Understanding the primary differences
Sometimes its good to have the facts related to technologies clear.. Python, a versatile and widely-used programming language, offers developers various data structures to work with. Two fundamental data structures in Python are lists and tuples. While they may seem similar at first glance, they possess distinct characteristics that make them suitable for different use cases. In this comprehensive comparison, we delve into the major differences between Python lists and tuples.This article Python Lists vs. Tuples is written to give you a nice understanding about which technique you must use where and why.
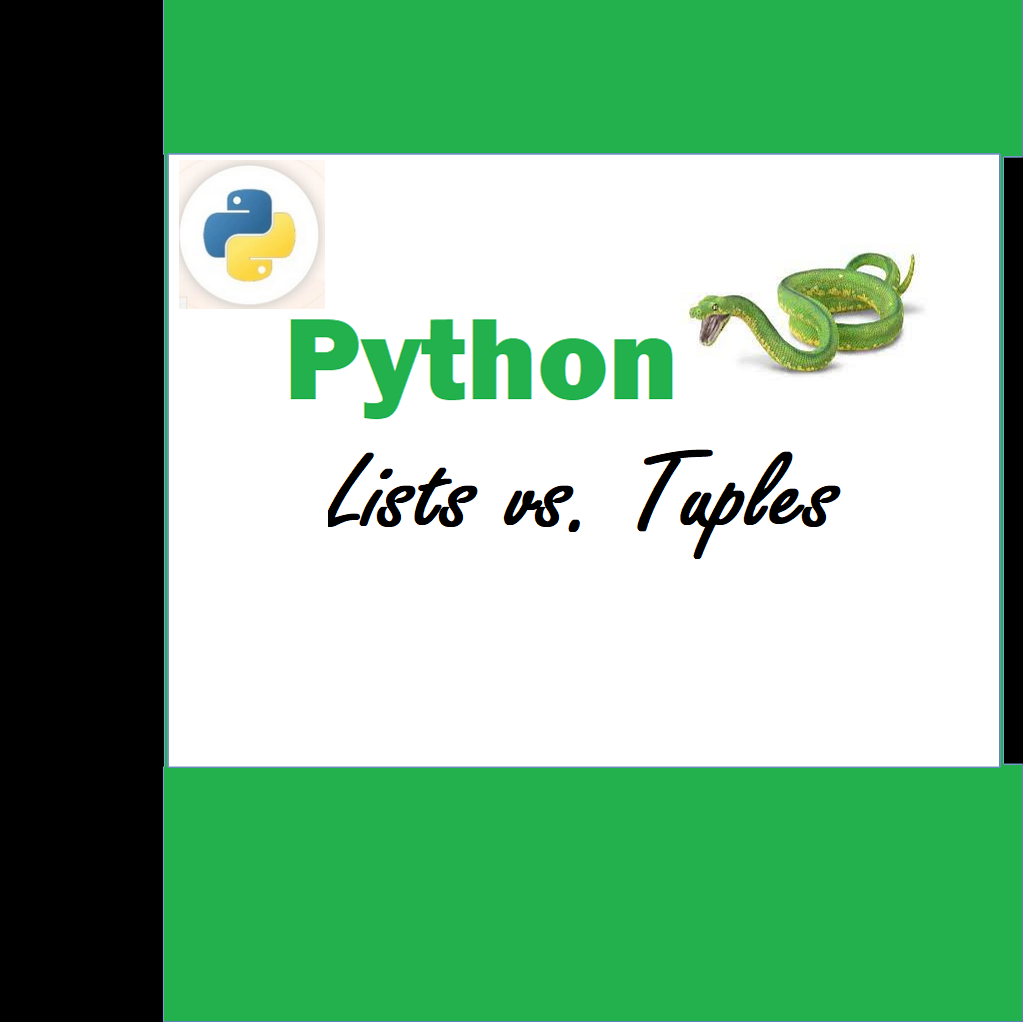
Lists
Python lists are versatile data structures that play a fundamental role in storing and organizing collections of items. Understanding how lists work under the hood is crucial for harnessing their full potential in Python programming. In this article, we’ll delve into the mechanics of Python lists, exploring their functionality, internal representation, and common operations.
Anatomy of Python Lists
At its core, a Python list is an ordered collection of elements, allowing for heterogeneous data types within the same list. Lists are mutable, meaning their contents can be altered after creation. Each element in a list is assigned a unique index, starting from 0 for the first element, 1 for the second, and so forth. This index facilitates efficient access and manipulation of list elements.
Internal Representation
Python lists are implemented as dynamic arrays, providing fast access to elements through indexing. Internally, lists are represented as contiguous blocks of memory, with each element occupying a fixed-size slot. When a list is created, Python allocates a certain amount of memory to accommodate initial elements. As the list grows, additional memory may be allocated to accommodate more elements.
Dynamic Resizing
One of the key features of Python lists is their ability to dynamically resize as elements are added or removed. When the capacity of a list is exceeded due to appending new elements, Python automatically reallocates memory to accommodate the expanded list. This dynamic resizing strategy ensures that lists can efficiently handle collections of varying sizes without excessive memory overhead.
Common Operations
Python lists support a wide range of operations for manipulating their contents. Some common operations include:
- Appending Elements: Adding elements to the end of a list using the
append()
method. - Inserting Elements: Inserting elements at a specific position within the list using the
insert()
method. - Accessing Elements: Accessing individual elements or slices of elements using indexing or slicing.
- Modifying Elements: Modifying existing elements by assigning new values to specific indices.
- Removing Elements: Removing elements from a list using methods such as
remove()
,pop()
, or slicing assignments.
Therefore it can be rightly said that,
- Lists are mutable, ordered collections of items enclosed within square brackets [].
- Mutable nature implies that elements within a list can be altered or modified after creation.
- Lists allow for heterogeneous elements, meaning they can hold different data types within the same list.
Tuples
Python tuples, akin to lists, are fundamental data structures that facilitate the organization and manipulation of data in Python. Unlike lists, tuples are immutable, offering unique characteristics and functionalities. In this article, we embark on an exploration of Python tuples, unravelling their inner workings, properties, and common usage scenarios.
Anatomy of Python Tuples
At their core, Python tuples are ordered collections of elements, enclosed within parentheses and separated by commas. Similar to lists, tuples support heterogeneous data types, enabling the storage of different kinds of data within the same tuple. However, the crucial distinction lies in their immutability; once a tuple is created, its elements cannot be modified, added, or removed.
Internal Representation
Internally, Python tuples are represented as fixed-size arrays, akin to lists. However, unlike lists, tuples do not support dynamic resizing or modification of elements. Upon creation, a tuple allocates a contiguous block of memory to store its elements. This static nature of tuples ensures data integrity and efficient memory usage, making them ideal for scenarios where immutability is desired.
Immutable Nature
The immutability of tuples imparts several advantages, including:
- Data Integrity: Since tuple elements cannot be altered after creation, tuples provide a reliable mechanism for preserving the integrity of data. This property is particularly valuable in scenarios where data consistency is paramount.
- Hashability: Tuples are hashable data types, meaning they can be used as keys in dictionaries and elements in sets. The immutability of tuples ensures that their hash values remain constant, facilitating efficient indexing and retrieval operations.
Common Operations
Although tuples are immutable, they support various operations for accessing and manipulating their elements. Some common operations include:
- Indexing: Accessing individual elements within a tuple using zero-based indexing.
- Slicing: Extracting contiguous subsets of elements from a tuple using slicing notation.
- Concatenation: Combining two or more tuples to create a new tuple using the
+
operator. - Iteration: Iterating through tuple elements using loops or comprehension constructs for processing or analysis.
Use Cases
Python tuples find utility in a myriad of use cases, including:
- Data Integrity: Tuples serve as immutable containers for storing configuration settings, database records, or constant values that should not be modified during program execution.
- Function Return Values: Tuples are commonly used to return multiple values from functions, encapsulating related data into a single immutable object.
- Tuple Unpacking: Tuples facilitate the unpacking of sequences into individual variables, simplifying assignments and function parameter passing.
So we can rightly say that ,
- Tuples are immutable, ordered collections of items enclosed within parentheses ().
- Immutable nature means once a tuple is created, its elements cannot be changed, added, or removed.
- Tuples also support heterogeneous elements, similar to lists.
Mutability
- Lists are mutable, allowing for dynamic changes. Elements can be added, removed, or modified using various methods such as append(), extend(), insert(), remove(), or slicing assignments.
- Tuples, on the other hand, are immutable. Once created, elements cannot be changed, added, or removed. Any operation that attempts to alter a tuple’s elements will result in an error.
Performance
- Lists are less memory-efficient compared to tuples because of their mutable nature. Lists require extra memory to accommodate potential changes in size and structure.
- Tuples are more memory-efficient due to their immutability. Once created, a tuple’s size and structure remain fixed, resulting in less memory overhead.
Syntax
- Lists are defined using square brackets [], and elements are separated by commas. Example: my_list = [1, 2, 3, 4, 5].
- Tuples are defined using parentheses (), and elements are separated by commas. Example: my_tuple = (1, 2, 3, 4, 5).
Use Cases
- Lists are preferred when dealing with collections of items where the size or content may change over time. They are suitable for scenarios requiring dynamic manipulation of elements, such as maintaining a list of tasks, storing user inputs, or representing sequences of data.
- Tuples are ideal for situations where immutability and integrity of data are desired. They are commonly used to represent fixed collections of elements, such as coordinates, database records, or configurations that should not be modified.
Iteration
- Both lists and tuples support iteration using loops such as for loops or comprehension expressions.
- Since tuples are immutable, iterating through them tends to be marginally faster than iterating through lists.
We can conclude it by this understanding – While both lists and tuples are essential data structures in Python, they serve different purposes based on their mutability, performance characteristics, and intended use cases. Lists are mutable and suitable for dynamic data manipulation, whereas tuples are immutable and provide data integrity. Understanding the distinctions between lists and tuples empowers Python developers to choose the appropriate data structure for their specific programming needs, thereby enhancing code efficiency and readability.
Curated for you:
Healthy Reading: