Today digital-first world, data is the lifeblood of businesses. From multinational corporations to small startups, every organization relies on databases to manage and secure critical information. Among the various database technologies available, Oracle Database stands out as one of the most robust and widely adopted solutions. The role of an Oracle Database Administrator (DBA) is pivotal in ensuring the smooth operation, maintenance, and optimization of these complex systems.
Oracle Database technology has revolutionized how data is stored, accessed, and analyzed. With its rich features like pluggable databases, Real Application Clusters (RAC), and autonomous data processing, Oracle has established itself as a leader in the database management landscape. For organizations managing vast amounts of structured and unstructured data, the expertise of an Oracle DBA is indispensable.
This article dives into the fundamentals of Oracle DBA, its history, practical usage, and how to implement Oracle Database using Python. We’ll also explore how to perform CRUD (Create, Read, Update, Delete) operations using Python, giving a hands-on approach to real-world application.
Comprehensive Table of Contents
Oracle DBA and Database Technology.
Responsibilities of an Oracle DBA.
Using Oracle Database with Python: Hands-On Examples.
Installing Required Libraries.
Creating a Customized Oracle Database.
Basic CRUD Operations in Python for Oracle Database.
5. Searching and Filtering Data.
8. Filtering with Multiple Conditions (AND, OR)
9. Search with Python (Example)
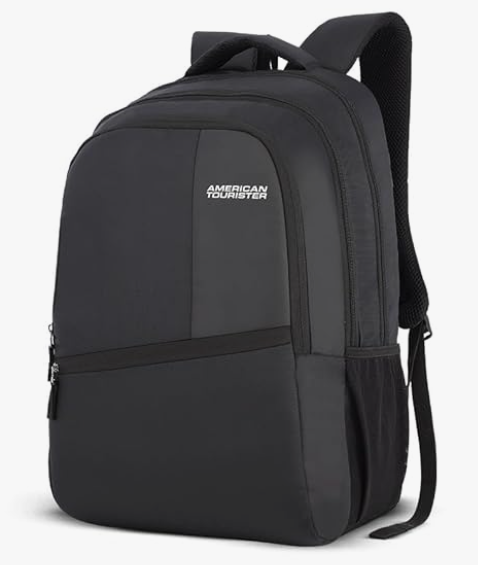
American Tourister Valex 28 Ltrs Large Laptop Backpack
Limited time deal
Priced at -44% discount for ₹1,399
What is Oracle DBA?
An Oracle Database Administrator (DBA) is a professional responsible for the installation, configuration, upgrading, monitoring, and maintenance of Oracle Database systems. Their expertise ensures the reliability, availability, and performance of the database infrastructure.
History of Oracle Database
Oracle Database traces its roots to 1977 when Larry Ellison, Bob Miner, and Ed Oates founded Relational Software Inc., later rebranded as Oracle Corporation. Inspired by Edgar F. Codd’s relational database model, the team developed the first commercially available relational database management system (RDBMS). Over the decades, Oracle evolved to become a multi-model database supporting relational, NoSQL, and blockchain formats.
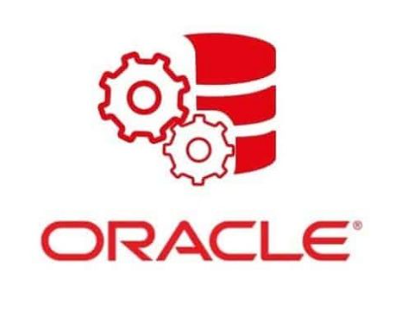
Where Oracle DBA is Used
Oracle DBAs are essential in sectors that require robust and secure data management solutions. Industries such as:
- Finance: Banks rely on Oracle for secure transactional processing and fraud detection.
- Healthcare: Medical systems use Oracle to manage patient records and streamline operations.
- Retail: Large-scale inventory management and sales analytics are powered by Oracle Databases.
- Government: Public sectors employ Oracle for citizen data management and large-scale e-governance projects.
- Telecommunications: Call detail records, billing, and network monitoring depend on Oracle’s reliability.
Responsibilities of an Oracle DBA
- Installing and upgrading Oracle software.
- Configuring database settings for optimal performance.
- Managing user access and database security.
- Backing up and recovering data to prevent loss.
- Monitoring database performance and applying necessary tuning.
- Managing storage, schemas, and database instances.
Using Oracle Database with Python: Hands-On Examples
Python, with its rich set of libraries, provides robust tools to interact with Oracle Databases. cx_Oracle, a widely used Python library, allows developers to execute SQL queries, manage connections, and handle database transactions seamlessly. Below, we demonstrate how to create a customized Oracle Database and perform basic CRUD operations using Python.
Installing Required Libraries
Before beginning, ensure you have Python installed. Use pip to install the necessary library:
pip install cx_Oracle
Creating a Customized Oracle Database
Here’s an example script to establish a new Oracle Database using Python:
import cx_Oracle
# Oracle Database Configuration
host = "localhost"
port = 1521
service_name = "orcl"
admin_user = "system"
admin_password = "oracle_password"
# Create connection string
dsn = cx_Oracle.makedsn(host, port, service_name=service_name)
try:
# Connect as SYSDBA
connection = cx_Oracle.connect(user=admin_user, password=admin_password, dsn=dsn, mode=cx_Oracle.SYSDBA)
cursor = connection.cursor()
# Create a new database user
cursor.execute("CREATE USER my_user IDENTIFIED BY my_password")
print("User 'my_user' created successfully.")
# Grant privileges
cursor.execute("GRANT CONNECT, RESOURCE TO my_user")
print("Privileges granted to 'my_user'.")
# Create a sample table
cursor.execute("""
CREATE TABLE my_user.sample_table (
id NUMBER PRIMARY KEY,
name VARCHAR2(50),
created_at DATE DEFAULT SYSDATE
)
""")
print("Table 'sample_table' created successfully.")
except cx_Oracle.Error as error:
print(f"Error occurred: {error}")
finally:
if cursor:
cursor.close()
if connection:
connection.close()
Basic CRUD Operations in Python for Oracle Database
Now that we’ve created a database, let’s explore how to perform basic CRUD operations (Create, Read, Update, Delete) on it.
1. Inserting Data (Create)
import cx_Oracle
def insert_data():
dsn = cx_Oracle.makedsn("localhost", 1521, service_name="orcl")
connection = cx_Oracle.connect(user="my_user", password="my_password", dsn=dsn)
cursor = connection.cursor()
try:
cursor.execute("INSERT INTO sample_table (id, name) VALUES (:1, :2)", (1, 'Alice'))
connection.commit()
print("Data inserted successfully.")
except cx_Oracle.Error as error:
print(f"Error occurred: {error}")
finally:
cursor.close()
connection.close()
insert_data()
2. Reading Data (Read)
def read_data():
dsn = cx_Oracle.makedsn("localhost", 1521, service_name="orcl")
connection = cx_Oracle.connect(user="my_user", password="my_password", dsn=dsn)
cursor = connection.cursor()
try:
cursor.execute("SELECT * FROM sample_table")
rows = cursor.fetchall()
for row in rows:
print(row)
except cx_Oracle.Error as error:
print(f"Error occurred: {error}")
finally:
cursor.close()
connection.close()
read_data()
3. Updating Data (Update)
def update_data():
dsn = cx_Oracle.makedsn("localhost", 1521, service_name="orcl")
connection = cx_Oracle.connect(user="my_user", password="my_password", dsn=dsn)
cursor = connection.cursor()
try:
cursor.execute("UPDATE sample_table SET name = :1 WHERE id = :2", ('Bob', 1))
connection.commit()
print("Data updated successfully.")
except cx_Oracle.Error as error:
print(f"Error occurred: {error}")
finally:
cursor.close()
connection.close()
update_data()
4. Deleting Data (Delete)
def delete_data():
dsn = cx_Oracle.makedsn("localhost", 1521, service_name="orcl")
connection = cx_Oracle.connect(user="my_user", password="my_password", dsn=dsn)
cursor = connection.cursor()
try:
cursor.execute("DELETE FROM sample_table WHERE id = :1", (1,))
connection.commit()
print("Data deleted successfully.")
except cx_Oracle.Error as error:
print(f"Error occurred: {error}")
finally:
cursor.close()
connection.close()
delete_data()
5. Searching and Filtering Data
To search and find data from a database, one typically uses SQL (Structured Query Language) commands. These commands allow querying a database to retrieve specific data based on various criteria. In Oracle Database, the most common command for searching and finding data is the SELECT statement. Below is a detailed guide on how to search and retrieve data using SQL commands:
The SELECT Statement
The SELECT statement is used to retrieve data from one or more tables in a database.
Basic Syntax
SELECT column1, column2, …
FROM table_name;
Example
Suppose you have a table named employees with the following columns: employee_id, first_name, last_name, department_id. To retrieve all data from the employees table:
SELECT * FROM employees;
The * symbol retrieves all columns.
Adding Filters with the WHERE Clause
The WHERE clause is used to filter results based on specified conditions.
Syntax
SELECT column1, column2
FROM table_name
WHERE condition;
Example
Retrieve all employees from the employees table who belong to department 10:
SELECT first_name, last_name
FROM employees
WHERE department_id = 10;
Using Wildcards for Partial Matching (LIKE Operator)
The LIKE operator is useful for searching data with partial matches.
Syntax
SELECT column1
FROM table_name
WHERE column_name LIKE pattern;
Wildcards in LIKE
- % represents zero or more characters.
- _ represents a single character.
Example
Retrieve all employees whose first name starts with “J”:
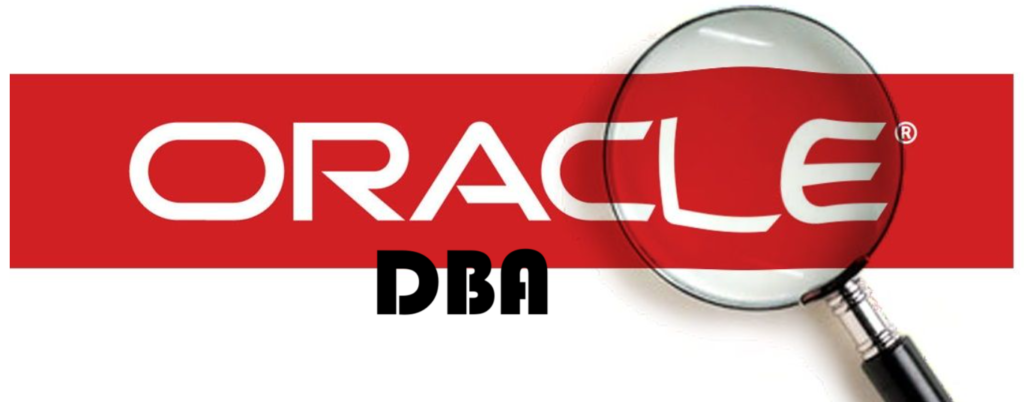
SELECT first_name, last_name
FROM employees
WHERE first_name LIKE 'J%';
Sorting Results with ORDER BY
The ORDER BY clause arranges the result set in ascending (ASC) or descending (DESC) order.
Syntax
ORDER BY column_name ASC|DESC;
SELECT column1, column2
FROM table_name
Example
Retrieve all employees sorted by their last name:
SELECT first_name, last_name
FROM employees
ORDER BY last_name ASC;
Retrieving Unique Records with DISTINCT
The DISTINCT keyword eliminates duplicate rows in the result set.
Example
Retrieve all unique department IDs from the employees table:
SELECT DISTINCT department_id
FROM employees;
6. Math Operations
Aggregating Data with Functions (COUNT, SUM, AVG, MAX, MIN)
Aggregate functions help summarize data.
Example
Count the total number of employees:
SELECT COUNT(*) AS total_employees
FROM employees;
Retrieve the maximum salary in the employees table:
SELECT MAX(salary) AS highest_salary
FROM employees;
7. Joins
Combining Data from Multiple Tables (JOIN)
The JOIN clause is used to combine rows from two or more tables based on a related column.
Example
Retrieve all employee names along with their department names (assuming departments table exists):
SELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
JOIN departments
ON employees.department_id = departments.department_id;
8. Filtering with Multiple Conditions (AND, OR)
Use AND and OR to combine multiple conditions.
Example
Retrieve employees from department 10 or with a salary greater than 50,000:
SELECT first_name, last_name
FROM employees
WHERE department_id = 10 OR salary > 50000;
9. Search with Python (Example)
If you’re using Python, you can also query data programmatically using libraries like cx_Oracle.
Python Example for Searching Data
import cx_Oracle
dsn = cx_Oracle.makedsn("localhost", 1521, service_name="orcl")
connection = cx_Oracle.connect(user="my_user", password="my_password", dsn=dsn)
cursor = connection.cursor()
try:
cursor.execute("SELECT first_name, last_name FROM employees WHERE department_id = :dept_id", {"dept_id": 10})
rows = cursor.fetchall()
for row in rows:
print(row)
except cx_Oracle.Error as error:
print(f"Error occurred: {error}")
finally:
cursor.close()
connection.close()
This script queries data for employees in department 10 using a parameterized query.

Key Takeaways
- Use SELECT to retrieve data from tables.
- Add filters with WHERE and combine conditions with AND or OR.
- Use LIKE for partial matches and wildcards for flexible searches.
- Sort results using ORDER BY and summarize with aggregate functions like COUNT or MAX.
- Combine data across tables with JOIN.
These SQL commands provide powerful tools for searching and finding data in an Oracle Database or any relational database system.
Conclusion
Oracle DBA and database technology are indispensable for modern businesses managing critical data at scale. With Oracle Database’s powerful features and the flexibility of Python programming, developers and administrators can achieve robust, scalable, and secure database systems. This combination provides an efficient pathway for implementing sophisticated data solutions tailored to organizational needs. Whether you are a seasoned DBA or a Python developer exploring Oracle, the possibilities are limitless.
Good Database Reads