Automatic Speech Recognition (ASR) technology has revolutionized the way humans interact with machines, making it possible to convert spoken language into text effortlessly. With advancements in machine learning and natural language processing (NLP), ASR systems have become an integral part of many applications, including virtual assistants, transcription services, and accessibility tools. Python, a versatile and popular programming language, has emerged as a preferred choice for developing ASR systems due to its rich ecosystem of libraries and frameworks.
This article delves into the fascinating world of ASR, highlighting its significance, implementation methods, and practical applications using Python. From understanding the basics of speech recognition to exploring advanced use cases, we’ll cover everything you need to know to get started. Whether you’re a developer aiming to integrate speech recognition into your application or an enthusiast curious about the technology, this guide has you covered.
Here’s what you can expect from this article:
- A thorough explanation of ASR and its working principles.
- An introduction to Python libraries like SpeechRecognition, PyDub, and DeepSpeech.
- Hands-on examples for building and testing ASR systems.
- Top 10 exclusive facts about ASR.
- Answers to 30 frequently asked questions about ASR.
By the end of this article, you’ll have a strong foundation in ASR and the skills to implement it in Python-based projects. Let’s get started.
Table of Contents
Automatic Speech Recognition (ASR) Using Python.
What is Automatic Speech Recognition (ASR)?.
Example 1: Transcribing an Audio File in a Specific Language.
Example 2: Real-Time Speech Recognition with Language Detection.
Example 3: Using Wav2Vec2 for Multilingual Speech Recognition.
Seamless Integration of ASR Technology in Django, Flask, and Other Web Projects.
3. Cross-Framework Integration Using WebSockets.
Job Opportunities and Salaries for ASR Python Developers.
Job Opportunities for ASR Python Developers.
Salaries for ASR Python Developers.
Career Growth in ASR Python Development
Emerging Trends Driving Demand.
What is Automatic Speech Recognition (ASR)?
Automatic Speech Recognition, often abbreviated as ASR, refers to the process of converting spoken language into written text. ASR systems utilize complex algorithms and models to analyze and transcribe audio inputs. These systems are designed to understand various accents, languages, and speech patterns, making them versatile and user-friendly.
ASR operates through the following key stages:
- Audio Capture: The system records spoken words using a microphone or an audio file.
- Feature Extraction: Audio signals are processed to extract relevant features like pitch, frequency, and amplitude.
- Acoustic Modeling: Statistical models predict the likelihood of certain sounds occurring together.
- Language Modeling: Context is added by analyzing word patterns and grammar.
- Transcription: The processed data is converted into readable text.
Python Libraries for ASR
Python offers several powerful libraries for implementing ASR systems. Here are the most popular ones:
- SpeechRecognition
- A simple and easy-to-use library for speech recognition.
- Supports multiple APIs like Google Speech API, IBM Watson, and Microsoft Bing Voice Recognition.
- Example:
import speech_recognition as sr
recognizer = sr.Recognizer()
with sr.AudioFile('audio_file.wav') as source:
audio_data = recognizer.record(source)
text = recognizer.recognize_google(audio_data)
print(text)
- PyDub
- A library for audio manipulation and pre-processing.
- Helps in converting audio formats and segmenting audio files.
- DeepSpeech
- An open-source ASR engine developed by Mozilla.
- Leverages deep learning for improved accuracy.
- Example:
from deepspeech import Model
model_file_path = 'deepspeech_model.pbmm'
scorer_file_path = 'deepspeech_scorer.scorer'
model = Model(model_file_path)
model.enableExternalScorer(scorer_file_path)
audio = load_audio('audio.wav')
text = model.stt(audio)
print(text)
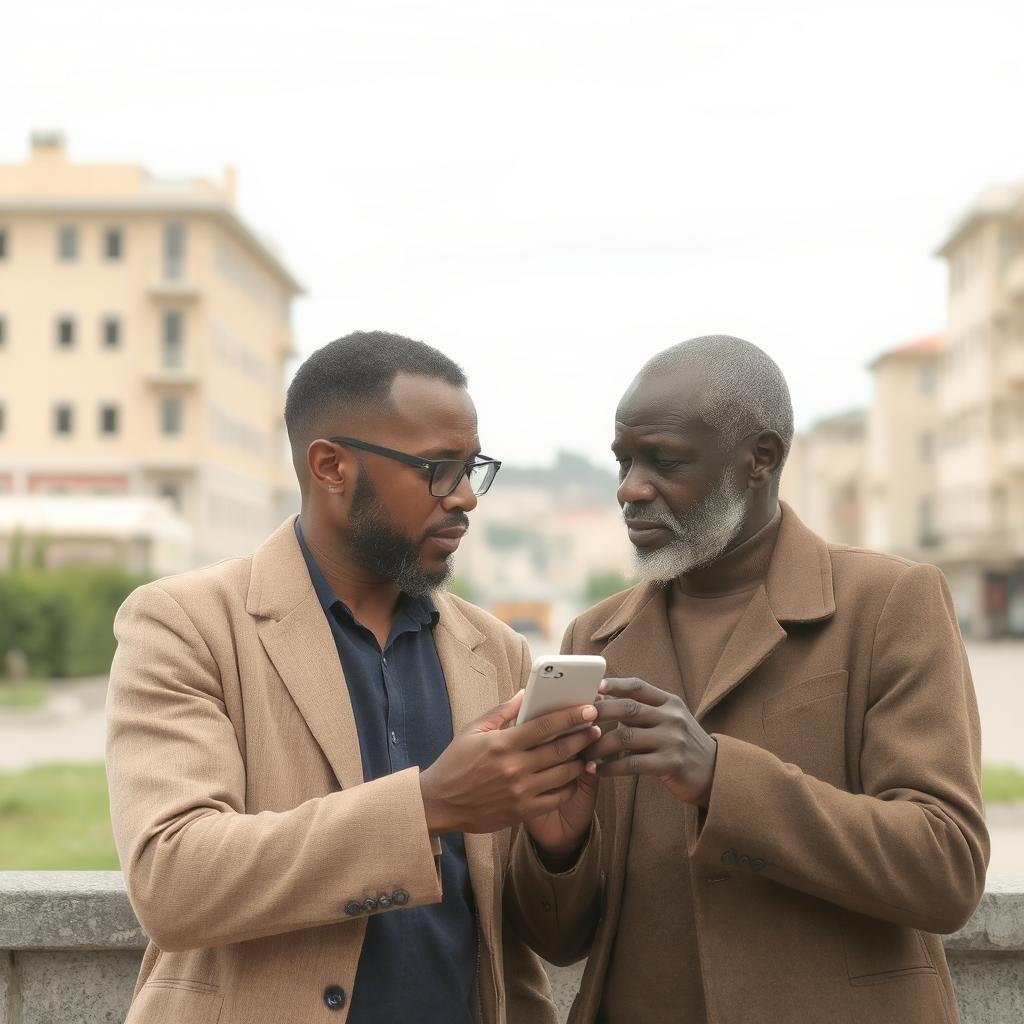
- Librosa
- Ideal for feature extraction and audio analysis.
- Frequently used for creating datasets for ASR systems.
- Wav2Vec2
- A state-of-the-art model developed by Facebook AI.
- Highly effective for pre-trained ASR tasks using transformers.
Implementing ASR with Python
Example 1: Transcribing an Audio File in a Specific Language
import speech_recognition as sr
# Initialize the recognizer
recognizer = sr.Recognizer()
# Load the audio file
audio_file = "example.wav"
with sr.AudioFile(audio_file) as source:
audio = recognizer.record(source)
# Recognize the speech (e.g., converting Spanish speech to text)
try:
text = recognizer.recognize_google(audio, language="es-ES")
print("Transcription:", text)
except sr.UnknownValueError:
print("Audio is unclear.")
except sr.RequestError as e:
print(f"API error: {e}")
Example 2: Real-Time Speech Recognition with Language Detection
import speech_recognition as sr
def live_speech_recognition():
recognizer = sr.Recognizer()
mic = sr.Microphone()
print("Listening...")
with mic as source:
recognizer.adjust_for_ambient_noise(source)
audio = recognizer.listen(source)
try:
# Detect and transcribe speech in Hindi
text = recognizer.recognize_google(audio, language="hi-IN")
print("You said (in Hindi):", text)
except sr.UnknownValueError:
print("Could not understand audio.")
except sr.RequestError as e:
print(f"API error: {e}")
live_speech_recognition()
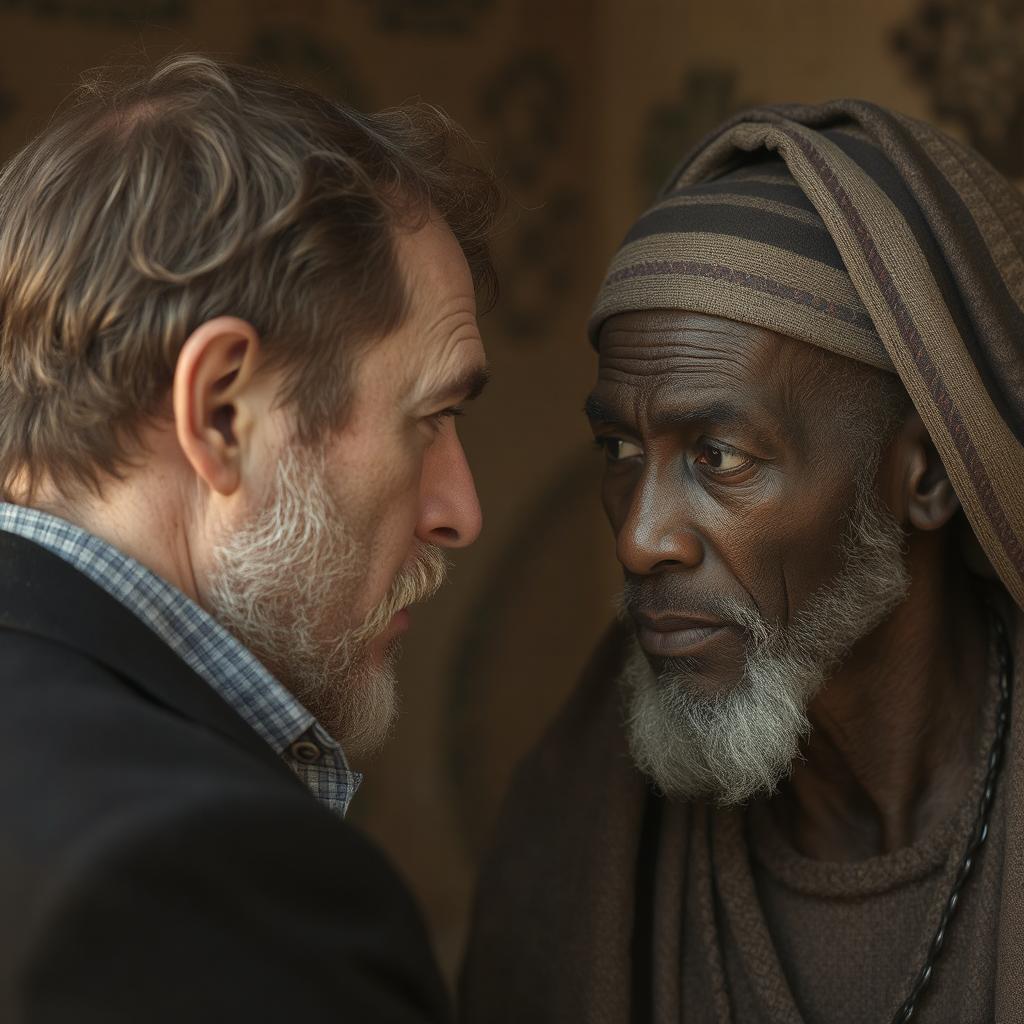
Its important to understand each other. Effective Communication does matter!
Example 3: Using Wav2Vec2 for Multilingual Speech Recognition
from transformers import Wav2Vec2ForCTC, Wav2Vec2Tokenizer
import torch
import librosa
# Load pre-trained model and tokenizer
model_name = "facebook/wav2vec2-large-xlsr-53"
tokenizer = Wav2Vec2Tokenizer.from_pretrained(model_name)
model = Wav2Vec2ForCTC.from_pretrained(model_name)
# Load and preprocess audio
file_path = "multilingual_audio.wav"
waveform, rate = librosa.load(file_path, sr=16000)
input_values = tokenizer(waveform, return_tensors="pt", padding="longest").input_values
# Perform speech recognition
with torch.no_grad():
logits = model(input_values).logits
predicted_ids = torch.argmax(logits, dim=-1)
# Decode the transcription
transcription = tokenizer.batch_decode(predicted_ids)
print("Transcription:", transcription[0])
These examples demonstrate how Python can handle various languages and real-time transcription needs. By specifying the desired language in the APIs or models, ASR systems become versatile and practical for diverse applications.
Applications of ASR
- Virtual Assistants – Alexa, Google Assistant, and Siri leverage ASR to understand user commands.
- Transcription Services – Automated tools convert audio recordings into text.
- Accessibility – Provides support for individuals with disabilities.
- Customer Service – IVR systems utilize ASR for efficient call routing.
- Education – Assists in language learning and lecture transcription.
Seamless Integration of ASR Technology in Django, Flask, and Other Web Projects
Automatic Speech Recognition (ASR) has become a cornerstone of modern web applications, allowing for innovative features like voice search, transcription, and voice command systems. Integrating ASR into web frameworks such as Django and Flask enables developers to create dynamic, user-friendly applications. Here’s a guide on how to achieve seamless ASR integration in these frameworks and other web-based projects.
1. ASR Integration in Django
Django is a robust and scalable framework widely used for web development. Integrating ASR in Django involves the following steps:
Steps for Integration:
- Install Required Libraries: Ensure you have an ASR library like SpeechRecognition or DeepSpeech installed, along with Django.
pip install SpeechRecognition django
- Set Up a Django View for ASR: Create a view that processes audio files uploaded by the user.
import speech_recognition as sr
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
@csrf_exempt
def transcribe_audio(request):
if request.method == 'POST' and 'audio' in request.FILES:
recognizer = sr.Recognizer()
audio_file = request.FILES['audio']
with sr.AudioFile(audio_file) as source:
audio_data = recognizer.record(source)
try:
text = recognizer.recognize_google(audio_data)
return JsonResponse({"transcription": text})
except sr.UnknownValueError:
return JsonResponse({"error": "Unable to recognize speech"})
return JsonResponse({"error": "Invalid request"})
- Create a Frontend Interface: Add a simple form in your Django template to upload audio files.
Use Cases:
- Voice-activated search engines.
- Real-time transcription in web-based meeting platforms.
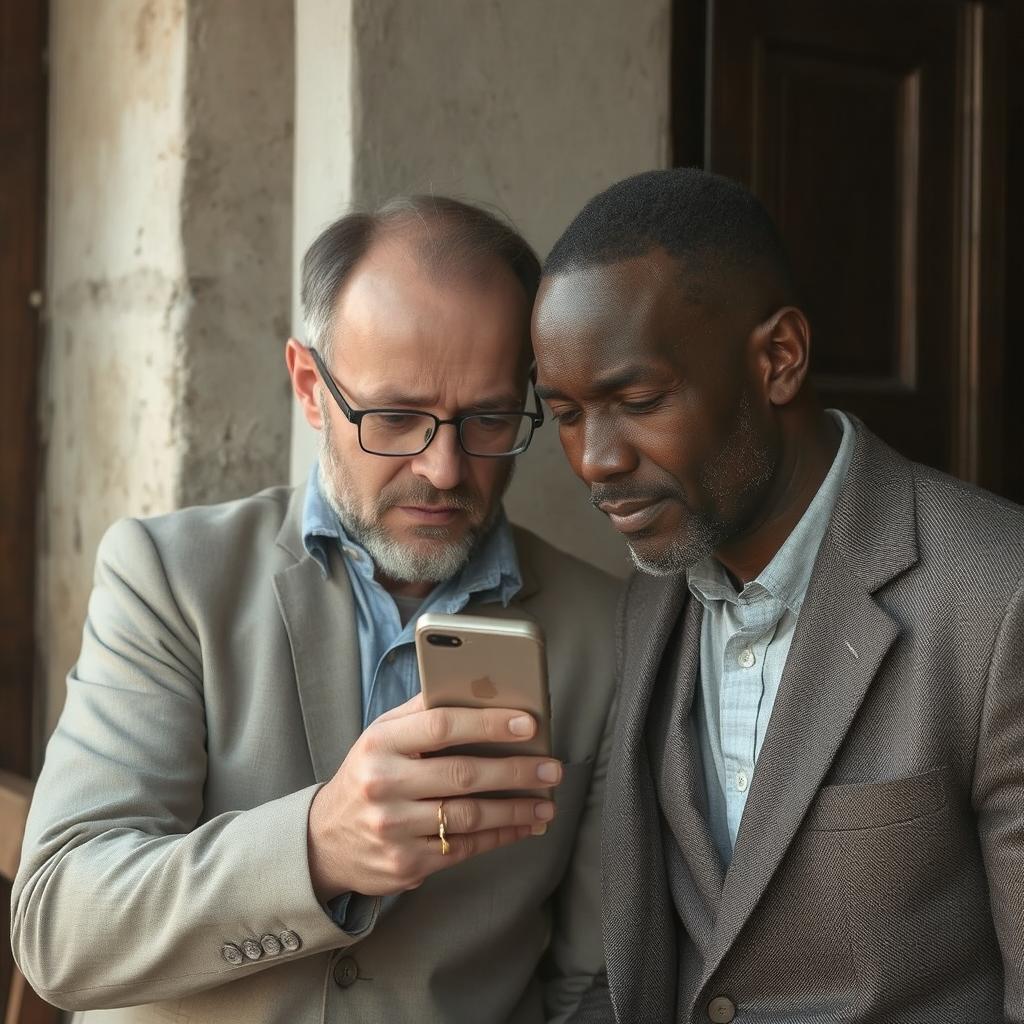
2. ASR Integration in Flask
Flask, a lightweight web framework, offers the flexibility to integrate ASR features with minimal setup.
Steps for Integration:
- Install Flask and ASR Libraries:
pip install flask SpeechRecognition
- Create a Flask Route for ASR:
from flask import Flask, request, jsonify
import speech_recognition as sr
app = Flask(__name__)
@app.route('/transcribe', methods=['POST'])
def transcribe():
if 'audio' not in request.files:
return jsonify({"error": "No audio file provided"})
recognizer = sr.Recognizer()
audio_file = request.files['audio']
with sr.AudioFile(audio_file) as source:
audio_data = recognizer.record(source)
try:
text = recognizer.recognize_google(audio_data)
return jsonify({"transcription": text})
except sr.UnknownValueError:
return jsonify({"error": "Unable to recognize speech"})
if __name__ == '__main__':
app.run(debug=True)
- Test the Endpoint: Use tools like Postman or create a frontend to upload audio files and view transcriptions.
Use Cases:
- Real-time customer support chatbots.
- Interactive voice response (IVR) systems for web apps.
3. Cross-Framework Integration Using WebSockets
For real-time ASR in both Django and Flask, WebSockets can be utilized to stream audio data.
Implementation:
- Use Django Channels or Flask-SocketIO for WebSocket support.
- Stream audio data from the client to the server in chunks and process it using ASR.
Example:
# Example using Flask-SocketIO
from flask import Flask
from flask_socketio import SocketIO, emit
import speech_recognition as sr
app = Flask(__name__)
socketio = SocketIO(app)
@socketio.on('audio_stream')
def handle_audio(data):
recognizer = sr.Recognizer()
audio = sr.AudioData(data, sample_rate=16000, sample_width=2)
try:
text = recognizer.recognize_google(audio)
emit('transcription', {"text": text})
except sr.UnknownValueError:
emit('transcription', {"error": "Unable to recognize speech"})
if __name__ == '__main__':
socketio.run(app)
Use Cases:
- Live transcription for online lectures.
- Real-time voice command execution in web dashboards.
4. ASR in Other Web Projects
If you’re not using Django or Flask, ASR can still be integrated into custom web solutions using REST APIs or JavaScript-based frameworks.
REST API Integration:
- Build an ASR REST API using Python libraries and deploy it with tools like FastAPI.
- Connect the API to a frontend built with React or Angular.
Example with FastAPI
from fastapi import FastAPI, File, UploadFile
import speech_recognition as sr
app = FastAPI()
@app.post("/transcribe/")
async def transcribe(audio: UploadFile = File(...)):
recognizer = sr.Recognizer()
with sr.AudioFile(audio.file) as source:
audio_data = recognizer.record(source)
text = recognizer.recognize_google(audio_data)
return {"transcription": text}
Frontend Integration:
- Use HTML5’s getUserMedia API to capture audio from the user.
- Send audio data to the server for processing.
5. Challenges and Solutions
While integrating ASR into web projects, developers may encounter the following challenges:
- Latency Issues: Streaming audio can introduce delays. Optimize by using lightweight ASR models or edge processing.
- Noise Handling: Incorporate noise-cancellation techniques during audio capture.
- Multi-Language Support: Use models like Wav2Vec2 for better language flexibility.
By leveraging Python’s robust ASR libraries, Django, Flask, and other web frameworks can seamlessly integrate speech recognition capabilities. Whether you’re building interactive voice interfaces or enhancing user accessibility, ASR technology offers a plethora of opportunities to innovate.
Job Opportunities and Salaries for ASR Python Developers
Automatic Speech Recognition (ASR) has rapidly evolved into a highly sought-after field, creating numerous opportunities for Python developers specializing in ASR. The rise of AI-powered tools, virtual assistants, transcription services, and accessibility technologies has driven the demand for skilled professionals who can implement and optimize ASR systems.
Job Opportunities for ASR Python Developers
- Speech Recognition Engineer
- Role: Design, train, and deploy ASR models to transcribe speech accurately across various languages and accents.
- Skills Required: Expertise in Python, machine learning frameworks (TensorFlow, PyTorch), and ASR libraries like SpeechRecognition, DeepSpeech, and Wav2Vec2.
- Industries Hiring: Tech companies, healthcare, customer service, and media.
- AI/ML Engineer
- Role: Build ASR systems as part of larger AI solutions for virtual assistants, voice search, or sentiment analysis.
- Skills Required: Knowledge of natural language processing (NLP), Python, and deep learning models.
- Data Scientist – Speech
- Role: Preprocess audio datasets, train ASR models, and fine-tune them for accuracy.
- Skills Required: Audio signal processing, Python, and statistical modeling.
- Full Stack Developer with ASR Expertise
- Role: Develop end-to-end solutions integrating ASR into web or mobile applications.
- Skills Required: Proficiency in Python for ASR and frameworks like Django, Flask, or FastAPI.
- Research Scientist
- Role: Conduct research to advance ASR technologies, focusing on model optimization and new applications.
- Skills Required: Strong Python programming skills and knowledge of state-of-the-art ASR models.
- ASR Consultant
- Role: Provide expertise to businesses looking to implement ASR solutions tailored to their needs.
- Skills Required: Experience with ASR deployment and API integration.
- Accessibility Specialist
- Role: Use ASR to create accessible solutions for people with disabilities, such as automated captions or speech-to-text services.
- Skills Required: Python, ASR, and an understanding of accessibility standards.
Salaries for ASR Python Developers
Salaries for ASR Python developers vary based on experience, location, and the specific role. Below are general ranges for key positions:
Role | Entry-Level (USD) | Mid-Level (USD) | Senior-Level (USD) |
Speech Recognition Engineer | $70,000 – $90,000 | $100,000 – $130,000 | $150,000+ |
AI/ML Engineer | $80,000 – $100,000 | $120,000 – $140,000 | $160,000+ |
Data Scientist – Speech | $75,000 – $95,000 | $110,000 – $140,000 | $150,000+ |
Full Stack Developer | $60,000 – $85,000 | $90,000 – $120,000 | $140,000+ |
Research Scientist | $90,000 – $120,000 | $130,000 – $160,000 | $180,000+ |
ASR Consultant | $100,000 – $130,000 | $140,000 – $170,000 | $200,000+ |
Accessibility Specialist | $65,000 – $85,000 | $95,000 – $120,000 | $130,000+ |
Factors Influencing Salaries
- Experience Level: Senior developers with deep expertise in ASR and Python command higher salaries.
- Geographic Location: Salaries are typically higher in tech hubs like Silicon Valley, New York, London, or Bangalore.
- Industry: ASR roles in AI-driven industries (e.g., tech giants or innovative startups) often pay more than traditional sectors.
- Certifications: Certifications in machine learning, deep learning, or specific ASR tools can boost salary prospects.
- Demand for Niche Skills: Proficiency in state-of-the-art ASR models like Wav2Vec2 or real-time ASR systems can lead to premium pay.
Career Growth in ASR Python Development
- Junior Developers: Begin with entry-level roles, contributing to ASR model implementation and API integration.
- Mid-Level Developers: Take on responsibilities for training custom models, optimizing accuracy, and deploying scalable systems.
- Senior Engineers: Lead ASR projects, mentor teams, and drive innovation in ASR technologies.
- Entrepreneurial Opportunities: Startups focused on voice technologies and accessibility solutions often need ASR expertise, opening avenues for entrepreneurial ventures.
Emerging Trends Driving Demand
- Voice-Activated Devices: IoT devices like smart speakers require ASR systems to enhance user interactions.
- Real-Time Applications: Live transcription and translation services are rapidly expanding.
- Accessibility Solutions: ASR is crucial for compliance with global accessibility standards.
- Healthcare Applications: Medical transcription and patient documentation automation are growing fields.
- Media and Entertainment: Automated captioning and subtitle generation are now industry standards.
By mastering ASR tools in Python, developers can tap into a thriving job market and secure lucrative, impactful roles in this rapidly evolving field.
Top 10 Exclusive Facts About ASR
- Origin: The first speech recognition system, Audrey, was developed by Bell Labs in 1952.
- Complexity: Modern ASR systems can recognize over 120 languages and dialects.
- Accuracy: ASR models trained on deep learning achieve up to 95% accuracy.
- Real-Time: Some systems can transcribe speech in real-time with minimal latency.
- Market Growth: The ASR market is projected to exceed $30 billion by 2030.
- Integration: ASR is integral to IoT devices like smart speakers.
- Challenges: Background noise and accent variations are major hurdles for ASR systems.
- Open Source: Many ASR frameworks, like DeepSpeech, are available for free.
- Healthcare: ASR aids in medical transcription, saving hours of manual effort.
- Future: Advancements in neural networks promise even more human-like recognition capabilities.
Top 30 FAQs About ASR
- What is ASR?
Automatic Speech Recognition (ASR) is the process of converting spoken language into text.
- How does ASR work?
It uses algorithms to process audio signals, recognize speech patterns, and transcribe them into text.
- Which Python library is best for ASR?
Popular libraries include SpeechRecognition, DeepSpeech, and Wav2Vec2.
- What are the key applications of ASR?
ASR is used in virtual assistants, transcription services, accessibility tools, and customer service.
- Can ASR understand multiple languages?
Yes, most modern ASR systems support multiple languages.
- Is ASR free to use?
Many open-source libraries like DeepSpeech are free, but APIs may have usage costs.
- What is the accuracy of ASR?
Depending on the model and conditions, ASR accuracy can range from 80% to 95%.
- How to handle noisy audio?
Pre-processing techniques like noise reduction can improve recognition.
- What is a language model in ASR?
It predicts word sequences to ensure meaningful transcription.
- Can ASR work offline?
Yes, some libraries like Vosk support offline recognition.
- What hardware is required for ASR?
A microphone and a standard computer are sufficient for basic ASR.
- Which deep learning model is best for ASR?
Models like Wav2Vec2 and DeepSpeech are popular for deep learning-based ASR.
- What is the role of neural networks in ASR?
Neural networks improve accuracy by learning complex patterns in speech.
- What file formats are supported?
Common formats include WAV, MP3, and FLAC.
- Is ASR suitable for medical transcription?
Yes, many healthcare providers use ASR for medical documentation.
- What is the cost of commercial ASR APIs?
Pricing varies; Google Speech-to-Text costs around $0.006 per 15 seconds of audio.
- How to improve ASR performance?
Use high-quality audio, pre-processing, and fine-tuning of models.
- Can ASR recognize accents?
Advanced models handle accents, but accuracy may vary.
- What is the role of feature extraction?
Extracting features like frequency and amplitude helps in accurate recognition.
- What industries benefit most from ASR?
Healthcare, education, customer service, and media heavily use ASR.
- How to train a custom ASR model?
Collect a dataset, preprocess it, and train using frameworks like TensorFlow or PyTorch.
- What are acoustic models?
They map audio signals to phonemes or basic speech units.
- Can ASR differentiate between speakers?
Yes, speaker diarization techniques can identify different speakers.
- Is ASR secure?
Encryption and privacy policies ensure security in ASR applications.
- What is the future of ASR?
The future involves more human-like interactions and multi-modal integration.
- Can ASR transcribe live conversations?
Yes, real-time ASR systems can handle live audio streams.
- What challenges does ASR face?
Accents, background noise, and homophones are common challenges.
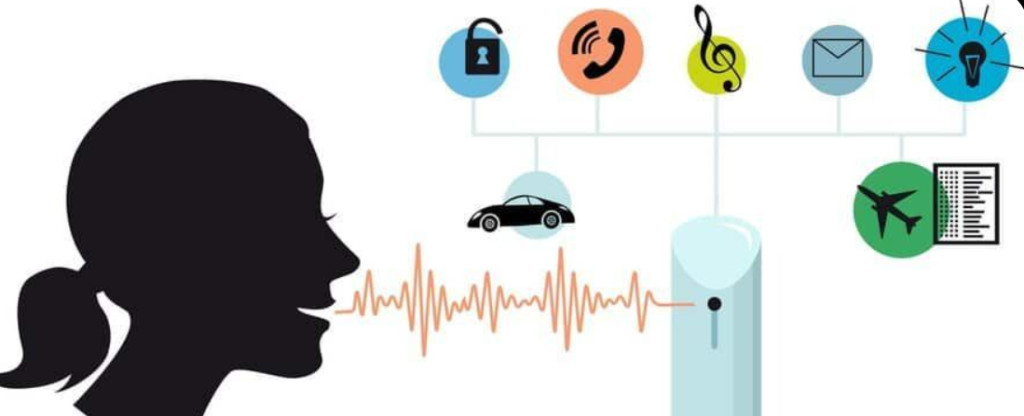
- Which ASR API is fastest?
Google Speech-to-Text and Amazon Transcribe are known for speed.
- What is the difference between ASR and NLP?
ASR converts speech to text, while NLP processes and understands the text.
- Can ASR be used for translation?
Yes, when integrated with machine translation systems.
Conclusion
Automatic Speech Recognition (ASR) is a transformative technology that bridges the gap between human communication and machine understanding. With Python’s robust ecosystem, developing ASR systems has become accessible to developers and researchers worldwide. Whether it’s for creating smart virtual assistants, automating transcription tasks, or enhancing accessibility, ASR continues to unlock new possibilities.
Python libraries like SpeechRecognition, DeepSpeech, and Wav2Vec2 offer flexible and efficient tools to implement ASR. By leveraging these libraries, developers can build applications that cater to diverse use cases across industries such as healthcare, education, and customer service. Despite challenges like handling noisy audio and accent variations, advancements in neural networks and deep learning have significantly improved ASR’s accuracy and reliability.
The future of ASR is promising, with trends leaning towards more natural interactions, multi-modal recognition, and enhanced language support. As the technology evolves, it’s crucial to stay informed and experiment with new tools and techniques. By understanding the fundamentals and exploring the potential of Python-based ASR, you can contribute to this dynamic field and create impactful solutions.
Best For You