Go, also known as Golang, is an open-source programming language developed by Google engineers Robert Griesemer, Rob Pike, and Ken Thompson in 2007. Designed to address the challenges of modern software development, Go emphasizes simplicity, efficiency, and readability. Its minimalist syntax eliminates unnecessary complexity, making it ideal for building scalable, high-performance systems. With built-in support for concurrency through goroutines and channels, Go simplifies parallel processing, enabling developers to create efficient networked and distributed applications.
Go’s robust standard library and powerful tooling—such as the go command for dependency management, testing, and cross-compilation—streamline development workflows. The language’s static typing and compilation ensure type safety and fast execution, while garbage collection automates memory management. These features have made Go a favorite for cloud-native applications, microservices, and DevOps tools. Companies like Docker, Kubernetes, and Netflix rely on Go for its ability to handle large-scale, concurrent workloads with minimal overhead.
Over the years, Go has gained traction for its balance between performance and productivity. Its growing ecosystem, active community, and compatibility with modern architectures position it as a go-to language for backend services, CLI tools, and APIs. By prioritizing developer experience and system efficiency, Go bridges the gap between low-level control and high-level abstraction, empowering teams to deliver reliable software in less time.
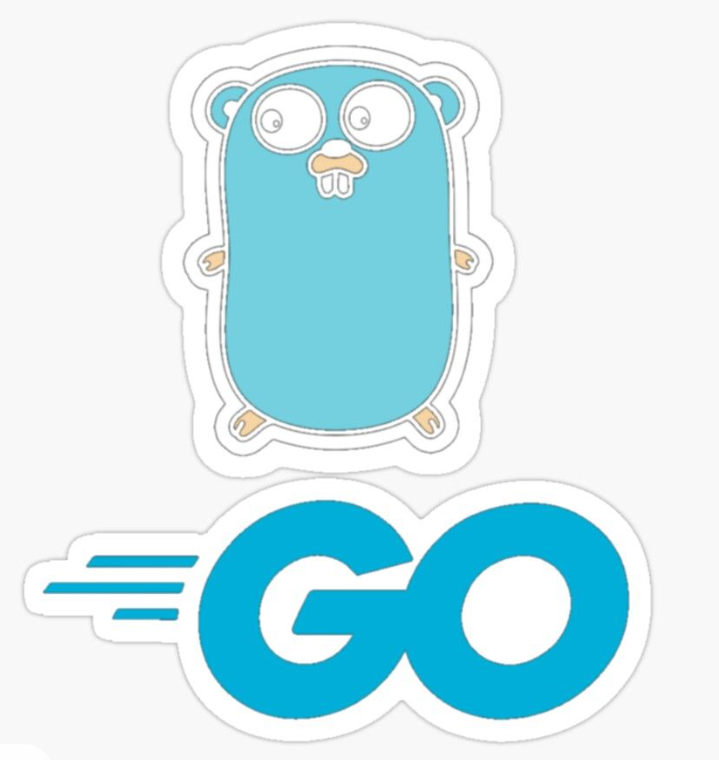
Interview Questions & Answers
- What is Go? Explain its key features.
Go is an open-source, statically typed, compiled language designed for simplicity and efficiency. Key features include garbage collection, concurrency via goroutines/channels, fast compilation, and strong standard libraries. - What is the difference between var and const?
var declares variables whose values can change, while const declares immutable constants. - What is a goroutine?
A goroutine is a lightweight thread managed by the Go runtime, enabling concurrent execution. - How do you create a goroutine?
Prefix a function call with the go keyword: go myFunction(). - What are channels in Go?
Channels are typed conduits for communication between goroutines using <- operator. - Buffered vs. unbuffered channels?
Buffered channels hold a limited number of values without blocking. Unbuffered channels block until sender/receiver synchronize. - How does error handling work in Go?
Errors are returned as values, typically as the last return parameter. Use errors.New or fmt.Errorf to create errors. - Explain the defer keyword.
defer delays a function’s execution until the surrounding function returns, often used for cleanup. - What is an interface in Go?
An interface defines a set of method signatures. Types implicitly implement interfaces by implementing all methods. - Difference between new and make?
new(T) allocates memory for type T and returns a pointer. make initializes slices, maps, or channels (reference types). - What is a struct?
A struct is a user-defined composite type containing named fields of different types. - What is the zero value in Go?
Variables declared without explicit initialization are set to their zero value (e.g., 0 for integers, nil for pointers). - Method vs. function in Go?
A method is a function with a receiver argument, associating it with a type. - How are pointers used in Go?
Pointers store memory addresses. Use * to declare and & to reference addresses.
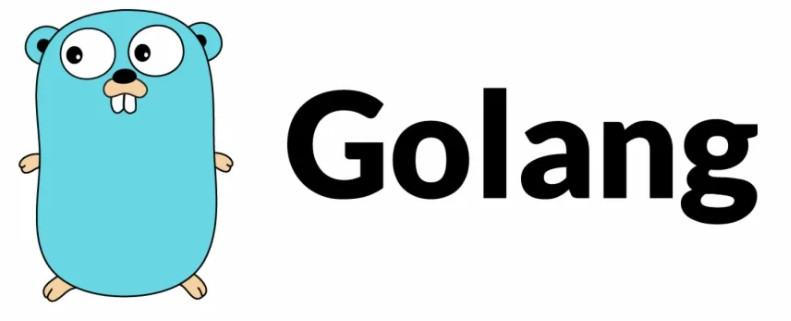
- How to initialize a package?
Use the init() function, which runs automatically when the package is imported. - Rune vs. byte in Go?
A byte is an alias for uint8, while a rune represents a Unicode code point (alias for int32). - How does Go implement composition?
Go uses struct embedding to compose types, promoting fields/methods of embedded structs. - Explain garbage collection in Go.
Go uses a concurrent mark-and-sweep collector to automatically manage memory. - How to write tests in Go?
Create _test.go files with functions prefixed TestXxx, using the testing package. - What is an empty interface (interface{})?
An empty interface can hold values of any type, often used for generic handling (e.g., fmt.Println). - What is a type assertion?
A type assertion checks if an interface value holds a specific type: val, ok := myVar.(int). - What are variadic functions?
Functions accepting a variable number of arguments via … syntax (e.g., func sum(nums …int)). - Purpose of the context package?
The context package manages cancellation, deadlines, and request-scoped values across goroutines. - How to create an HTTP server in Go?
Use http.ListenAndServe() with handlers defined via http.HandleFunc or by implementing http.Handler. - Slices vs. arrays?
Arrays have fixed size, while slices are dynamically sized views into arrays. - How to safely use maps?
Use synchronization (e.g., mutexes) for concurrent access. Maps are not thread-safe by default. - What is sync.Mutex?
A mutual exclusion lock to prevent race conditions by controlling access to shared resources. - Explain sync.WaitGroup.
A WaitGroup waits for a collection of goroutines to finish using Add(), Done(), and Wait(). - What is the select statement?
select allows a goroutine to wait on multiple channel operations, executing the first ready case. - How to handle panics?
Use recover() in a deferred function to catch panics and restore control. - What are Go modules?
Modules are dependency management units with go.mod defining dependencies and versions. - How to cross-compile in Go?
Set GOOS and GOARCH environment variables (e.g., GOOS=linux GOARCH=amd64 go build). - What is a method set?
A method set is the collection of methods attached to a type, determining interface implementation. - What is variable shadowing?
Shadowing occurs when a variable in a block has the same name as one in an outer scope. - Order of init() execution?
init() functions run after package variables are initialized and before main(). - How to serialize structs to JSON?
Use json.Marshal(), with struct tags (json:”fieldName”) to customize field names. - What is a closure?
A closure is a function that references variables from outside its body. - How to compare interfaces?
Interfaces are compared by their dynamic type and value. Use reflection for deep equality checks.
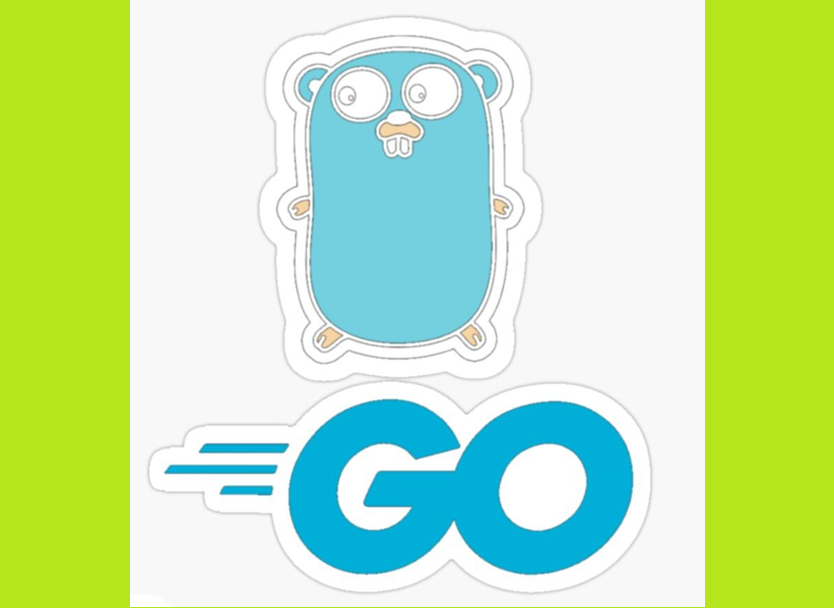
- How to detect race conditions?
Run tests with -race flag: go test -race. - When to use switch vs. if-else?
switch improves readability when checking multiple values or conditions. - What is a type switch?
A type switch compares the type of an interface value: switch v := x.(type) { case int: … }. - What is reflection in Go?
Reflection via the reflect package allows inspection of types and values at runtime. - GOPATH vs. Go modules?
GOPATH was the old workspace system. Modules (post Go 1.11) manage dependencies in a project-centric way. - How to import third-party packages?
Use go get or include the package in go.mod; imports are referenced via their module path. - How to make an HTTP request?
Use http.Get() or create a custom http.Client and http.NewRequest(). - When are defer arguments evaluated?
Arguments are evaluated immediately, but the deferred function executes later. - How to chain methods in Go?
Return the receiver from methods to enable chaining: func (c *Car) SetColor() *Car { …; return c }. - What is embedding an interface?
Embedding an interface in another includes its methods, promoting composition. - How to avoid cyclic imports?
Refactor shared code into a separate package or use interface definitions in a neutral package. - Difference between http.Handler and http.HandlerFunc?
http.Handler is an interface with ServeHTTP(). http.HandlerFunc adapts a function to implement Handler.
Conclusion on Go Language
Go’s rise in popularity underscores its effectiveness in solving real-world engineering problems. Its focus on simplicity, concurrency, and performance addresses the demands of today’s distributed systems and cloud computing environments. By reducing boilerplate code and offering intuitive concurrency primitives, Go enables developers to write maintainable, efficient software without sacrificing speed or scalability.
The language’s thriving community and comprehensive toolchain further enhance its appeal. Features like Go modules for dependency management, integrated testing frameworks, and cross-platform compilation simplify project maintenance. Additionally, Go’s compatibility with web assembly (WASM) and its use in cutting-edge fields like blockchain and AI highlight its adaptability to emerging technologies.
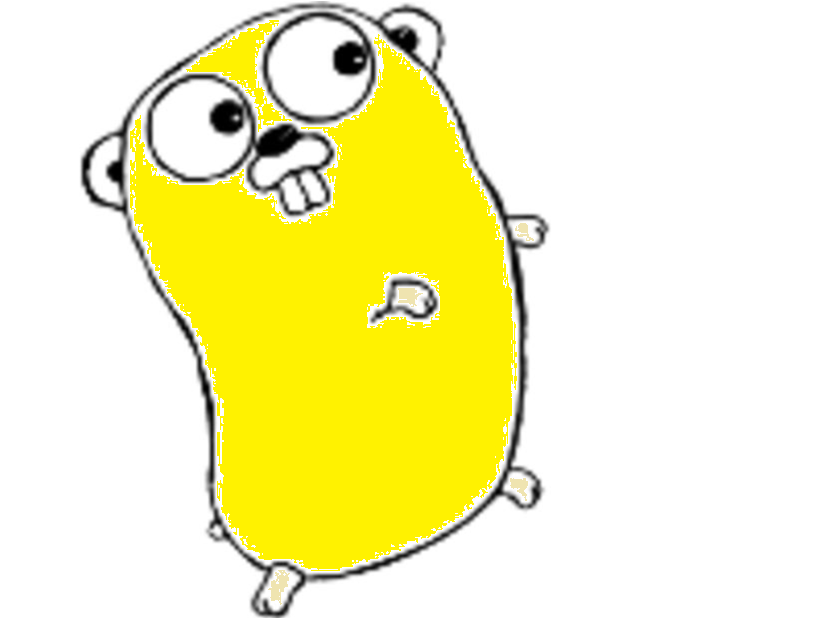
As software systems grow increasingly complex, Go’s design philosophy—simplicity as a core tenet—ensures its longevity. Whether for startups or tech giants, Go provides a pragmatic foundation for building resilient, high-performance applications. With continuous improvements from the Go team and widespread industry adoption, the language is poised to remain a critical tool in the modern developer’s arsenal.
Curated Reads