Artificial Intelligence (AI) has become a cornerstone of modern software development, enabling applications to deliver smarter, faster, and more personalized experiences. Python, with its rich ecosystem of AI/ML libraries and frameworks, is the go-to language for integrating AI into applications. Below are actionable ideas to extend your application using Python AI, along with practical implementation strategies.
In today’s rapidly evolving digital landscape, integrating Artificial Intelligence (AI) into applications is no longer a luxury but a necessity to stay competitive. Python, with its simplicity and robust ecosystem of AI libraries, has emerged as the language of choice for developers aiming to infuse intelligence into their software. From automating mundane tasks to delivering hyper-personalized user experiences, AI empowers applications to think, learn, and adapt. Whether you’re building a customer-facing app or an enterprise tool, leveraging Python AI can unlock unprecedented efficiency, scalability, and innovation, transforming how users interact with technology.
The versatility of Python AI spans domains like natural language processing (NLP), computer vision, predictive analytics, and more. Imagine a chatbot resolving customer queries in real time, a recommendation system curating content tailored to individual preferences, or an automated workflow that processes data without human intervention. These capabilities are not just futuristic concepts—they are achievable today with Python’s accessible frameworks and pre-trained models. This article explores actionable strategies to extend your application’s functionality using Python AI, providing code examples, use cases, and deployment tips to help you embark on your AI journey.
Contents
1. Enhance User Interaction with NLP.
2. Add Computer Vision Capabilities.
3. Predictive Analytics for Decision-Making.
4. Build Recommendation Systems.
6. Boost Security with AI-Driven Monitoring.
Step 2: Create the Chatbot Logic.
1. Enhance User Interaction with NLP
Idea: Integrate natural language processing (NLP) to build chatbots, sentiment analysis tools, or automated content summarizers.
- Implementation:
- Use libraries like spaCy or NLTK for text preprocessing.
- Leverage Hugging Face Transformers or GPT-4 via APIs for advanced tasks like conversational AI.
- Deploy a Flask/FastAPI backend to serve your model and connect it to your app’s frontend.
Example:
from transformers import pipeline
chatbot = pipeline(“text-generation”, model=”gpt-3.5-turbo”)
response = chatbot(“How do I reset my password?”)
Use Case: A customer support chatbot that resolves queries 24/7, reducing response times.
2. Add Computer Vision Capabilities
Idea: Enable image or video analysis for applications like medical imaging, security, or augmented reality.
- Implementation:
- Use OpenCV for basic image processing.
- Train custom object detection models with TensorFlow or PyTorch.
- Optimize models for edge devices with TensorFlow Lite.
Example:
import cv2
face_cascade = cv2.CascadeClassifier("haarcascade_frontalface.xml")
faces = face_cascade.detectMultiScale(image, scaleFactor=1.1, minNeighbors=5)
Use Case: A retail app that lets users upload photos to find similar products.
3. Predictive Analytics for Decision-Making
Idea: Forecast trends, detect anomalies, or personalize user experiences using ML.
- Implementation:
- Use scikit-learn for regression/classification.
- Build time-series models with Prophet or PyCaret.
- Visualize insights with Matplotlib or Plotly.
Example:
from sklearn.ensemble import RandomForestRegressor
model = RandomForestRegressor()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
Use Case: An e-commerce app predicting inventory demand based on historical sales.
4. Build Recommendation Systems
Idea: Deliver personalized content, products, or media recommendations.
- Implementation:
- Use collaborative filtering with Surprise or LightFM.
- Implement deep learning-based systems with TensorFlow Recommenders.
- A/B test recommendations to optimize engagement.
Example:
from lightfm import LightFM
model = LightFM(loss=”warp”)
model.fit(user_item_interactions, epochs=30)
Use Case: A streaming platform suggesting movies based on viewing history.
5. Automate Workflows with AI
Idea: Streamline repetitive tasks like document processing, data entry, or email sorting.
- Implementation:
- Extract text from documents using PyTesseract (OCR).
- Classify emails with scikit-learn or automate replies with NLP.
- Use LangChain to build AI agents for multi-step workflows.
Example:
import pytesseract
text = pytesseract.image_to_string(“invoice.jpg”)
Use Case: Automatically categorizing and routing support tickets.
6. Boost Security with AI-Driven Monitoring
Idea: Detect fraud, authenticate users via biometrics, or monitor network traffic.
- Implementation:
- Train anomaly detection models with Isolation Forest or Autoencoders.
- Implement face recognition using DeepFace or FaceNet.
Use Case: A banking app flagging unusual transactions in real time.
7. Real-Time Data Processing
Idea: Analyze streaming data (e.g., sensors, social media) for instant insights.
- Implementation:
- Use Apache Kafka with PySpark for data pipelines.
- Deploy lightweight models with ONNX Runtime for low-latency inference.
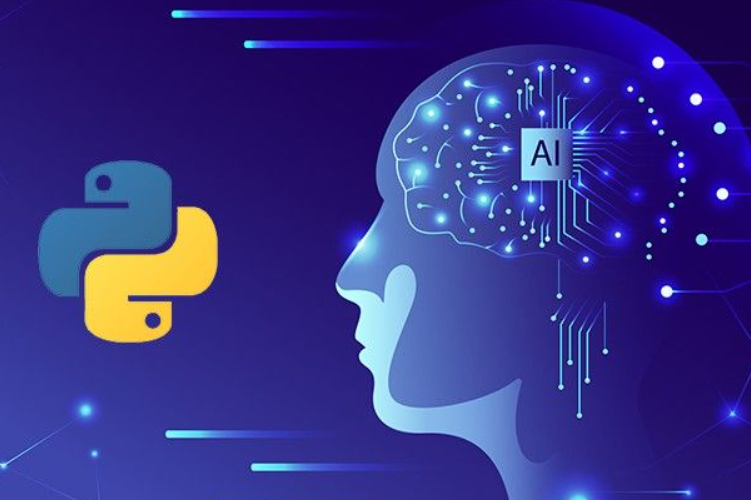
Example:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName(“RealTimeAnalysis”).getOrCreate()
stream = spark.readStream.format(“kafka”).option(“kafka.bootstrap.servers”, “localhost:9092”).load()
Use Case: A fitness app providing live feedback during workouts.
Realworld Example Use Case
Here’s a practical example of creating a simple AI chatbot using Python, Hugging Face’s Transformers library (for a pre-trained model), and Flask for integration into a web application:
Step 1: Install Dependencies
pip install flask transformers torch
Step 2: Create the Chatbot Logic
# chatbot.py
from transformers import AutoModelForCausalLM, AutoTokenizer
import torch
# Load pre-trained model and tokenizer (DialoGPT-medium)
tokenizer = AutoTokenizer.from_pretrained("microsoft/DialoGPT-medium")
model = AutoModelForCausalLM.from_pretrained("microsoft/DialoGPT-medium")
def get_chatbot_response(user_input, chat_history_ids=None):
# Tokenize the user input
new_user_input_ids = tokenizer.encode(
user_input + tokenizer.eos_token,
return_tensors="pt"
)
# Append to the chat history (if exists)
if chat_history_ids is not None:
bot_input_ids = torch.cat([chat_history_ids, new_user_input_ids], dim=-1)
else:
bot_input_ids = new_user_input_ids
# Generate response
chat_history_ids = model.generate(
bot_input_ids,
max_length=1000,
pad_token_id=tokenizer.eos_token_id,
temperature=0.7,
repetition_penalty=1.3
)
# Decode and return the response
response = tokenizer.decode(
chat_history_ids[:, bot_input_ids.shape[-1]:][0],
skip_special_tokens=True
)
return response, chat_history_ids
Step 3: Create the Flask App
# app.py
from flask import Flask, request, jsonify
from chatbot import get_chatbot_response
app = Flask(__name__)
@app.route('/')
def home():
return "Chatbot API is running!"
@app.route('/chat', methods=['POST'])
def chat():
data = request.json
user_message = data.get('message')
# Get chatbot response
response, _ = get_chatbot_response(user_message)
return jsonify({
"user_message": user_message,
"bot_response": response
})
if __name__ == "__main__":
app.run(host='0.0.0.0', port=5000, debug=True)
Step 4: Test the Chatbot
- Start the Flask app:
python app.py
- Send a POST request (using curl, Postman, or a simple HTML form):
curl -X POST http://localhost:5000/chat \
-H “Content-Type: application/json” \
-d ‘{“message”: “Hi, how are you?”}’
Sample Response:
{
“user_message”: “Hi, how are you?”,
“bot_response”: “I’m doing well, thanks! How can I help you today?”
}
Integration Options
- Web Interface: Add an HTML/JavaScript frontend:
Run
<!– templates/index.html –>
<!DOCTYPE html>
<html>
<body>
<div id="chatbox"></div>
<input type="text" id="userInput" placeholder="Type your message...">
<button onclick="sendMessage()">Send</button>
<script>
async function sendMessage() {
const userInput = document.getElementById('userInput').value;
const response = await fetch('/chat', {
method: 'POST',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify({message: userInput})
});
const data = await response.json();
document.getElementById('chatbox').innerHTML +=
`<p>You: ${data.user_message}</p>
<p>Bot: ${data.bot_response}</p>`;
}
</script>
</body>
</html>
- Discord/Slack Integration: Use webhooks to connect the Flask API to messaging platforms.
- Mobile Apps: Use the same Flask API as a backend for Android/iOS apps.
Key Enhancements
- Conversation History: Store chat history using sessions or a database.
- Better Models: Use GPT-3.5/4 via OpenAI API for more sophisticated responses:
import openai
openai.api_key = "YOUR_API_KEY"
def get_gpt_response(prompt):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": prompt}]
)
return response.choices[0].message.content
- Error Handling: Add try-catch blocks for robustness.
- Rate Limiting: Use Flask-Limiter to prevent abuse.
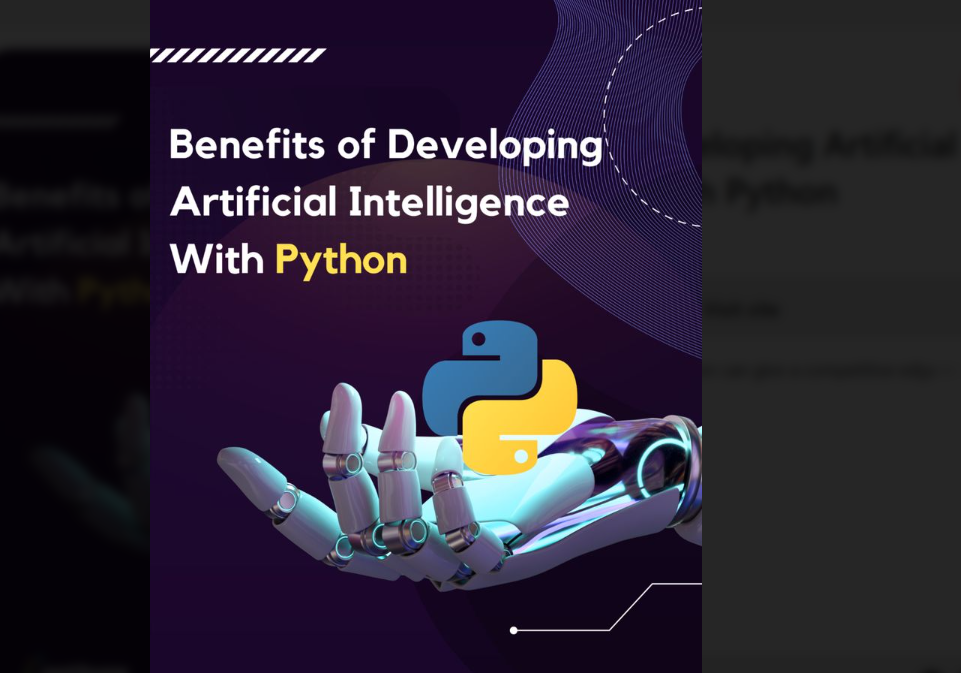
This example demonstrates how to create a basic AI chatbot that can be integrated into any Python application. By combining Hugging Face’s pre-trained models with Flask, you can quickly build and deploy conversational AI features. For production use, consider:
- Adding authentication
- Using async processing (e.g., Celery)
- Containerizing with Docker
- Deploying to cloud platforms like AWS or Heroku
Getting Started: Tips for Success
- Start Small: Begin with a proof of concept (e.g., a single AI feature).
- Leverage Pre-Trained Models: Use platforms like Hugging Face Hub to save time.
- Focus on Scalability: Containerize models with Docker and deploy on cloud services (AWS SageMaker, Google AI Platform).
- Monitor and Iterate: Track performance with tools like MLflow and retrain models periodically.
Conclusion
Integrating AI into your application with Python opens a world of possibilities, enabling smarter decision-making, enhanced user engagement, and streamlined operations. By harnessing libraries like TensorFlow, PyTorch, and Hugging Face Transformers, developers can implement cutting-edge AI features without reinventing the wheel. Whether you’re enhancing an existing product or building from scratch, the examples and frameworks discussed—from chatbots to predictive models—demonstrate that AI adoption is both practical and impactful, even for teams with limited resources.
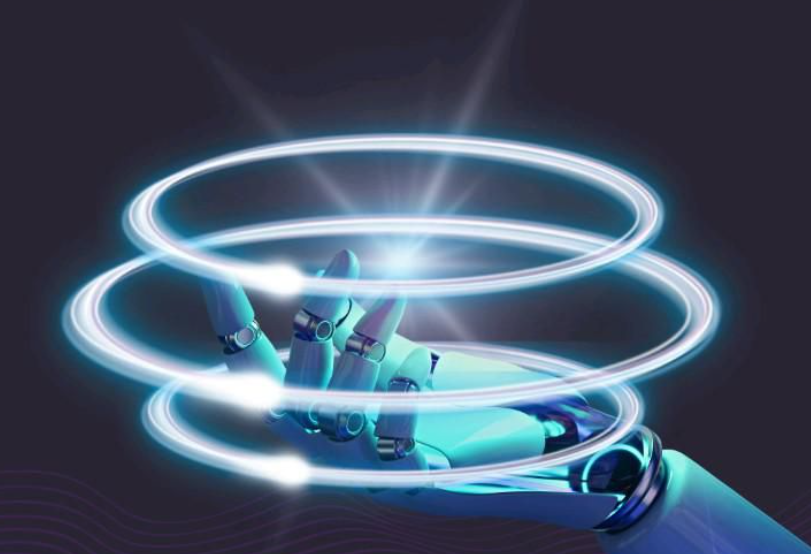
As AI continues to advance, staying ahead requires continuous experimentation and adaptation. Start small by automating a single workflow or adding a conversational interface, then scale iteratively. Tools like Flask and FastAPI simplify integration, while cloud platforms ensure scalability. By embracing Python AI, you’re not just extending your application’s capabilities—you’re future-proofing it. The time to act is now: explore the code snippets, experiment with APIs, and transform your application into an intelligent, dynamic solution that stands out in a crowded market.
Python’s simplicity and powerful AI libraries make it effortless to extend applications with intelligent features. Whether enhancing user interaction, automating workflows, or unlocking data-driven insights, AI can transform your app into a dynamic, future-ready tool. Start experimenting today—pick one idea, integrate it, and iterate!
Python AI