Python’s with
statement is a powerful tool for managing resources. Introduced in PEP 343 and available since Python 2.5, it simplifies exception handling and ensures that clean-up code is executed, making the code cleaner and more readable. This feature has evolved over time, and Python 3.12 continues to leverage its benefits to enhance resource management.
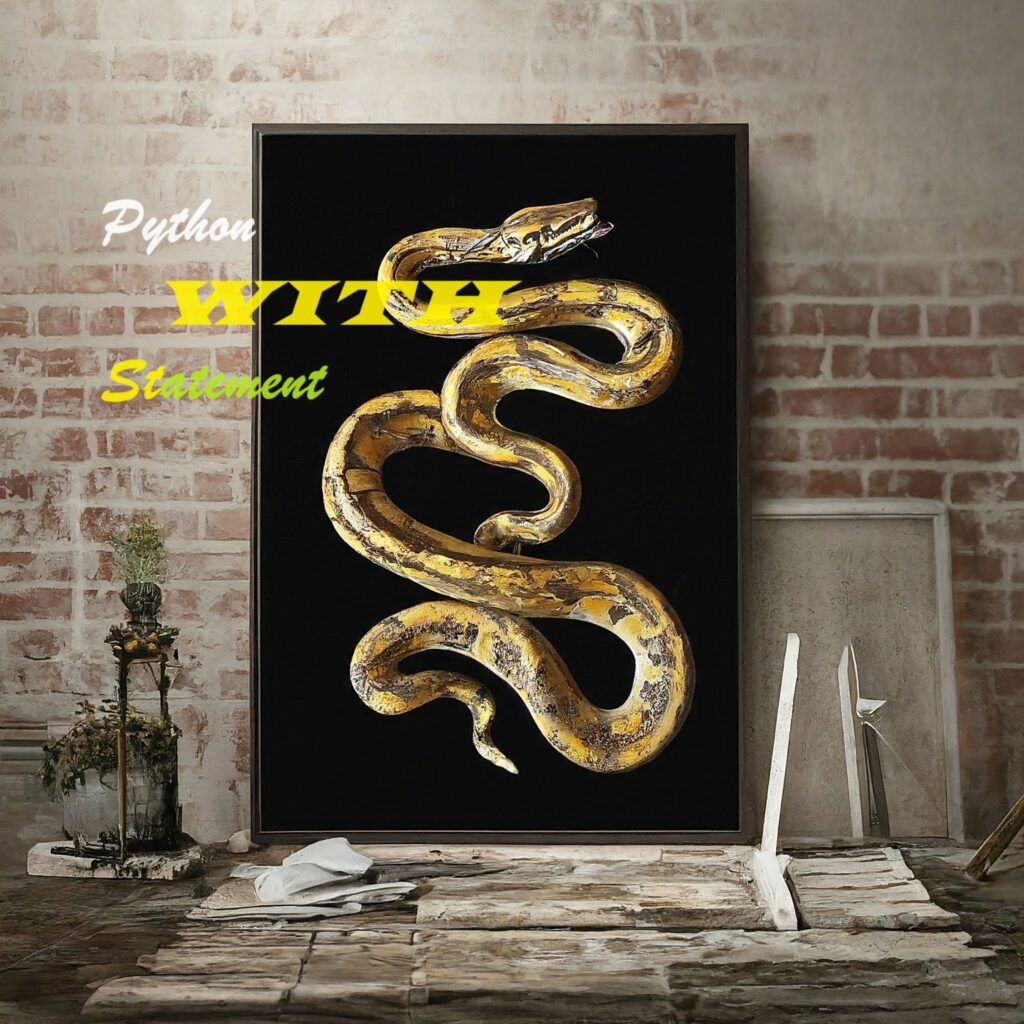
The Basics of the with
Statement
The with
statement simplifies resource management by abstracting the try-except-finally pattern, which is commonly used to handle resources like file I/O, network connections, and locks. The typical structure of the with
statement is:
with expression as variable:
# Your code here
The expression
is expected to return a context manager, which must implement two methods: __enter__
and __exit__
.
__enter__(self)
: This method is executed before the code block is run. It can set up the resource and optionally return a value that is assigned tovariable
.__exit__(self, exc_type, exc_value, traceback)
: This method is executed after the code block is run, regardless of whether the block exits normally or via an exception. It handles any clean-up operations, such as closing a file or releasing a lock.
Real-World Examples
Let’s dive into several real-world examples to illustrate the usage and benefits of the with
statement in Python.
Example 1: File Handling
File operations are a common use case for the with
statement. Without with
, file handling requires explicit closing of the file, which can be prone to errors if not handled correctly.
Without with
:
file = open('example.txt', 'w')
try:
file.write('Hello, world!')
finally:
file.close()
With with
:
pythonCopy codewith open('example.txt', 'w') as file:
file.write('Hello, world!')
In this example, the with
statement ensures that the file is properly closed after the block of code is executed, even if an exception occurs.
Example 2: Handling Database Connections
Managing database connections efficiently is crucial for maintaining performance and reliability in applications. The with
statement helps in ensuring that connections are closed after use.
import sqlite3
with sqlite3.connect('example.db') as connection:
cursor = connection.cursor()
cursor.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)')
cursor.execute('INSERT INTO users (name) VALUES (?)', ('Alice',))
connection.commit()
Here, the with
statement ensures that the database connection is closed properly, preventing potential connection leaks.
Example 3: Threading Locks
In multithreaded applications, managing locks is critical to avoid race conditions. The with
statement can simplify lock management.
import threading
lock = threading.Lock()
def thread_safe_function():
with lock:
# Critical section of code
print('Thread-safe code execution')
# Creating multiple threads
threads = [threading.Thread(target=thread_safe_function) for _ in range(5)]
for thread in threads:
thread.start()
for thread in threads:
thread.join()
In this example, the with
statement ensures that the lock is acquired before the critical section is executed and released afterward, making the code thread-safe.
Example 4: Custom Context Managers
You can create your own context managers using the contextlib
module, which provides utilities for creating and working with context managers.
from contextlib import contextmanager
@contextmanager
def managed_resource(resource):
try:
resource.setup()
yield resource
finally:
resource.teardown()
class Resource:
def setup(self):
print("Setting up resource")
def teardown(self):
print("Tearing down resource")
with managed_resource(Resource()) as res:
print("Using resource")
In this example, a custom context manager is created using the contextmanager
decorator. The setup
and teardown
methods of the Resource
class are called automatically by the with
statement.
Example 5: Suppressing Exceptions
The contextlib
module also provides a suppress
context manager, which can be used to ignore specified exceptions.
from contextlib import suppress
with suppress(FileNotFoundError):
open('non_existent_file.txt')
print("This will not raise an exception if the file does not exist")
In this case, the suppress
context manager ignores the FileNotFoundError
exception, allowing the program to continue executing.
Deep Dive: Context Manager Protocol
To fully understand the with
statement, it’s important to delve into the context manager protocol, which consists of the __enter__
and __exit__
methods.
Implementing a Context Manager Class
Let’s create a custom context manager by implementing the protocol directly.
class MyContextManager:
def __enter__(self):
print("Entering the context")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting the context")
if exc_type:
print(f"An exception occurred: {exc_value}")
return True # Suppress the exception
with MyContextManager() as manager:
print("Inside the context")
raise ValueError("An example exception")
Output:
Entering the context
Inside the context
Exiting the context
An exception occurred: An example exception
In this example, the MyContextManager
class implements the __enter__
and __exit__
methods. The __exit__
method suppresses the exception by returning True
.
Enhancements in Python 3.12
Python 3.12 introduces several enhancements and optimizations that further improve the usability and performance of the with
statement. Some notable changes include:
1. Performance Improvements
Python 3.12 includes various performance optimizations that make context manager operations faster. These optimizations reduce the overhead of entering and exiting context blocks, making the with
statement more efficient in resource-intensive applications.
2. Enhanced Error Messages
Error messages related to context managers have been improved to provide more clarity and detail. This helps developers diagnose and fix issues more quickly.
3. New Context Managers
Python 3.12 expands the standard library with additional context managers for common tasks. For example, the contextlib
module has been extended to include new utility functions that simplify context management.
Advanced Usage Patterns
Let’s explore some advanced usage patterns of the with
statement, demonstrating its flexibility and power in various scenarios.
Nested Context Managers
You can nest multiple context managers within a single with
statement for better readability and resource management.
with open('file1.txt', 'w') as file1, open('file2.txt', 'w') as file2:
file1.write('Hello from file1')
file2.write('Hello from file2')
This approach ensures that both files are properly closed, even if an error occurs while working with either file.
Context Manager for Timing Code Execution
Measuring the execution time of code blocks is a common requirement in performance analysis. You can create a context manager for this purpose.
import time
class Timer:
def __enter__(self):
self.start_time = time.time()
return self
def __exit__(self, exc_type, exc_value, traceback):
self.end_time = time.time()
print(f"Elapsed time: {self.end_time - self.start_time:.4f} seconds")
with Timer():
# Code block to measure
sum(range(1000000))
In this example, the Timer
context manager measures and prints the elapsed time of the code block.
Practical Considerations
While the with
statement simplifies resource management, it’s essential to understand its limitations and best practices.
1. Context Managers and Performance
While the with
statement introduces minimal overhead, in performance-critical sections, even small overheads can accumulate. Always profile your code to ensure that the use of context managers is justified.
2. Context Manager Nesting
Nesting too many context managers can make the code harder to read. When managing multiple resources, consider refactoring the code to use helper functions or classes to maintain readability.
3. Exception Handling
Context managers handle exceptions by design, but it’s crucial to handle specific exceptions appropriately within the __exit__
method. Returning True
from __exit__
suppresses the exception, which may not always be the desired behavior.
Conclusion
The with
statement is a fundamental feature in Python that streamlines resource management, ensuring that resources are properly acquired and released. Its use extends across various domains, from file handling and database connections to threading and custom resource management.
Python 3.12 continues to enhance the with
statement, providing performance improvements, better error messages, and new context managers. Understanding and effectively utilizing the with
statement is essential for writing clean, maintainable, and efficient Python code.
By mastering the with
statement and its underlying context manager protocol, you can significantly improve the robustness and reliability of your applications, making resource management a seamless and error-free process. Whether you’re handling files, managing database connections, or ensuring thread safety, the with
statement is a powerful ally in your Python programming toolkit.
More for you: FastAPI: The Next Generation of Web Frameworks in Python – LearnXYZ
if you work as freelancer if any buyer message you on fiverr, upwork, freelancer or anywhere Quick Alert for Freelancers and Entrepreneurs Software will instantly notified you by Phone call.
if you are looking whatsapp bulk message sender , web scraper, social leads extractor for free with original license for 1 year. join our telegram channel by clicking my name.
Thanks to Diviashop, my business is fully set up, and the payment solutions are working perfectly.
The advice and tips on this blog have been truly helpful. I’ve gained so much insight into various topics. If you’re interested in learning about business formation for non-US and UK residents, click my name.
tony robbins – business accelerator 2024
Download Free Internet marketing Courses & Money Making Courses With Free & Premium Plan. Get it Today.
Diviashop is a highly recommended, trusted, and reputable site since 2022, delivering 100 quality service for setting up high-risk merchant accounts.
Hello, Neat post. There’s an issue along with your website in web explorer, would test thisK IE still is the marketplace chief and a good component of folks will leave out your fantastic writing due to this problem.
I am genuinely thankful to the owner of this website for sharing his brilliant ideas. I can see how much you’ve helped everybody who comes across your page. By the way, here is my webpage UY5 about Thai-Massage.
Utilize platforms dedicated to web 2.0 submissions for expanding your online presence.
Your tips on email marketing strategy implementation have been insightful.