Traditionally Python is not considered a systems programming language, but it is a versatile language that can be used for a wide range of tasks, including some aspects of systems programming. Systems programming typically involves low-level tasks like operating system development, device driver programming, and hardware control, where performance, memory management, and control over system resources are critical. Lets discover how Python as a systems programming language fairs in a programmers world. While Python may not be the first choice for these specific tasks, it can still play a role in systems programming in the following ways:
- Scripting and Automation: Python is often used for scripting and automating tasks on a system. It can interact with the operating system, manage files, and execute system commands. This makes it valuable for tasks like system administration, configuration management, and deployment automation.
- Prototyping and Proof of Concept: Python’s ease of use and readability make it an excellent choice for quickly prototyping ideas and testing concepts. Many systems software and components are initially prototyped in Python before being implemented in lower-level languages.
- Gluing Different Components: Python can be used to connect and integrate different components of a system. It can interface with libraries and APIs written in other languages, making it a suitable choice for building middleware and bridging components.
- Data Analysis and Visualization: In systems programming, analyzing system performance and resource utilization is critical. Python’s extensive libraries for data analysis, like NumPy, pandas, and Matplotlib, can be used for these purposes.
- Web Services and RESTful APIs: Python can be used to create web services and RESTful APIs that are crucial for modern systems architecture, allowing different components of a system to communicate with each other.
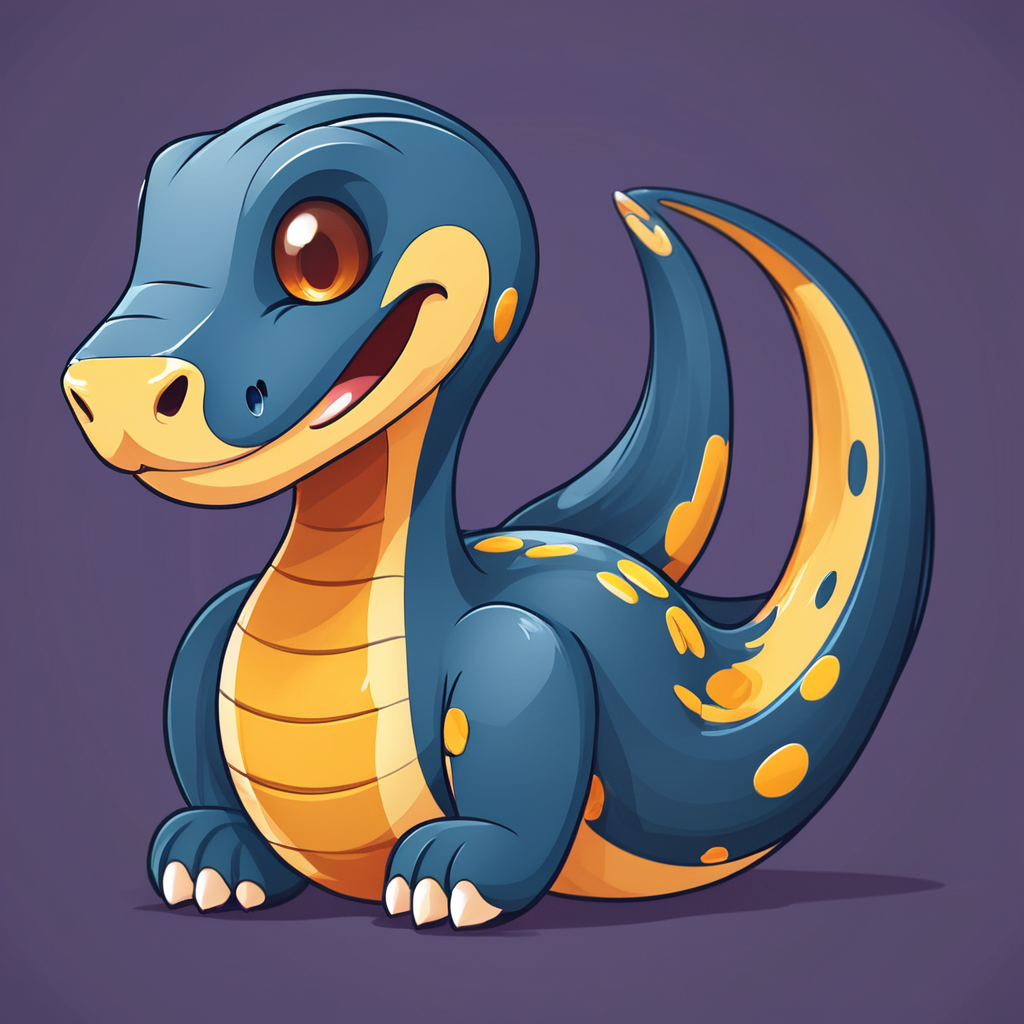
- Cross-Platform Development: Python is a cross-platform language, meaning code written in Python can run on various operating systems with minimal modifications. This can be advantageous in systems programming when portability is required.
- High-Level Abstractions: While systems programming often involves working at a low level, Python provides high-level abstractions that can simplify some aspects of system development. For instance, libraries like asyncio can be used for asynchronous programming, which is essential for building scalable systems.
However, there are some limitations to using Python for systems programming:
- Performance: Python is an interpreted language and generally slower than languages like C or C++ for low-level, performance-critical tasks.
- Memory Management: Python uses automatic memory management (garbage collection), which may not be suitable for tasks that require precise control over memory.
- Limited Hardware Control: Python may not provide the level of control needed for tasks like device driver development.
In nutshell, while Python is not a traditional systems programming language, it can be a valuable tool in the systems programmer’s toolbox, particularly for tasks that involve scripting, automation, prototyping, and high-level system management. For tasks requiring low-level control and maximum performance, other languages like C or Rust are typically preferred.
Python as a gluing language
Using Python as a “gluing” language to integrate and connect various components in a software system is a common practice. It allows you to leverage Python’s simplicity and readability to create interfaces and facilitate communication between different parts of your system. Here’s how you can use Python as a gluing language with some examples:
1. Interfacing with External Programs:
Python can execute external programs and capture their output or input data into your application. This can be useful for integrating third-party tools or services. For example, you can use the `subprocess` module to run a command-line tool and capture its output:
import subprocess
result = subprocess.run([‘ls’, ‘-l’], stdout=subprocess.PIPE)
print(result.stdout.decode(‘utf-8’))
2. Using APIs:
Python can make HTTP requests to RESTful APIs or interact with other APIs using libraries like `requests`. This is particularly handy for integrating web services into your system. Here’s an example using the GitHub API:
import requests
response = requests.get(‘https://api.github.com/users/username’)
data = response.json()
print(data[‘name’])
3. Message Queues:
Python can work with message queuing systems like RabbitMQ, Kafka, or AWS SQS to facilitate communication between different components of your system. Libraries like `pika` (for RabbitMQ) or `kafka-python` (for Kafka) can be used for this purpose.
4. Database Integration:
Python has libraries for connecting to various databases. For instance, you can use `sqlite3` for SQLite, `psycopg2` for PostgreSQL, or `pymysql` for MySQL. This enables you to store and retrieve data from databases in your system.
import sqlite3
conn = sqlite3.connect(‘mydatabase.db’)
cursor = conn.cursor()
cursor.execute(‘SELECT * FROM mytable’)
data = cursor.fetchall()
conn.close()
5. WebSocket Communication:
If your system requires real-time communication, you can use Python libraries like `websockets` to implement WebSocket communication between components.
import asyncio
import websockets
async def handle_client(websocket, path):
async for message in websocket:
await websocket.send(f”Received: {message}”)
start_server = websockets.serve(handle_client, “localhost”, 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
6. Scripting and Automation:
Python can automate tasks by scheduling scripts or using tools like `cron`. This can be helpful for routine system maintenance and data processing.
7. Custom APIs and Middleware:
You can create custom APIs and middleware using Python frameworks like Flask or Django to act as intermediaries between different components, allowing them to communicate efficiently.
By using Python in these ways, you can “glue” together various components in your system, making it easier to build and maintain complex software architectures. Python’s versatility, large standard library, and extensive third-party libraries make it a valuable choice for this purpose.
Example of real-world middleware in python
Let’s do this and utilize flask for this purpose and create an example
To write a custom middleware in Python, you’ll typically be working with web frameworks like Flask or Django. Middleware sits between the incoming HTTP request and the view (or route) and can perform various tasks like authentication, logging, request/response manipulation, and more. Below, I’ll guide you through creating a custom middleware in Flask as an example:
1. Install Flask:
If you haven’t already, install Flask using pip:
pip install Flask
2. Create a Flask Application:
Create a basic Flask application to get started:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
3. Create Custom Middleware:
Now, let's create custom middleware that logs information about each incoming request.
from flask import Flask, request
app = Flask(__name__)
# Custom middleware
@app.before_request
def log_request_info():
"""
This function will be executed before every request.
It logs information about the incoming request.
"""
path = request.path
method = request.method
headers = request.headers
print(f"Received {method} request for {path}")
print(f"Headers: {headers}")
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
In this example, `@app.before_request` is a decorator provided by Flask, which registers the `log_request_info` function to run before every request.
4. Run the Application:
Save the code to a Python file (e.g., `app.py`) and run it:
python app.py
Now, when you access the root URL (`http://localhost:5000/`), you’ll see log messages in the terminal about each incoming request.
You can expand upon this middleware by adding more functionality, such as:
- Implementing authentication checks.
- Modifying the request or response objects.
- Handling error conditions.
- Storing data in a database or external service.
It is important to remember that this is just a basic example of custom middleware in Flask. In a real-world scenario, you might need more complex logic and possibly additional middleware components to handle various aspects of your application. The key is to define functions or classes that interact with the request and response objects within the framework’s middleware system.
Writing APIs using python
Yes, you can absolutely write an API using Python. Python is a popular choice for building APIs due to its simplicity, readability, and the availability of powerful web frameworks. Two of the most commonly used web frameworks for building APIs in Python are Flask and Django. Here’s a brief overview of both options:
1. Flask:
Flask is a lightweight micro-framework for building web applications, including APIs. It’s known for its simplicity and flexibility, making it a popular choice for small to medium-sized projects and rapid development. To create a basic API using Flask, you can follow these steps:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route(‘/api/hello’, methods=[‘GET’])
def hello():
return jsonify(message=’Hello, World!’)
if __name__ == ‘__main__’:
app.run(debug=True)
You can then run this Flask application, and it will provide an API endpoint at `http://localhost:5000/api/hello`.
2. Django:
Django is a more comprehensive web framework that includes many built-in features and tools for building robust web applications, including APIs. Django REST framework (DRF) is a popular extension for building APIs with Django. Here’s a simple example of creating an API endpoint using DRF:
from django.urls import path
from rest_framework.response import Response
from rest_framework.decorators import api_view
@api_view([‘GET’])
def hello(request):
return Response({‘message’: ‘Hello, World!’})
urlpatterns = [
path(‘api/hello’, hello),
]
This is just a small part of what Django and DRF can do. They offer a wide range of features for building APIs, including authentication, serialization, and more.
When building an API in Python, you can also use various third-party libraries and tools to simplify common tasks. For example:
– SQLAlchemy: For working with databases.
– Flask-RESTful or FastAPI: Frameworks/extensions built specifically for building APIs.
– Swagger/OpenAPI: For API documentation.
– Authentication libraries: Like OAuth2 or JWT for securing your API.
Your choice between Flask, Django, or another framework depends on the complexity of your project, your familiarity with the framework, and your specific requirements. Both Flask and Django are capable of building production-ready APIs, so you can choose the one that best fits your needs.
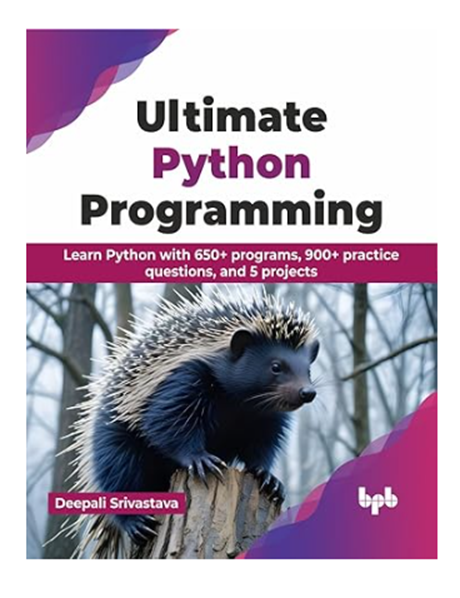
Ultimate Python Programming: Learn Python with 650+ programs, 900+ practice questions, and 5 projects
A detailed example
Below is a complete example of creating a Python Flask API for user login and logging. The example includes user authentication using a simple dictionary-based user database and logs login activities.
from flask import Flask, request, jsonify
app = Flask(__name__)
# Simulated user database (replace with a real database in production)
users = {
'user1': {'username': 'user1', 'password': 'password1'},
'user2': {'username': 'user2', 'password': 'password2'}
}
# Log storage (you can use a proper logging library in a production app)
logs = []
# Route for user login
@app.route('/login', methods=['POST'])
def login():
data = request.get_json()
username = data.get('username')
password = data.get('password')
# Check if the user exists in the database
if username in users and users[username]['password'] == password:
# Log the successful login
logs.append(f"User '{username}' logged in.")
return jsonify({'message': 'Login successful'}), 200
else:
# Log the failed login attempt
logs.append(f"Failed login attempt for user '{username}'.")
return jsonify({'message': 'Login failed'}), 401
# Route to retrieve login logs
@app.route('/logs', methods=['GET'])
def get_logs():
return jsonify({'logs': logs})
if __name__ == '__main__':
app.run(debug=True)
In this example:
1. We create a Flask application and define two routes: `/login` for user login and `/logs` for retrieving login logs.
2. A simulated user database (`users`) stores user information with usernames and passwords. In a real-world application, you’d use a proper database system.
3. The `/login` route accepts POST requests with JSON data containing the username and password. It validates the credentials against the user database and logs the login activity (successful or failed).
4. The `/logs` route allows retrieving the login logs.
Okay please remember that this is a simplified example for demonstration purposes. In a production application, you should implement more secure authentication mechanisms, use secure password storage, and handle user sessions to manage logins properly. Additionally, consider using a logging library like Python’s built-in `logging` module for comprehensive logging.
We may conclude by saying that – Python, while not traditionally categorized as a systems programming language, offers a versatile set of capabilities that can be effectively harnessed in system-related tasks. Its strengths lie in its simplicity, readability, and a wide range of libraries and frameworks that enable it to play a crucial role in system development. Python can serve as a scripting language for automation, act as a glue between different system components, and be utilized for prototyping and proof of concept.
However, it’s important to acknowledge that Python does have limitations when compared to lower-level languages like C or Rust, particularly in terms of performance and memory management. In scenarios where precise control over hardware resources is essential, Python may not be the first choice.
Nonetheless, Python’s adaptability and cross-platform compatibility make it a valuable asset for developers working on a variety of system-related tasks, from web services and API development to data analysis and automation. Its role in system programming is evolving, thanks in part to ongoing improvements in performance and interoperability with lower-level languages. Ultimately, the choice of Python as a system programming language depends on the specific requirements of the project and the trade-offs between ease of development and performance optimization.
Curated Reads