Here is an article on demand for Python dictionaries ~ Mastering python dictionaries, also known as dicts, are versatile data structures that play a pivotal role in storing, retrieving, and manipulating data. With their key-value pair organization, dictionaries offer unparalleled flexibility and efficiency for handling complex data structures. In this article, we delve into the intricacies of Python dictionaries, exploring their functionality, operations, and best practices for optimal usage. In Java and C#, the equivalent data structure to Python dictionaries is typically known as a “Map.” Correspondingly, in C#, the System.Collections.Generic
namespace provides the Dictionary<TKey, TValue>
class, which is equivalent to Python dictionaries. In both Java and C#, Maps and Dictionaries respectively provide similar functionalities to Python dictionaries, including dynamic data management, key-value pair mappings, efficient lookup operations, and iteration over key-value pairs. These data structures are widely used in both languages for various programming tasks requiring associative data structures.
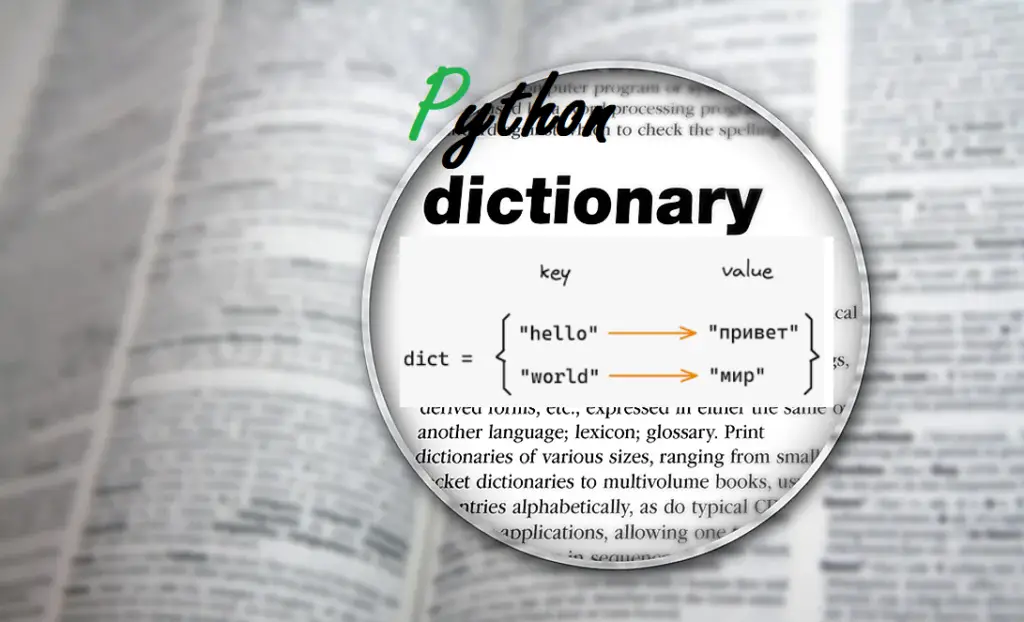
Table of Contents
When to Use Python Dictionaries
Advantages of Python Dictionaries.
Introduction to Python Dictionaries.
Key Features of Python Dictionaries.
Common Operations on Python Dictionaries.
Best Practices for Using Python Dictionaries.
Use Cases for Python Dictionaries.
Differences between Dictionaries, Lists and Tuples.
Listed Differences Between Dictionaries, Lists, and Tuples.
When to Use Python Dictionaries
So when should one use dictionaries? Python dictionaries are ideal for scenarios that involve key-value pair mappings, fast lookup operations, and dynamic data management. Here are some situations where using dictionaries is advantageous:
- Mapping Relationships: Dictionaries are well-suited for representing mappings between entities, such as mapping user IDs to their corresponding profiles, or mapping product names to their prices. This makes dictionaries invaluable for managing relationships and associations in data.
- Fast Lookup Time: Dictionaries offer constant-time lookup operations (O(1)), making them highly efficient for retrieving values based on their associated keys. When quick access to data based on unique identifiers is essential, dictionaries excel over other data structures.
- Dynamic Data Storage: Dictionaries provide flexibility for dynamically adding, modifying, or deleting key-value pairs, enabling the management of evolving datasets. This makes dictionaries ideal for scenarios where data is subject to frequent updates or changes.
- Data Integrity: With dictionaries, keys must be unique, ensuring data integrity and preventing duplicate entries. This property is valuable for maintaining consistency and accuracy in data representations.
- JSON Serialization: Dictionaries serve as the primary data structure for representing JSON (JavaScript Object Notation) data, making them indispensable for tasks such as data interchange, serialization, and deserialization.
When to Use Tuples
Python tuples, with their immutable and ordered characteristics, are well-suited for scenarios that require fixed collections of elements with a specific order. Here are some situations where using tuples is advantageous:
- Data Integrity: Tuples are immutable, meaning their elements cannot be changed after creation. This property ensures data integrity and prevents accidental modifications, making tuples suitable for representing constant or unchanging data.
- Function Return Values: Tuples are commonly used to return multiple values from functions, encapsulating related data into a single immutable object. This simplifies function interfaces and facilitates the handling of complex return values.
- Efficient Memory Usage: Tuples consume less memory compared to lists due to their immutability. In scenarios where memory efficiency is critical, such as handling large datasets or resource-constrained environments, tuples offer an advantage over mutable data structures.
- Parallel Assignments: Tuples support parallel assignments, allowing multiple variables to be assigned values simultaneously. This feature simplifies assignments and enhances code readability, particularly in situations where related data needs to be processed together.
Advantages of Python Dictionaries
Python dictionaries offer several advantages over other data structures, including:
- Fast Lookup Time: Dictionaries provide constant-time lookup operations, enabling efficient retrieval of values based on keys.
- Dynamic Data Management: Dictionaries support dynamic addition, modification, and deletion of key-value pairs, making them adaptable to changing data requirements.
- Data Integrity: Dictionaries enforce uniqueness of keys, ensuring data integrity and preventing duplicate entries.
- Flexibility: Dictionaries can store heterogeneous data types, allowing for versatile data representation and manipulation.
- Serialization: Dictionaries are well-suited for representing JSON data, facilitating data interchange, serialization, and deserialization tasks.
Python dictionaries are invaluable for managing key-value pair mappings, facilitating fast lookup operations, and dynamically managing data. Tuples, with their immutable and ordered characteristics, are advantageous for representing fixed collections of elements with specific order and data integrity requirements. Understanding the strengths and use cases of dictionaries and tuples empowers Python developers to choose the most appropriate data structure for their specific programming needs.
Introduction to Python Dictionaries
Python dictionaries are unordered collections of key-value pairs, enclosed within curly braces {}. Each key-value pair maps a unique key to its corresponding value, allowing for efficient lookup and retrieval of data. Dictionaries can store heterogeneous data types, including integers, strings, lists, tuples, or even other dictionaries.
Key Features of Python Dictionaries
- Fast Lookup Time: Dictionaries employ hash tables internally, enabling constant-time lookup operations. This makes dictionaries highly efficient for accessing and retrieving values based on their associated keys, even for large datasets.
- Mutable Nature: Dictionaries are mutable, meaning their contents can be modified after creation. This flexibility allows for dynamic updates, additions, or deletions of key-value pairs, making dictionaries adaptable to changing data requirements.
- Uniqueness of Keys: Each key within a dictionary must be unique. If duplicate keys are encountered during dictionary creation or modification, the latest key-value pair overrides the previous one.
- Key Immunity: Keys in dictionaries are immutable objects, ensuring their integrity and hashability. Immutable objects such as strings, numbers, or tuples are commonly used as dictionary keys.
Common Operations on Python Dictionaries
- Accessing Elements: Retrieving values from a dictionary using keys through direct indexing or the get() method.
- Adding or Modifying Elements: Assigning values to new keys or existing keys to add or update key-value pairs within a dictionary.
- Removing Elements: Deleting key-value pairs from a dictionary using the del keyword or the pop() method.
- Iterating Through Elements: Traversing dictionaries using loops such as for loops to perform operations on key-value pairs.
- Dictionary Comprehensions: Constructing dictionaries using concise and readable comprehension syntax for creating dictionaries dynamically.
Best Practices for Using Python Dictionaries
- Choose Descriptive Keys: Use meaningful and descriptive keys to enhance code readability and maintainability.
- Avoid Mutating Keys: Since keys are immutable, avoid modifying objects used as keys to prevent unexpected behavior.
- Handle Missing Keys: Employ techniques such as the get() method or the defaultdict class to handle missing keys gracefully and prevent KeyError exceptions.
- Consider Memory Usage: Be mindful of memory usage, especially when working with large dictionaries, as they can consume significant memory resources.
- Leverage Dictionary Methods: Familiarize yourself with built-in dictionary methods and utilize them effectively to streamline code logic and improve efficiency.
Use Cases for Python Dictionaries
- Data Storage and Retrieval: Dictionaries are ideal for storing structured data, such as user profiles, configuration settings, or database records, and facilitating fast retrieval based on unique identifiers.
- Mapping Relationships: Dictionaries are commonly used to represent mappings between entities, such as mapping employee IDs to their corresponding names or product codes to prices.
- Caching and Memoization: Dictionaries can be employed for caching expensive function results or implementing memoization techniques to optimize performance by storing previously computed values.
- JSON Serialization: Dictionaries serve as the primary data structure for representing JSON (JavaScript Object Notation) data, making them indispensable for data interchange and serialization tasks.
Differences between Dictionaries, Lists and Tuples
Feature | Dictionaries | Lists | Tuples |
Mutability | Mutable | Mutable | Immutable |
Order | Unordered | Ordered | Ordered |
Accessing Elements | Accessed by key | Accessed by index | Accessed by index |
Syntax | Enclosed in curly braces {} | Enclosed in square brackets [] | Enclosed in parentheses () |
Usage | Key-value pairs | Collections of items | Collections of items |
Performance | Fast lookup time (O(1)) | Fast indexing (O(1)) | Fast indexing (O(1)) |
Mutating Elements | Add, modify, delete key-value pairs | Add, remove, modify elements | Not possible |
Hashability | Keys must be hashable | N/A | N/A |
Memory Efficiency | May consume more memory due to hash table | Consumes memory proportional to size | Consumes less memory due to immutability |
Listed Differences Between Dictionaries, Lists, and Tuples
- Mutability: Dictionaries are mutable, allowing for dynamic changes to key-value pairs. Lists are mutable, enabling additions, removals, and modifications of elements. Tuples are immutable, meaning their elements cannot be changed after creation.
- Order: Dictionaries are unordered collections, while lists maintain the order of elements and tuples also preserve order.
- Accessing Elements: Dictionaries are accessed by keys, whereas lists and tuples are accessed by index.
- Syntax: Dictionaries are enclosed in curly braces
{}
, lists in square brackets[]
, and tuples in parentheses()
. - Usage: Dictionaries are used for key-value pair mappings, lists for collections of items where order matters, and tuples for collections of items with fixed order and immutability.
- Performance: Dictionaries offer fast lookup time (O(1)) due to hash tables. Lists and tuples also offer fast indexing (O(1)), but lists may have slightly slower performance due to their dynamic resizing.
- Mutating Elements: Dictionaries allow adding, modifying, and deleting key-value pairs. Lists support adding, removing, and modifying elements. Tuples do not allow mutation of elements after creation.
- Hashability: Dictionaries require keys to be hashable objects. Lists and tuples do not have this requirement.
- Memory Efficiency: Dictionaries may consume more memory due to the overhead of hash tables. Lists consume memory proportional to their size, and tuples consume less memory due to their immutability.
Sample Code
# Creating a dictionary to store student grades
student_grades = {
“Alice”: 85,
“Bob”: 90,
“Charlie”: 75,
“David”: 88,
“Emma”: 92
}
# Adding a new student and their grade
student_grades[“Frank”] = 78
# Modifying a student’s grade
student_grades[“Charlie”] = 80
# Removing a student from the dictionary
del student_grades[“David”]
# Accessing a student’s grade
alice_grade = student_grades[“Alice”]
print(“Alice’s grade:”, alice_grade)
# Checking if a student is in the dictionary
is_emma_present = “Emma” in student_grades
print(“Is Emma present?”, is_emma_present)
# Iterating through the dictionary
print(“Student Grades:”)
for student, grade in student_grades.items():
print(student + “:”, grade)
Here’s some code that would help you to get all unique values stored in a python dictionary
If you want to create a Python dictionary where each value is unique, you can achieve this by ensuring that each value you add to the dictionary is unique. Here’s a code example that demonstrates how to achieve this:
def add_unique_value(dictionary, key, value):
“””
Add a unique value to the dictionary for the given key.
If the key already exists and the value is not unique, raise an error.
“””
if value in dictionary.values():
raise ValueError(“Value already exists in the dictionary.”)
else:
dictionary[key] = value
# Example usage:
my_dict = {}
add_unique_value(my_dict, “key1”, 10)
add_unique_value(my_dict, “key2”, 20)
add_unique_value(my_dict, “key3”, 30)
# This will raise an error since 20 already exists in the dictionary.
# add_unique_value(my_dict, “key4”, 20)
print(my_dict)
In this code:
- The add_unique_value function takes a dictionary, a key, and a value as input.
- It checks if the given value already exists in the dictionary using value in dictionary.values().
- If the value is not already present, it adds the key-value pair to the dictionary.
- If the value already exists, it raises a ValueError.
- You can use this function to ensure that each value added to the dictionary is unique.
In a nutshell, Python dictionaries are indispensable tools for managing and manipulating key-value pairs efficiently. With their fast lookup time, mutable nature, and wide range of operations, dictionaries offer unparalleled versatility for a myriad of programming tasks. By mastering the intricacies of Python dictionaries and adhering to best practices, developers can harness the full potential of dictionaries to build robust, scalable, and efficient Python applications.
Related Reads:
Самые актуальные новинки мировых подиумов.
Актуальные мероприятия самых влиятельных подуимов.
Модные дома, торговые марки, haute couture.
Новое место для трендовых людей.
Очень свежие события индустрии.
Абсолютно все эвенты известнейших подуимов.
Модные дома, торговые марки, высокая мода.
Интересное место для модных людей.
Модные заметки по подбору превосходных луков на каждый день.
Мнения профессионалов, новости, все новые коллекции и мероприятия.