Node.js: Powering Modern Web Development
Node.js is an open-source, cross-platform JavaScript runtime environment built on Chrome’s V8 engine. It enables developers to execute JavaScript code server-side, making it ideal for building scalable network applications. Unlike traditional server-side languages, Node.js uses an event-driven, non-blocking I/O model, which optimizes throughput and efficiency for real-time applications like chat platforms, streaming services, and APIs. Its single-threaded event loop architecture handles multiple concurrent clients without creating separate threads, reducing resource overhead. Node.js is widely adopted due to its lightweight nature, npm (Node Package Manager) ecosystem with over a million packages, and seamless integration with modern web frameworks like Express.js and NestJS.
Key Features and Use Cases
Node.js excels in asynchronous programming, allowing tasks like file operations, database queries, or API calls to run in the background without blocking the main thread. This makes it suitable for high-traffic applications requiring real-time data processing. Developers leverage Node.js for building RESTful APIs, microservices architectures, and single-page applications (SPAs). It also supports full-stack JavaScript development, enabling code reuse between frontend and backend. Popular tools like Webpack, Socket.IO, and GraphQL libraries integrate smoothly with Node.js. Its cross-platform compatibility ensures applications run seamlessly on Windows, Linux, and macOS. Companies like Netflix, LinkedIn, and Uber use Node.js to enhance performance and scalability.
Ecosystem and Best Practices
The Node.js ecosystem thrives on community-driven modules, frameworks, and tools. Express.js simplifies routing and middleware management, while NestJS offers a modular structure for enterprise-grade apps. Developers prioritize error handling, security practices (e.g., input validation, using Helmet.js), and performance optimization (clustering, caching). Debugging tools like Node Inspector and logging libraries (Winston, Morgan) streamline troubleshooting. With the rise of serverless computing, platforms like AWS Lambda and Vercel support Node.js for event-driven functions. Regular updates, adherence to ES6+ features, and leveraging TypeScript for type safety ensure robust codebases.
Top 50 Node.js Technical Interview Questions & Answers
- What is Node.js?
A JavaScript runtime built on Chrome’s V8 engine, enabling server-side scripting with non-blocking I/O. - Explain the event loop.
A single-threaded loop that processes asynchronous callbacks, handling concurrent operations efficiently. - Blocking vs. non-blocking code?
Blocking code halts execution until a task completes; non-blocking code allows other tasks to run meanwhile. - What is npm?
Node Package Manager: a tool for installing, managing, and sharing JavaScript packages. - Purpose of
package.json
?
Stores project metadata, dependencies, scripts, and configuration details.
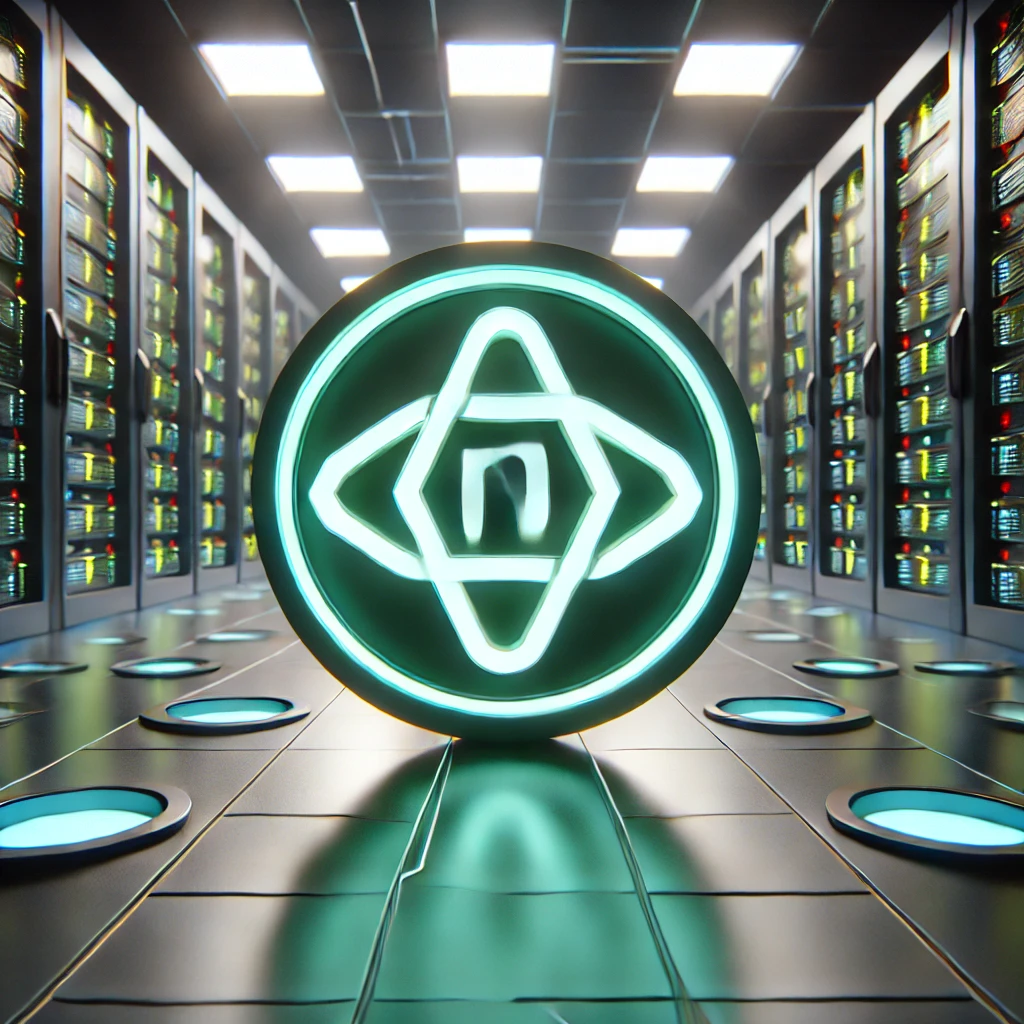
- What are streams in Node.js?
Objects for handling reading/writing data sequentially, useful for large datasets (e.g.,fs.createReadStream()
). - Explain clustering in Node.js.
Thecluster
module creates child processes (workers) to utilize multi-core systems, improving performance. - How to handle errors in Node.js?
Usetry/catch
,.catch()
for promises, or error-first callbacks (e.g.,function(err, data)
). - What is middleware?
Functions that execute during a request-response cycle, modifying req/res objects (e.g., Express.js middleware). - RESTful API best practices?
Use HTTP methods (GET, POST), status codes, JSON responses, and versioning (e.g.,/api/v1/resource
). - What is the
require()
function?
Imports modules, JSON files, or local files into the current script. - Global objects in Node.js?
__dirname
,__filename
,process
,console
, andglobal
. - What is REPL?
Read-Eval-Print Loop: interactive shell for executing JavaScript commands. - How to manage environment variables?
Useprocess.env
with packages likedotenv
for loading.env
files. - Difference between
exports
andmodule.exports
?exports
is a reference tomodule.exports
; reassigningexports
breaks the reference. - What is the EventEmitter class?
A core module for handling custom events using.on()
and.emit()
. - Explain callback hell.
Nested callbacks leading to unreadable code; solved using Promises or async/await. - What is a Promise?
An object representing the eventual completion (or failure) of an asynchronous operation. - How does
async/await
work?
Syntactic sugar over Promises;await
pauses execution until a Promise resolves. - What is a Buffer?
A class for handling binary data, useful for file operations or network streams.
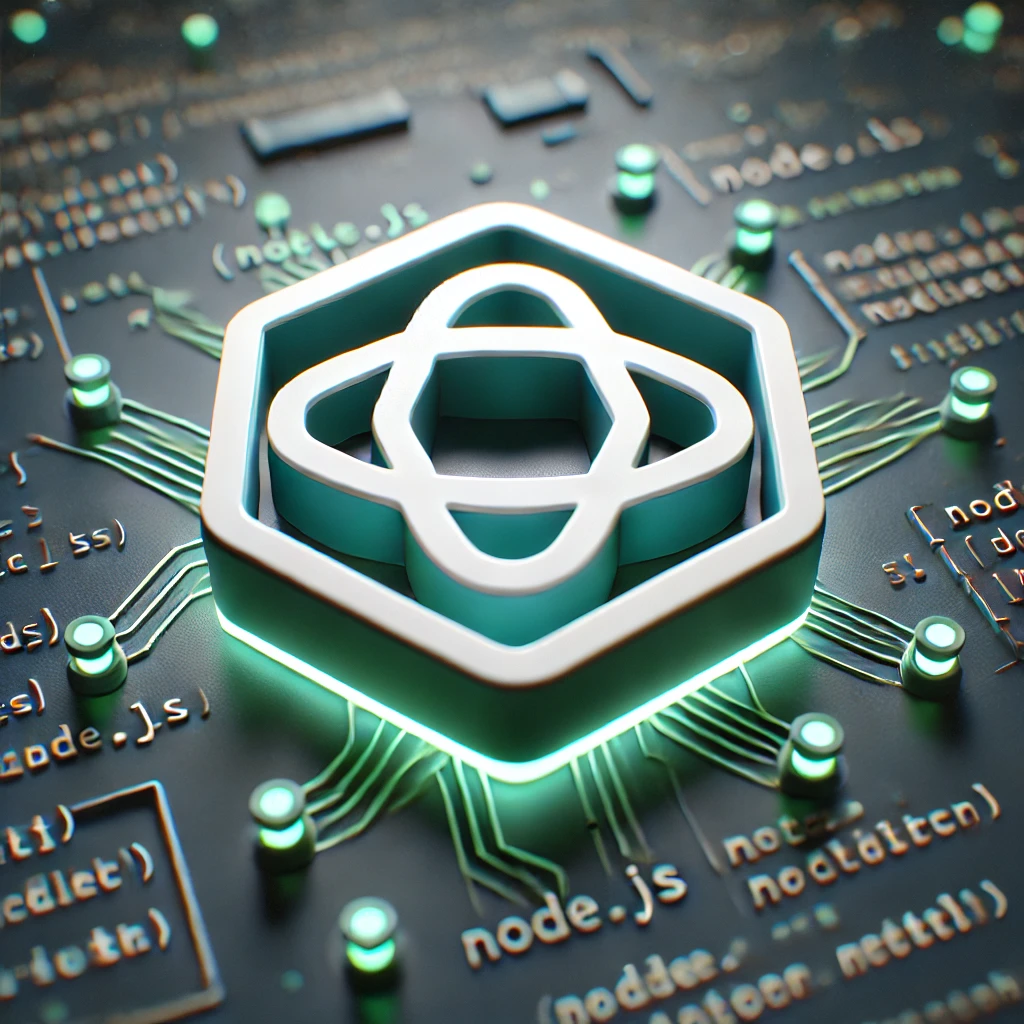
- How to connect Node.js with MongoDB?
Use Mongoose ODM or the native MongoDB driver. - What is JWT?
JSON Web Token: a compact, URL-safe token for secure authentication/authorization. - Explain CORS.
Cross-Origin Resource Sharing: a security feature to restrict cross-domain requests. - What is a REST API?
An architectural style using HTTP methods to perform CRUD operations on resources. - How to secure an Express.js app?
Use Helmet.js, sanitize inputs, rate limiting, and HTTPS. - What is a singleton pattern?
A design pattern ensuring a class has only one instance. - What is the purpose of
next()
in Express?
Passes control to the next middleware function. - How to debug Node.js apps?
Useconsole.log()
,debugger
, or tools like Node Inspector and Chrome DevTools. - What is NPM scripting?
Automating tasks (e.g., testing, builds) viascripts
inpackage.json
. - Explain the module wrapper function.
Node.js wraps modules in a function to providerequire
,module
, etc. - What is a stub?
A function simulating component behavior during testing. - How to handle file uploads?
Use middleware like Multer in Express.js. - What is a child process?
A process created by a parent process usingspawn()
,exec()
, orfork()
. - Difference between
process.nextTick()
andsetImmediate()
?nextTick()
executes before the next event loop iteration;setImmediate()
after. - What is a reverse proxy?
A server forwarding client requests to backend servers (e.g., Nginx with Node.js). - What is GraphQL?
A query language for APIs, allowing clients to request specific data. - How to implement caching?
Use Redis or in-memory caching with packages likenode-cache
. - Explain middleware chaining.
Multiple middleware functions executed sequentially viaapp.use()
or route-specific methods. - What is the purpose of
app.listen()
?
Binds and listens for connections on a specified port. - How to structure a Node.js project?
Use MVC pattern, separate routes, controllers, models, and config files. - What is WebSocket?
A protocol enabling real-time, bidirectional communication (e.g., via Socket.IO). - How to prevent memory leaks?
Avoid global variables, remove event listeners, and monitor heap usage. - What is the
fs
module?
A core module for file system operations (read/write files, directories). - How to implement authentication?
Use Passport.js, OAuth, or JWT with bcrypt for password hashing. - What is Babel?
A tool transpiling modern JavaScript (ES6+) into backward-compatible versions. - Explain the Observer pattern.
A design pattern where objects (observers) watch and react to state changes. - What is load balancing?
Distributing network traffic across multiple servers to optimize performance. - How to use environment variables?
Access viaprocess.env
and load usingdotenv.config()
. - What is PM2?
A process manager for Node.js apps, supporting clustering and auto-restarts. - Testing frameworks in Node.js?
Mocha, Jest, and Jasmine for unit/integration testing; Supertest for API testing.
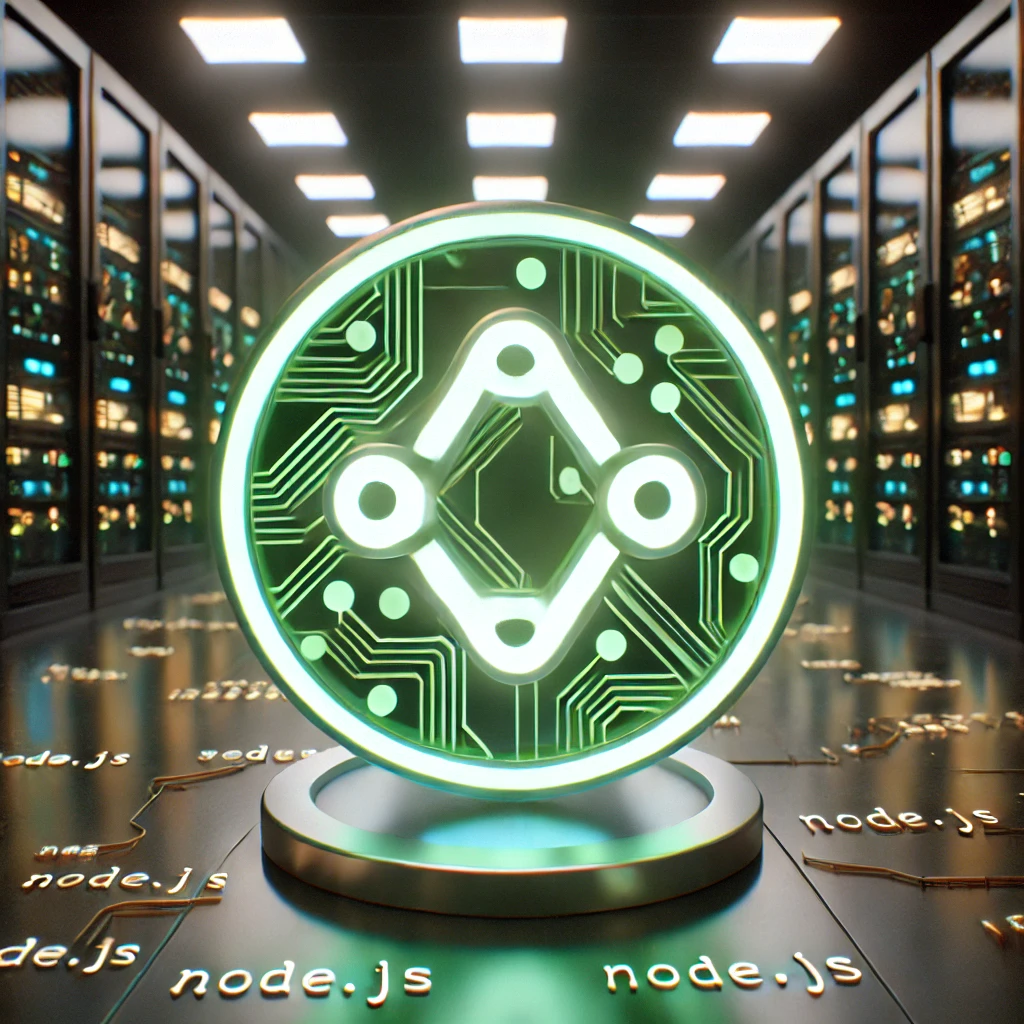
Conclusion on Node.js
As of 2025, Node.js remains a dominant force in backend development, powering countless web applications, APIs, and microservices. Its asynchronous, event-driven architecture continues to provide exceptional scalability and efficiency, making it a top choice for real-time applications like chat platforms, IoT solutions, and cloud-based services.
With ongoing improvements in performance, security, and native ECMAScript module support, Node.js has further solidified its role in modern development stacks. The growing ecosystem of packages, enhanced by tools like Deno and Bun, has expanded JavaScript’s backend capabilities, ensuring developers have access to cutting-edge features.
Despite competition from emerging runtimes and alternative frameworks, Node.js maintains its relevance due to its vast community, enterprise adoption, and integration with serverless computing. As it continues to evolve, Node.js remains a reliable and future-proof solution for scalable, high-performance applications in 2025 and beyond.
Cracking the interview is tricky as you must know all the key technical aspects of this. Node is a successful JS framework and as such you must get yourself familiar to actual example implementation and key articulation process for node. Good Luck!
Curated Reads: