Python Functions: A Comprehensive Guide
In the last article (Chapter) we saw how to handle Python exceptions, in this article we will delve into Python, functions. Python functions are reusable blocks of code that perform specific tasks. They help organize code, reduce redundancy, and enhance modularity. Functions are defined using the def keyword and can take arguments, perform computations, and return values. Lets have a look at each benefit we can obtain by using the function in detail.
Table of Contents
Benefits of Python Functions and Why You Should Use Them..
Types of User-defined Functions.
Writing Efficient Functions in Python 3.12
Benefits of Python Functions and Why You Should Use Them
Python functions are an essential part of writing clean, efficient, and reusable code. Functions help in organizing the code, reducing redundancy, and improving maintainability. Below are the key benefits of using functions in Python:
1. Code Reusability
Functions allow you to write a block of code once and reuse it multiple times in your program. Instead of duplicating code, you can define a function and call it whenever needed. This makes the code shorter and easier to manage.
Example:
def greet(name):
return f"Hello, {name}!"
# Reuse the greet function multiple times
print(greet("Alice"))
print(greet("Bob"))
2. Modular Code
Functions help you break down a large problem into smaller, manageable pieces. This modular approach makes it easier to understand, debug, and update the code. If one part of the program needs a change, you can modify the specific function without affecting the rest of the code.
Example:
def calculate_area(length, width):
return length * width
def calculate_perimeter(length, width):
return 2 * (length + width)
# Separate functions for modular code
area = calculate_area(10, 5)
perimeter = calculate_perimeter(10, 5)
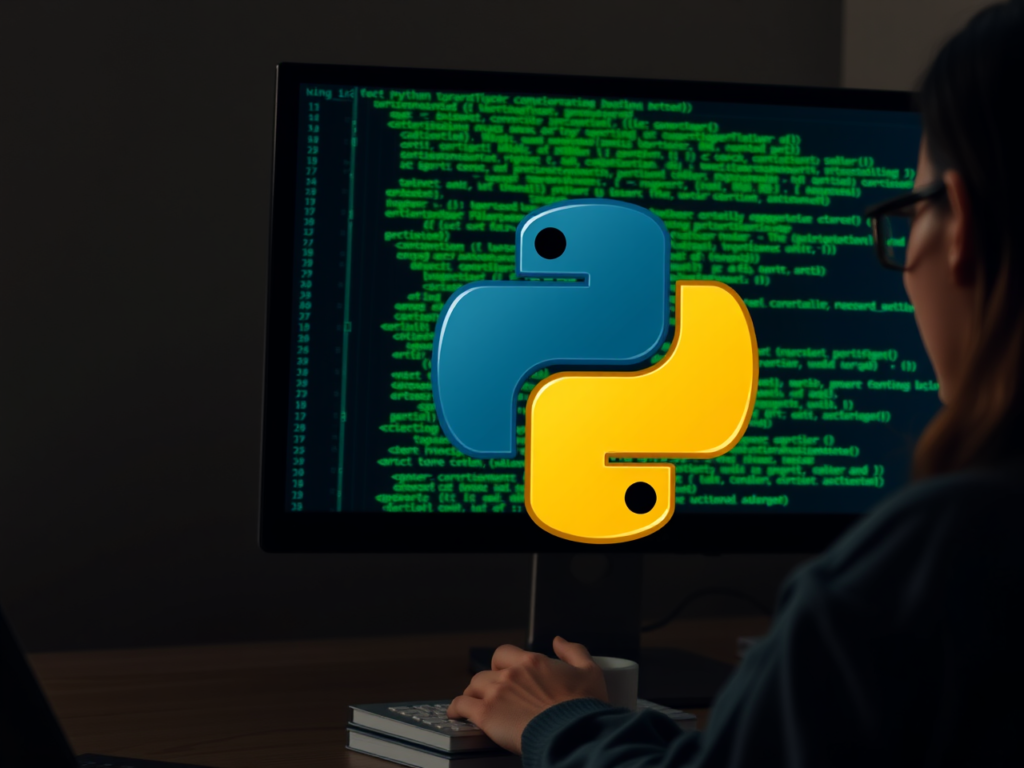
3. Improves Readability and Maintainability
Functions allow you to give descriptive names to blocks of code. This makes the code more readable because it provides a clear, logical flow. Well-named functions also make it easier for others (or even your future self) to understand and maintain the code over time.
Example:
def is_even(number):
return number % 2 == 0
# Descriptive function names improve code readability
if is_even(8):
print("8 is an even number")
4. Encapsulation and Abstraction
Functions encapsulate logic, meaning the internal workings of the function are hidden from the user. You only need to know what the function does, not how it works. This abstraction helps in focusing on higher-level logic without worrying about low-level details.
Example:
# You don’t need to know how square() works internally, just use it
def square(x):
return x * x
result = square(5) # Focus on the result, not the internal mechanism
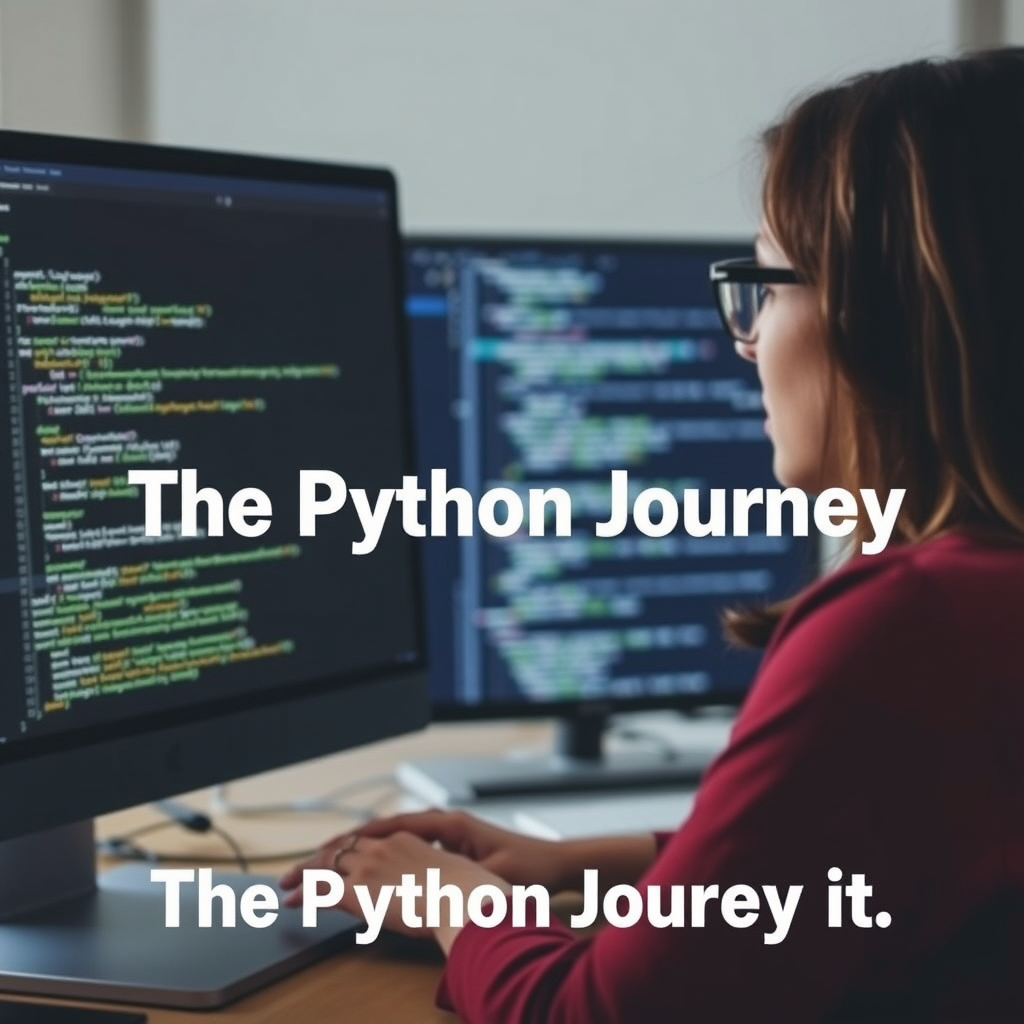
5. Easier Testing and Debugging
Functions make it easy to test small parts of the code independently. Instead of testing the entire program at once, you can test individual functions to ensure they work correctly. This makes debugging easier and reduces the risk of introducing bugs.
Example:
def add(a, b):
return a + b
# You can test the add() function in isolation
assert add(3, 5) == 8
assert add(-1, 1) == 0
6. Reduces Redundancy
By using functions, you can avoid repeating the same code multiple times. This reduces redundancy and helps prevent errors. If a change is needed, you only need to update the function in one place, and it will reflect everywhere the function is called.
Example:
# Without functions: repeating the same code
print(2 * 3.14159 * 5) # Circumference of a circle with radius 5
print(2 * 3.14159 * 10) # Circumference of a circle with radius 10
# With functions: no repetition
def circumference(radius):
return 2 * 3.14159 * radius
print(circumference(5))
print(circumference(10))
7. Supports Recursion
Python functions can call themselves (recursion), which is helpful for solving problems that can be divided into smaller, identical problems (e.g., calculating factorials or performing tree traversals).
Example:
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1)
# Calculate the factorial of 5
print(factorial(5)) # Output: 120
8. Parameterization and Flexibility
Functions allow you to pass different arguments, providing flexibility to handle different inputs dynamically. This allows you to write generic functions that can work with a wide variety of input values.
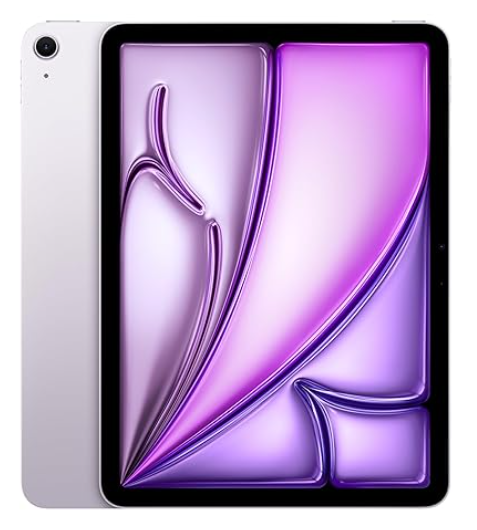
Apple iPad Air 11″ (M2): Liquid Retina Display, 128GB, Landscape 12MP Front Camera / 12MP Back Camera, Wi-Fi 6E, Touch ID, All-Day Battery Life — Purple
₹59,900/-
Example:
def greet(name, greeting="Hello"):
return f"{greeting}, {name}!"
# You can change the greeting dynamically
print(greet("Alice")) # Output: Hello, Alice!
print(greet("Bob", "Hi")) # Output: Hi, Bob!
9. Supports Functional Programming Paradigms
Python functions support functional programming features like higher-order functions (functions that take other functions as arguments) and lambda expressions (anonymous functions), allowing for more concise and expressive code.
Example:
# Using a higher-order function with lambda
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, numbers)) # Output: [1, 4, 9, 16, 25]
Using functions in Python provides numerous benefits, including code reuse, modularity, readability, and reduced redundancy. They encapsulate logic, improve maintainability, and support efficient testing and debugging. By leveraging functions, you can write more organized, flexible, and scalable code, making your Python development faster and more efficient. Functions are an indispensable tool in any Python programmer’s toolkit!
Types of Functions in Python
Python supports different types of functions:
- Built-in Functions: Python comes with pre-defined functions like print(), len(), and type(), which provide basic functionalities.
- User-defined Functions: These are functions created by the programmer to perform specific tasks.
Example of a User-defined Function:
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Types of User-defined Functions
1. Default Argument Functions
Functions can have default arguments, meaning the argument takes a default value if none is provided.
Example:
def greet(name="Guest"):
return f"Hello, {name}!"
print(greet()) # Output: Hello, Guest!
2. Keyword Argument Functions
In keyword arguments, the caller can pass values by explicitly stating parameter names.
Example:
def greet(name, message):
return f"{message}, {name}!"
print(greet(name="Alice", message="Hi"))
3. Variable-Length Arguments Functions
These functions allow passing an arbitrary number of arguments using *args or **kwargs.
Example:
def sum_numbers(*args):
return sum(args)
print(sum_numbers(1, 2, 3, 4)) # Output: 10
3. Nested Functions
Nested functions are functions defined within other functions. They can only be accessed inside the enclosing function.
Example:
def outer():
def inner():
return "Inner Function"
return inner()
print(outer()) # Output: Inner Function
4. Functions within a Class (Methods)
When functions are defined inside a class, they are called methods. A method can be accessed using an object of that class.
Example:
class Person:
def greet(self, name):
return f"Hello, {name}!"
person = Person()
print(person.greet("Alice")) # Output: Hello, Alice!
Writing Efficient Functions in Python 3.12
Writing efficient functions in Python is crucial to optimize performance and resource usage, especially when working with larger applications. With Python 3.12, developers can take advantage of several tricks to write more efficient, readable, and maintainable functions. Here are some of the best practices to improve function efficiency.
Avoid Global Variables
Global variables slow down function execution because the function has to search for the variable in multiple scopes (local, enclosing, and global). Instead, pass variables as arguments to functions. This avoids the overhead of searching for global variables.
Example:
# Avoid this
x = 10
def inefficient_function():
return x + 5
# Better approach
def efficient_function(x):
return x + 5
Use Built-In Functions and Libraries
Python has a rich set of built-in functions and libraries optimized in C, which are generally faster than custom implementations in pure Python. Functions like sum(), max(), min(), and list comprehensions are more efficient than writing loops manually.
Example:
# Inefficient - using manual loop
def sum_list(lst):
total = 0
for i in lst:
total += i
return total
# Efficient - using built-in sum() function
def efficient_sum_list(lst):
return sum(lst)
Leverage List Comprehensions and Generator Expressions
List comprehensions are faster and more efficient than traditional loops for creating lists. Generator expressions, which use lazy evaluation, are even more memory-efficient, especially when working with large datasets.
Example:
# List comprehension (efficient)
squares = [x**2 for x in range(10)]
# Generator expression (memory-efficient)
squares_gen = (x**2 for x in range(10))
The generator version does not create the entire list in memory, making it ideal for working with large datasets.
Use functools.lru_cache for Memoization
Python’s functools.lru_cache allows you to cache function results. This is particularly useful for recursive functions or functions that are repeatedly called with the same inputs. It helps avoid recalculating the same results, thus boosting efficiency.
Example:
from functools import lru_cache
# Inefficient recursive Fibonacci
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Efficient with lru_cache
@lru_cache(maxsize=None)
def efficient_fibonacci(n):
if n <= 1:
return n
return efficient_fibonacci(n-1) + efficient_fibonacci(n-2)
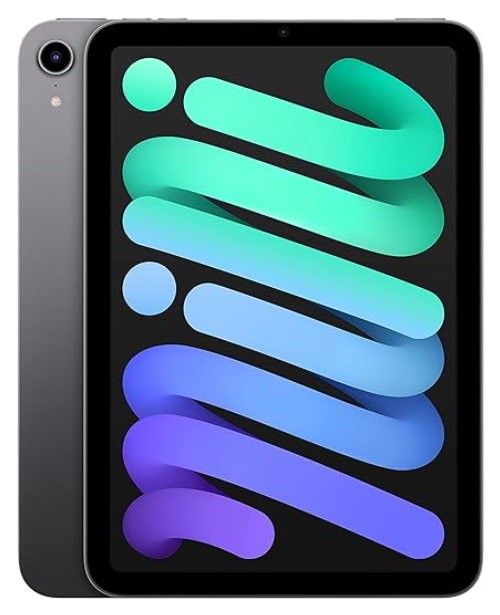
Apple iPad Mini (6th Generation)
With A15 Bionic chip, 21.08 cm (8.3″) Liquid Retina Display, 64GB, Wi-Fi 6, 12MP front/12MP Back Camera, Touch ID, All-Day Battery Life – Space Grey
-3% Off ₹48,399
Minimize Function Calls in Loops
Calling a function inside a loop can significantly slow down performance, especially if the function is simple. Instead, move the function call outside the loop or inline the function if possible.
Example:
# Less efficient
def calculate_square(x):
return x**2
for i in range(1000000):
square = calculate_square(i)
# More efficient (move logic inside the loop)
for i in range(1000000):
square = i**2
Avoid Unnecessary Object Creation
Repeatedly creating new objects inside functions can lead to memory bloat and slow down performance. Reuse objects where possible, and prefer immutable types like tuples when appropriate.
Example:
# Inefficient - unnecessary object creation
def create_list(n):
result = []
for i in range(n):
result.append(i)
return result
# Efficient - use list comprehension
def create_list_efficiently(n):
return [i for i in range(n)]
Accessing Python Functions Across Files
In Python, functions can be stored in different files (modules) and accessed from other files or modules, which helps organize large codebases. Here’s how you can access Python functions across files, whether they are in classes or not.
Accessing Functions from a Class in a Different File
- Step 1: Create a Python file (module) with a class and its methods.
File: math_operations.py
# math_operations.py
class MathOperations:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
- Step 2: Import and access the class and its methods from another file.
File: main.py
# main.py
from math_operations import MathOperations
# Create an instance of the class
math_ops = MathOperations()
# Call the methods
result_add = math_ops.add(5, 3)
result_subtract = math_ops.subtract(10, 4)
print(f"Addition: {result_add}, Subtraction: {result_subtract}")
In this example, we import the MathOperations class from math_operations.py and create an instance to access its methods.
Accessing Functions from a File Without a Class (Plain Functions)
- Step 1: Create a Python file with regular functions.
File: utility.py
# utility.py
def greet(name):
return f"Hello, {name}!"
def square(num):
return num**2
- Step 2: Import and access the functions in another file.
File: main.py
# main.py
from utility import greet, square
# Call the functions
print(greet("Alice")) # Output: Hello, Alice!
print(square(5)) # Output: 25
Here, we import the functions greet() and square() directly from utility.py and call them in main.py without the need for any class.
Accessing Functions from a File in a Different Directory
If your file is in a different directory, you can use Python’s sys.path or a relative import.
- Step 1: Suppose you have the following directory structure:
project/
│
├── src/
│ └── utility.py
│
└── main.py
- Step 2: In main.py, you can import utility.py from the src directory.
File: main.py
# main.py
import sys
sys.path.append('src')
from utility import greet
# Use the imported function
print(greet("Bob")) # Output: Hello, Bob!
Here, we use sys.path.append() to add the src directory to Python’s search path and then import the function.
Conclusion
In Python, functions can be categorized into several types, including user-defined functions with default or variable-length arguments, nested functions, and methods within classes. These enable efficient code organization, reusability, and better structure for complex programs.
To write efficient functions in Python 3.12, it’s important to leverage built-in functions, minimize function calls inside loops, avoid unnecessary global variables, and take advantage of caching techniques like lru_cache. In addition, accessing functions across different files in Python is straightforward—whether using classes or plain functions—thanks to Python’s modular import system. By organizing functions effectively across files and ensuring their efficiency, Python code can be optimized for both performance and maintainability.
Curated Reads
Technoob I very delighted to find this internet site on bing, just what I was searching for as well saved to fav
Very nice style and design and excellent content, practically nothing else we want : D.
Thank you! Jasper. Glad you spent sometime on my web blog.
I just could not depart your web site prior to suggesting that I really loved the usual info an individual supply in your visitors Is gonna be back regularly to check up on new posts
This is the best comment I’ve read since sometime. Thank you! for your encouraging words.