What they are –
Python is an incredibly versatile programming language that empowers developers to create efficient, clean, and maintainable code. Among the many features Python offers, decorators stand out as a powerful tool for modifying or extending the behaviour of functions and methods dynamically. Introduced in Python 2.4, decorators have since become a staple in modern Python programming due to their elegance and utility.
At their core, decorators are functions that wrap another function or method to enhance or alter its behaviour without permanently modifying its source code. This approach adheres to the principles of the Open/Closed Principle, a key concept in software design, which advocates that software entities should be open for extension but closed for modification.
Imagine you’re tasked with logging the execution time of several functions in your application. You could add repetitive logging code to each function, but this would lead to bloated and less readable code. Here’s where decorators shine. With a simple, reusable decorator, you can encapsulate the logging behaviour and apply it to multiple functions seamlessly.
Decorators are not only about adding functionality; they’re also instrumental in enforcing access controls, memoization, and validations. Python developers often encounter built-in decorators like @staticmethod, @classmethod, and @property in their projects. In addition to these, user-defined decorators unlock the potential to customize and streamline workflows.
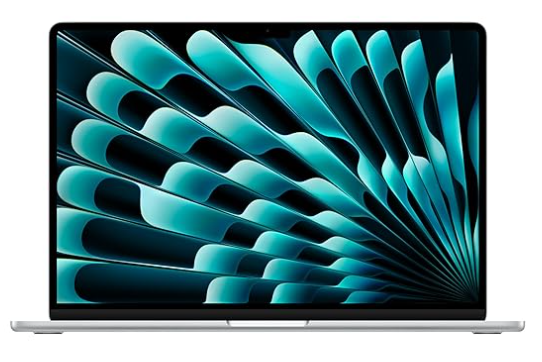
Apple 2024 MacBook Air 15″ Laptop with M3 chip
Priced at ₹1,27,990
Now we are diving into Pythons advanced concepts – Python Decorators. We are going to see what magic these advanced concepts provide us. Typically, this article delves deep into Python decorators, exploring their syntax, use cases, and real-world applications. We’ll provide practical examples using flask, share exclusive insights, and address common questions to equip you with the knowledge to leverage decorators effectively in your Python projects.
Table of Contents
Common Use Cases for Decorators.
Top 10 Exclusive Facts About Python Decorators.
What Are Python Decorators?
A decorator in Python (Python Decorators as we call it) is a function that modifies or extends the behaviour of another function or method. It’s applied using the @decorator_name syntax placed above the function definition.
Syntax of a Decorator
Here’s the basic syntax:
# Defining a decorator
def my_decorator(func):
def wrapper():
print("Something before the function runs.")
func()
print("Something after the function runs.")
return wrapper
# Applying the decorator
@my_decorator
def say_hello():
print("Hello, World!")
say_hello()
Output:
Something before the function runs.
Hello, World!
Something after the function runs.
Anatomy of a Decorator
A typical decorator has three components:
- The Wrapper Function: Defines additional behaviour to execute before or after the target function.
- The Closure: Ensures the wrapper function retains access to the target function.
- The Return Statement: Returns the modified function.
Types of Python Decorators
1. Function Decorators
These are used to modify the behaviour of functions.
Example:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello"
print(greet())
Output:
HELLO
2. Class Decorators
These modify or enhance classes.
Example:
def add_method(cls):
cls.new_method = lambda self: "New Method Added!"
return cls
@add_method
class MyClass:
pass
obj = MyClass()
print(obj.new_method())
Output:
New Method Added!
Common Use Cases for Decorators
- Logging
import time
def log_execution_time(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Execution time: {end_time - start_time} seconds")
return result
return wrapper
@log_execution_time
def slow_function():
time.sleep(2)
return "Done"
print(slow_function())
- Access Control
def require_login(func):
def wrapper(user):
if not user.get('is_logged_in'):
return "Access Denied"
return func(user)
return wrapper
@require_login
def view_dashboard(user):
return "Welcome to the dashboard"
user = {'username': 'John', 'is_logged_in': False}
print(view_dashboard(user))
- Memoization
from functools import lru_cache
@lru_cache(maxsize=32)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(35))
Real-World Case Studies
1. Web Frameworks
Frameworks like Flask and Django rely heavily on decorators for routing.
Example (Flask):
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to the Homepage"
if __name__ == '__main__':
app.run(debug=True)
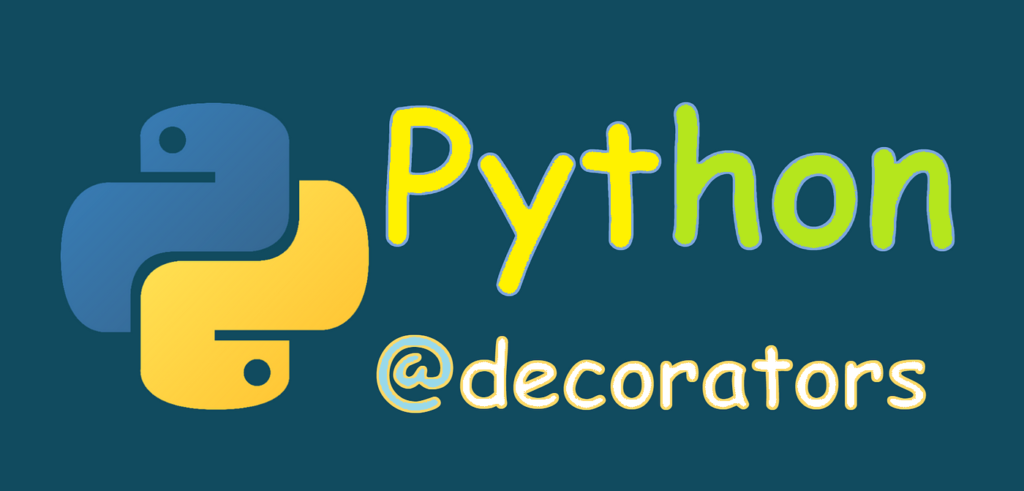
2. Testing Frameworks
Decorators are commonly used for setup and teardown logic in testing frameworks like pytest.
Example:
import pytest
@pytest.fixture
def setup_data():
return {"key": "value"}
def test_example(setup_data):
assert setup_data["key"] == "value"
Top 10 Exclusive Facts About Python Decorators
- Decorators follow the “First-Class Functions” principle in Python, treating functions as objects.
- They can accept arguments themselves, known as parameterized decorators.
- Nested decorators allow stacking multiple behaviour’s.
- Python’s built-in functools.wraps ensures the original function’s metadata is preserved.
- Decorators can be dynamically applied at runtime.
- They are commonly used in meta-programming.
- Python’s standard library includes pre-built decorators like @staticmethod and @classmethod.
- They’re pivotal in asynchronous programming with @asyncio.coroutine (deprecated) and async def.
- Decorators support chaining by returning callable objects.
- They’re widely used in middleware implementations.
FAQs About Python Decorators
- What is a Python decorator?
A decorator is a function that modifies the behaviour of another function or method.
- Why use Python decorators?
To reuse code, enforce behaviour’s, and maintain clean, DRY (Don’t Repeat Yourself) principles.
- Can a decorator take arguments?
Yes, decorators can take arguments when wrapped in another function.
- What is functools.wraps?
A utility to preserve the metadata of the original function when using decorators.
- Can decorators be nested?
Yes, multiple decorators can be applied to a single function.
- What are built-in decorators in Python?
Examples include @staticmethod, @classmethod, and @property.
- Are decorators exclusive to functions?
No, they can also be used on classes.
- What is a closure in the context of decorators?
A closure allows the wrapper function to retain access to the decorated function.
- Can decorators return non-functions?
Yes, they can return any callable object.
- Are decorators compatible with async functions?
Yes, you can decorate asynchronous functions.
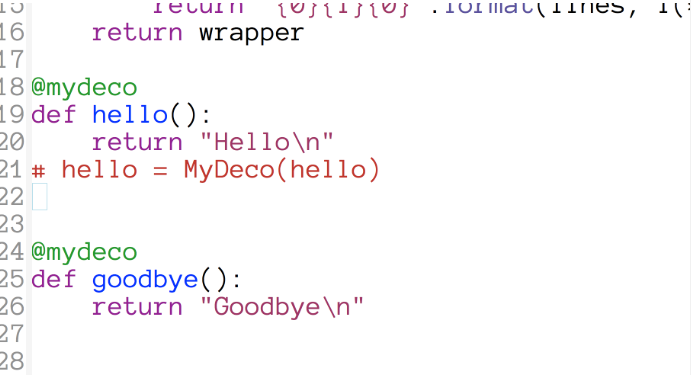
- How are multiple decorators applied?
By stacking them one above the other in the order of execution.
- What is a parameterized decorator?
A decorator that takes arguments to customize its behaviour.
- Can decorators modify function arguments?
Yes, by intercepting and altering arguments in the wrapper function.
- Is there a performance overhead to using decorators?
Minimal overhead; the wrapper function introduces an extra call layer.
- How do you debug decorators?
Use functools.wraps and logging for clarity and metadata preservation.
- What is a class method decorator?
The @classmethod decorator transforms a method into a class method.
- Can decorators be used in inheritance?
Yes, they work seamlessly with inherited methods and classes.
- What is the difference between @staticmethod and @classmethod?
@staticmethod defines a method that doesn’t access class data, while @classmethod passes the class itself as the first argument.
- How do decorators handle exceptions?
Wrapper functions can include exception handling for robustness.
- What are callable objects in decorators?
Objects with a __call__ method, allowing them to be used as functions.
- Can a decorator be conditional?
Yes, by implementing logic in the wrapper to enable or disable behaviour.
- Do decorators change the function signature?
Without functools.wraps, the wrapper’s signature overrides the original.
- Can you decorate lambda functions?
No, as lambda functions cannot have a name for decorators to reference.
- What is functools.lru_cache?
A decorator for caching results of expensive function calls.
- How do you apply a decorator to all methods of a class?
By iterating through the class’s attributes and applying the decorator dynamically.
- What is middleware in the context of decorators?
Middleware uses decorators to wrap functionality for request/response handling.
- Can decorators be used in multithreading?
Yes, decorators can manage thread-safe operations or locks.
Conclusion
Python decorators exemplify the elegance and power of Python’s design philosophy. They offer a clean way to extend and customize the behaviour of functions and methods while maintaining modularity. By mastering decorators, developers can significantly enhance their code’s reusability, readability, and efficiency.
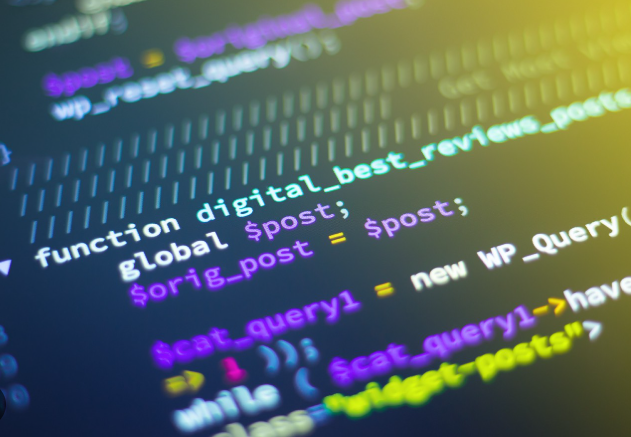
Whether you’re developing a web application, implementing access controls, or optimizing performance, python decorators provide a robust solution. Their prevalence in frameworks like Flask and Django underscores their importance in real-world Python programming. As you continue to explore decorators, you’ll uncover endless possibilities to simplify and streamline your workflows. Embracing this feature not only elevates your skills but also deepens your appreciation for Python’s versatility.
Essential Learning