In today’s app-driven world, mobile applications are more interactive and personalized than ever. They access our location, photos, contacts, and more to provide a tailored user experience. However, this access requires permissions from the user, which is where React Native permissions come into play, especially for developers creating apps on both iOS and Android platforms. Let’s dive into what React Native permissions are, why they’re important, and how to handle them in your apps.
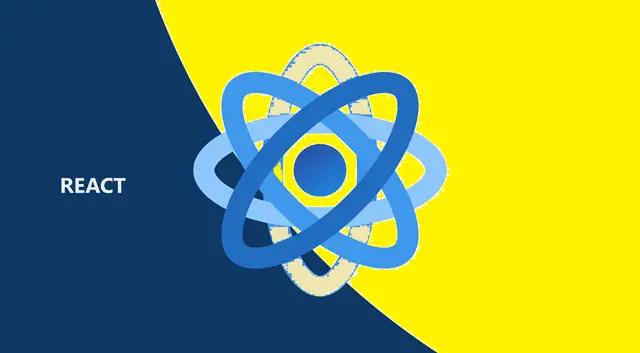
React Native has emerged as a frontrunner in the cross-platform mobile development space, enabling developers to craft beautiful and functional apps for both iOS and Android with a single codebase. A crucial aspect of developing these applications is managing permissions—a process that involves requesting and obtaining consent from users to access certain features or data on their devices. React Native’s approach to handling permissions offers a unique blend of challenges and opportunities that warrant a closer look.
One of the most compelling features of React Native is its cross-platform nature, which inherently brings forth the challenge of handling permissions across different operating systems. iOS and Android have distinct permission models and user interfaces, making uniformity in permission requests a task easier said than done. Developers need to navigate these differences, ensuring that permission requests are not only compliant with platform guidelines but also user-friendly. This challenge, however, presents an opportunity for developers to deeply understand user interaction patterns and tailor experiences that are both intuitive and respectful of user privacy across platforms.
What are React Native Permissions?
React Native is a popular framework for developing mobile applications using JavaScript. It enables developers to create apps for both iOS and Android with a single codebase. Permissions in React Native are rules that apps must follow to access personal information or features on a user’s device, such as the camera, GPS, and contacts list. At the heart of permission requests is the principle of user trust and transparency. Users are becoming increasingly aware of their digital privacy and are more cautious about granting apps access to personal information or device features. React Native developers have the opportunity to design permission request flows that are clear, honest, and provide sufficient context. This is more than a technical requirement—it’s a chance to foster trust with the user by transparently communicating why certain permissions are needed and how they enhance the app’s functionality or user experience.
The Role of Libraries
The React Native ecosystem is rich with libraries that simplify complex tasks, including managing permissions. Libraries such as react-native-permissions
abstract away the nitty-gritty details of requesting permissions, offering a unified API that works across iOS and Android. This not only speeds up development time but also ensures that permission requests are consistent and maintain high standards of user experience. However, relying on external libraries also requires a commitment to keep them updated and an understanding of their inner workings to some extent, to mitigate any potential security or functionality issues.
Ethical Considerations and Best Practices
Developers must tread carefully, balancing the need for specific permissions with respect for user privacy. Best practices suggest asking for permissions only when necessary, and ideally, at the point in the app where the permission’s need is evident. This approach minimizes user frustration and confusion, potentially increasing the likelihood of permission grants. Moreover, it underscores the developer’s role not just as a coder but as a guardian of user privacy, tasked with making ethical decisions about data access and handling.
Permissions are crucial for two main reasons
- Privacy: They protect users’ privacy by ensuring that apps don’t access more information than necessary.
- Security: They help secure users’ data by controlling app access to sensitive information.
Why are Permissions Important in React Native?
Permissions are a cornerstone of user trust and app functionality. They allow users to control their privacy and data security. For developers, understanding and correctly implementing permissions is vital to creating apps that are both functional and respectful of user privacy. Additionally, app stores require apps to handle permissions correctly as a condition for approval.
Handling Permissions in React Native
React Native provides a straightforward approach to managing permissions, but the implementation can differ slightly between iOS and Android due to their distinct permission models.
1. Understanding Permission Types
First, identify the types of permissions your app needs. Common permissions include:
- Location
- Camera
- Microphone
- Contacts
Each permission type corresponds to specific functionalities within your app.
2. Requesting Permissions
Permissions should be requested at the point in your app where the access is needed. For instance, if your app needs to access the user’s camera to take a photo, the permission request should occur when they attempt to use this feature.
React Native’s API for permissions has evolved, and for the most straightforward and unified way of handling permissions, using a library like react-native-permissions
is recommended. This library offers a consistent API that works across iOS and Android and simplifies the process of requesting permissions.
3. Checking Permission Status
Before attempting to access a feature that requires permission, your app should check if permission has already been granted. If permission has not been granted, your app should request it. If the user denies permission, your app should respect their choice and either not use the feature or provide an alternative way to complete the task.
4. Handling Denied Permissions
It’s important to handle denied permissions gracefully. If a user denies a permission, consider showing a polite explanation of why the permission is necessary for a particular feature. For critical features, you might guide users on how to enable permissions from the system settings.
Best Practices
- Request permissions sparingly: Only ask for permissions that are essential for your app to function.
- Explain why you need permissions: Whenever you request a permission, clearly explain why you need it. This transparency builds trust.
- Test permissions: Regularly test your app’s permissions on different devices and OS versions to ensure a smooth user experience.
Practically doing it
Below are simplified code samples demonstrating how to handle permissions in React Native using the react-native-permissions
library. This library streamlines the process of requesting and checking permissions across iOS and Android. Before you begin, ensure you have installed the library in your project
npm install react-native-permissions
yarn add react-native-permissions
Don’t forget to link the library and update your Android or iOS project settings as required by the library’s documentation.
Example: Requesting Camera Permission
This example demonstrates how to request camera permission when a user wants to use the camera feature in your app.
First, import the library:
import {check, request, PERMISSIONS, RESULTS} from 'react-native-permissions';
const requestCameraPermission = async () => {
const result = await request(
Platform.OS === 'ios' ? PERMISSIONS.IOS.CAMERA : PERMISSIONS.ANDROID.CAMERA,
);
if (result === RESULTS.GRANTED) {
console.log('The permission is granted');
// You can now use the camera
} else {
console.log('The permission is denied');
// Handle the denial gracefully
}
};
Example: Checking Camera Permission Status
Before attempting to access the camera, it’s a good practice to check if your app already has permission. This can be done as follows:
const checkCameraPermission = async () => {
const status = await check(
Platform.OS === 'ios' ? PERMISSIONS.IOS.CAMERA : PERMISSIONS.ANDROID.CAMERA,
);
if (status === RESULTS.GRANTED) {
console.log('You can use the camera');
// Permission is granted, and you can access the camera
} else if (status === RESULTS.DENIED) {
console.log('Camera permission denied');
// Permission denied but requestable
requestCameraPermission(); // Optionally, request permission here
} else if (status === RESULTS.BLOCKED) {
console.log('Camera permission is blocked. Please enable it from settings');
// Permission is permanently denied and must be enabled through app settings
}
};
Handling Denied Permissions Gracefully
When permissions are denied, especially if they are critical to your app, it’s important to guide users appropriately. Here’s a simple approach to handle a denial:
const handleDeniedPermission = (permissionName) => { Alert.alert( "Permission Denied", `We need your permission for ${permissionName} to proceed. You can enable it from app settings.`, [ { text: "Cancel", style: "cancel" }, { text: "Open Settings", onPress: () => Linking.openSettings() } ] );};
This function uses Alert
from react-native
to show a message to the user, and if the user agrees, it directs them to the app’s settings using Linking.openSettings()
where they can manually enable the permission.
These examples provide a basic framework for handling permissions in React Native apps. Remember, handling permissions responsibly not only complies with app store policies but also builds trust with your users by respecting their privacy and security. Always stay updated with the latest React Native documentation and libraries for handling permissions, as APIs and best practices can evolve.
As React Native continues to evolve, so too will its approach to managing permissions. Future updates may bring more streamlined APIs or new challenges as operating systems update their permission models. Developers will need to stay informed and adaptable, ready to refine their permission handling strategies to meet the latest standards and user expectations.
React Native permissions are a pivotal aspect of mobile app development, balancing user experience with privacy and security. By understanding the types of permissions and handling them appropriately, developers can create apps that not only offer great functionality but also respect and protect user data. Remember, the key to successful permission management is transparency and respect for the user’s privacy and choices.
In short, React Native’s handling of permissions is a microcosm of the broader challenges and opportunities facing mobile app developers today. It’s a test of technical skill, ethical judgment, and user-centric design thinking. Successfully navigating this aspect of development can lead to more trustworthy, engaging, and user-friendly apps, contributing to a healthier, more privacy-conscious app ecosystem.
Your blog is a treasure trove of wisdom and positivity I appreciate how you always seem to know just what your readers need to hear
Hi my loved one I wish to say that this post is amazing nice written and include approximately all vital infos Id like to peer more posts like this.