Python is one of the most popular programming languages in the world today, largely due to its simplicity, versatility, and extensive library support. One of the most essential constructs in Python is the for loop, a type of loop that allows developers to repeat a block of code multiple times. Understanding how the for loop works in Python can significantly enhance your coding skills and make your programs more efficient, especially when working with collections like lists, dictionaries, and files. In this article, we’ll cover everything you need to know about Python’s for loop, from basic syntax to real-world use cases, while highlighting its importance in various programming scenarios.
Table of Contents
Introduction to Python For Loop.
Understanding the Basics of Python For Loop Syntax.
Python For Loop and the range() Function.
Real-World Applications of Python For Loop.
Introduction to Python For Loop
The concept of loops in programming is fundamental; they allow you to execute a set of instructions repeatedly without rewriting the code for each iteration. Python offers two main types of loops: for loops and while loops. Here, we’ll focus on the for loop, which is particularly useful for iterating over a collection, such as a list or tuple, or even through characters in a string. By leveraging Python’s powerful for loop, you can easily automate repetitive tasks, traverse data structures, and manipulate collections with just a few lines of code.
In Python, the for loop differs slightly from traditional programming languages. Instead of relying on numeric indexes, Python’s for loop iterates over the elements directly, which makes the code more readable and aligns with Python’s philosophy of being clear and concise. This design choice eliminates the need for complex index management and provides a direct way to work with each item in a sequence. As we delve into this guide, you’ll understand how Python’s for loop operates and see how it fits into a variety of real-world programming scenarios.
We’ll start with a basic overview of the for loop syntax and gradually move on to more advanced applications. By the end, you’ll be equipped with the knowledge to implement Python for loops in multiple contexts, from list processing and dictionary iteration to nested loops and more complex data-handling tasks.
Whether you’re working on data analysis, software development, or automation, the Python for loop will serve as a cornerstone in your coding toolkit.
Understanding the Basics of Python For Loop Syntax
The syntax of the Python for loop is simple and straightforward:
for item in collection:
# Code to execute in each iteration
Here:
- item represents each element in the collection as the loop iterates.
- collection is the sequence or iterable object you want to iterate over, like a list, tuple, dictionary, set, or string.
- The indented block below the for statement contains the code that executes for each item in the collection.
For example, here’s a basic for loop that iterates over a list of numbers and prints each one:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
print(num)
Explanation:
In this code snippet, the for loop iterates through each element in the numbers list. In each iteration, the current element (num) is printed.
Python For Loop and the range() Function
One of the most common applications of the for loop in Python is using it with the range() function, which generates a sequence of numbers. This is useful when you want to repeat an action a specific number of times without relying on an existing list or tuple.
for i in range(5):
print(i)
This loop will print numbers from 0 to 4. The range() function generates numbers starting from 0 and stops before the specified endpoint, making it a half-open interval.
Customizing range() for Step Sizes and Start Points
Python’s range() function can also take up to three arguments: range(start, stop, step). This allows for more flexibility in controlling the loop’s behavior.
For example, to print even numbers between 0 and 10, we can use:
for i in range(0, 11, 2):
print(i)
Real-World Applications of Python For Loop
The for loop is not only useful for basic tasks; it’s also a powerful tool in a variety of programming applications. Let’s explore some practical examples:
1. Iterating Over a List of Items
A common use of for loops is to iterate over elements in a list. This can be particularly useful when you want to process or manipulate each item individually.
Example: Finding the Sum of a List of Numbers
numbers = [10, 20, 30, 40]
total = 0
for num in numbers:
total += num
print("Total:", total)
This code snippet sums up all elements in the numbers list.
2. Iterating Over a String
Strings in Python are iterable, which means you can use a for loop to iterate through each character individually.
Example: Counting Vowels in a String
text = "hello world"
vowels = "aeiou"
vowel_count = 0
for char in text:
if char in vowels:
vowel_count += 1
print("Number of vowels:", vowel_count)
This code calculates the number of vowels in the text string by iterating through each character and checking if it is a vowel.
3. Using For Loop with Dictionaries
Dictionaries are a vital data structure in Python and are often used to store key-value pairs. When you iterate over a dictionary with a for loop, you can choose to iterate over keys, values, or both.
Example: Iterating Over Keys and Values
student_scores = {"Alice": 85, "Bob": 92, "Charlie": 78}
for name, score in student_scores.items():
print(f"{name}: {score}")
In this example, the items() method retrieves each key-value pair from the dictionary, allowing the for loop to unpack them into name and score.
4. Nested For Loops
A for loop can contain another for loop inside it, known as a nested loop. Nested loops are particularly useful when working with multi-dimensional data, such as lists of lists or matrices.
Example: Printing a Matrix
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
for row in matrix:
for element in row:
print(element, end=" ")
print()
This code iterates over each row in the matrix and then iterates over each element within the row, effectively printing a matrix in a readable format.
5. Using For Loop with the enumerate() Function
The enumerate() function in Python is an incredibly useful tool that allows you to access both the index and the value of each element in a loop. This is particularly helpful when you need the position of an item while iterating through a list.
Example: Accessing Index and Value
fruits = [“apple”, “banana”, “cherry”]
for index, fruit in enumerate(fruits):
print(f”Index {index}: {fruit}”)
In this example, enumerate() provides both the index and the value, making it easy to keep track of each element’s position in the list.
6. For Loop for File Handling
Python for loops can also be used to read files line-by-line, which is extremely useful for text processing tasks, such as data parsing and log analysis.
Example: Reading a File Line-by-Line
with open(“sample.txt”, “r”) as file:
for line in file:
print(line.strip())
Here, each line in sample.txt is read and printed without extra spaces, due to the strip() method.
7. Using For Loop with List Comprehensions
List comprehensions are a Pythonic way to create lists by combining for loops and conditional statements into a single line of code. They are especially helpful for generating new lists based on existing lists.
Example: Generating a List of Squares
numbers = [1, 2, 3, 4, 5]
squares = [num ** 2 for num in numbers]
print(squares)
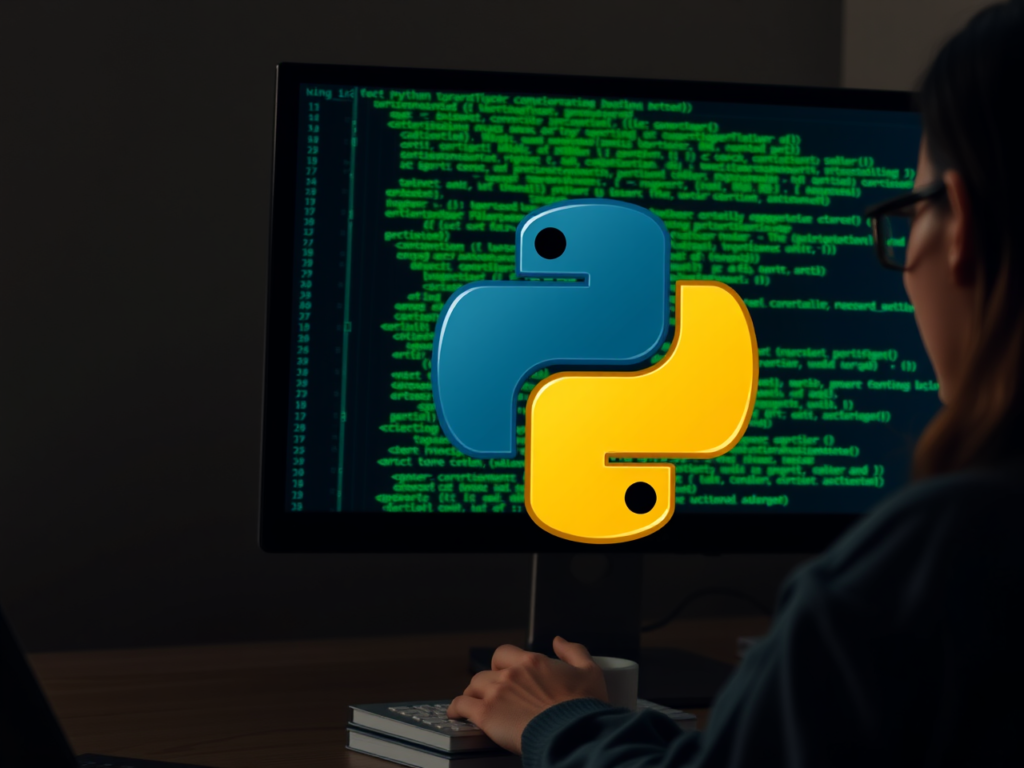
This list comprehension creates a new list of squares by iterating over numbers and squaring each element.
8. Filtering Data Using For Loop
for loops are often used to filter data by conditionally appending elements to a new list. This approach is helpful when working with large datasets or cleaning data for analysis.
Example: Filtering Even Numbers
numbers = [1, 2, 3, 4, 5, 6]
evens = [num for num in numbers if num % 2 == 0]
print("Even numbers:", evens)
This code snippet creates a new list evens by filtering out all numbers that are not even.
9. Break and Continue in Python For Loop
The break and continue statements can alter the flow of a for loop. break exits the loop entirely, while continue skips to the next iteration without completing the current one.
Example: Using break and continue
for i in range(10):
if i == 5:
break
elif i % 2 == 0:
continue
print(i)
In this code:
- continue skips even numbers.
- break stops the loop when i reaches 5.
Conclusion
The Python for loop is a fundamental construct that simplifies many programming tasks. From basic iteration over lists and strings to handling complex data structures like dictionaries and matrices, the for loop in Python is both versatile and easy to understand. By mastering the for loop, you unlock the ability to automate tasks, filter data, process files, and handle data structures efficiently.
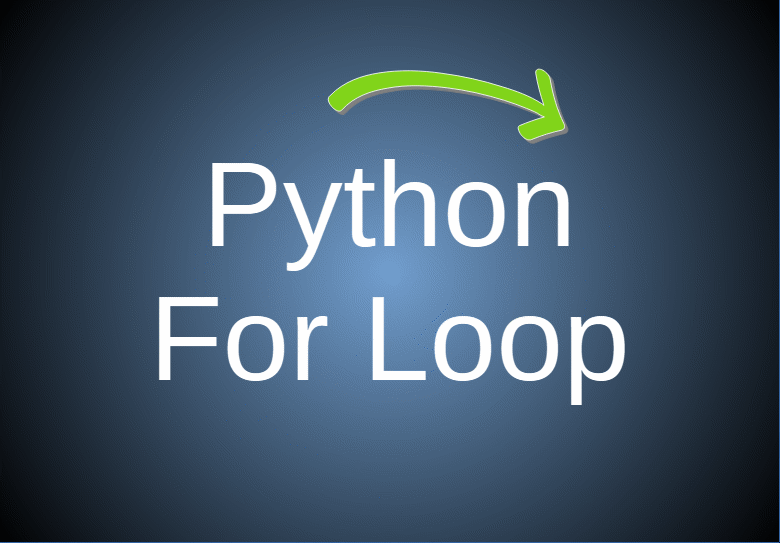
Python’s simplicity in handling loops allows developers to focus more on problem-solving and less on the syntactic overhead. As you continue practicing with for loops in real-world projects, you’ll find them invaluable for tasks involving repetitive processes and data manipulation. Whether you’re building software, analyzing data, or managing files, the for loop is an essential tool that brings both power and flexibility to your programming skillset.
Curated for you
I do agree with all the ideas you have introduced on your post They are very convincing and will definitely work Still the posts are very short for newbies May just you please prolong them a little from subsequent time Thank you for the post
Ezippi naturally like your web site however you need to take a look at the spelling on several of your posts. A number of them are rife with spelling problems and I find it very bothersome to tell the truth on the other hand I will surely come again again.
The articles you write help me a lot and I like the topic