Databases are the backbone of modern applications, managing vast amounts of data for diverse industries. Whether it’s an e-commerce site managing product inventories or a banking system handling millions of transactions daily, the structure of a database is critical for its performance and utility. One of the essential aspects of database management is maintaining and modifying database structures. This is where SQL (Structured Query Language) commands come into play.
Among the most powerful SQL commands is the DROP command, which allows users to permanently remove objects from a database. It plays a crucial role in database administration, providing the ability to delete tables, views, indexes, schemas, and even entire databases. However, with great power comes great responsibility—misusing the DROP command can lead to significant data loss or the corruption of a database structure.
The DROP command is indispensable for developers, database administrators, and system architects alike. It ensures the removal of obsolete or redundant database objects, keeping the system clean and optimized. However, it must be used cautiously, as it deletes objects permanently without the possibility of recovery unless backups are in place. This makes understanding the DROP command, its syntax, applications, and best practices essential for anyone working with databases.
In this article, we will dive deep into the DROP command, exploring its syntax, use cases, and common pitfalls. Real-world examples will demonstrate its application in Python, a popular language for database interaction. We’ll also discuss best practices to ensure that the command is used responsibly.
Table of Contents
What is the DROP Command in SQL?.
Using the DROP Command in Python.
Best Practices for Using the DROP Command.
Common Pitfalls and How to Avoid Them..
Advanced Example: Using DROP in PostgreSQL with Python.
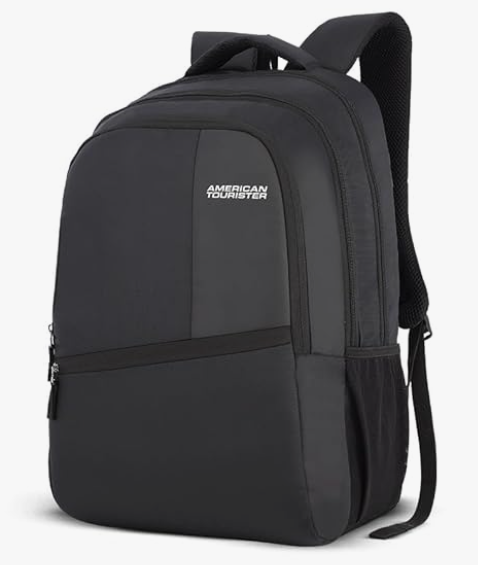
American Tourister Valex 28 Ltrs Large Laptop Backpack
Limited time deal
Priced at -44% discount for ₹1,399
What is the DROP Command in SQL?
The DROP command is a Data Definition Language (DDL) statement in SQL that is used to remove database objects permanently. Unlike the DELETE command, which removes rows of data from a table while preserving the table’s structure, the DROP command eliminates the object itself. This includes all associated data, indexes, triggers, and constraints.
Key Features of the DROP Command
- Irreversible Action: Once an object is dropped, it cannot be recovered unless a backup exists.
- Wide Scope: It can be used to delete various database objects such as tables, schemas, databases, indexes, and views.
- Improves Database Maintenance: It helps in removing obsolete objects, which optimizes database performance.
Common Use Cases
- Removing Redundant Tables: When a table is no longer needed, dropping it frees up space and simplifies the database schema.
- Deleting Test Databases: During development, test databases or tables are often created and later removed using the DROP command.
- Resetting a Database: In some cases, it’s necessary to drop all tables or the database itself to start from scratch.
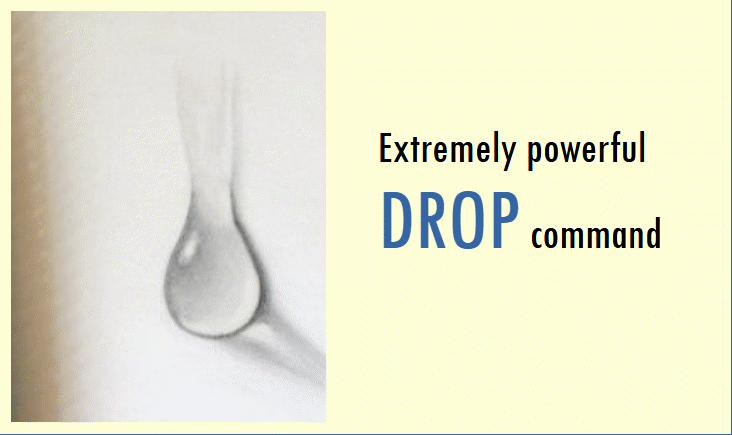
How to Use the DROP Command
Syntax
The syntax for the DROP command varies depending on the object being removed. Below are some common examples:
- Drop a Table
DROP TABLE table_name;
This command permanently deletes the specified table and all its data.
- Drop a Database
DROP DATABASE database_name;
This removes the entire database and all associated objects.
- Drop a Schema
DROP SCHEMA schema_name CASCADE;
Here, the CASCADE option ensures that all objects within the schema are also dropped.
- Drop an Index
DROP INDEX index_name;
This deletes an index, which can improve performance if the index is redundant.
Using the DROP Command in Python
Python, with libraries such as sqlite3, psycopg2, or SQLAlchemy, is widely used for database management. These libraries allow seamless execution of SQL commands, including DROP. Let’s explore a practical example using SQLite.
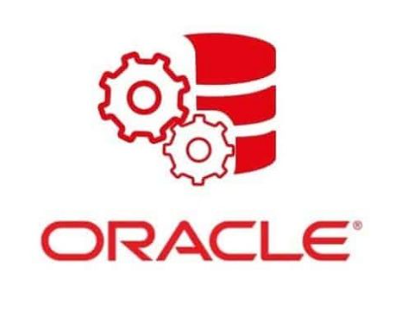
Example 1: Dropping a Table in SQLite
This script demonstrates how to create a database, add a table, and then drop it using Python.
import sqlite3
# Connect to a database (or create one if it doesn't exist)
connection = sqlite3.connect('example.db')
# Create a cursor object
cursor = connection.cursor()
# Create a table
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
email TEXT NOT NULL UNIQUE
)
''')
print("Table 'users' created.")
# Drop the table
cursor.execute('DROP TABLE IF EXISTS users')
print("Table 'users' dropped.")
# Commit the changes and close the connection
connection.commit()
connection.close()
Explanation of the Code
- Database Connection: A connection is established to the SQLite database using sqlite3.connect().
- Cursor Execution: The cursor.execute() method executes SQL commands.
- Create and Drop Table: The script first creates a users table and then drops it using the DROP TABLE command.
- Safety Mechanism: The IF EXISTS clause ensures that no error is raised if the table doesn’t exist.
Best Practices for Using the DROP Command
To avoid potential data loss and ensure proper usage, follow these best practices:
- Always Backup Data
Before dropping any object, ensure that a backup is in place to recover data if needed. - Use IF EXISTS Clause
Adding IF EXISTS prevents errors if the object to be dropped doesn’t exist. - Restrict Access
Limit the execution of DROP commands to authorized users to prevent accidental deletions. - Test in a Development Environment
Test DROP commands in a staging or development environment before executing them in production. - Document Changes
Maintain a log of all DROP commands executed to track modifications in the database schema.
Common Pitfalls and How to Avoid Them
- Accidental Data Loss
Misusing the DROP command can lead to the unintended deletion of critical objects. Avoid this by thoroughly reviewing the command before execution. - Dependency Issues
Dropping an object that other objects depend on can break the database. Use the CASCADE option cautiously. - No Undo Mechanism
Since DROP is irreversible, ensure the command is part of a well-planned strategy. - Performance Impact
Dropping large objects in production can impact database performance. Schedule such operations during maintenance windows.
Advanced Example: Using DROP in PostgreSQL with Python
PostgreSQL offers rich features for database management. Here’s how to drop a table in PostgreSQL using Python with the psycopg2 library.
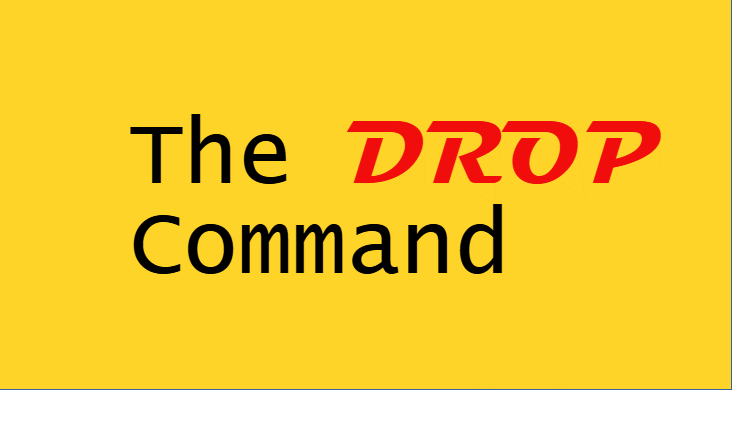
Python Code
import psycopg2
# Database connection parameters
db_config = {
'dbname': 'testdb',
'user': 'postgres',
'password': 'your_password',
'host': 'localhost',
'port': '5432'
}
try:
# Connect to PostgreSQL database
connection = psycopg2.connect(**db_config)
cursor = connection.cursor()
# Drop a table if it exists
cursor.execute('DROP TABLE IF EXISTS employees')
print("Table 'employees' dropped successfully.")
# Commit the changes
connection.commit()
except (Exception, psycopg2.DatabaseError) as error:
print(f"Error: {error}")
finally:
# Close the database connection
if connection:
cursor.close()
connection.close()
print("PostgreSQL connection closed.")
Explanation
- Connection Setup: The script connects to a PostgreSQL database using psycopg2.
- Error Handling: Exceptions are handled gracefully to ensure database integrity.
- Execution: The DROP TABLE command removes the employees table if it exists.
Conclusion
The DROP command is a critical tool in database management, offering the ability to delete various database objects permanently. Its versatility makes it a go-to option for removing obsolete or redundant objects, but it must be used with caution to prevent data loss or structural issues.
Incorporating the DROP command into Python scripts with libraries like sqlite3 and psycopg2 simplifies database administration tasks. By adhering to best practices and being mindful of its irreversible nature, users can harness its power effectively.
Good reads
Thanks for sharing. I read many of your blog posts, cool, your blog is very good.
Thank you for stopping by! I appreciate your kind words. Keep in touch with us on social media –
Website: https://www.learnxyz.in
Facebook: https://www.facebook.com/groups/530719219330002
YouTube: https://www.youtube.com/@LearnXYZ-In
Page: https://www.facebook.com/people/Learnxyzin/61572213195329/
Twitter/X: https://x.com/LearnxyzIn
Spotify: https://open.spotify.com/show/6sjYYVRjgsmpflhpwCm1Qb?si=KpV3gxStQCeUcTeveRHNxQ
We are glad that we have been of value to you. We use affiliates on our webpages, if you prefer, please consider purchasing from the in-page links and advertisements. This will help us support the team and this website. Thanks! Good Day & Visit Again!