Today we will look at the extent reports the reporting tool for our automated test cases. Execution of each test step is logged in this report. It is quite handy to find and figure out the issue with your test script in case of failures.
Extent Reports
All the test case logging and debug messages are done in extent reports. They are basically available here – D:\MAGICBLOCKS\GIT\OBQA\Automation\Jkrux.TestAutomation\Jkrux.TestAutomation.Tests\Execution_Reports
For multiple test case execution a suite report is generated as below. You can click on the Pass/Fail button on the right side pane to expand the test to reveal the steps and find out where it might have failed.
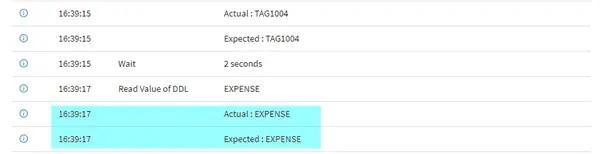
For multiple test case execution a suite report is generated as below. You can click on the Pass/Fail button on the right side pane to expand the test to reveal the steps and find out where it might have failed.
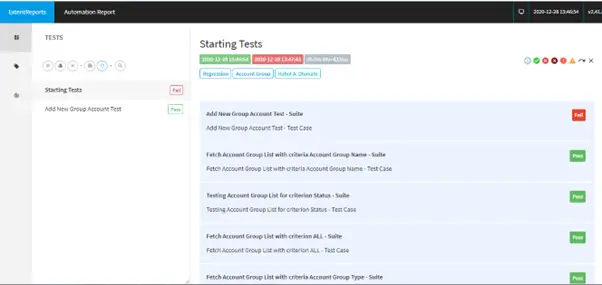
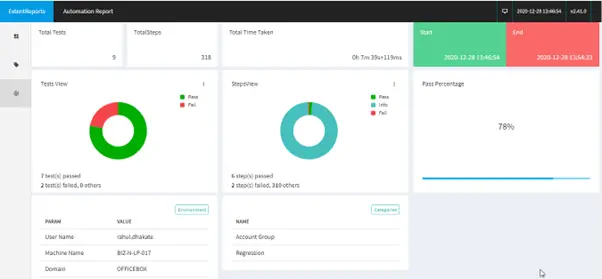
For all the tests a detail graphical report is also generated simultaneously that gives us the details of pass percentage and a visual walk through of the suite execution.
Next we try to figure out how you can create test suites for your scripts so that you can run multiple scripts at one go – Batch Run
Test Suites
Single Login SuiteMulti Login Suite |
The Test Suites reside in Suites directory in solution explorer. Primarily we have two types of suites
For every type of suite you run we have the extent report take care of all the execution for every individual test case.
Single Login
In Single Login Suite, we can login once and execute a bunch of test cases and then logout. The login and logout process is only done once through-out the suite execution process. It is mostly upto the user to make sure that the previous test cases in the stack finish or are terminated properly or else subsequent tests may fail. The implications could be – If an unhandled dialog box (Example: Import dialog box etc) remains open from the execution of a previous test case, all the rest may fail as they are not able to locate their UI elements in this context.
SAMPLE SINGLE LOGIN SUITE
/*
* Test Case Name: Account Suite
* Author: Rahul A. Dhakate
* Created Date: 11-12-2020
* Updated By:
* Updated Date:
* Comments:
*/
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Jkrux.TestAutomation.Framework;
using OpenQA.Selenium;
using OpenQA.Selenium.Support.PageObjects;
using System.Diagnostics;
using Jkrux.TestAutomation.Tests.Pages;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using RelevantCodes.ExtentReports;
using NUnit.Framework;
using Jkrux.TestAutomation.Tests.Tests.Accounts.AccntGroup;
namespace Jkrux.TestAutomation.Tests.Suites
{
[TestClass]
class AccountSuiteSingleLogin : BaseTest
{
ExtentTest testReportParent = ExtReport.StartTest("JKRUX CNF ACCOUNT SUITE");
LoginPage oLoginPage = new LoginPage(); //Login page instantiation
[OneTimeSetUp]
public void StartTest()
{
SetReportEnvironment(testReportParent, Settings.projectPath, Settings.testCaseExecutedBy);
testReportParent.AssignCategory("Regression", "Login Page");
TestReport = ExtReport.StartTest("Starting Single Login Tests");
TestReport.Log(LogStatus.Info, "Start Test", "Logging In");
Dictionary<string, string> loginData = GetDataFromExcelRow(
Settings.dataFilePath, oLoginPage.DataFile, oLoginPage.WorkSheet, 2);
//Login with account user
oLoginPage.DoLogin(loginData, 2)
.WaitUntil(2);
}
[SetUp]
public void Initialize() { }
//Create Constructors for your test cases here
[Test, Order(010), Category("Accounts")]
public void SingleLoginAccntTest()
{
SingleLoginAccntTest oSingleLoginAccntTest = new SingleLoginAccntTest();
oSingleLoginAccntTest.SingleLoginAccnt_Test();
}
[OneTimeTearDown]
public void CloseTestExecution()
{
TestReport.Log(LogStatus.Info, "Test Case: ", "Test Case Execution Finished");
oLoginPage
.WaitUntil(05);
if (TestReport != null)
{
testReportParent.AppendChild(TestReport);
ExtReport.EndTest(TestReport);
ExtReport.Flush();
TestReport = null;
}
}
[TearDown]
public void CleanUp()
{
TestReport.Log(LogStatus.Info, "Test Case: ", "Logging out");
oLoginPage.DoLogout()
.WaitUntil(2);
}
}
}
Multi Login (Or Simple Suite)
In multiple login suite, we write the test cases as independent entities. They are not dependent on a suite for login or logout process. Every test has its own methods for that. Why we are calling this simple is because in this suite we can ignore where the previous test is , if it has passed or failed and if there is any residue left from previous execution. We can start execution of the test suite a fresh again. That means each test logs in and logs out independently from other.
SAMPLE MULTI LOGIN SUITE
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Jkrux.TestAutomation.Framework;
using OpenQA.Selenium;
using OpenQA.Selenium.Support.PageObjects;
using System.Diagnostics;
using Jkrux.TestAutomation.Tests.Pages;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using RelevantCodes.ExtentReports;
using NUnit.Framework;
using Jkrux.TestAutomation.Tests.Tests.Accounts.AccntGroup;
namespace Jkrux.TestAutomation.Tests.Suites
{
[TestClass]
class AccountSuite : BaseTest
{
ExtentTest testReportParent = ExtReport.StartTest("JKRUX CNF ACCOUNT SUITE");
BrowserType testBrowser = Settings.DefaultBrowser;
[OneTimeSetUp]
public void StartTest()
{
SetReportEnvironment(testReportParent, Settings.projectPath, Settings.testCaseExecutedBy);
testReportParent.AssignCategory("Regression", "Accounts");
//TestReport = ExtReport.StartTest("Test(s) Execution Started ", testBrowser.ToString() + " For Testing Account Group Scenarios");
//TestReport.Log(LogStatus.Info, "Start Test", "Logging In");
}
[SetUp]
public void Initialize() { }
//Create Constructors for your test cases here
[Test, Order(010), Category("Accounts")]
public void FetchAccountGroupListWithCriterionGroupTypeTest()
{
FetchAccountGroupListWithCriterionGroupTypeTest oFetchAccountGroupListByGroupType = new FetchAccountGroupListWithCriterionGroupTypeTest();
oFetchAccountGroupListByGroupType.FetchAccountGroupListWithCriterionGroupType_Test();
}
[Test, Order(015), Category("Accounts")]
public void FetchAccountGroupListWithCriterionAccountGroupNameTest()
{
FetchAccountGroupListWithCriterionAccountGroupNameTest oFetchAccountGroupListByGroupName = new FetchAccountGroupListWithCriterionAccountGroupNameTest();
oFetchAccountGroupListByGroupName.FetchAccountGroupListWithCriterionAccountGroupName_Test(); //The test which we want to call
}
[Test, Order(020), Category("Accounts")]
public void FetchAccountGroupListWithCriterionAccountStatusTest()
{
FetchAccountGroupListWithCriterionAccountStatusTest oFetchAccountGroupListByStatus = new FetchAccountGroupListWithCriterionAccountStatusTest();
oFetchAccountGroupListByStatus.FetchAccountGroupListWithCriterionAccountStatus_Test();
}
[Test, Order(025), Category("Accounts")]
public void FetchAccountGroupListWithCriterionAllTest()
{
FetchAccountGroupListWithCriterionAllTest oFetchAccountGroupListWithCriterionAllTest = new FetchAccountGroupListWithCriterionAllTest();
oFetchAccountGroupListWithCriterionAllTest.FetchAccountGroupListWithCriterionAll_Test();
}
[OneTimeTearDown]
public void CloseTestExecution() {
if (TestReport != null)
{
testReportParent.AppendChild(TestReport);
ExtReport.EndTest(TestReport);
ExtReport.Flush();
TestReport = null;
}
}
}
}
In the next, part I will be sharing some tips and tricks, so don’t miss that one out. It will be helpful in some basic implementations for you.