Low-Level Programming Language
In the early 1970s, the computing world was rapidly evolving, and there was a pressing need for a powerful, yet flexible programming language that could bridge the gap between high-level languages and assembly language. At Bell Labs, Dennis Ritchie and his team were working on the Unix operating system, which required a language capable of both system programming and efficient hardware manipulation. Existing languages at the time, like B and assembly, were either too low-level, leading to complex and error-prone code, or lacked the performance needed for operating systems. The creation of C addressed these issues by combining the efficiency and control of assembly language with the readability and structure of high-level languages. C is often treated as low level language. It introduced key features like direct memory access, pointer arithmetic, and a rich set of operators, which enabled precise hardware manipulation while maintaining a level of abstraction that simplified programming tasks. Additionally, C’s portability allowed Unix, and subsequently other software, to be easily adapted across different hardware platforms. This combination of power, efficiency, and flexibility made C an indispensable tool in the development of operating systems, compilers, and embedded systems, securing its place as a foundational language in the world of computing.
In the realm of programming languages, C holds a distinctive place, often referred to as a low-level language that bridges the gap between hardware and software. Since its inception in the early 1970s by Dennis Ritchie at Bell Labs, C has cemented its position as a cornerstone of modern computing. Despite the advent of newer, high-level languages, C’s relevance persists due to its unparalleled control over hardware, efficient memory management, and the performance optimization it offers. This guide aims to delve into why C remains a preferred choice for system programming, embedded systems, and performance-critical applications, while also comparing it with other prominent low-level languages.
What is a Low-Level Language?
Before diving into the specifics of C, it’s essential to understand what constitutes a low-level language. Unlike high-level languages, which are designed to be easy for humans to read and write, low-level languages operate closer to machine code. They provide little abstraction from a computer’s instruction set architecture, which means the programmer has more control over the hardware. This control is a double-edged sword; while it allows for precise optimization, it also requires a deeper understanding of the hardware and can lead to more complex and error-prone code.
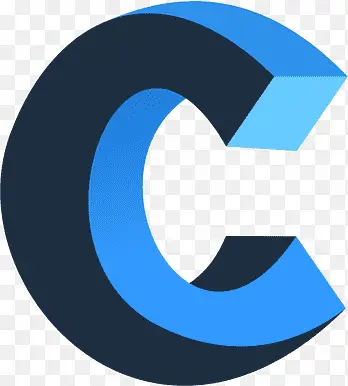
The Origins and Evolution of C
C was developed as a system programming language for the Unix operating system. Its design was influenced by the earlier language B, and it incorporated features that were essential for low-level programming while also providing constructs that supported structured programming. Over the decades, C has evolved through various standards, with ANSI C (C89), ISO C (C90), C99, C11, and the latest C18, each iteration bringing enhancements and new features while maintaining the core principles that make C a powerful tool for system-level programming.
Key Features of C
- Direct Memory Access: C allows direct manipulation of memory addresses, which is crucial for system-level programming. This feature provides the programmer with the ability to manage hardware resources efficiently.
- Pointer Arithmetic: C’s support for pointers is one of its defining features. Pointers offer a way to dynamically manage memory, create complex data structures like linked lists and trees, and interact directly with hardware.
- Portability: Although C is considered a low-level language, it is also highly portable. Programs written in C can be compiled and run on a wide variety of machines with minimal changes, making it an ideal choice for cross-platform development.
- Efficiency: C is designed for performance. Its constructs map efficiently to machine instructions, allowing for fine-grained optimization that can significantly boost execution speed.
- Rich Library Support: The C Standard Library provides a wealth of functions for performing common tasks, ranging from input/output operations to complex mathematical computations, which simplifies development and increases productivity.
Why Choose C?
1. System-Level Programming
C’s low-level capabilities make it an ideal choice for system programming, including operating systems, embedded systems, and real-time applications. The Unix operating system, various Linux distributions, and many real-time operating systems are written in C, demonstrating its dominance in this domain.
2. Performance-Critical Applications
When performance is paramount, C is often the language of choice. Its ability to directly interact with hardware, coupled with fine-grained control over system resources, makes it suitable for applications where speed and efficiency are critical. Examples include game development, high-frequency trading systems, and scientific computing.
3. Embedded Systems
C’s proximity to hardware and minimal runtime overhead make it a popular choice for embedded systems. From microcontrollers in household appliances to complex automotive control systems, C is the go-to language for developing firmware that operates under stringent resource constraints.
4. Learning and Teaching
Despite its complexity, C is often recommended as a first language for learning programming. Understanding C provides a solid foundation in computer science principles, such as memory management, data structures, and algorithms, which are transferable to other languages and paradigms.
Comparative Analysis of Low-Level Languages
To understand C’s standing among low-level languages, it’s insightful to compare it with other prominent low-level languages: Assembly, Rust, and C++. The table below provides a comparative analysis based on various criteria.
Feature | C | Assembly | Rust | C++ |
---|---|---|---|---|
Abstraction Level | Low | Very Low | Low-Mid | Low-Mid |
Memory Management | Manual (via pointers) | Manual (registers) | Automatic (ownership) | Manual/Automatic (RAII) |
Performance | High | Very High | High | High |
Safety | Low | Very Low | High (borrow checker) | Medium |
Ease of Use | Moderate | Difficult | Moderate | Moderate |
Portability | High | Low | High | High |
Learning Curve | Steep | Very Steep | Moderate | Steep |
Use Cases | Systems, Embedded | Systems | Systems, Web, Embedded | Systems, Applications |
Concurrency Support | Limited | None | Strong (built-in) | Strong (libraries) |
Community & Ecosystem | Mature, Extensive | Niche, Limited | Growing, Supportive | Mature, Extensive |
In-Depth Comparison
Assembly Language
Abstraction Level and Control: Assembly language provides the lowest level of abstraction, offering direct control over hardware through instructions specific to the CPU architecture. This level of control is unmatched, allowing for extremely fine-tuned optimizations. However, it comes at the cost of complexity and portability.
Performance and Efficiency: As the closest language to machine code, Assembly offers the highest performance and efficiency. Each line of Assembly code corresponds directly to a machine instruction, ensuring optimal use of hardware resources.
Memory Management: Memory management in Assembly is entirely manual. Programmers must allocate and manage memory, which can lead to efficient but error-prone code. This lack of abstraction is both a strength and a weakness.
Portability: Assembly language is inherently non-portable. Code written for one CPU architecture will not run on another without significant modification. This limits its use in cross-platform applications.
Ease of Use and Learning Curve: Learning Assembly is challenging due to its low-level nature. It requires an in-depth understanding of computer architecture and instruction sets, making it less accessible to beginners.
Rust
Safety and Memory Management: Rust introduces the concept of ownership with a sophisticated borrow checker to ensure memory safety and prevent data races. This system allows Rust to manage memory automatically while still offering performance close to that of C and C++.
Performance: Rust is designed for high performance, and its safety features do not significantly impact its speed. It achieves a good balance between low-level control and high-level safety, making it suitable for system programming.
Concurrency Support: Rust excels in concurrency with built-in support for concurrent programming. Its ownership model ensures that data races are caught at compile time, making concurrent programming safer and more reliable.
Ease of Use and Learning Curve: While Rust’s syntax is more modern and user-friendly than C’s, the ownership model introduces a learning curve. However, once mastered, it provides powerful tools for safe and efficient system programming.
C++
Abstraction Level: C++ builds upon C by adding object-oriented features and higher-level abstractions. This allows for more structured and reusable code, although it comes at the cost of increased complexity.
Memory Management: C++ offers both manual and automatic memory management. The RAII (Resource Acquisition Is Initialization) principle helps manage resources, while smart pointers provide automatic memory management, reducing the risk of memory leaks.
Performance: C++ retains the high performance of C while providing additional features for code optimization. The ability to use inline Assembly code allows for critical sections to be highly optimized.
Safety: While C++ improves safety over C with features like smart pointers and exception handling, it still allows for unsafe operations, and the programmer must be vigilant to avoid common pitfalls such as buffer overflows and dangling pointers.
Community and Ecosystem: C++ has a mature and extensive ecosystem with a vast array of libraries and frameworks. Its community is active and supportive, providing ample resources for learning and problem-solving.
Summary
I’ld say give me one language forever and I would stick to it. Some folks might ask some great questions and I myself had curiosity about it. Check for instance Voyager 1 and 2 .. They were written in legacy languages and they still kick the ass.
Is C Used in Aerospace Programming?
Yes, the C programming language is widely used in aerospace programming. The aerospace industry demands highly reliable, efficient, and performance-critical software systems, making C an ideal choice due to its low-level capabilities, direct hardware manipulation, and efficient memory management.
Does NASA Use C?
NASA extensively uses the C programming language for its software development. Many of NASA’s systems, from spacecraft and satellite control systems to ground control and data processing systems, rely on C for its robustness and efficiency. The simplicity and direct control over hardware provided by C are crucial for the stringent requirements of space missions where reliability and performance are paramount.
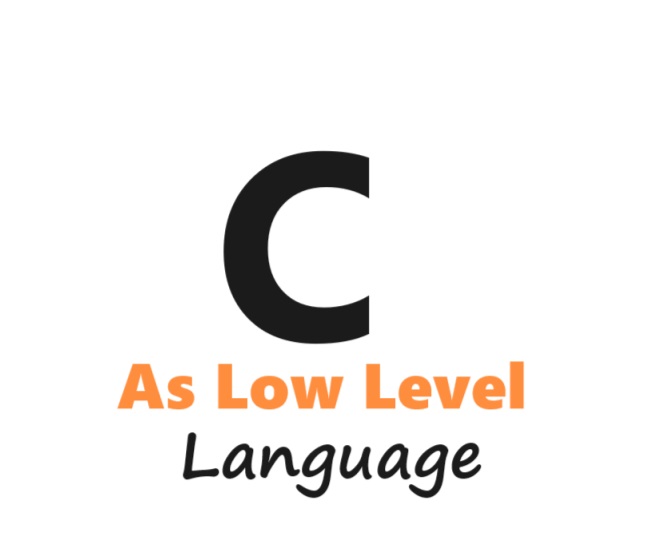
Who Else Uses C Today?
Apart from NASA, C is utilized by various other organizations and industries for its unparalleled performance and control over hardware. Some notable users include:
- Embedded Systems: C is a dominant language in the development of embedded systems used in automotive electronics, medical devices, consumer electronics, and industrial control systems. Companies like Intel, Qualcomm, and Bosch use C for firmware and hardware interfacing.
- Operating Systems: Many operating systems, including Linux, Windows, and various real-time operating systems, have components written in C. The Linux kernel, in particular, is primarily developed in C, highlighting its critical role in system programming.
- Telecommunications: The telecommunications industry relies on C for the development of network protocols, routers, and switches. Companies such as Cisco and Ericsson use C for performance-critical applications that manage vast amounts of data traffic.
- Finance and Trading: High-frequency trading systems and financial modeling applications require the speed and efficiency provided by C. Financial institutions and trading firms use C to develop software that can process large datasets with minimal latency.
- Game Development: While higher-level languages and engines are popular in game development, C is still used for performance-intensive components like physics engines, graphics rendering, and real-time simulations. Companies like EA and Ubisoft utilize C for such purposes.
- Academic and Research: Many academic institutions and research labs use C for teaching computer science fundamentals, as well as for developing algorithms and simulations in various scientific fields.
In summary, C continues to be a cornerstone in various industries due to its efficiency, control, and versatility, making it indispensable in aerospace programming and beyond.
C remains a vital language in the programming landscape due to its unparalleled control over hardware, efficiency, and portability. Its role in system-level programming, performance-critical applications, and embedded systems is irreplaceable. While newer languages like Rust offer enhanced safety features and modern conveniences, C’s simplicity and directness keep it relevant for many use cases. Understanding C not only provides a solid foundation in programming principles but also opens the door to mastering other languages and technologies. Whether you are a novice looking to learn the fundamentals or a seasoned programmer optimizing performance-critical code, C offers a robust and enduring platform to build upon.
Curated for you:
C vs. C++: The Evolution of Programming Languages – LearnXYZ
It appears that you know a lot about this topic. I expect to learn more from your upcoming updates. Of course, you are very much welcomed to my website 67U about Online Business.
Thank you for writing this post!