A dexterous start..
Object Oriented Programming (OOP) is one of the most fundamental paradigms in modern software development. It allows developers to model real-world entities using code, creating a more intuitive and scalable way to manage complex applications. We finished our previous session on Python Basics a few days ago. You can access the articles and free PDF book for download here. Today we dive into the Object Oriented Realm of Programming languages. This article explains OOPs in the most generic way possible. So the concepts should be applicable to any OOPS language out there. Object Oriented Programming in Python series will bring special focus on OOP with the language. OOP plays a pivotal role due to Python’s flexible nature and its focus on readability and simplicity. It is exciting way of writing programs where all your code is arranged in a proper way with a few rule to make your coding life easier.
This article delves deep into the concept of OOP, focusing on its features, core principles, and impact in Python. It will also explore why OOP is crucial in modern programming and how Python leverages it for software design.
What is Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. In OOP, objects represent entities or concepts that possess attributes (data) and behaviors (methods).
Python, being a high-level and versatile programming language, fully embraces the OOP paradigm. Its OOP features are designed to be intuitive and straightforward, making it easier for developers to implement real-world models and systems.
The primary objective of OOP is to group related data and functions in a way that enhances modularity, reuse, and scalability. By encapsulating related logic and behavior into objects, developers can build systems that are easy to maintain, modify, and extend over time.
Table of Contents
What is Object-Oriented Programming (OOP)
Core Concepts of Object-Oriented Programming.
How OOP Enhances Software Design.
Advantages of Using OOP in Python.
Real-World Applications of OOP in Python.
Best Practices for OOP in Python.
Creative and Artful Ways to Write Object-Oriented Programming (OOP) in Python plus.
1. Think in Terms of Real-World Objects.
2. Use Design Patterns Creatively.
3. Leverage Magic Methods for Operator Overloading.
4. Use Composition Over Inheritance When Appropriate.
5. Polymorphism for Maximum Flexibility.
6. Abstract Classes to Enforce Structure.
7. Dynamic Class Creation with type()
8. Make Use of Property Decorators for Elegant Getters and Setters.
Core Concepts of Object-Oriented Programming
Understanding the core concepts of OOP is essential to mastering object-oriented design in Python. These concepts include:
- Classes and Objects
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Classes and Objects
In OOP, classes are blueprints or templates used to create objects. A class defines the properties and behaviors that an object created from that class will have. An object is an instance of a class and represents an individual unit that holds data (attributes) and functions (methods).
Example:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return f"{self.name} is barking!"
# Creating an object
my_dog = Dog("Rex", "Labrador")
print(my_dog.bark())
In this example, the class Dog serves as a template, and my_dog is an instance of that class. The object my_dog contains attributes like name and breed, and behaviors like bark().
Encapsulation
Encapsulation refers to the concept of bundling data and methods within a class while restricting access to some of the class’s components. It allows for data protection by controlling how the internal attributes of an object can be accessed or modified. Encapsulation in Python is achieved using access specifiers like private (__) or protected (_) members.
Example:
class BankAccount:
def __init__(self, balance):
self.__balance = balance
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance
# Encapsulation in action
account = BankAccount(1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
Here, the balance is encapsulated and can only be modified through specific methods, ensuring data protection.
Inheritance
Inheritance allows one class (the child or derived class) to inherit attributes and behaviors from another class (the parent or base class). This promotes code reuse and establishes a hierarchical relationship between classes.
Example:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclass must implement abstract method")
class Dog(Animal):
def speak(self):
return f"{self.name} barks"
class Cat(Animal):
def speak(self):
return f"{self.name} meows"
# Inheritance in action
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy barks
print(cat.speak()) # Output: Whiskers meows
In this case, Dog and Cat classes inherit from the Animal class and provide their specific implementations of the speak method.
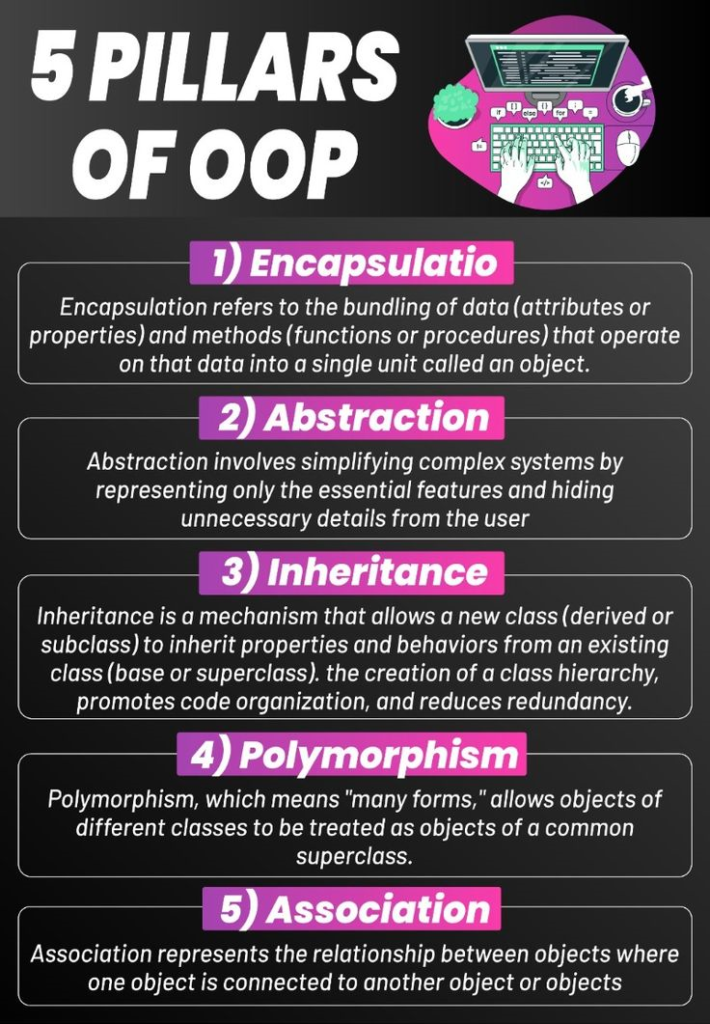
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It provides flexibility in method implementation and allows different objects to respond to the same method in different ways.
Example:
def animal_sound(animal):
print(animal.speak())
# Polymorphism in action
dog = Dog("Buddy")
cat = Cat("Whiskers")
animal_sound(dog) # Output: Buddy barks
animal_sound(cat) # Output: Whiskers meows
Here, both dog and cat respond to the same speak method in their unique ways, demonstrating polymorphism.
Abstraction
Abstraction hides the internal complexity of systems and only exposes the essential features. In Python, abstraction is achieved through the use of abstract classes, where abstract methods are defined but not implemented. Subclasses are expected to provide concrete implementations for these methods.
Example:
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
# Abstraction in action
rect = Rectangle(10, 5)
print(rect.area()) # Output: 50
The abstract class Shape serves as a template for specific shapes like Rectangle, enforcing the structure of the area method.
Why OOP Matters in Python
Python’s support for OOP makes it a powerful and versatile language. Python was designed with simplicity and clarity in mind, and OOP fits naturally into that design philosophy. OOP provides several advantages when working with Python, including:
- Improved Code Readability: Python’s clean syntax combined with OOP principles makes the code more readable and maintainable.
- Reusability: By allowing developers to reuse classes and methods, OOP helps in reducing redundant code.
- Scalability: Python’s OOP model allows for building complex applications that can scale easily.
- Modularity: Python’s OOP model encourages modular code, making debugging, testing, and maintaining systems easier.
These benefits underscore the importance of mastering OOP in Python, especially for developers aiming to work on larger, more complex projects.
How OOP Enhances Software Design
Object-Oriented Programming in Python significantly enhances software design in several key ways:
1. Modularity and Organization
OOP encourages the design of modular systems. Each class represents a single concept, and methods within those classes represent the behavior related to that concept. This separation of concerns makes the codebase easier to navigate and manage.
2. Code Reusability
Through inheritance and polymorphism, OOP promotes code reuse. Developers can create a base class that defines common functionality, and specific subclasses can inherit or override this functionality as needed. This prevents code duplication and allows faster development.
3. Maintenance and Extensibility
When new features or updates are needed, OOP systems are much easier to modify. Changes in one part of the codebase are unlikely to affect other areas because of the encapsulated structure. Additionally, new classes and features can be added without altering existing code.
4. Encouraging Best Practices
OOP encourages developers to follow best practices such as DRY (Don’t Repeat Yourself) and Single Responsibility Principle. This leads to cleaner, more maintainable code over time.
5. Design Patterns
OOP aligns with many design patterns such as Singleton, Factory, and Observer, which are widely used in Python projects to solve common design problems.
Advantages of Using OOP in Python
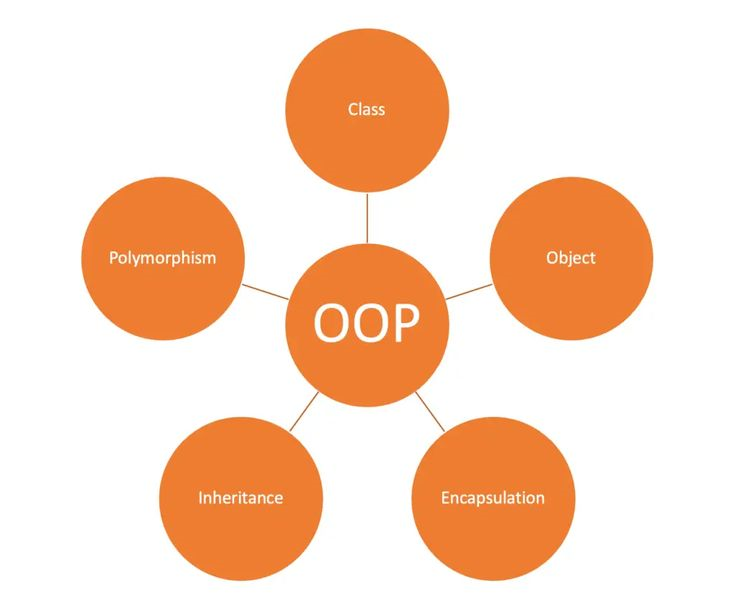
- Natural and Intuitive Syntax: Python’s syntax for OOP is straightforward and natural, making it easier for developers to understand the concept of objects and classes.
- Powerful Libraries and Frameworks: Many Python libraries and frameworks are built around OOP principles. For instance, Django, a popular web framework, is heavily object-oriented, making OOP essential for web developers in Python.
- Facilitates Collaboration: Python’s OOP model makes it easier for teams to collaborate on large projects. Clear class structures, inheritance, and modular design allow developers to work on different parts of a project independently.
- Data Security: Encapsulation allows Python developers to protect sensitive data by restricting access to certain attributes and methods.
- Real-World Problem Solving: Since OOP is modeled around real-world entities and behaviors, it is ideal for simulating real-world problems in code. Python’s OOP structure is widely used in scientific simulations, games, and complex applications.
Real-World Applications of OOP in Python
Python’s OOP capabilities are applied across various industries and domains, showcasing its versatility in building complex systems. Some key real-world applications include:
1. Web Development
Frameworks like Django and Flask leverage OOP to structure web applications. In Django, for instance, models (representing database tables) are defined as classes, making it easier to interact with databases in an OOP manner.
2. Game Development
Games are built around OOP because they can model characters, environments, and interactions using objects. Libraries like Pygame utilize OOP principles to create and manage different game elements like players, enemies, and weapons.
3. Data Science and Machine Learning
Libraries such as TensorFlow, scikit-learn, and Pandas follow OOP principles. Classes such as DataFrame in Pandas or models in scikit-learn allow for modular and reusable code in data manipulation and model training.
4. Software Development
Large-scale applications, such as enterprise-level software or content management systems (CMS), rely on OOP for maintainability and scalability. Python’s OOP features make it an excellent choice for developing these systems.
5. Automation and Scripting
Python’s OOP paradigm is useful for writing reusable automation scripts. Developers can model different automation processes as objects and reuse these in multiple scripts, enhancing productivity.
Best Practices for Object Oriented Programming in Python
To make the most of Python’s OOP features, developers should adhere to the following best practices:
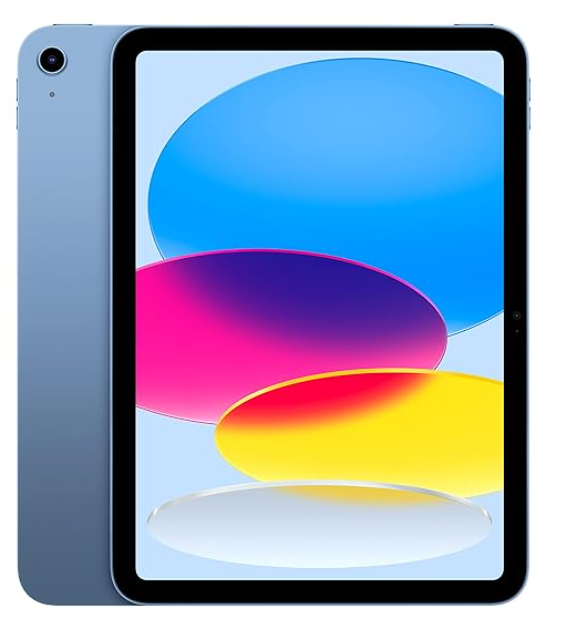
Apple iPad (10th Generation)
Equipped with A14 Bionic chip, 27.69 cm (10.9″) Liquid Retina Display, 64GB, Wi-Fi 6, 12MP front/12MP Back Camera, Touch ID, All-Day Battery Life – Blue
Priced at -14% ₹29,999
1. Follow the Principle of Encapsulation
Always encapsulate data to protect the integrity of the object. Use private or protected attributes when needed, and provide getter/setter methods to access or modify them.
2. Keep Classes Simple
Each class should have a single responsibility. Avoid bloated classes that handle too many tasks, as this leads to code that is hard to maintain and debug.
3. Use Inheritance Wisely
While inheritance is powerful, overusing it can lead to complex class hierarchies that are difficult to manage. Always ensure that subclasses are true specializations of their base classes.
4. Leverage Composition Over Inheritance
In some cases, it’s better to use composition (i.e., including objects of other classes within a class) rather than inheritance. This provides more flexibility in terms of object behavior.
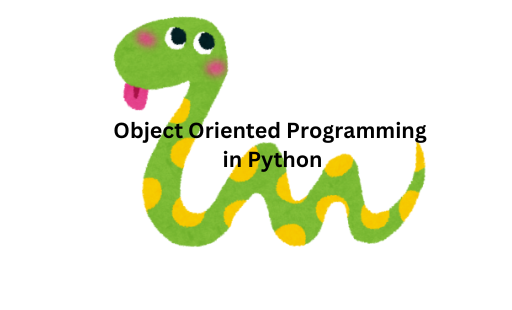
5. Document Your Classes and Methods
Write clear and concise docstrings for each class and method. This improves code readability and makes it easier for other developers to understand your code.
6. Practice Polymorphism
Polymorphism allows you to treat objects of different classes in the same way, which enhances code flexibility. Use interfaces and abstract classes to achieve polymorphism where necessary.
Creative and Artful Ways to Write Object-Oriented Programming (OOP) in Python plus – Best Tips and Tricks
While this could have been an independent article all in itself, I thought I should stick this in here. Simple reason, interest. Object-Oriented Programming (OOP) in Python is an elegant paradigm for structuring your code, allowing you to create flexible and reusable software architectures. However, beyond just understanding OOP principles like inheritance, encapsulation, and polymorphism, it’s essential to apply them creatively to make your code both beautiful and functional. In this article, we will explore tips and tricks to help you write more artistic, modular, and efficient OOP-based programs in Python.
1. Think in Terms of Real-World Objects
One of the most creative ways to approach OOP in Python is to design your classes and objects based on real-world entities. This encourages you to think of the problem as if you were modeling real-life scenarios.
Example:
Imagine you’re designing a system for a library. Think of everything as an object: books, readers, librarians, and transactions. Each object will have its own attributes and methods.
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.is_available = True
def borrow(self):
if self.is_available:
self.is_available = False
else:
print(f"{self.title} is currently unavailable.")
def return_book(self):
self.is_available = True
class Reader:
def __init__(self, name):
self.name = name
self.borrowed_books = []
def borrow_book(self, book):
if book.is_available:
book.borrow()
self.borrowed_books.append(book)
else:
print(f"{book.title} is already borrowed.")
Here, Book and Reader objects map directly to real-world concepts. This approach makes the code not only easy to understand but also intuitive to extend.
2. Use Design Patterns Creatively
Design patterns are templates or blueprints for solving common design problems. When used properly, they add structure and flexibility to your code. Some of the most popular design patterns for Python’s OOP systems include:
a. Factory Pattern
The Factory Pattern can be useful when you need to create multiple instances of similar classes but don’t want the client code to worry about the details of class instantiation.
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == 'dog':
return Dog()
elif animal_type == 'cat':
return Cat()
else:
return None
# Factory usage
factory = AnimalFactory()
dog = factory.create_animal('dog')
This pattern abstracts the creation process, making your code flexible enough to handle new classes without major refactoring.
b. Singleton Pattern
In some cases, you may want to ensure that only one instance of a class is created during the program’s execution. The Singleton Pattern helps you achieve this.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
# Singleton usage
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # Output: True
The Singleton Pattern ensures a class only has one instance, useful for things like database connections or logger classes.
3. Leverage Magic Methods for Operator Overloading
Python’s magic methods (also called dunder methods) can add a layer of creativity to your classes by allowing you to define how objects should behave with built-in operations like addition, subtraction, or comparisons.
Example:
Let’s make a class that allows you to “add” two books together in a library system, combining their authors or information.
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
def __add__(self, other):
return f"Collaboration between {self.author} and {other.author}."
book1 = Book("Python 101", "John Doe")
book2 = Book("Advanced Python", "Jane Smith")
print(book1 + book2) # Output: Collaboration between John Doe and Jane Smith.
By overriding the __add__ method, you can create custom behavior for when objects are “added” together. Magic methods like __repr__, __str__, and __len__ can also be customized for creative output.
4. Use Composition Over Inheritance When Appropriate
While inheritance is a central concept of OOP, composition often leads to more flexible and modular code. Composition involves building classes by combining different components (other classes) instead of using inheritance hierarchies.
Example:
Instead of making Car inherit from multiple vehicle-related classes, you can use composition to include an engine and a fuel system as components.
class Engine:
def start(self):
print("Engine started.")
class FuelSystem:
def refuel(self):
print("Refueling the car.")
class Car:
def __init__(self):
self.engine = Engine()
self.fuel_system = FuelSystem()
def drive(self):
self.engine.start()
print("Car is now driving.")
self.fuel_system.refuel()
# Composition in action
my_car = Car()
my_car.drive()
Here, Car contains instances of Engine and FuelSystem, using composition to include functionality without deep inheritance trees.
5. Polymorphism for Maximum Flexibility
Polymorphism allows you to write more general and flexible code by allowing objects of different types to be used interchangeably. To apply polymorphism creatively, think of different classes that share a common interface or method signature but have unique implementations.
Example:
In a gaming application, you may have different types of characters like warriors, mages, and archers, but they all “attack” in different ways.
class Character:
def attack(self):
raise NotImplementedError("Subclass must implement this method")
class Warrior(Character):
def attack(self):
return "Warrior strikes with a sword!"
class Mage(Character):
def attack(self):
return "Mage casts a fireball!"
class Archer(Character):
def attack(self):
return "Archer shoots an arrow!"
# Polymorphism in action
characters = [Warrior(), Mage(), Archer()]
for character in characters:
print(character.attack())
Here, the attack() method is polymorphic, allowing each character class to implement it differently.
6. Abstract Classes to Enforce Structure
Abstract classes are useful when you want to enforce a certain structure in your code without specifying how it should be implemented. This gives freedom to subclass while ensuring a shared interface.
Example:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
return "Bark!"
class Cat(Animal):
def sound(self):
return "Meow!"
# Using an abstract class
dog = Dog()
cat = Cat()
print(dog.sound()) # Output: Bark!
print(cat.sound()) # Output: Meow!
Abstract classes make sure that all child classes implement the necessary methods, promoting consistency across the codebase.
7. Dynamic Class Creation with type()
Python’s type() function can be used to dynamically create new classes at runtime. This technique, though advanced, can open up new ways to generate classes based on dynamic input or conditions.
Example:
def create_class(class_name, base_classes, class_dict):
return type(class_name, base_classes, class_dict)
# Creating a dynamic class
Dog = create_class("Dog", (object,), {"sound": lambda self: "Bark!"})
my_dog = Dog()
print(my_dog.sound()) # Output: Bark!
This dynamic class creation can be useful when you need to generate classes based on input data or during runtime, giving your program more adaptability.
8. Make Use of Property Decorators for Elegant Getters and Setters
In Python, you can use the @property decorator to turn class methods into attributes, allowing for more Pythonic and readable code when dealing with getters and setters.
Example:
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def radius(self):
return self._radius
@radius.setter
def radius(self, value):
if value < 0:
raise ValueError("Radius cannot be negative")
self._radius = value
# Using property decorators
circle = Circle(10)
print(circle.radius) # Output: 10
circle.radius = 15
print(circle.radius) # Output: 15
The @property decorator provides a clean and efficient way to manage attributes while maintaining control over how they are accessed and modified.
9. Leverage Python’s super() for Clean Code in Inheritance
The super() function in Python simplifies calls to methods from a parent class in subclasses. Using super() helps avoid explicitly naming the parent class, making your code more maintainable, especially in cases of multiple inheritance.
Example:
class Animal:
def __init__(self, name):
self.name = name
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
# Clean inheritance with super()
dog = Dog("Rex", "Labrador")
print(dog.name) # Output: Rex
print(dog.breed) # Output: Labrador
By calling super(), we ensure that the parent class (Animal) is initialized properly, without having to refer to it directly. This makes the code more flexible and less error-prone.
Writing object-oriented programs in Python is not just about applying principles like inheritance and polymorphism; it’s also about adopting creative strategies to make your code elegant, reusable, and efficient. By thinking in terms of real-world objects, leveraging design patterns, using magic methods, and practicing composition, you can bring an artful touch to your OOP designs. Following these tips and tricks will help you write Python OOP code that is not only functional but also a joy to work with.
Conclusion
Object-Oriented Programming is a critical paradigm for building scalable, modular, and maintainable systems in Python. By understanding the core concepts—such as classes, encapsulation, inheritance, polymorphism, and abstraction—you can harness the full power of OOP to write efficient and effective Python code.
Python’s simplicity and flexibility make it an ideal language for implementing OOP principles. Whether you’re developing a web application, a game, or a data science project, mastering OOP in Python opens up endless possibilities for software development.
Embracing OOP not only makes your code more organized but also prepares you for tackling complex real-world problems in a structured and efficient manner.
New Python Users : Start Here
I have been browsing online more than three hours today yet I never found any interesting article like yours It is pretty worth enough for me In my view if all website owners and bloggers made good content as you did the internet will be a lot more useful than ever before
Your article helped me a lot, is there any more related content? Thanks!