The Power of Functional Programming in Modern Software Development
In the ever-evolving landscape of programming languages, Haskell stands out as a beacon of functional programming, offering a unique paradigm that has influenced many modern languages and frameworks. Named after the logician Haskell Curry, this language, developed in the late 1980s, is known for its strong static typing, lazy evaluation, and emphasis on immutability. These characteristics make Haskell not only a powerful tool for academic purposes but also a robust choice for real-world software development.
Haskell’s design focuses on purity, where functions are treated as first-class citizens and side effects are minimized. This approach leads to code that is not only concise and elegant but also easier to reason about and test. As we delve deeper into the intricacies of Haskell, we will explore its syntax, core concepts, and the advantages it brings to the table, especially in comparison to more mainstream languages like Java, Python, and C.
In today’s competitive tech landscape, choosing the right programming language can significantly impact the efficiency and success of software projects. While Java boasts widespread use in enterprise environments, Python is celebrated for its simplicity and versatility, and C is revered for its performance and control. Haskell, although not as universally adopted, offers distinct benefits that make it a valuable addition to any developer’s toolkit.
This article aims to provide a comprehensive overview of Haskell, highlighting its unique features and how it compares to Java, Python, and C. Through a detailed comparative analysis, we will uncover the strengths and weaknesses of each language, enabling you to make an informed decision about the best tool for your next project.
Understanding Haskell
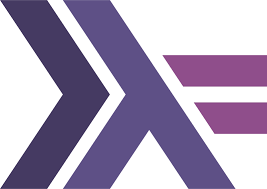
Core Concepts of Haskell
- Functional Programming Paradigm: Haskell is a purely functional programming language, meaning functions are the primary means of computation, and they are treated as first-class citizens. This paradigm emphasizes immutability and avoids side effects, resulting in more predictable and maintainable code.
- Strong Static Typing: Haskell uses a strong static type system, which catches many errors at compile time. The type system is based on Hindley-Milner type inference, allowing for concise code without sacrificing type safety. This reduces runtime errors and improves code reliability.
- Lazy Evaluation: One of Haskell’s defining features is its lazy evaluation strategy. Expressions are not evaluated until their values are needed, which can lead to performance improvements and the ability to define potentially infinite data structures.
- Immutability: In Haskell, data is immutable by default. This immutability simplifies concurrent programming because it eliminates issues related to shared mutable state, such as race conditions and deadlocks.
- Higher-Order Functions: Haskell allows functions to take other functions as arguments and return them as results. This leads to highly modular and reusable code, as common patterns can be abstracted into higher-order functions.
Benefits of Haskell
- Conciseness and Readability: Haskell’s syntax is clean and expressive, enabling developers to write concise and readable code. This reduces the cognitive load and helps maintain large codebases more efficiently.
- Robustness: The strong type system and immutability features of Haskell lead to fewer bugs and more reliable software. Type inference and pattern matching contribute to early error detection during the development process.
- Concurrency and Parallelism: Haskell’s design makes it well-suited for concurrent and parallel programming. The language provides robust abstractions for handling concurrent tasks, which is increasingly important in modern multi-core processors.
- Academic and Industry Adoption: While Haskell is often associated with academic research, it is also used in industry for complex and high-assurance software systems. Companies in finance, telecommunications, and other sectors leverage Haskell’s strengths to build reliable and performant applications.
Comparative Analysis of Haskell, Java, Python, and C
To provide a clear understanding of where Haskell stands in comparison to other popular programming languages, we will examine several key factors: syntax, performance, ease of learning, use cases, and community support.
Feature | Haskell | Java | Python | C |
---|---|---|---|---|
Programming Paradigm | Purely functional | Object-oriented | Multi-paradigm (primarily object-oriented) | Procedural, with support for structured programming |
Typing | Strong static typing | Strong static typing | Dynamic typing | Weak static typing |
Evaluation Strategy | Lazy evaluation | Eager evaluation | Eager evaluation | Eager evaluation |
Syntax | Concise and expressive | Verbose and structured | Simple and readable | Low-level, close to hardware |
Performance | Generally slower due to lazy evaluation, optimized for specific tasks | High performance, especially in large-scale applications | Moderate performance, slower than compiled languages | High performance, suitable for system programming |
Ease of Learning | Steep learning curve due to unique concepts | Moderate learning curve, widely taught | Easy to learn, beginner-friendly | Steep learning curve, especially for complex tasks |
Use Cases | Academic research, financial systems, complex algorithms | Enterprise applications, Android development, web services | Web development, data analysis, scripting, AI/ML | System programming, embedded systems, game development |
Community Support | Active academic and niche industry community | Large, active community with extensive resources | Large, active community with extensive resources | Large, active community with extensive resources |
Detailed Comparison
Syntax and Readability
Haskell’s syntax is designed to be concise and expressive. The use of function application, pattern matching, and list comprehensions allows developers to write clear and elegant code. For example, a simple function to calculate the factorial of a number can be written in just a few lines:
factorial :: Integer -> Integer
factorial 0 = 1
factorial n = n * factorial (n - 1)
In contrast, Java’s syntax is more verbose, requiring boilerplate code for class definitions and method declarations:
public class Factorial {
public static long factorial(int n) {
if (n == 0) return 1;
else return n * factorial(n - 1);
}
}
Python strikes a balance between simplicity and readability, making it a popular choice for beginners:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
C, being a low-level language, offers a syntax that is close to the hardware, providing fine-grained control but at the cost of verbosity and complexity:
#include <stdio.h>
long factorial(int n) {
if (n == 0) return 1;
else return n * factorial(n - 1);
}
int main() {
int num = 5;
printf("Factorial of %d is %ld\n", num, factorial(num));
return 0;
}
Performance
Performance is a crucial factor when choosing a programming language. Haskell’s lazy evaluation can lead to inefficiencies in certain scenarios, but it also allows for optimizations that are not possible in eagerly evaluated languages. For instance, Haskell can handle infinite lists and perform computations only when necessary, potentially reducing overhead.
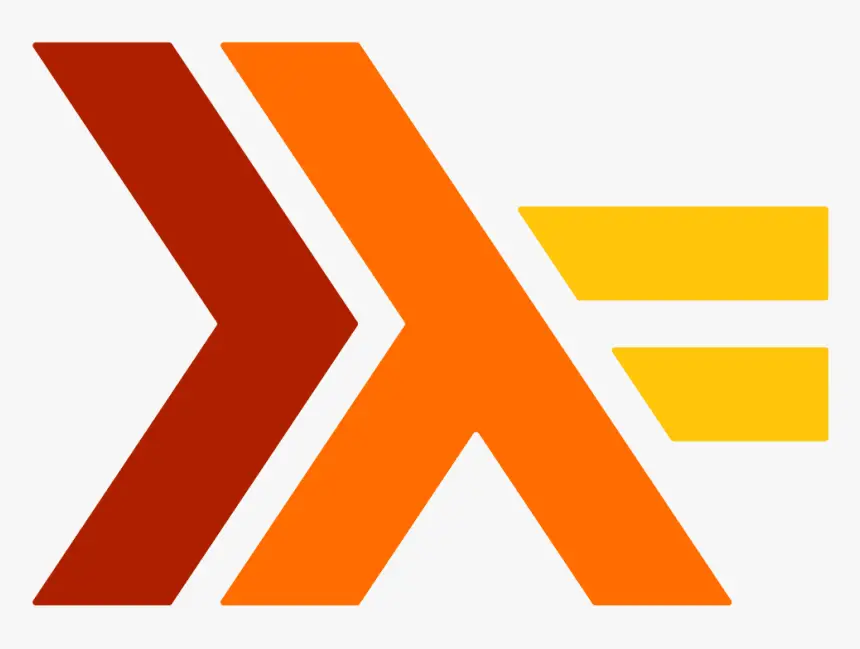
Java, known for its performance and scalability, is widely used in large-scale enterprise applications. Its Just-In-Time (JIT) compiler and mature runtime environment (JVM) enable high-performance execution, especially in long-running applications.
Python, an interpreted language, is generally slower than compiled languages like Java and C. However, its extensive standard library and third-party modules (such as NumPy for numerical computations) can mitigate performance bottlenecks in many use cases.
C, with its low-level access to memory and hardware, is unmatched in performance. It is the language of choice for system programming, real-time applications, and scenarios where maximum performance is critical.
Ease of Learning
Haskell has a steep learning curve, primarily due to its unique functional paradigm and advanced type system. Developers transitioning from imperative or object-oriented languages may find Haskell’s concepts challenging initially. However, once mastered, Haskell’s expressive power and conciseness can lead to increased productivity.
Java, with its widespread use in academia and industry, has a moderate learning curve. Its object-oriented approach is familiar to many developers, and extensive documentation and community support are readily available.
Python is renowned for its ease of learning, making it an ideal first language for beginners. Its simple and readable syntax, combined with a vast ecosystem of libraries and frameworks, allows developers to quickly build and deploy applications.
C, despite its power and performance, has a steep learning curve. Understanding pointers, memory management, and low-level operations requires a solid grasp of computer science fundamentals. However, learning C can provide valuable insights into how computers work at a hardware level.
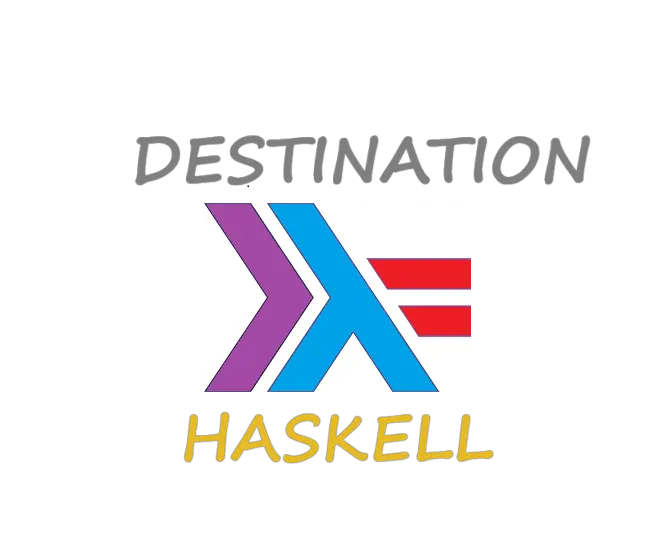
Industries and Companies Using Haskell in Production
Financial Services
Haskell is widely used in the financial services industry, where correctness, reliability, and performance are critical. Financial systems often require complex mathematical computations and the ability to handle large volumes of data efficiently. Haskell’s strong type system and immutability make it a suitable choice for these applications, reducing the risk of errors and improving code maintainability.
Examples of Companies:
- Standard Chartered: This multinational banking and financial services company uses Haskell for its financial software infrastructure. Haskell’s robust type system and purity help in creating reliable and maintainable code, essential for financial applications.
- Jane Street: A quantitative trading firm that relies heavily on functional programming. While they are more famous for their use of OCaml, they also utilize Haskell for various internal tools and systems.
Technology and Software Development
In the technology sector, Haskell is used for building high-assurance software and tools where reliability and correctness are paramount. Haskell’s strong type system and functional paradigm are leveraged to develop robust and efficient software solutions.
Examples of Companies:
- Facebook: Facebook uses Haskell for its anti-spam infrastructure. The language’s expressive power and reliability help in writing robust systems that can efficiently handle spam detection algorithms.
- GitHub: The popular version control platform has used Haskell for several of its internal tools. Haskell’s efficiency in handling complex algorithms and data processing tasks makes it an attractive choice for backend development.
Telecommunications
The telecommunications industry requires software that can handle concurrent processes efficiently and reliably. Haskell’s inherent support for concurrency and parallelism makes it an excellent choice for this sector.
Examples of Companies:
- AT&T: The American telecommunications giant has used Haskell for some of its internal systems, particularly where reliability and performance are critical. Haskell’s ability to manage concurrent processes and its strong type system are significant advantages in this industry.
Data Science and Research
Haskell is also utilized in academic and research settings, particularly in fields that require rigorous mathematical computations and data analysis. Its functional programming paradigm and strong type system make it ideal for developing complex algorithms and processing large datasets.
Examples of Companies:
- Obsidian Systems: This company uses Haskell to build custom software solutions, particularly in data science and research domains. Haskell’s capabilities in handling complex data structures and computations make it an excellent choice for these applications.
- SimSpace: A company specializing in cybersecurity simulation, using Haskell to create robust and efficient simulation environments. The language’s expressiveness and reliability help in developing high-assurance software required for cybersecurity applications.
Healthcare
In the healthcare industry, software reliability and correctness are of utmost importance due to the potential impact on patient safety. Haskell’s type safety and immutability are leveraged to develop systems that need to be highly reliable and maintainable.
Examples of Companies:
- Mercury: A healthcare technology company that uses Haskell to develop software solutions for the medical industry. Haskell’s ability to create reliable and maintainable code helps ensure that healthcare applications function correctly and safely.
Why These Industries Choose Haskell
- Reliability and Correctness: Haskell’s strong type system and purity help in writing code that is less prone to errors. This is particularly important in industries like finance and healthcare, where software errors can have significant consequences.
- Maintainability: Haskell’s emphasis on immutability and functional programming leads to more maintainable codebases. This is beneficial in large-scale software systems that require long-term maintenance and evolution.
- Concurrency and Parallelism: Haskell’s design makes it well-suited for concurrent and parallel programming, essential for telecommunications and real-time data processing applications.
- Expressiveness: Haskell allows developers to write concise and expressive code, which is particularly useful in research and data science, where complex algorithms and data manipulations are common.
- Performance: While Haskell’s lazy evaluation can sometimes be a performance bottleneck, it also allows for optimizations that can significantly improve performance in specific scenarios. This balance of performance and correctness makes it an attractive choice for various industries.
Use Cases
Haskell excels in domains that require robust and maintainable code. Its strong type system and immutability make it suitable for applications where correctness and reliability are paramount. Haskell is often used in academic research, financial systems, and complex algorithms, where its mathematical foundations and expressiveness shine.
Java is the go-to language for enterprise applications, web services, and Android development. Its portability, performance, and extensive ecosystem make it a versatile choice for a wide range of applications. Java’s compatibility with big data frameworks like Hadoop and Spark further enhances its utility in data-intensive environments.
Python’s versatility makes it a popular choice for web development, data analysis, scripting, and artificial intelligence/machine learning (AI/ML). Framework
Suggested for you : Bjarne Stroustrup – LearnXYZ
May I request more information on the subject? All of your articles are extremely useful to me. Thank you!
yes. I’ll try to compile and put more information on all the requested topics. It might take some time but will try to put it.
Thank you for writing this post!
I’m so in love with this. You did a great job!!