In this article we cover the Top 50 Ruby Technical Interview Questions with Answers. Ruby is a dynamic, open-source programming language known for its simplicity and productivity. Designed by Yukihiro “Matz” Matsumoto in 1995, Ruby emphasizes human-readable syntax and follows the principle of “least surprise,” making it intuitive for developers. It supports multiple programming paradigms, including object-oriented, functional, and imperative programming. Ruby’s flexibility and powerful metaprogramming capabilities have made it a favorite for web development, particularly with the Ruby on Rails framework.
One of Ruby’s standout features is its elegant and concise syntax, which allows developers to write less code while achieving more functionality. The language is highly expressive, with built-in methods that simplify complex operations. Ruby’s object-oriented nature means everything is an object, including primitive data types like integers and strings. Additionally, Ruby’s robust ecosystem, supported by gems (libraries), enhances its functionality for tasks ranging from web scraping to machine learning.
Despite being an interpreted language, Ruby delivers impressive performance for most applications. Its community-driven development ensures continuous improvements, keeping it relevant in modern software development. Companies like GitHub, Shopify, and Airbnb rely on Ruby for its scalability and maintainability. Whether for scripting, automation, or full-stack development, Ruby remains a versatile and developer-friendly language.
Top 50 Ruby Programming Technical Interview Questions with Answers
- What is Ruby?
- Ruby is a dynamic, object-oriented scripting language designed for simplicity and productivity.
- Who created Ruby?
- Yukihiro “Matz” Matsumoto in 1995.
- What is IRB in Ruby?
- Interactive Ruby Shell, a REPL for executing Ruby commands.
- Explain Ruby’s principle of “least surprise.”
- Ruby behaves intuitively, minimizing confusion for developers.
- What are Ruby gems?
- Libraries or plugins that extend Ruby’s functionality.
- How does Ruby handle garbage collection?
- Automatically manages memory using a mark-and-sweep algorithm.
- What is the difference between
nil
andfalse
in Ruby?nil
represents absence, whilefalse
is a Boolean value.
- What are symbols in Ruby?
- Immutable, memory-efficient identifiers prefixed with a colon (
:symbol
).
- Immutable, memory-efficient identifiers prefixed with a colon (
- How do you define a class in Ruby?
class MyClass; end
- What is the use of
yield
in Ruby?- Invokes a block passed to a method.
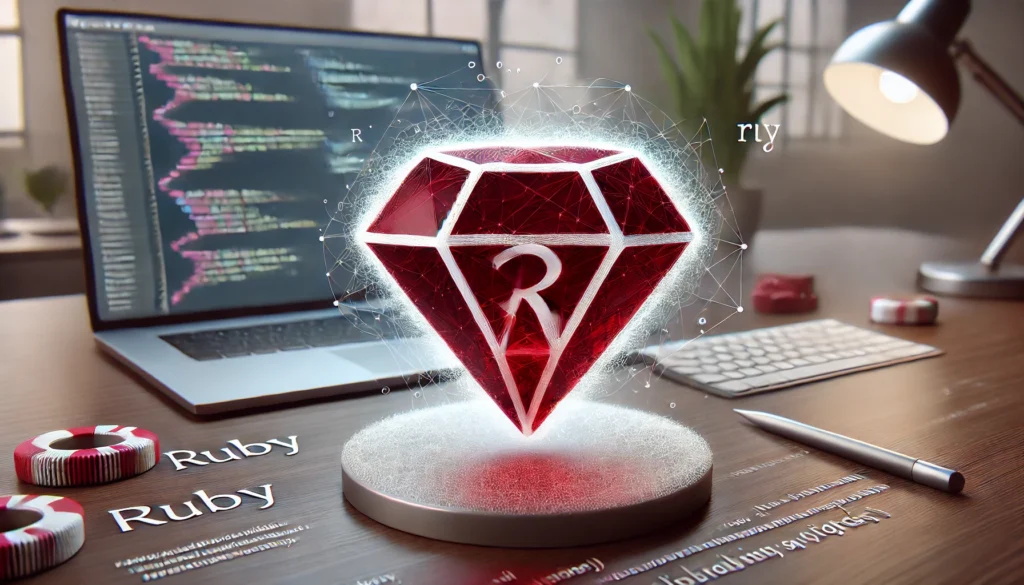
- Explain Ruby’s mixins.
- Modules included in classes to add functionality (e.g.,
include Enumerable
).
- Modules included in classes to add functionality (e.g.,
- What is the difference between
puts
,print
, andp
?puts
adds a newline,print
doesn’t, andp
inspects the object.
- How do you handle exceptions in Ruby?
- Using
begin-rescue-ensure-end
blocks.
- Using
- What is a Proc in Ruby?
- A reusable block stored as an object (
Proc.new { |x| x * 2 }
).
- A reusable block stored as an object (
- What is RVM?
- Ruby Version Manager for managing multiple Ruby installations.
- How does Ruby support multiple inheritance?
- Via mixins (modules) since it doesn’t support multiple inheritance directly.
- What is the purpose of
self
in Ruby?- Refers to the current object or class context.
- What are frozen strings in Ruby?
- Immutable strings (
str.freeze
) to optimize memory.
- Immutable strings (
- How do you iterate over a hash in Ruby?
hash.each { |key, value| puts "#{key}: #{value}" }
- What is the difference between
==
and===
in Ruby?==
checks equality,===
checks case equality (used incase
statements).
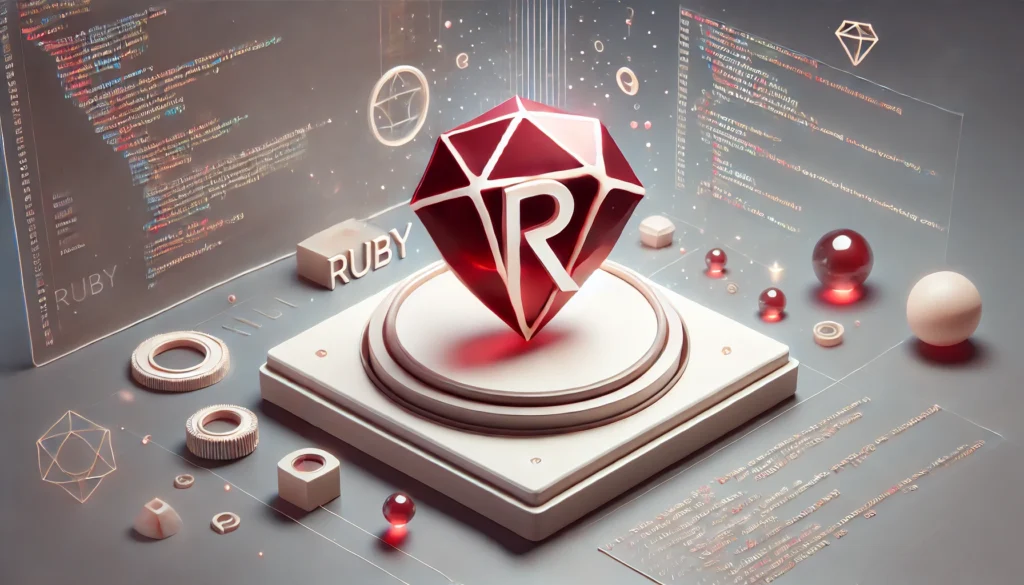
- What is Ruby?
- Ruby is a dynamic, object-oriented scripting language designed for simplicity and productivity.
- Who created Ruby?
- Yukihiro “Matz” Matsumoto in 1995.
- What is IRB in Ruby?
- Interactive Ruby Shell, a REPL for executing Ruby commands.
- Explain Ruby’s principle of “least surprise.”
- Ruby behaves intuitively, minimizing confusion for developers.
- What are Ruby gems?
- Libraries or plugins that extend Ruby’s functionality.
- How does Ruby handle garbage collection?
- Automatically manages memory using a mark-and-sweep algorithm.
- What is the difference between
nil
andfalse
in Ruby?nil
represents absence, whilefalse
is a Boolean value.
- What are symbols in Ruby?
- Immutable, memory-efficient identifiers prefixed with a colon (
:symbol
).
- Immutable, memory-efficient identifiers prefixed with a colon (
- How do you define a class in Ruby?
class MyClass; end
- What is the use of
yield
in Ruby?- Invokes a block passed to a method.
- Explain Ruby’s mixins.
- Modules included in classes to add functionality (e.g.,
include Enumerable
).
- Modules included in classes to add functionality (e.g.,
- What is the difference between
puts
,print
, andp
?puts
adds a newline,print
doesn’t, andp
inspects the object.
- How do you handle exceptions in Ruby?
- Using
begin-rescue-ensure-end
blocks.
- Using
- What is a Proc in Ruby?
- A reusable block stored as an object (
Proc.new { |x| x * 2 }
).
- A reusable block stored as an object (
- What is RVM?
- Ruby Version Manager for managing multiple Ruby installations.
- How does Ruby support multiple inheritance?
- Via mixins (modules) since it doesn’t support multiple inheritance directly.
- What is the purpose of
self
in Ruby?- Refers to the current object or class context.
- What are frozen strings in Ruby?
- Immutable strings (
str.freeze
) to optimize memory.
- Immutable strings (
- How do you iterate over a hash in Ruby?
hash.each { |key, value| puts "#{key}: #{value}" }
- What is the difference between
==
and===
in Ruby?==
checks equality,===
checks case equality (used incase
statements).
- What is a lambda in Ruby?
- A stricter Proc that checks argument count (
lambda { |x| x * 2 }
).
- A stricter Proc that checks argument count (
- How do you define a singleton method in Ruby?
def object.method_name; end
- What is the
super
keyword in Ruby?- Calls the parent class’s method of the same name.
- Explain Ruby’s
attr_accessor
,attr_reader
, andattr_writer
.- Auto-generates getters/setters for instance variables.
- What is duck typing in Ruby?
- Focuses on an object’s behavior rather than its type.
- How do you load a Ruby file?
require 'filename'
orload 'filename.rb'
- What is the difference between
private
andprotected
methods?private
restricts access to the instance,protected
allows same-class access.
- What is
$LOAD_PATH
in Ruby?- Stores directories where Ruby looks for required files.
- How do you create a thread in Ruby?
Thread.new { puts "Hello" }
- What is metaprogramming in Ruby?
- Writing code that generates or modifies code at runtime.
- How does
respond_to?
work in Ruby?- Checks if an object can execute a method (
obj.respond_to?(:method)
).
- Checks if an object can execute a method (
- What is the
Enumerable
module in Ruby?- Provides traversal and search methods like
map
,select
, andreduce
.
- Provides traversal and search methods like
- How do you define a constant in Ruby?
- Uppercase name (
CONST = 10
).
- Uppercase name (
- What is the
||=
operator in Ruby?- Conditional assignment (
x ||= 5
assigns ifx
isnil
orfalse
).
- Conditional assignment (
- What is
Struct
in Ruby?- A shortcut for creating simple data classes (
Person = Struct.new(:name, :age)
).
- A shortcut for creating simple data classes (
- How do you handle file I/O in Ruby?
File.open("file.txt", "r") { |file| file.read }
- What is the difference between
String#gsub
andString#sub
?gsub
replaces all occurrences,sub
replaces the first.
- How do you sort a hash by value in Ruby?
hash.sort_by { |k, v| v }
- What is
Kernel#eval
in Ruby?- Executes a string as Ruby code (use cautiously).
- How do you implement a singleton class in Ruby?
require 'singleton'
andinclude Singleton
in the class.
- What is the
tap
method in Ruby?- Yields self to a block and returns self (
obj.tap { |o| o.x = 1 }
).
- Yields self to a block and returns self (
- How do you memoize a method in Ruby?
@var ||= expensive_operation
- What is the
%w
syntax in Ruby?- Creates an array of strings (
%w(apple banana)
→["apple", "banana"]
).
- Creates an array of strings (
- How do you define a class method in Ruby?
def self.method_name; end
- What is
method_missing
in Ruby?- Handles calls to undefined methods dynamically.
- How do you flatten a nested array in Ruby?
array.flatten
- What is the
<=>
(spaceship) operator in Ruby?- Compares two objects (-1, 0, or 1 for sorting).
- How do you benchmark code in Ruby?
Benchmark.measure { code_to_test }
- What is
ActiveSupport
in Ruby on Rails?- A collection of utility methods and extensions.
- How do you write a DSL (Domain-Specific Language) in Ruby?
- Using metaprogramming and method chaining (e.g., RSpec).
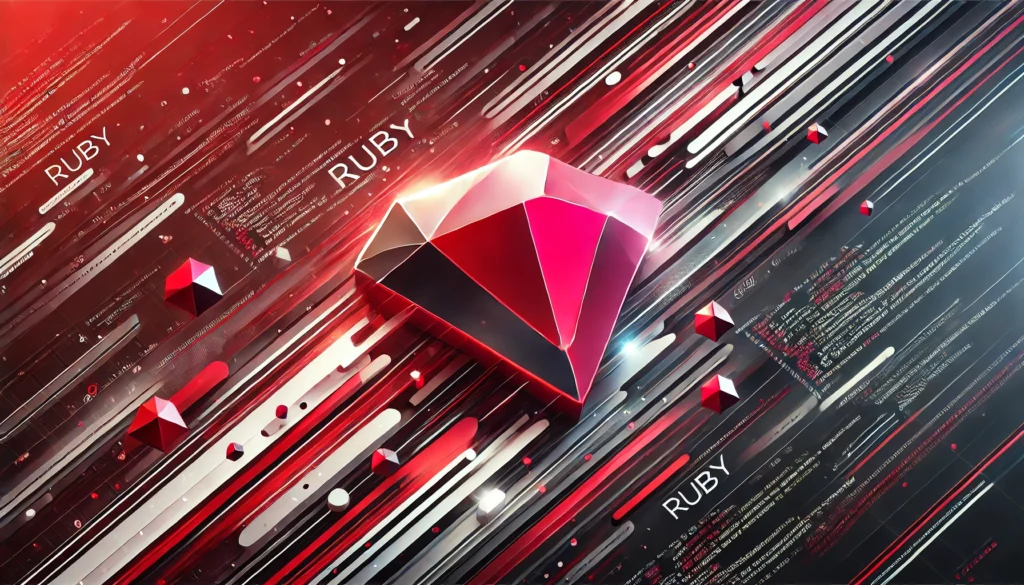
Conclusion
Mastering Ruby requires a deep understanding of its object-oriented principles, metaprogramming capabilities, and idiomatic syntax. Interviewers often assess candidates on core concepts like blocks, modules, and exception handling, as well as practical skills like debugging and performance optimization. A strong grasp of Ruby’s ecosystem—gems, Rails, and testing frameworks—demonstrates readiness for real-world development.
Beyond syntax, successful Ruby developers emphasize clean, maintainable code and familiarity with design patterns. Questions on memory management, concurrency, and algorithmic problem-solving test a candidate’s ability to write efficient Ruby applications. Practicing with open-source projects or coding challenges can solidify these skills.
Ultimately, Ruby interviews evaluate both technical proficiency and problem-solving creativity. Whether you’re applying for a backend role or a full-stack position, showcasing your ability to leverage Ruby’s elegance and power will set you apart. Continuous learning and hands-on coding remain the best preparation for acing Ruby interviews.
Curated for you