We started Python with the basics done right in the last chapter, Chapter I. In this chapter we are going to have a look at Python Variables and Data Types in detail. If you want to check out how to setup Python go through this article. Now, lets dive in.
Table of Contents
Chapter II Variables and Data Types.
Type Checking and Type Conversion.
Python Variables and Data Types
Python variables and data types form the foundation of writing any program in the language. Understanding how to define variables and the various data types available will help you store, manage, and manipulate data effectively. Let’s explore these concepts in depth, with practical examples to guide you.
Python Variables
A variable in Python is a name that refers to a memory location where data is stored. Variables in Python are dynamically typed, which means you don’t need to declare the type of a variable when you create it; the type is determined based on the value assigned.
Variable Declaration
In Python, you declare a variable by assigning a value using the assignment operator =. The syntax is:
variable_name = value
Here’s an example:
x = 10 # Assigning an integer value to the variable x
y = 3.14 # Assigning a floating-point value to the variable y
name = “Alice” # Assigning a string value to the variable name
In the above code:
- x is an integer.
- y is a floating-point number (float).
- name is a string.
Reassigning Variables
You can change the value of a variable by simply assigning a new value to it. Python allows a variable to store different types of data at different times, thanks to its dynamic typing.
x = 10 # x is an integer
x = “Hello” # Now x is a string
print(x) # Output: Hello
Python automatically handles the memory allocation and data type reassignment.
Multiple Assignment
Python also allows you to assign values to multiple variables in a single line. This is known as multiple assignment:
a, b, c = 5, 10, "Hello"
print(a) # Output: 5
print(b) # Output: 10
print(c) # Output: Hello
In this case, the values 5, 10, and “Hello” are assigned to a, b, and c, respectively.
Swapping Variables
In Python, you can easily swap the values of two variables without needing a temporary variable:
x, y = 1, 2
x, y = y, x
print(x) # Output: 2
print(y) # Output: 1
Data Types in Python
Data types define the kind of data that can be stored and manipulated within a program. Python provides several built-in data types that allow you to work with different kinds of data, such as numbers, strings, and more.
Redmi Note 13 5G (Prism Gold, 6GB RAM, 128GB Storage) | 5G Ready | 120Hz Bezel-Less AMOLED | 7.mm Slimmest Note Ever | 108MP Pro-Grade Camera
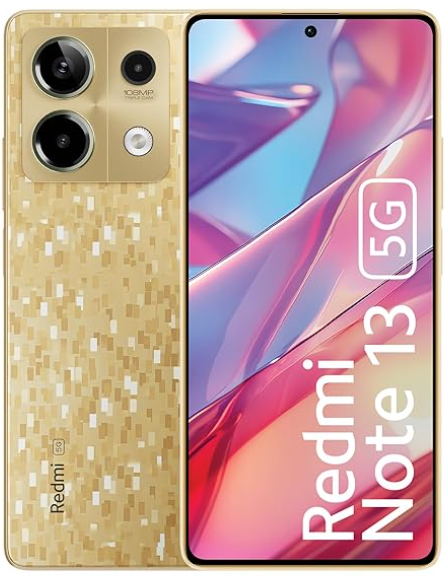
Numeric Data Types
Python supports three main numeric data types:
- Integers (int)
- Floating-point numbers (float)
- Complex numbers (complex)
a. Integers (int)
Integers are whole numbers without a decimal point. They can be positive, negative, or zero.
x = 10 # Positive integer
y = -5 # Negative integer
z = 0 # Zero
b. Floating-Point Numbers (float)
Floating-point numbers represent real numbers with decimal points. Python automatically treats numbers with decimals as floats.
x = 3.14 # Floating-point number
y = -0.001 # Negative float
Python also supports scientific notation for very large or small numbers:
x = 1e3 # 1e3 is 1 * 10^3, which is 1000.0
y = 5.67e-2 # 5.67e-2 is 5.67 * 10^-2, which is 0.0567
c. Complex Numbers (complex)
Complex numbers consist of a real part and an imaginary part, denoted as a + bj, where a is the real part and b is the imaginary part. Python provides native support for complex numbers.
z = 2 + 3j # 2 is the real part, 3j is the imaginary part
print(z.real) # Output: 2.0
print(z.imag) # Output: 3.0
Boolean Data Type (bool)
A boolean data type can hold one of two values: True or False. Booleans are often used in conditional statements to control the flow of the program.
is_raining = True
is_sunny = False
print(is_raining) # Output: True
print(is_sunny) # Output: False
String Data Type (str)
Strings are sequences of characters enclosed in single (‘), double (“), or triple quotes (”’ or “””). Strings are used to represent text.
message = “Hello, World!”
name = ‘Alice’
multiline = “””This is a
multiline string”””
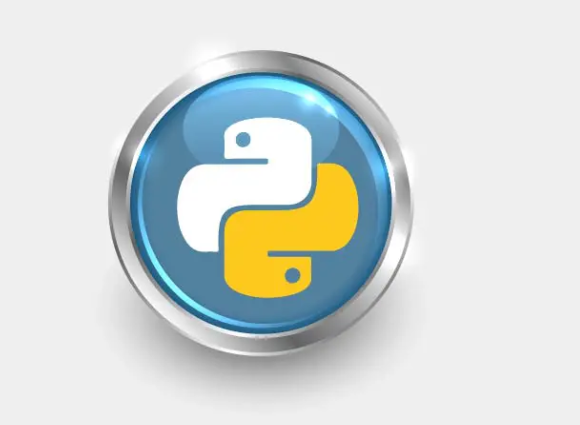
- String indexing: You can access individual characters in a string using index positions, starting from 0 for the first character.
message = “Hello”
print(message[0]) # Output: H
print(message[-1]) # Output: o (negative index counts from the end)
- String slicing: You can also extract a substring using slicing syntax.
message = “Hello, World!”
print(message[0:5]) # Output: Hello
List Data Type (list)
A list is an ordered, mutable collection of items, which can be of different data types. Lists are defined using square brackets [].
fruits = [“apple”, “banana”, “cherry”]
print(fruits[1]) # Output: banana
- Lists are mutable, meaning you can change the elements of a list after it has been created.
fruits[1] = “blueberry”
print(fruits) # Output: [‘apple’, ‘blueberry’, ‘cherry’]
Tuple Data Type (tuple)
A tuple is similar to a list, but it is immutable, meaning once it is created, its values cannot be modified. Tuples are defined using parentheses ().
coordinates = (10, 20)
print(coordinates[0]) # Output: 10
Set Data Type (set)
A set is an unordered collection of unique items, defined using curly braces {}. Sets are useful when you need to ensure that all elements are unique.
unique_numbers = {1, 2, 3, 4, 4, 5}
print(unique_numbers) # Output: {1, 2, 3, 4, 5} (duplicates are removed)
Sets also support mathematical operations like union, intersection, and difference.
Dictionary Data Type (dict)
A dictionary stores data as key-value pairs, where each key is unique. Dictionaries are defined using curly braces {} with keys and values separated by colons :.
person = {“name”: “Alice”, “age”: 25, “city”: “New York”}
print(person[“name”]) # Output: Alice
- Modifying dictionaries: You can add or update entries in a dictionary.
person[“age”] = 26
person[“email”] = “alice@example.com”
print(person)
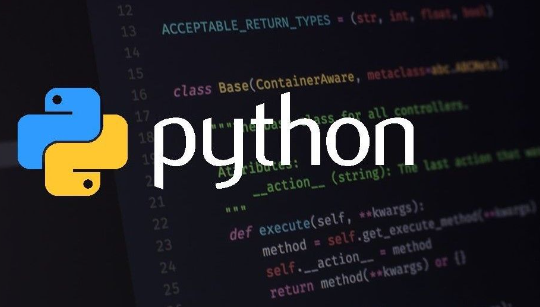
Type Checking and Type Conversion
Type Checking
You can use the type() function to check the data type of a variable.
x = 10
print(type(x)) # Output: <class ‘int’>
y = 3.14
print(type(y)) # Output: <class ‘float’>
Type Conversion
Python provides functions to explicitly convert one data type into another. This process is known as type casting.
- Convert string to integer:
num_str = "100"
num_int = int(num_str)
print(num_int) # Output: 100
print(type(num_int)) # Output: <class 'int'>
- Convert integer to string:
x = 50
x_str = str(x)
print(x_str) # Output: "50"
print(type(x_str)) # Output: <class 'str'>
- Convert float to integer (Note that this will truncate the decimal):
y = 3.99
y_int = int(y)
print(y_int) # Output: 3
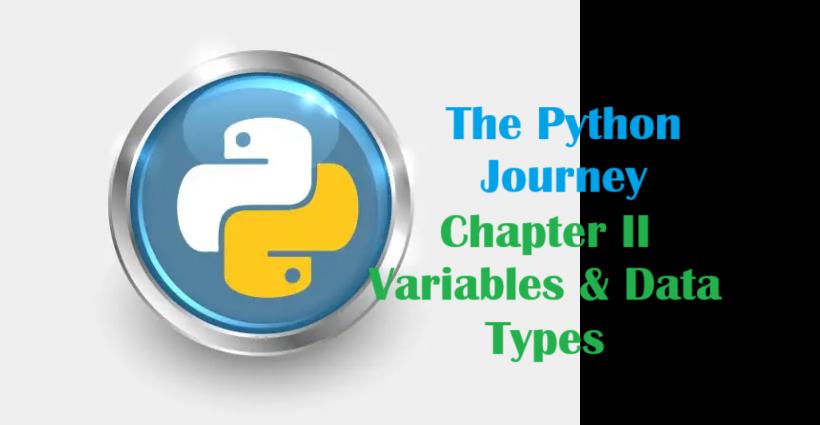
Python variables and data types are the backbone of any program. Variables provide a way to store data, and Python’s dynamic typing allows for flexibility in managing different types of data. Whether you’re working with numbers, text, collections, or more complex data types like dictionaries and sets, Python offers a powerful and intuitive way to handle them all. Understanding these fundamentals will allow you to efficiently manipulate data and solve problems using Python.
- Variables: Variables store data in memory. Python doesn’t require explicit declaration of types.
- Data Types: Python’s standard types include integers, floats, strings, and booleans.
Curated Reads