JavaScript is the Backbone of Modern Web Development. Here in this article we have a look at the top 50 JavaScript technical interview questions with their answers. JavaScript is a versatile, high-level programming language that powers interactive and dynamic web applications. Initially created to add interactivity to static HTML pages, it has evolved into a full-fledged ecosystem used for front-end, back-end (Node.js), mobile (React Native), and even desktop app development. Its event-driven, non-blocking architecture makes it ideal for real-time applications like chat platforms and gaming. With frameworks like React, Angular, and Vue.js, JavaScript continues to dominate web development, offering scalability and cross-platform compatibility.
One of JavaScript’s key strengths is its asynchronous capabilities, enabling features like AJAX calls and Promises/Async-Await for seamless data fetching without page reloads. The language follows prototypal inheritance, unlike classical inheritance in Java or C++, making it unique yet powerful. Modern JavaScript (ES6+) introduces arrow functions, destructuring, modules, and classes, enhancing readability and maintainability. Its vast npm (Node Package Manager) repository provides countless libraries, making development faster and more efficient.
Despite its flexibility, JavaScript has quirks like hoisting, closures, and type coercion, which can lead to unexpected behaviors if not understood properly. However, its widespread adoption, active community, and continuous updates (ECMAScript standards) ensure it remains a must-learn language for developers. Whether building a simple website or a complex enterprise application, JavaScript’s adaptability and performance make it indispensable in today’s tech landscape.
Top 50 JavaScript Technical Interview Questions & Answers
- What is JavaScript?
A scripting language used to create dynamic web content. - Difference between
let
,const
, andvar
?var
is function-scoped,let
andconst
are block-scoped.const
cannot be reassigned. - Explain closures.
A function that retains access to its outer scope even after execution. - What is hoisting?
Variable/function declarations are moved to the top of their scope during compilation. - What is the
this
keyword?
Refers to the object it belongs to (context-dependent). - Explain event bubbling.
Events propagate from the target element up to the root. - What are Promises?
Objects representing eventual completion/error of an async operation. - Difference between
==
and===
?==
checks value (with coercion),===
checks value and type. - What is async/await?
Syntactic sugar for Promises, making async code look synchronous. - What is the DOM?
Document Object Model, a tree representation of HTML.
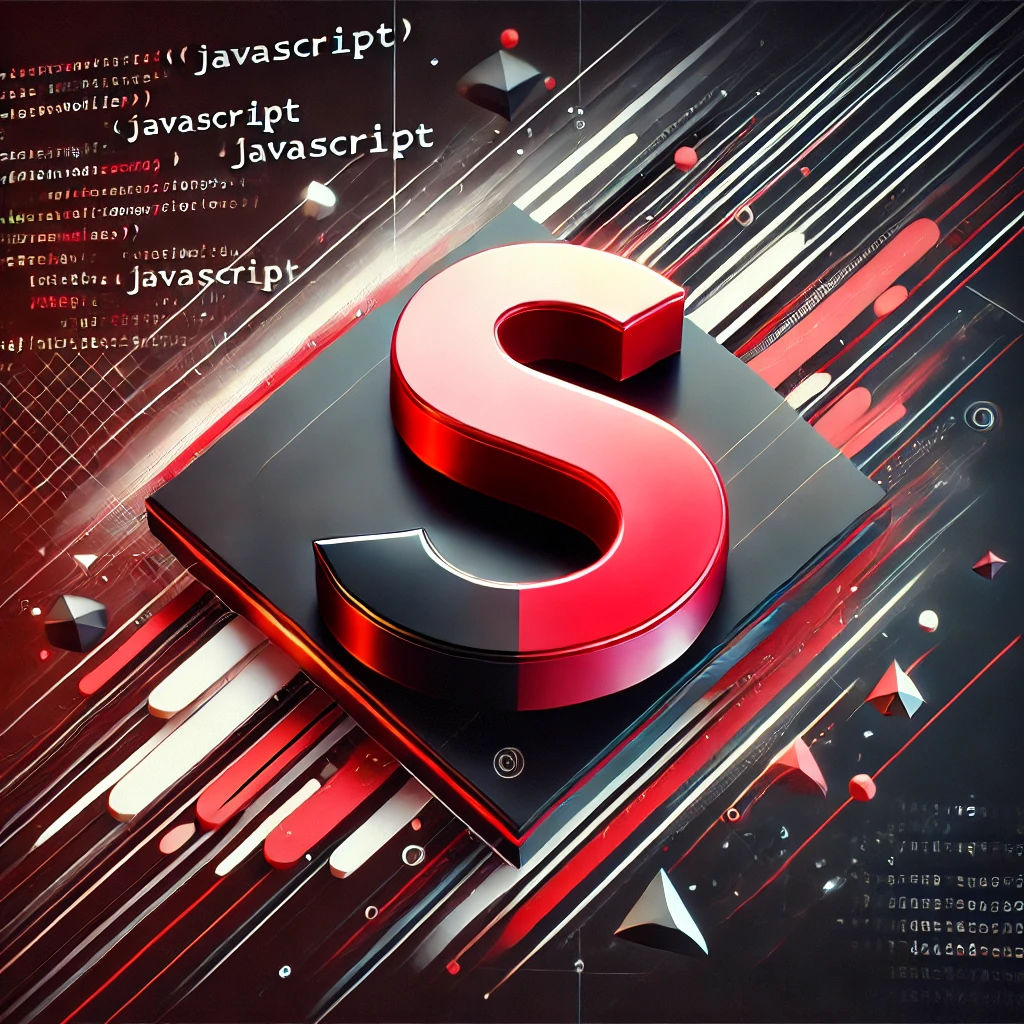
- Explain callback hell.
Nested callbacks leading to unreadable code. Solved with Promises/async-await. - What is a pure function?
A function that always returns the same output for the same input. - What is REST API?
An architectural style for networked applications using HTTP methods. - What is JSON?
Lightweight data interchange format (JavaScript Object Notation). - Explain prototypal inheritance.
Objects inherit properties/methods from prototypes. - What is
localStorage
?
Web storage for persisting data beyond page sessions. - What is a higher-order function?
A function that takes/returns another function. - What is the spread operator?
...
expands iterables into individual elements. - What is CORS?
Cross-Origin Resource Sharing, a security feature. - What is a IIFE?
Immediately Invoked Function Expression:(function(){})()
. - What is memoization?
Caching function results to optimize performance. - What is the event loop?
Handles async callbacks in JavaScript’s single-threaded model. - Difference between
null
andundefined
?null
is intentional absence,undefined
is uninitialized. - What is a constructor function?
A function used to create objects withnew
. - What is Babel?
A JS compiler that converts ES6+ to backward-compatible code. - What is a promise chain?
Multiple.then()
calls handling sequential async operations. - What is
bind()
?
Creates a new function with a fixedthis
value. - What is a generator function?
Functions that can be paused/resumed usingyield
. - What is the difference between
forEach
andmap
?map
returns a new array;forEach
doesn’t. - What is debouncing?
Delaying function execution until after a pause in events. - What is the purpose of
use strict
?
Enforces stricter parsing/error handling in JS. - What is a WeakMap?
A collection with weakly referenced keys (garbage-collected). - What is currying?
Transforming a function to take arguments one at a time. - What is the difference between
slice
andsplice
?slice
returns a portion;splice
modifies the array. - What is a service worker?
A script running in the background for offline functionality. - What is the difference between
call
andapply
?call
takes arguments separately;apply
takes an array.
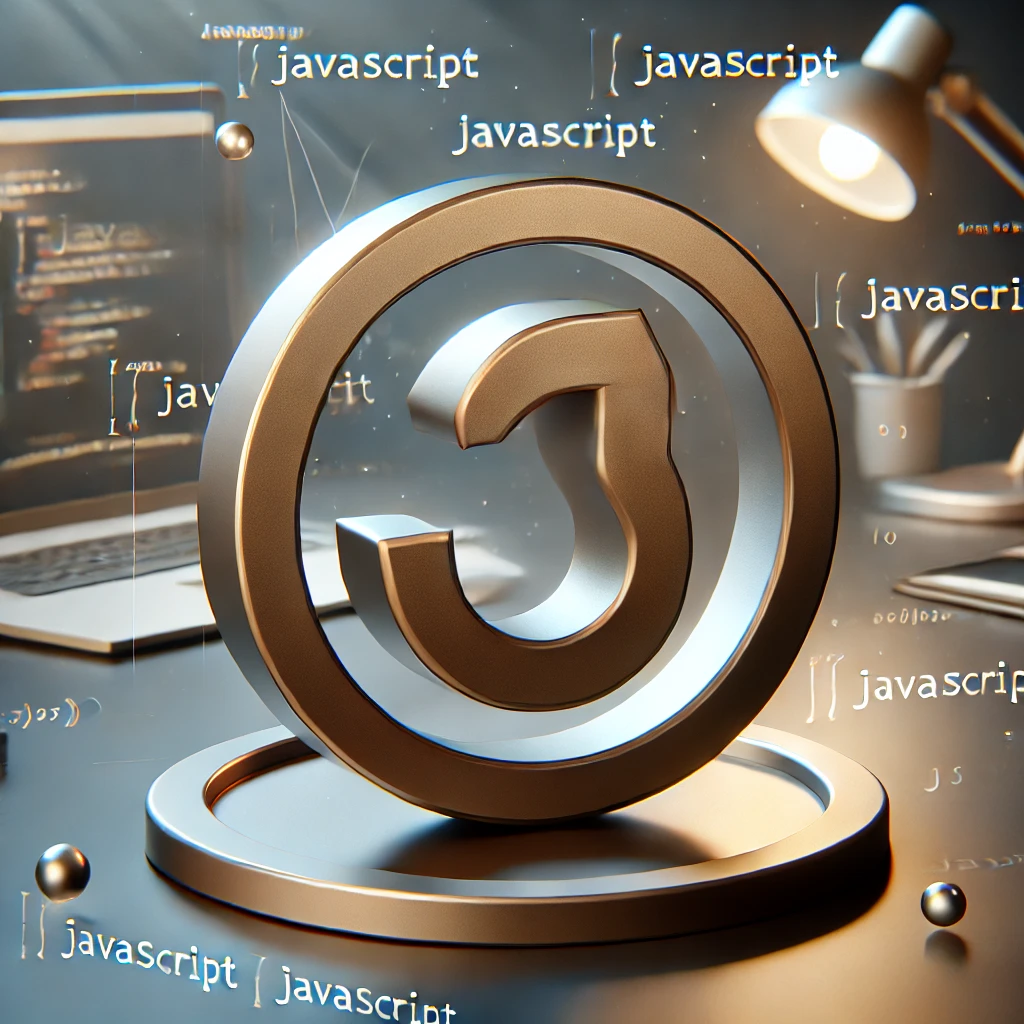
- What is a Proxy in JavaScript?
An object wrapping another object to intercept operations. - What is the purpose of
Object.freeze()
?
Prevents modification of an object’s properties. - What is the Temporal Dead Zone?
The phase wherelet
/const
variables are inaccessible before declaration. - What is Webpack?
A module bundler for JavaScript applications. - What is a tagged template literal?
A function parsing template literals (e.g.,html``...
). - What is the difference between
class
andprototype
?class
is syntactic sugar over prototypal inheritance. - What is a Web Component?
Reusable custom HTML elements with encapsulated functionality. - What is the difference between
push
andconcat
?push
modifies the array;concat
returns a new array. - What is the purpose of
Symbol
?
Creates unique, immutable identifiers. - What is a state management library?
Tools like Redux for managing application state. - What is the purpose of
Array.reduce()
?
Reduces an array to a single value via a callback. - What is a WebSocket?
A protocol for real-time, bidirectional communication. - What is tree shaking?
Eliminating unused code during bundling. - What is TypeScript?
A typed superset of JavaScript for large-scale apps.
Conclusion: Mastering JavaScript for Technical Interviews
JavaScript remains a cornerstone of modern web development, making it a critical skill for any developer. Excelling in a JavaScript interview requires a deep understanding of core concepts like closures, hoisting, prototypal inheritance, and asynchronous programming. By mastering these fundamentals, along with modern ES6+ features, you demonstrate not only technical proficiency but also the ability to write efficient, scalable code. Employers look for candidates who can explain these concepts clearly and apply them to real-world problems, so practice is key.
Beyond theory, hands-on experience with frameworks like React, Angular, or Vue.js, as well as backend technologies like Node.js, can set you apart. Interviewers often test problem-solving skills through coding challenges, algorithmic questions, and system design scenarios. Familiarity with tools like Webpack, Babel, and testing libraries (Jest, Mocha) further strengthens your profile. Additionally, understanding performance optimization, security best practices, and debugging techniques shows that you can build robust applications.
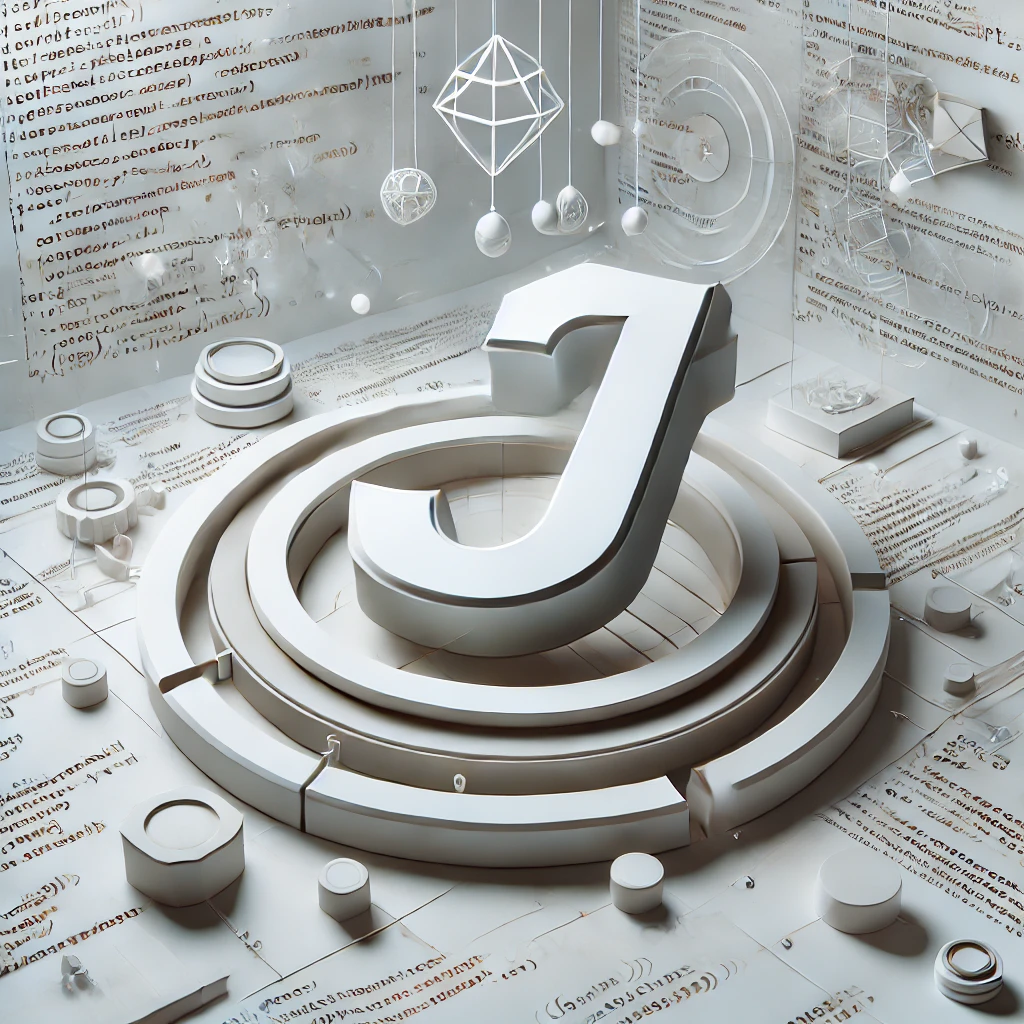
Finally, confidence and communication are just as important as technical knowledge. Being able to articulate your thought process, walk through solutions step-by-step, and adapt to feedback will leave a lasting impression. Stay updated with the latest JavaScript trends, contribute to open-source projects, and practice mock interviews to refine your skills. With dedication and the right preparation, you can ace your JavaScript interview and advance your career in web development.
Curated Reads