CSS Programming Language, commonly known as CSS, is a foundational language used in web development to control the presentation of web pages. CSS allows developers to separate the structure (HTML) from the style (colors, fonts, layout) of a website, making it easier to manage and improve its visual appearance. Since its creation in 1996 by the World Wide Web Consortium (W3C), CSS has undergone multiple enhancements to support more complex designs, layouts, animations, and interactions.
CSS is used in tandem with HTML and JavaScript to create modern, responsive websites. HTML provides the structure, JavaScript adds interactivity, and CSS ensures that everything looks appealing. By using CSS, developers can ensure a consistent look and feel across all pages of a website, control the layout for various devices and screen sizes, and apply animations or dynamic effects. CSS is essential for responsive design, a crucial aspect of web development today, ensuring that websites look good on desktops, tablets, and mobile devices.
Moreover, CSS plays a significant role in improving website performance. By reducing the amount of inline styling and centralizing it into external stylesheets, web browsers can cache stylesheets, leading to faster page load times. This is particularly important for SEO, as search engines prioritize sites with quick load times.
In this chapter, we will delve deep into CSS’s syntax, how it works with HTML, its different selectors, properties, and more advanced features like Flexbox and Grid. Real-world examples will be included to illustrate how CSS is used to build modern web interfaces.
Table Of Contents
- What is CSS?
- Definition and Purpose of CSS
- History and Evolution of CSS
- Importance of CSS in Web Development
- How CSS Works
- The Relationship Between HTML, CSS, and JavaScript
- How CSS Styles Are Applied to HTML
- The Cascade and Inheritance Concept
- CSS Syntax and Structure
- Basic CSS Syntax: Selectors, Properties, and Values
- Inline, Internal, and External CSS
- Comments in CSS
- CSS Selectors
- Types of Selectors: Universal, Class, ID, Element
- Pseudo-Classes and Pseudo-Elements
- Attribute Selectors
- CSS Box Model
- Explanation of the Box Model: Content, Padding, Border, Margin
- Width and Height Properties
- Handling Overflow
- CSS Layout Techniques
- CSS Positioning: Static, Relative, Absolute, and Fixed Positioning
- Flexbox: Flexible Layouts for Web Design
- CSS Grid: Grid-Based Layout Systems
- Responsive Design: Media Queries and Mobile-First Approach
- Styling Text and Fonts
- Setting Font Properties: Size, Family, Weight
- Text Alignment, Decoration, and Line Height
- Google Fonts and Web Fonts Integration
- Colors, Backgrounds, and Gradients
- Working with Colors: RGB, Hex, HSL
- Background Properties: Images, Colors, and Repeat Options
- CSS Gradients: Linear and Radial Gradients
- CSS Transitions and Animations
- How to Create Smooth Transitions
- Keyframe Animations
- Real-world Example: Animating Buttons and Hover Effects
- CSS Frameworks and Libraries
- Overview of Popular CSS Frameworks (Bootstrap, Tailwind CSS)
- When and Why to Use CSS Frameworks
- Examples of Responsive Websites Using CSS Frameworks
- Keeping CSS Organized: Comments and Consistent Naming
- Avoiding Common Pitfalls like Specificity Issues
- Optimizing CSS for Performance and SEO
- Sample CSS File (A good starting point)
- Common FAQs about CSS
1. What is CSS?
CSS, or Cascading Style Sheets, is a language used to describe the presentation of a document written in HTML or XML. It is responsible for how the web page looks, including aspects like layout, colors, fonts, and spacing. CSS allows for separating content from design, giving developers the flexibility to make global changes across multiple pages by simply editing one stylesheet.
CSS was developed to enhance HTML’s limitations in designing web pages. HTML is great for structuring content but lacks the styling features needed to create visually appealing websites. The first version of CSS, CSS1, was introduced by W3C in 1996, followed by CSS2 in 1998, and the current CSS3 standard, which is modular and allows for easier updates and new features.
CSS is a critical component of modern web development. Without it, websites would look plain, with no formatting beyond the basic structure provided by HTML. CSS also enables responsive design, making websites adaptable to various screen sizes and devices.
2. How CSS Works
CSS works by applying style rules to HTML elements. Each rule targets an HTML element or group of elements and applies specific styles. For example, you can apply a different background color to a <div> or change the font size of a <p> element. These style rules can be written inline, within the HTML document (internal CSS), or stored in a separate CSS file (external CSS).
CSS’s “Cascading” nature means that styles are applied in a specific order based on their location and importance. This is referred to as the “cascade.” When multiple style rules apply to the same element, CSS uses the cascade to determine which rule takes precedence. The browser reads and applies these rules from top to bottom, respecting the inheritance and specificity rules.
3. CSS Syntax and Structure
CSS follows a simple yet powerful syntax, where each rule consists of a selector, property, and value:
selector {
property: value;
}
- Selector: Targets the HTML element (e.g., p for paragraphs).
- Property: Defines the style aspect (e.g., color or font-size).
- Value: Specifies the style (e.g., red or 16px).
CSS can be applied in three ways:
- Inline CSS: Directly within HTML elements using the style attribute.
- Internal CSS: Inside a <style> tag in the HTML <head>.
- External CSS: In a separate .css file linked to the HTML document.
4. CSS Selectors
CSS selectors are patterns used to select and style HTML elements. The most common types include:
- Universal Selector (*): Targets all elements.
- Element Selector: Targets all instances of a specific HTML element (e.g., p for all paragraphs).
- Class Selector (.classname): Targets elements with a specific class attribute.
- ID Selector (#idname): Targets the element with a specific ID.
- Pseudo-Classes and Pseudo-Elements: These selectors allow targeting of specific states (e.g., :hover for hovering over elements) or parts of an element (e.g., ::before to insert content before an element).
5. CSS Box Model
Every HTML element can be seen as a box, composed of four parts:
- Content: The actual content inside the element.
- Padding: Space between the content and the border.
- Border: The edge surrounding the padding.
- Margin: Space outside the border, separating the element from other elements.
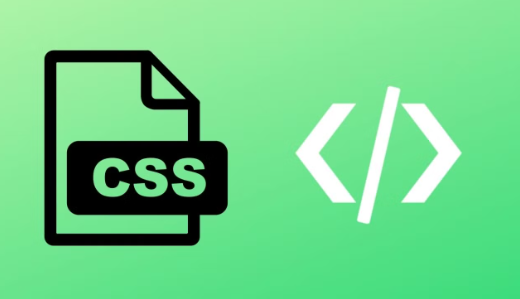
Understanding the box model is crucial for controlling the layout and spacing of web pages.
6. CSS Layout Techniques
CSS provides multiple layout techniques to arrange elements:
- Positioning: Using static, relative, absolute, or fixed to control how elements are placed.
- Flexbox: A layout system designed to provide a more efficient way to arrange items in a container.
- Grid: A powerful two-dimensional layout system that divides the page into rows and columns, allowing for complex layouts.
- Media Queries: Enable responsive design by applying different styles for various screen sizes.
7. Styling Text and Fonts
CSS allows you to style text using properties like:
- font-family: Choose the font for the text.
- font-size: Set the size of the text.
- text-align: Align text left, right, center, or justify.
You can also import web fonts like Google Fonts to enhance typography.
8. Colors, Backgrounds, and Gradients
CSS supports multiple color formats (e.g., RGB, Hex) and allows for styling backgrounds with images or colors. You can also create smooth gradients, which are widely used in modern web design for creating transitions between colors.
9. CSS Transitions and Animations
CSS transitions and animations can bring elements to life. You can define how properties change over time, enabling smooth transitions for effects like button hovers. Keyframes allow creating complex animations that transform properties in stages.
10. CSS Frameworks and Libraries
Popular CSS frameworks like Bootstrap and Tailwind CSS provide pre-designed components and utilities that help speed up development. These frameworks are widely used to create responsive, mobile-first websites with minimal custom styling.
11. CSS Best Practices
To maintain efficient, scalable CSS, follow best practices such as:
- Organized code: Keep your CSS well-structured with comments.
- Consistent naming conventions: Avoid conflicts by using clear, consistent class and ID names.
- Minimize specificity: Use simple selectors to avoid specificity issues.
Optimizing CSS improves site performance and SEO, ensuring fast load times and better rankings.
Sample CSS File
Here’s a sample CSS file for a simple dummy website that styles the layout, typography, and basic elements like navigation, buttons, and footer. The website could be for a personal portfolio, blog, or a basic company landing page.
/* General Styling */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Arial', sans-serif;
line-height: 1.6;
color: #333;
background-color: #f4f4f4;
padding: 20px;
}
h1, h2, h3 {
color: #333;
margin-bottom: 20px;
}
p {
margin-bottom: 15px;
color: #555;
}
/* Header Styling */
header {
background-color: #4CAF50;
color: #fff;
padding: 20px 0;
text-align: center;
}
header h1 {
font-size: 2.5rem;
}
/* Navigation Styling */
nav {
display: flex;
justify-content: center;
background-color: #333;
}
nav ul {
list-style-type: none;
display: flex;
}
nav ul li {
margin: 0 20px;
}
nav ul li a {
color: #fff;
text-decoration: none;
font-weight: bold;
padding: 15px;
}
nav ul li a:hover {
background-color: #4CAF50;
border-radius: 5px;
}
/* Main Section */
.main-content {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
margin: 20px 0;
}
.main-content .card {
background-color: #fff;
padding: 20px;
margin: 10px;
width: 30%;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.main-content .card img {
width: 100%;
height: auto;
}
.main-content .card h3 {
margin-bottom: 10px;
}
.main-content .card p {
margin-bottom: 10px;
color: #777;
}
.main-content .card a {
display: inline-block;
padding: 10px 20px;
background-color: #4CAF50;
color: #fff;
text-decoration: none;
border-radius: 5px;
transition: background-color 0.3s ease;
}
.main-content .card a:hover {
background-color: #333;
}
/* Footer */
footer {
background-color: #333;
color: #fff;
text-align: center;
padding: 10px 0;
position: absolute;
width: 100%;
bottom: 0;
}
footer p {
margin: 0;
}
footer a {
color: #4CAF50;
text-decoration: none;
}
/* Media Queries for Responsiveness */
@media (max-width: 768px) {
.main-content {
flex-direction: column;
align-items: center;
}
.main-content .card {
width: 80%;
}
nav ul li {
margin: 0 10px;
}
}
Explanation of the Sample CSS File:
- Global Reset and General Styling:
- The * { margin: 0; padding: 0; } resets default margins and paddings across all elements.
- body sets up the basic font, background color, and text color for the entire site.
- Header Section:
- The header section has a green background with white text and is center-aligned.
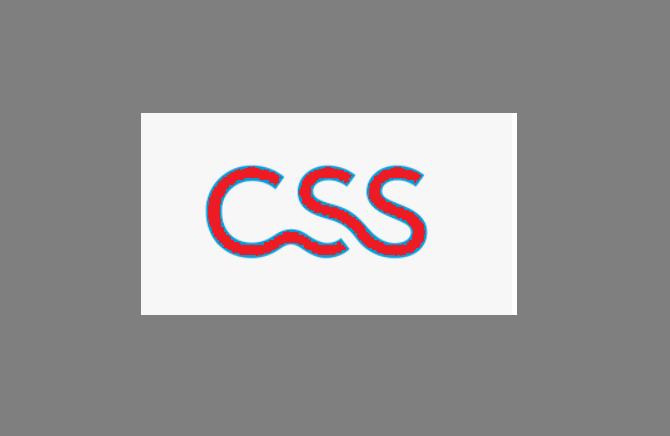
- Navigation:
- The navigation bar uses flexbox for horizontal alignment of links. Each list item (menu link) is styled with a hover effect that changes the background to green.
- Main Content Section:
- This section is designed to hold multiple cards (e.g., for blog posts or project showcases).
- Each card has an image, title, description, and a button-style link. The box-shadow property adds a subtle shadow to the cards for a 3D effect.
- Footer:
- The footer has a dark background with white text and links styled in green.
- Media Queries:
- Responsive design is handled with media queries. Below 768px screen width (for tablets and smaller devices), the layout changes from a 3-column design to a single column, making the content easier to view on smaller screens.
This CSS file gives you a good starting point for creating a basic responsive website layout. The key areas (header, navigation, main content, and footer) are styled in a modern, clean design. You can further expand it depending on the website’s complexity.
Common FAQs About CSS
1. What is CSS, and why is it important?
CSS (Cascading Style Sheets) is a language used to style and format web pages. It allows developers to control the look and feel of a website by applying styles such as colors, fonts, layouts, and animations. CSS is crucial because it separates content (HTML) from design, making websites more visually appealing, easier to maintain, and faster to load.
2. What are the different ways to apply CSS to a web page?
CSS can be applied in three ways:
- Inline CSS: Written directly inside an HTML element using the style attribute.
- Internal CSS: Included in the <head> section of an HTML file using the <style> tag.
- External CSS: Written in a separate .css file and linked to the HTML document using the <link> tag. External CSS is the most preferred method as it allows for easier maintenance and reusability across multiple web pages.
3. What is the CSS box model?
The CSS box model refers to the structure of an HTML element, which includes:
- Content: The text or images inside the element.
- Padding: The space between the content and the border.
- Border: The edge surrounding the padding.
- Margin: The space outside the border, separating the element from other elements. Understanding the box model is crucial for managing element layout and spacing on web pages.
4. What are CSS selectors?
CSS selectors are used to target and style specific HTML elements. Common types of selectors include:
- Element Selector: Targets HTML tags like p, h1, or div.
- Class Selector: Targets elements with a specific class attribute (e.g., .classname).
- ID Selector: Targets elements with a unique ID (e.g., #idname).
- Attribute Selector: Targets elements based on their attributes (e.g., [type=”text”]).
5. What is the difference between a class and an ID in CSS?
- Class: A class can be applied to multiple elements on the same page and is defined with a dot (.) in CSS (e.g., .classname).
- ID: An ID is unique and can only be applied to one element per page. It is defined with a hash symbol (#) in CSS (e.g., #idname). IDs have higher specificity than classes, meaning they will override class styles if both are applied to the same element.
6. What are CSS media queries?
Media queries are used to apply different styles depending on the user’s device or screen size. They are essential for creating responsive designs. A common example is:
@media (max-width: 768px) {
/* Styles for tablets and smaller devices */
}
This ensures that your website looks good on desktops, tablets, and mobile devices by adapting the layout and styles accordingly.
7. What are CSS Flexbox and Grid?
- Flexbox: A layout model designed to help align and distribute space among items within a container. It is one-dimensional, meaning it works either in a row or a column.
- CSS Grid: A two-dimensional layout system that allows you to create complex grid-based layouts. It supports both rows and columns and offers more control for creating structured layouts.
8. What are pseudo-classes and pseudo-elements in CSS?
- Pseudo-classes: Used to style elements based on their state or position, such as when an element is hovered over or the first child in a list (e.g., :hover, :first-child).
- Pseudo-elements: Allow you to style specific parts of an element, such as the first letter or inserting content before or after an element (e.g., ::before, ::first-letter).
9. What is the difference between position: absolute and position: relative?
- position: absolute: Positions an element relative to its nearest positioned ancestor (not the default document flow). It is removed from the normal document flow.
- position: relative: Positions an element relative to its normal position in the document flow. It remains in the flow, and other elements around it are not affected.
10. What are common CSS units used for length?
CSS supports several units to define the size of elements:
- px: Pixels (absolute unit).
- %: Percentage (relative to parent element).
- em: Relative to the font size of the parent element.
- rem: Relative to the font size of the root element (typically the <html> element).
- vw: Viewport width, relative to the full width of the viewport.
- vh: Viewport height, relative to the full height of the viewport.
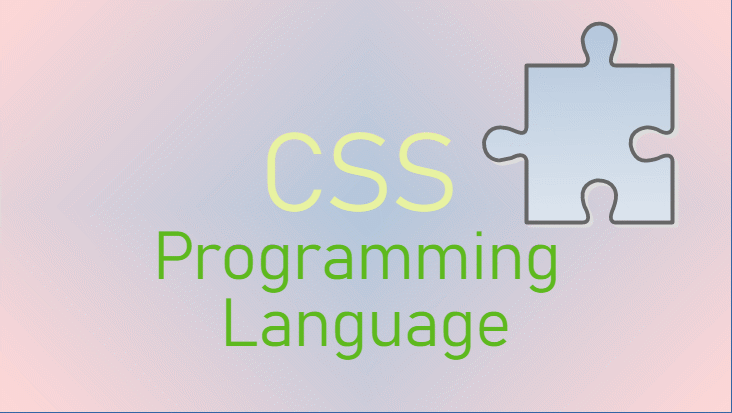
11. How can I center an element using CSS?
There are multiple ways to center an element depending on the layout:
- Horizontal centering with text-align: For inline elements or text, use text-align: center; in the parent container.
- Vertical and horizontal centering with Flexbox: For block elements, you can use Flexbox:
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
This centers the child element both vertically and horizontally within the container.
12. What is a CSS framework, and why should I use one?
A CSS framework is a pre-designed library of styles and components that help speed up the web development process. Popular frameworks include Bootstrap and Tailwind CSS. These frameworks provide pre-built grids, buttons, navigation, and other UI elements, making it easier to create responsive, mobile-first websites without writing extensive custom CSS.
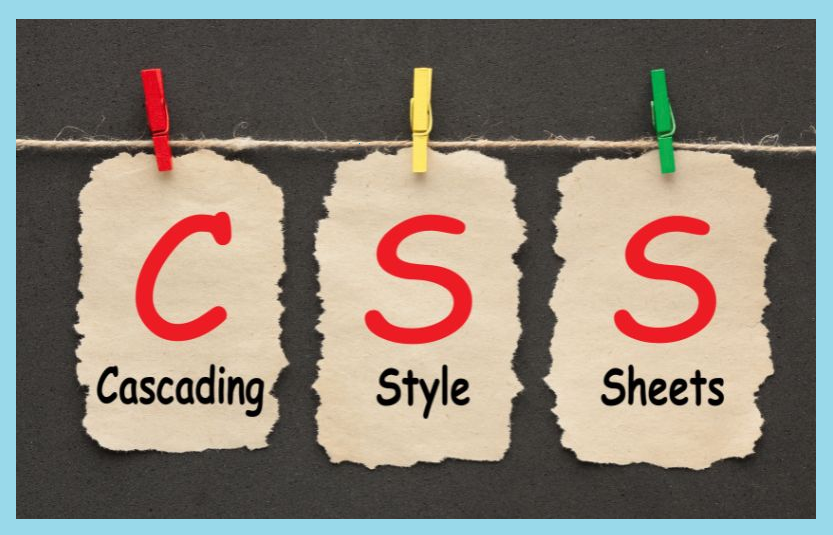
13. What is the purpose of !important in CSS?
The !important rule is used to give a CSS property higher precedence. When applied, it will override any other conflicting styles, regardless of specificity:
p {
color: red !important;
}
However, it should be used sparingly, as it can make debugging and maintaining styles more difficult.
14. What is CSS specificity?
CSS specificity determines which style rules are applied when multiple rules target the same element. Specificity is calculated based on the type of selectors used:
- Inline styles have the highest specificity.
- ID selectors have more specificity than class or element selectors.
- Class selectors are more specific than element selectors. Understanding specificity helps avoid conflicts between different style rules.
15. How can I optimize CSS for performance?
To optimize CSS for performance:
- Minimize the use of large and complex selectors: Use simpler, more direct selectors.
- Remove unused CSS: Tools like PurgeCSS can help you eliminate unused styles.
- Use external stylesheets: Place CSS in an external file, allowing the browser to cache it for faster loading.
- Minify CSS: Remove whitespace, comments, and extra characters using a CSS minification tool.
- Reduce the number of @import rules: Use <link> to include external stylesheets instead of @import inside CSS files, which can delay loading.
These FAQs provide a foundational understanding of CSS and cover many common questions developers may encounter when learning or working with CSS in web development.
This article gives you a comprehensive guide to CSS, covering its basic structure, how it works, advanced techniques, and best practices for efficient web design.
For you
Noodlemagazine: I like the efforts you have put in this, regards for all the great content.
My brother recommended I might like this web site He was totally right This post actually made my day You cannt imagine just how much time I had spent for this information Thanks