A leap year is a year that contains an additional day, making it 366 days long instead of the usual 365 days. This extra day is added to the calendar to keep it synchronized with the Earth’s revolutions around the Sun.
The standard calendar year consists of approximately 365.25 days, as it takes about 365.25 days for the Earth to complete one orbit around the Sun. To account for this fractional part of a day, a leap year is added every four years.
However, this rule is slightly adjusted to account for the fact that the Earth’s orbit is not precisely 365.25 days. It takes about 365.2425 days for the Earth to orbit the Sun. To account for this discrepancy, the rule for leap years is modified as follows:
– A year that is divisible by 4 is a leap year, except for…
– Years that are divisible by 100 are not leap years, except for…
– Years that are divisible by 400 are leap years.
By following this rule, the average length of the calendar year is brought closer to the actual length of the Earth’s orbit, minimizing the cumulative discrepancy over time. Leap years are important for maintaining the alignment of the calendar with the Earth’s seasons, and they ensure that important events like equinoxes and solstices occur around the same time each year. Enough told about science, lets figure out what this article really deals with. 😊
Leap year logic in python
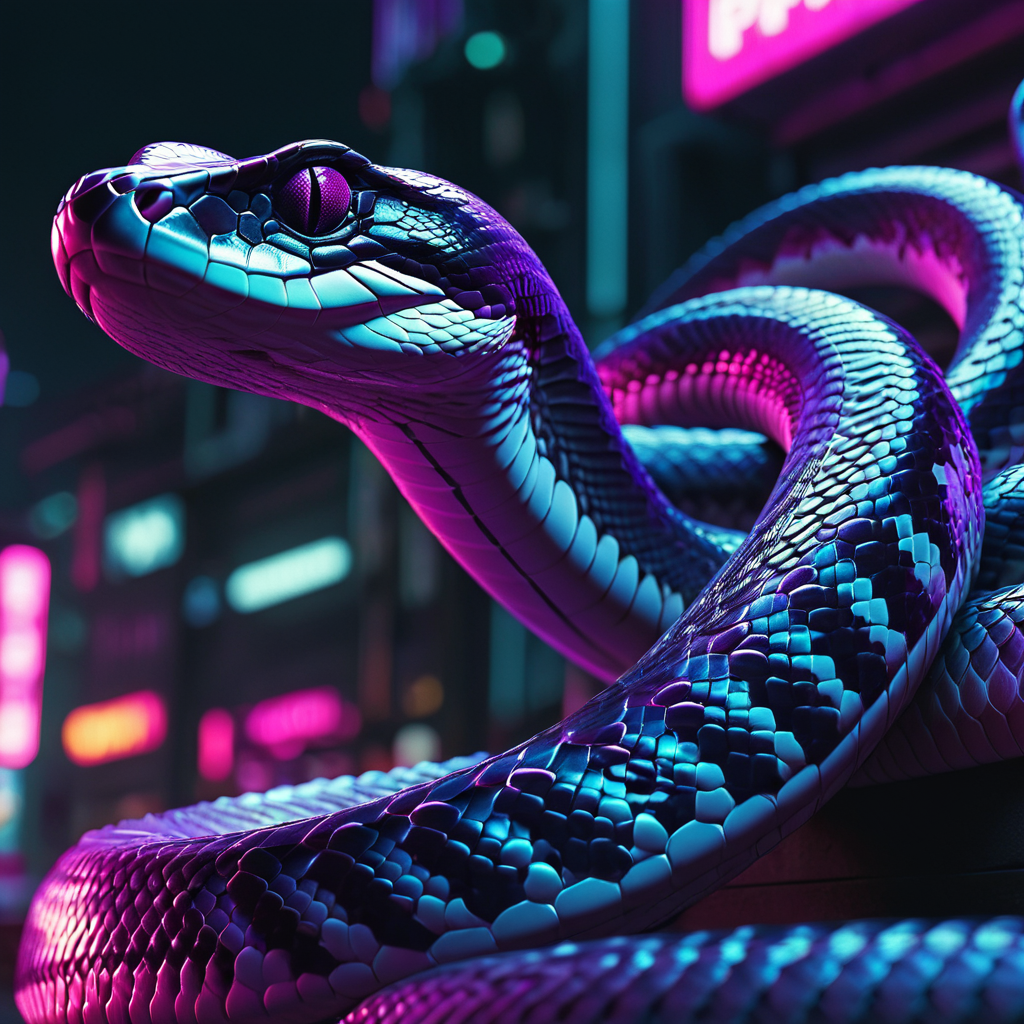
In Python, you can determine if a year is a leap year by using the following logic:
def is_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
return True
else:
return False
else:
return True
else:
return False
year = 2024
if is_leap_year(year):
print(year, "is a leap year.")
else:
print(year, "is not a leap year.")
In this code snippet, the is_leap_year() function takes a year as input and checks if it satisfies the leap year conditions. It returns True if it is a leap year and False otherwise. The conditions are as follows:
- If the year is divisible by 4, it is a potential leap year.
- If the year is divisible by 100, it is not a leap year unless…
- If the year is divisible by 400, it is a leap year.
You can call the is_leap_year() function with a specific year and then use conditional statements to display the result accordingly. In the example above, the year 2024 is tested to check if it’s a leap year.
Why even bother about leap year calculations where it might be required ?
One real-world example where leap year calculation is required is in financial systems or software that deal with interest calculations or time-based calculations. Leap year calculation is important to ensure accurate calculations of interest accruals, loan durations, payment schedules, or any other time-dependent financial calculations.
For instance, let’s consider a scenario where a bank provides a loan with an annual interest rate. The interest on the loan accrues on a daily basis, and the bank needs to calculate the correct interest amount based on the number of days between two dates, taking leap years into account.
In this case, accurate calculation of the number of days between two dates requires considering the leap years correctly. Incorrectly assuming all years to be 365 days long could lead to incorrect interest calculations, potentially impacting the borrower or the lender. Therefore, financial systems or software that involve interest calculations, payment schedules, or any other time-dependent financial operations need to incorporate leap year calculations to ensure precise and reliable results.
Real world example
In this program, the is_leap_year()
function is the same as the earlier example, which checks if a year is a leap year. The calculate_interest()
function takes the start year, end year, and interest rate as input parameters.
The function calculates the total number of days between the start year and end year, considering 365 days per non-leap year. It then iterates through each year in the range and counts the number of leap years using the is_leap_year()
function. The leap year count is added to the total number of days.
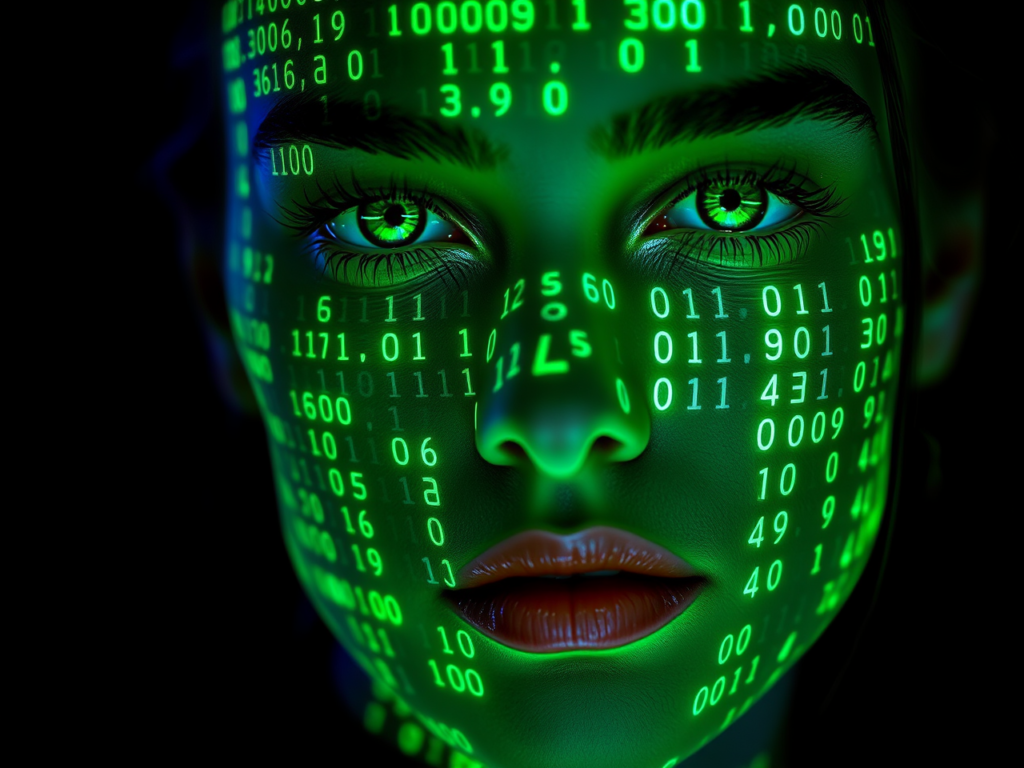
Finally, the function calculates the interest by multiplying the total number of days (including leap years) with the interest rate. The calculated interest is returned.
def is_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
else:
else:
else:
def calculate_interest(start_year, end_year, interest_rate):
leap_year_count =
total_days = (end_year - start_year) *
for year inrange(start_year, end_year + 1):
if is_leap_year(year):
leap_year_count +=
total_days += leap_year_count
interest = total_days * interest_rate
return interest
start_year =
end_year =
interest_rate =
interest = calculate_interest(start_year, end_year, interest_rate)
print(f"The interest for the period from {start_year} to {end_year} is: {interest}")
In the example code, the program calculates the interest for the period from 2020 to 2023 with an interest rate of 0.05 (5%). The result is then printed to the console. You can modify the input parameters according to your specific financial system requirements.
Standalone version of above program
In this version, the program contains a main()
function that serves as the entry point. The user is prompted to input the start year, end year, and interest rate, which are then passed to the calculate_interest()
function.
The calculate_interest()
function calculates the interest in the same manner as before. It uses the is_leap_year()
function to determine the number of leap years within the given range.
Finally, the program prints the calculated interest to the console. You can run this standalone program by executing it in a Python environment. It will prompt you to enter the necessary input, calculate the interest, and display the result.
def is_leap_year(year):
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
else:
else:
else:
def calculate_interest(start_year, end_year, interest_rate):
leap_year_count =
total_days = (end_year - start_year) *
for year inrange(start_year, end_year + 1):
if is_leap_year(year):
leap_year_count +=
total_days += leap_year_count
interest = total_days * interest_rate
return interest
def main():
start_year = int(input("Enter the start year: "))
end_year = int(input("Enter the end year: "))
interest_rate = float(input("Enter the interest rate: "))
interest = calculate_interest(start_year, end_year, interest_rate)
print(f"The interest for the period from {start_year} to {end_year} is: {interest}")
if __name__ == "__main__":
main()
As you can see python is a brilliantly simple language with powerful libraries. The Python program provided above offers a practical solution for calculating leap years within a financial system. By incorporating the `is_leap_year()` function, it accurately determines whether a year is a leap year or not. The `calculate_interest()` function utilizes this leap year calculation to accurately compute the interest based on the given start year, end year, and interest rate. The standalone version of the program allows users to input the necessary parameters and obtain the calculated interest conveniently. By considering leap years, financial systems can ensure precise and reliable calculations for various time-dependent financial operations.
Wow! This could be one particular of the most useful blogs We’ve ever arrive across on this subject. Basically Wonderful. I’m also an expert in this topic so I can understand your hard work.