Ajax, an acronym for Asynchronous JavaScript and XML, has transformed web development since its emergence in the early 2000s. It introduced a paradigm shift, enabling web applications to send and retrieve data from a server asynchronously without interfering with the display and behavior of the existing page. This led to the development of more dynamic, responsive, and user-friendly web applications, moving away from the traditional, synchronous page refresh model that dominated the early web.
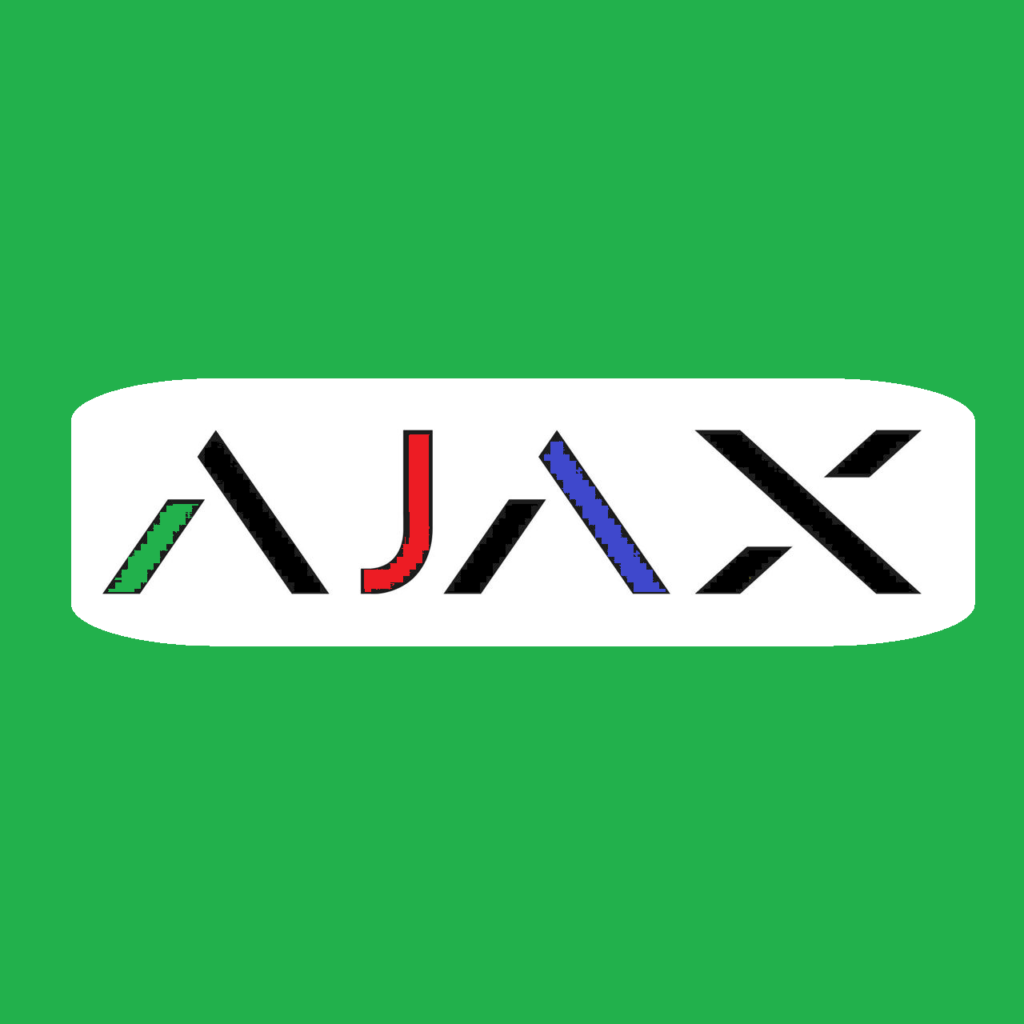
At its core, Ajax is not a single technology but a combination of several existing technologies: HTML and CSS for presentation, the Document Object Model (DOM) for dynamic display and interaction, XML for the interchange of data, XSLT for data manipulation, and, most importantly, JavaScript to bind these technologies together and handle asynchronous communication. Over time, other data formats like JSON have become more popular for data interchange due to their simplicity and efficiency.
The real power of Ajax lies in its ability to improve the user experience. Before Ajax, interactions requiring server communication meant a full page reload, leading to a sluggish user experience. With Ajax, only small portions of the web page need to be updated, which makes applications faster and more interactive. This capability has become the cornerstone of modern web applications, from simple forms to complex single-page applications (SPAs).
In this article, we will delve into the intricacies of Ajax programming, exploring its components, advantages, and best practices. We will also look at practical examples to illustrate how Ajax can be implemented in real-world applications. By the end of this article, you will have a comprehensive understanding of how Ajax works and how it can be leveraged to build efficient, dynamic web applications.
The Building Blocks of Ajax
1. JavaScript
JavaScript is the backbone of Ajax. It is the language that orchestrates the asynchronous requests to the server and processes the responses. With JavaScript, you can manipulate the DOM to update the web page dynamically without requiring a full page reload.
2. XML/JSON
Initially, XML was the standard format for data interchange in Ajax applications. It is a markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable. However, JSON (JavaScript Object Notation) has largely supplanted XML due to its lighter weight and ease of use with JavaScript. JSON is a text-based data format that is easy to read and write for humans and easy to parse and generate for machines.
3. XMLHttpRequest
The XMLHttpRequest object is the key to Ajax. It allows web pages to make HTTP requests to servers asynchronously. Using this object, a web page can fetch data from a server after the page has loaded, update the page with the new data, and interact with the user without having to reload the entire page.
4. The DOM
The Document Object Model (DOM) represents the structure of a web page as a tree of objects. JavaScript can interact with the DOM to update the page dynamically. This means that after receiving data from the server via an Ajax request, JavaScript can manipulate the DOM to reflect the new data without needing to refresh the entire page.
How Ajax Works
Understanding how Ajax works is crucial for implementing it effectively. Here is a step-by-step breakdown of the process:
- Event Occurs: An event occurs on the web page, such as a user clicking a button or loading the page.
- Create XMLHttpRequest Object: JavaScript creates an instance of the XMLHttpRequest object.
- Configure the Request: The XMLHttpRequest object is configured with the details of the request (method, URL, etc.).
- Send the Request: The request is sent to the server asynchronously.
- Server Processes Request: The server processes the request and returns the data (usually in JSON format).
- Handle the Response: JavaScript receives the data from the server and processes it.
- Update the DOM: The DOM is updated to reflect the new data, providing a seamless user experience.
Example: A Simple Ajax Request
Let’s look at a simple example to illustrate how Ajax works. Suppose we want to load user data from a server and display it on a web page without reloading the page.
<!DOCTYPE html>
<html>
<head>
<title>Ajax Example</title>
<script type="text/javascript">
function loadUserData() {
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/user', true);
xhr.onreadystatechange = function () {
if (xhr.readyState == 4 && xhr.status == 200) {
var user = JSON.parse(xhr.responseText);
document.getElementById('user-name').innerHTML = user.name;
document.getElementById('user-email').innerHTML = user.email;
}
};
xhr.send();
}
</script>
</head>
<body>
<h1>User Information</h1>
<p id="user-name"></p>
<p id="user-email"></p>
<button onclick="loadUserData()">Load User Data</button>
</body>
</html>
n this example, when the “Load User Data” button is clicked, the loadUserData
function is called. This function creates an XMLHttpRequest
object, configures it to send a GET request to https://example.com/api/user
, and then sends the request. When the server responds, the response data (assumed to be in JSON format) is parsed and used to update the content of the web page.
Advantages of Using Ajax
Ajax offers several significant advantages that have made it an essential tool in modern web development:
1. Improved User Experience
Ajax allows for the creation of highly responsive web applications. Users can interact with the application without experiencing delays or disruptions caused by full page reloads. This leads to a smoother, more intuitive user experience.
2. Reduced Server Load
By only requesting the data that needs to be updated, Ajax reduces the amount of data transmitted between the client and server. This can significantly reduce server load and bandwidth usage, improving overall performance.
3. Enhanced Performance
Since Ajax requests are asynchronous, the web application remains responsive while waiting for the server’s response. This can lead to faster performance, especially for applications that require frequent server interactions.
4. Modularity and Reusability
Ajax promotes a modular approach to web development. Components can be developed and tested independently, making the codebase more maintainable and reusable.
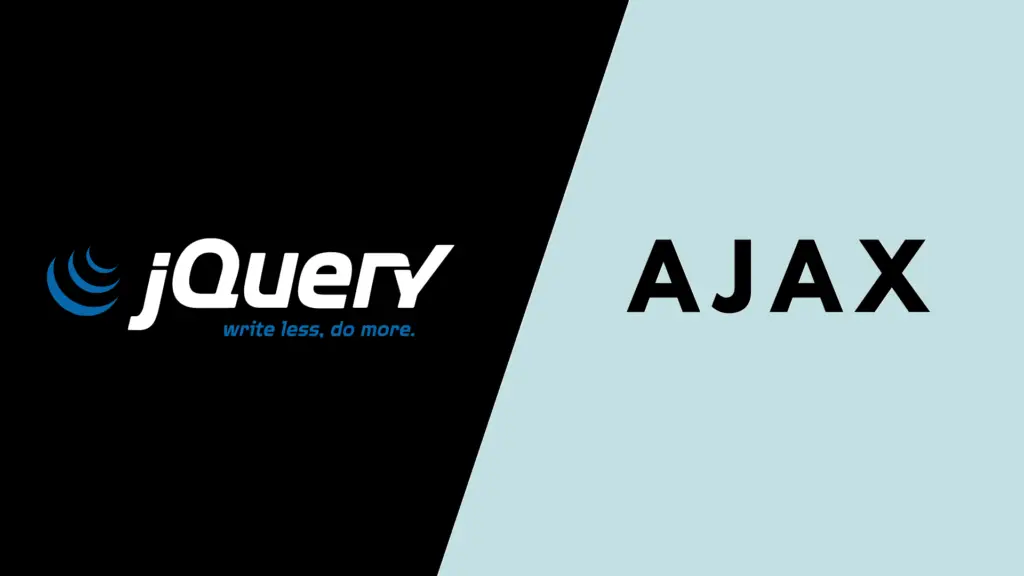
Best Practices for Ajax Programming
To get the most out of Ajax, it’s important to follow best practices. Here are some key recommendations:
1. Graceful Degradation
Not all users will have JavaScript enabled, and some might be using browsers that don’t fully support Ajax. Ensure your application degrades gracefully by providing fallback options. For example, if JavaScript is disabled, ensure that forms can still be submitted traditionally.
2. Minimize Requests
Avoid sending unnecessary Ajax requests. Aggregate multiple requests into a single request when possible. This can reduce the number of round-trips to the server, saving bandwidth and improving performance.
3. Error Handling
Always implement robust error handling for your Ajax requests. Handle different HTTP status codes appropriately and provide user-friendly error messages. Logging errors can also help in diagnosing issues.
4. Security Considerations
Ajax applications are susceptible to common web security vulnerabilities, such as cross-site scripting (XSS) and cross-site request forgery (CSRF). Implement security measures to protect your application, such as validating and sanitizing inputs, using secure communication channels (HTTPS), and implementing CSRF tokens.
5. Optimize Performance
Use performance optimization techniques like caching responses and minimizing the size of data being transmitted. Additionally, consider using techniques like lazy loading to defer the loading of certain data until it is actually needed by the user.
6. Use Modern JavaScript Frameworks
Modern JavaScript frameworks like React, Vue.js, and Angular provide built-in support for Ajax and can simplify the development process. They offer powerful abstractions for handling data binding, state management, and component-based development, making it easier to build complex web applications.
Real-World Applications of Ajax
Ajax is used in a wide range of web applications. Here are some common scenarios where Ajax shines:
1. Form Validation
Ajax is often used for real-time form validation. Instead of submitting the form and waiting for the server to respond, Ajax can be used to validate form fields on-the-fly as the user types. This provides immediate feedback to the user and improves the overall user experience.
2. Autocomplete and Suggestion Features
Many modern web applications use Ajax to implement autocomplete and suggestion features. For example, search engines and online stores use Ajax to provide real-time search suggestions as the user types in the search box. This can make the search process faster and more intuitive.
3. Single-Page Applications (SPAs)
Ajax is a fundamental technology for single-page applications. SPAs load a single HTML page and dynamically update the content as the user interacts with the application. This provides a seamless and highly responsive user experience, similar to that of a desktop application.
4. Real-Time Updates
Web applications that require real-time updates, such as chat applications and social media feeds, rely heavily on Ajax. By using Ajax to periodically fetch new data from the server, these applications can provide real-time updates without requiring the user to refresh the page.
5. Infinite Scrolling
Ajax is commonly used to implement infinite scrolling, where additional content is loaded dynamically as the user scrolls down the page. This technique is often used in social media feeds and news websites to provide a continuous stream of content.
Example: Real-Time Chat Application
Let’s build a simple real-time chat application using Ajax to demonstrate its capabilities in a real-world scenario.
<!DOCTYPE html>
<html>
<head>
<title>Ajax Chat</title>
<style>
#chat-box {
width: 300px;
height: 400px;
border: 1px solid #ccc;
overflow-y: scroll;
}
#message {
width: 200px;
}
</style>
<script type="text/javascript">
function sendMessage() {
var message = document.getElementById('message').value;
var xhr = new XMLHttpRequest();
xhr.open('POST', 'https://example.com/api/sendMessage', true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onreadystatechange = function () {
if (xhr.readyState == 4 && xhr.status == 200) {
loadMessages();
}
};
xhr.send(JSON.stringify({ message: message }));
}
function loadMessages() {
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://example.com/api/getMessages', true);
xhr.onreadystatechange = function () {
if (xhr.readyState == 4 && xhr.status == 200) {
var messages = JSON.parse(xhr.responseText);
var chatBox = document.getElementById('chat-box');
chatBox.innerHTML = '';
for (var i = 0; i < messages.length; i++) {
var p = document.createElement('p');
p.innerHTML = messages[i].text;
chatBox.appendChild(p);
}
}
};
xhr.send();
}
setInterval(loadMessages, 3000); // Fetch new messages every 3 seconds
</script>
</head>
<body>
<div id="chat-box"></div>
<input type="text" id="message" />
<button onclick="sendMessage()">Send</button>
</body>
</html>
In this example, the chat application consists of a chat box for displaying messages, an input field for typing messages, and a button to send messages. The sendMessage
function sends the user’s message to the server using an Ajax POST request. The loadMessages
function fetches the latest messages from the server using an Ajax GET request and updates the chat box. The setInterval
function is used to periodically fetch new messages from the server every 3 seconds, providing real-time updates.
The inference
Ajax has revolutionized the way web applications are developed, enabling the creation of highly dynamic, responsive, and interactive user experiences. By allowing asynchronous communication between the client and server, Ajax eliminates the need for full page reloads, resulting in faster and more efficient applications. Its integration with existing technologies like JavaScript, XML/JSON, and the DOM makes it a versatile and powerful tool for modern web development.
To leverage the full potential of Ajax, it’s important to follow best practices, such as implementing graceful degradation, minimizing requests, handling errors properly, and considering security implications. Additionally, using modern JavaScript frameworks can simplify the development process and help build robust applications.
Ajax is widely used in various real-world scenarios, from form validation and autocomplete features to single-page applications and real-time updates. Its ability to enhance user experience and performance has made it an indispensable part of web development.
As web technologies continue to evolve, Ajax remains a cornerstone of dynamic web applications, and understanding its principles and applications is crucial for any web developer aiming to create modern, efficient, and user-friendly web applications.