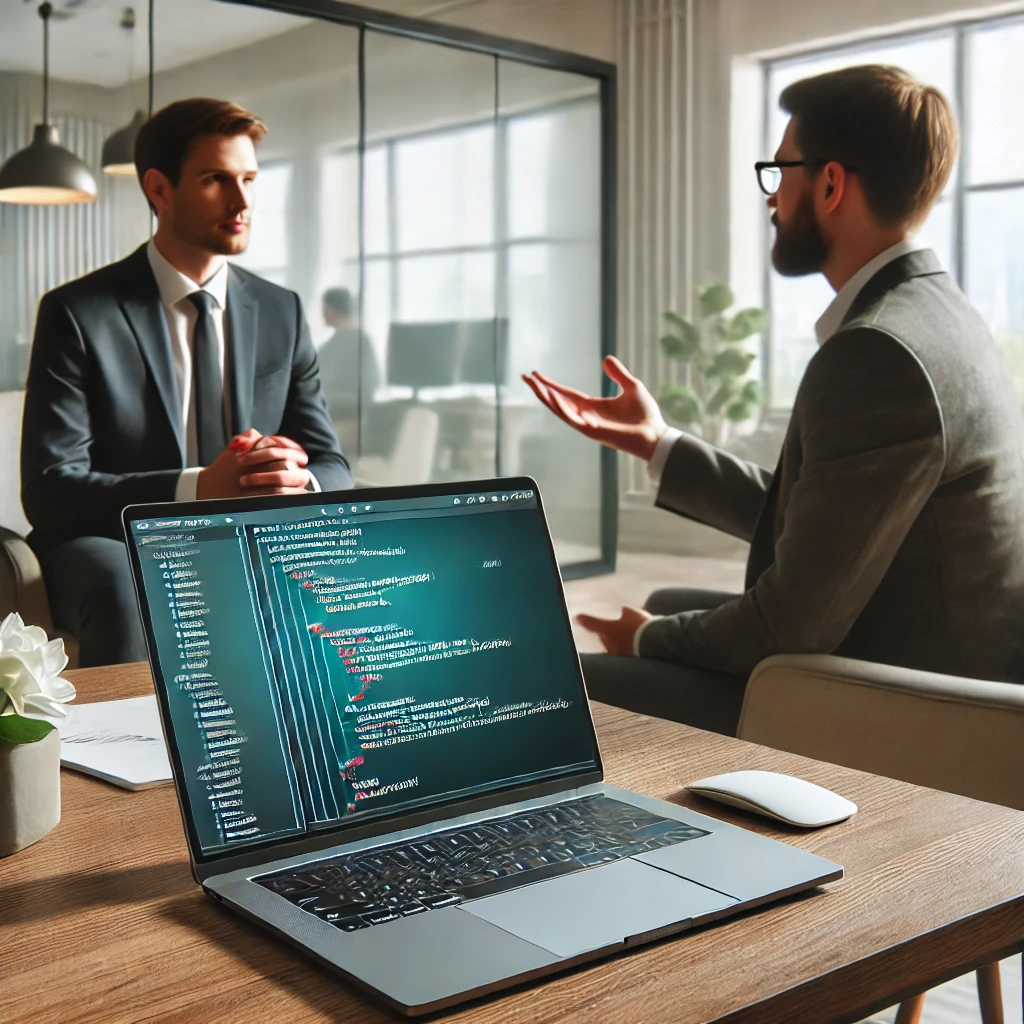
Conclusion
Selenium remains a cornerstone of modern test automation, allowing testers to build robust, scalable, and maintainable test scripts. As businesses continue to prioritize automation testing for faster software releases and improved quality assurance, the demand for skilled Selenium professionals continues to grow.
By mastering Selenium concepts, including WebDriver commands, locators, synchronization techniques, and framework integration, candidates can enhance their technical skills and stand out in interviews. Additionally, keeping up with new features in Selenium 4, such as relative locators, improved grid functionality, and better debugging tools, will give job seekers a competitive edge.
Automation testing is evolving with new technologies like AI-driven testing, cloud-based execution, and continuous integration tools such as Jenkins and Docker. To stay ahead in the field, it is essential for testers to explore these trends and expand their knowledge beyond Selenium.
Preparing for a Selenium interview requires a blend of theoretical understanding and hands-on practice. Practicing commonly asked questions, working on real-world automation projects, and troubleshooting complex scenarios will significantly enhance confidence and problem-solving abilities.
This guide serves as a stepping stone for individuals aiming to excel in their Selenium interviews and establish a successful career in automation testing. Keep learning, stay updated with industry trends, and continue refining your Selenium expertise to thrive in this ever-evolving field.
Curated Reads