Introduction to C++
C++ is a powerful, high-performance programming language that builds upon the foundation of C while introducing object-oriented programming (OOP) capabilities. Developed by Bjarne Stroustrup in the early 1980s, C++ was designed to provide programmers with better control over system resources and memory management while also incorporating abstraction, encapsulation, and polymorphism. Its versatility allows it to be used for a wide range of applications, from system software and game development to financial systems and real-time applications.
One of the key strengths of C++ is its balance between low-level memory manipulation and high-level abstraction. Unlike many modern languages that prioritize ease of use over performance, C++ gives developers direct access to hardware and memory management through pointers, dynamic allocation, and manual deallocation. At the same time, features like templates, the Standard Template Library (STL), and strong type-checking provide a robust framework for efficient and scalable software development. This makes C++ an ideal choice for performance-critical applications, such as embedded systems, graphics engines, and large-scale enterprise software.
Over the years, C++ has continuously evolved, with major updates like C++11, C++14, C++17, and C++20 introducing new features to enhance productivity and security. These improvements include smart pointers for safer memory management, lambda expressions for functional programming, and concurrency support for multithreading applications. Today, C++ remains a widely used and influential language, providing the foundation for many modern technologies and software systems.
Questions & Answers
Here is the complete list of 50 C++ technical interview questions and answers, covering beginner to advanced topics.
Basic C++ Interview Questions
1. What is C++?
C++ is a general-purpose programming language that extends C by adding object-oriented features like classes and inheritance.
2. What are the key features of C++?
- Object-oriented programming (OOP)
- Strongly typed language
- Supports dynamic memory allocation
- Rich Standard Template Library (STL)
- Low-level and high-level programming support
3. What is the difference between C and C++?
Feature | C | C++ |
Programming Paradigm | Procedural | Procedural + Object-Oriented |
Encapsulation | No | Yes |
Function Overloading | No | Yes |
Namespaces | No | Yes |
4. What is a class in C++?
A class is a blueprint for creating objects. It contains data members (variables) and member functions (methods).
5. What is an object in C++?
An object is an instance of a class. It represents a real-world entity and holds class properties.
6. What are access specifiers in C++?
Access specifiers define the accessibility of class members:
- public: Accessible from anywhere
- private: Accessible only within the class
- protected: Accessible within the class and derived classes
7. What is a constructor?
A constructor is a special function that initializes an object when it is created.
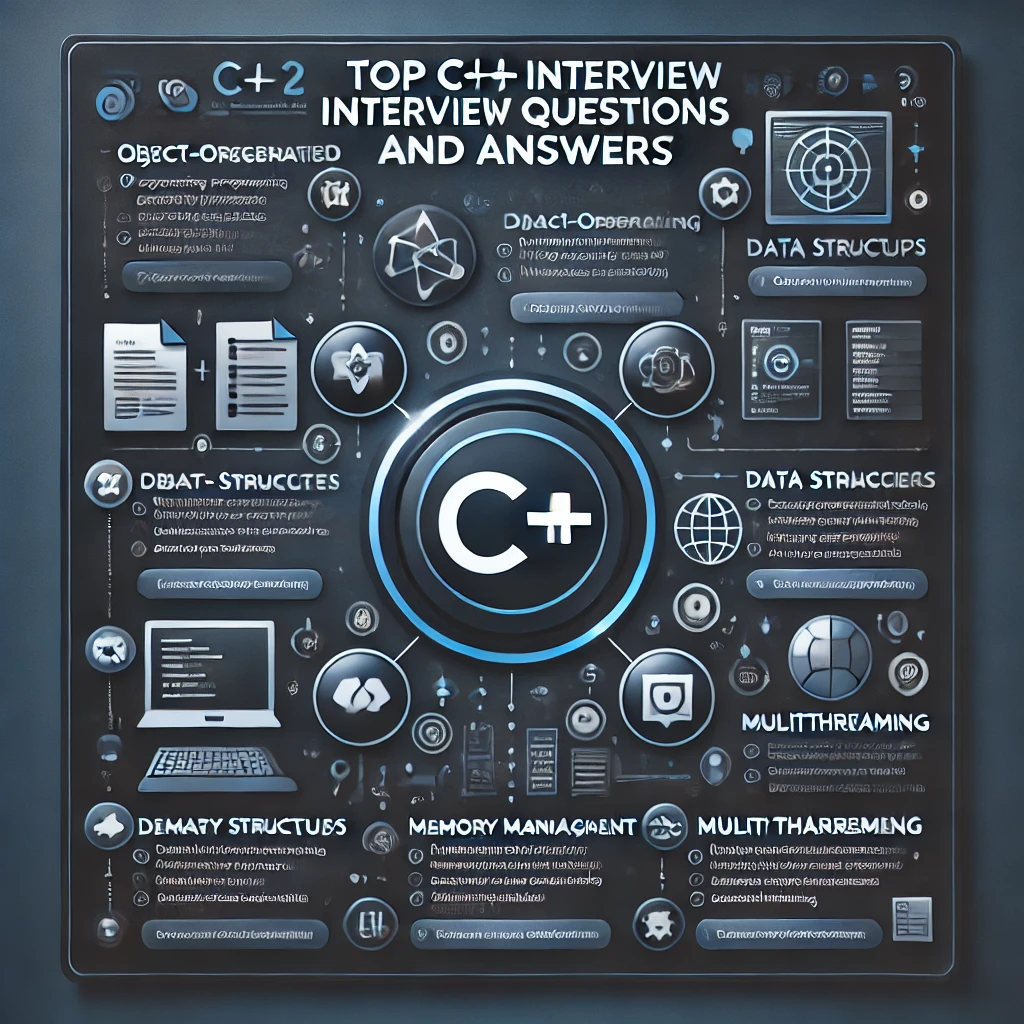
8. What are the types of constructors?
- Default Constructor: No parameters.
- Parameterized Constructor: Accepts parameters.
- Copy Constructor: Copies an object.
- Move Constructor: Moves an object (C++11).
9. What is function overloading?
Function overloading allows multiple functions with the same name but different parameters.
10. What is operator overloading?
Operator overloading allows defining custom behavior for operators like +, -, *, etc., for user-defined types.
Intermediate C++ Interview Questions
11. What is a virtual function?
A virtual function enables runtime polymorphism by allowing derived classes to override a function.
12. What is runtime polymorphism?
Runtime polymorphism is achieved using virtual functions, enabling method overriding.
13. What is compile-time polymorphism?
Compile-time polymorphism is achieved using function overloading and operator overloading.
14. What is an inline function?
An inline function suggests to the compiler to replace function calls with actual code to reduce function call overhead.
15. What is the “this” pointer?
The this pointer holds the memory address of the current object.
16. What is a friend function?
A friend function can access private and protected members of a class but is not a class member.
17. What is the difference between shallow copy and deep copy?
- Shallow copy: Copies only memory addresses.
- Deep copy: Copies actual data into a new memory allocation.
18. What is dynamic memory allocation?
Memory is allocated at runtime using new and freed using delete.
19. What is a static member in C++?
A static member belongs to a class rather than an instance, meaning all instances share it.
20. What is a destructor?
A destructor is a special function automatically called when an object goes out of scope.
Advanced C++ Interview Questions
21. What is the Standard Template Library (STL)?
STL provides reusable templates for data structures and algorithms.
22. What are the components of STL?
- Containers (vector, list, deque, map, etc.)
- Algorithms (sort, search, etc.)
- Iterators (used to traverse containers)
23. What is a smart pointer?
A smart pointer (unique_ptr, shared_ptr, weak_ptr) automatically manages memory allocation and deallocation.
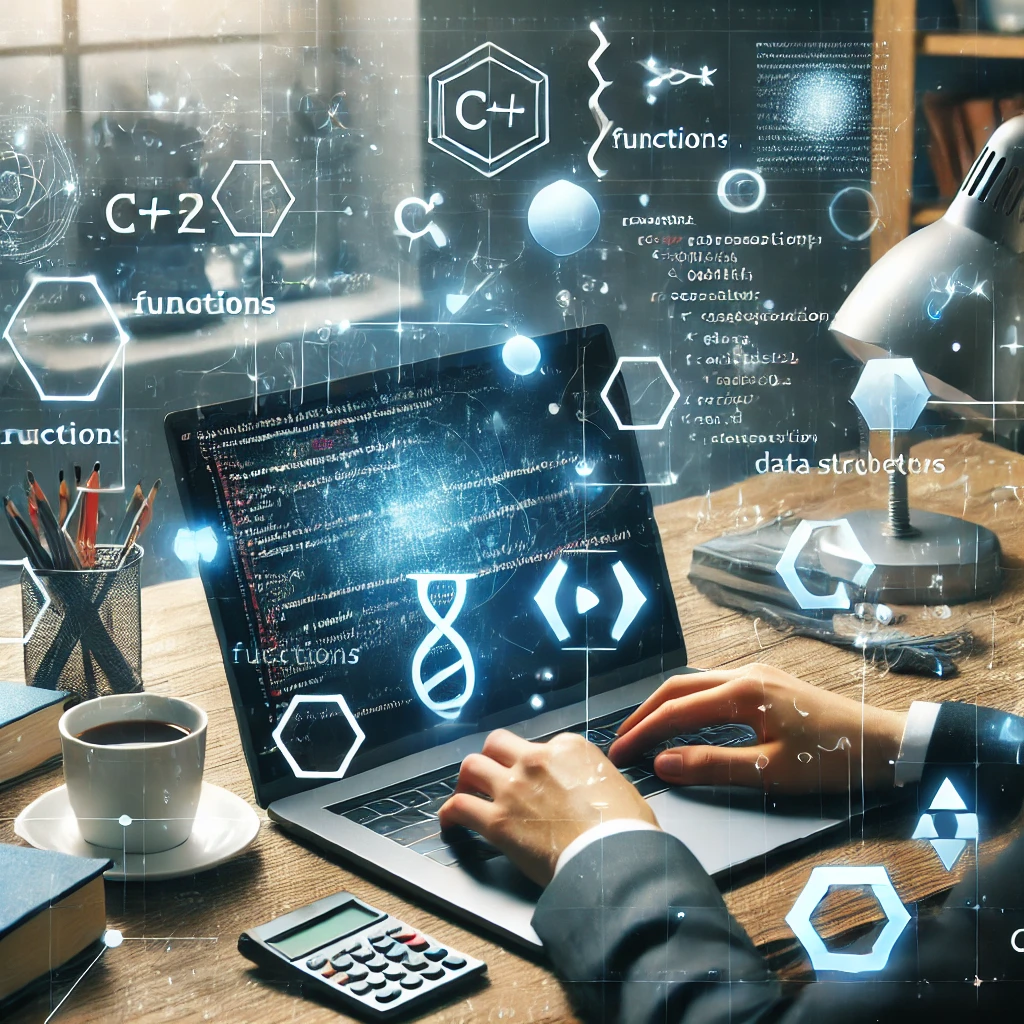
24. What is RAII?
Resource Acquisition Is Initialization (RAII) ensures resource management (like memory) is done via object lifetime.
25. What is the explicit keyword?
Prevents implicit type conversions when defining single-parameter constructors.
26. What are function pointers?
Function pointers store addresses of functions and allow dynamic function calls.
27. What is multiple inheritance?
A class can inherit from more than one base class.
28. What is the Diamond Problem?
A situation where a derived class inherits from two base classes that share a common base class, causing ambiguity.
29. What is a virtual base class?
A virtual base class prevents duplicate instances in multiple inheritance.
30. What is std::move()?
Moves ownership of resources instead of copying, improving efficiency.
C++ Coding & Debugging Questions
31. How to prevent memory leaks?
- Use smart pointers (unique_ptr, shared_ptr).
- Always pair new with delete.
32. How to reverse a string?
std::string str = “hello”;
std::reverse(str.begin(), str.end());
std::cout << str; // Output: olleh
33. How to find the size of an object?
Using sizeof() operator:
class A { int x; double y; };
std::cout << sizeof(A);
34. How to swap two numbers without a temp variable?
a = a + b;
b = a – b;
a = a – b;
35. How to detect a loop in a linked list?
Using Floyd’s Cycle Detection Algorithm.
C++ Multithreading & Optimization
36. What is multithreading?
Multithreading allows concurrent execution of multiple threads.
37. What is the difference between mutex and semaphore?
- Mutex: Ensures only one thread accesses a resource.
- Semaphore: Allows limited concurrent access.
38. How to create a thread?
std::thread t(func);
t.join();
39. What is deadlock?
When two or more threads wait indefinitely for each other.
Miscellaneous C++ Questions
40. What is placement new?
Allocates memory in a pre-allocated buffer.
41. What are volatile variables?
volatile prevents compiler optimizations, ensuring values are always read from memory.
42. What is a memory alignment issue?
Ensuring data is stored efficiently in memory to optimize access speed.
43. How is exception handling implemented?
Using try, catch, and throw.
44. What is a functor?
A class with an overloaded operator() to behave like a function.
45. What is std::move()?
Transfers resources instead of copying them.
46. How does reference counting work in shared_ptr?
shared_ptr keeps a reference count and deletes the object when the count reaches zero.
47. What is std::weak_ptr?
A weak reference to an object managed by shared_ptr, preventing circular dependencies.
48. How to sort a vector?
std::sort(v.begin(), v.end());
49. What is std::unordered_map?
A hash table-based associative container for fast lookups.
50. What is std::unique_lock?
A flexible lock management mechanism for std::mutex.
Conclusion on C++
C++ continues to be one of the most influential programming languages, offering a unique combination of performance, flexibility, and scalability. Its ability to work at both high and low levels makes it a preferred choice for developing operating systems, databases, and performance-intensive applications such as game engines and simulations. The language’s object-oriented and generic programming features enable the creation of modular, reusable, and efficient code, making software development more structured and maintainable.
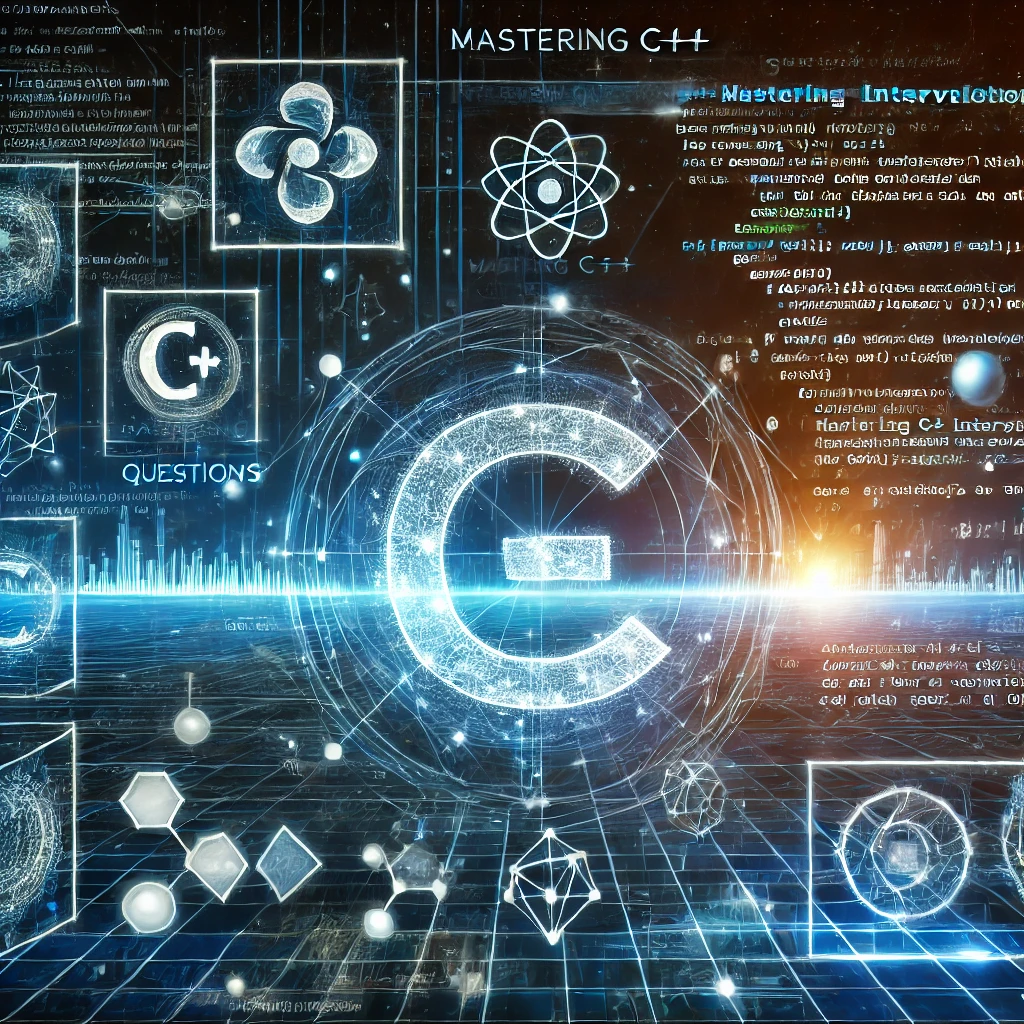
Despite the emergence of newer languages with automated memory management and simplified syntax, C++ remains relevant due to its superior performance and fine-grained control over system resources. Its continued evolution ensures that it adapts to modern programming needs, with newer standards bringing enhancements like improved concurrency handling, safer memory management, and more efficient coding practices. The thriving C++ community, along with vast libraries and frameworks, supports developers in building robust and high-performance applications across various domains.
As technology advances, C++ will remain a fundamental programming language, especially in areas where speed, reliability, and system-level access are crucial. Whether for beginners learning fundamental programming concepts or experienced developers building large-scale applications, C++ offers a solid foundation for software development. With its rich history, powerful features, and ongoing improvements, C++ is expected to continue shaping the future of programming for years to come.
Curated Reads