Python is one of the most widely used programming languages due to its simplicity and power. One of the key features of Python is its support for methods—functions defined inside a class. Python methods play a significant role in object-oriented programming (OOP) by encapsulating behaviours and allowing interactions with object data.
In this comprehensive guide, we’ll delve into:
- What methods are in Python.
- The different types of methods.
- How methods are defined and used.
- The importance and use of dunder methods (special methods) in Python.
- Real-world examples of how methods are applied in everyday business scenarios.
We will be focusing on Python 3.12 for all code examples.
Table of Contents
- What Are Methods in Python?
- Types of Methods in Python
- Defining and Calling Methods
- Dunder Methods (Special Methods)
- Real-World Examples
- Best Practices for Working with Methods in Python
- Conclusion
1. Methods in Python
A method in Python is essentially a function that belongs to a class. It operates on objects of the class and can access the data (attributes) stored in those objects. Methods are used to define the behaviours and actions that objects of a class can perform.
The basic syntax for a method inside a class is:
class ClassName:
def method_name(self, parameters):
# method body
- The first parameter of any instance method is self, which refers to the instance of the class.
2. Types of Methods in Python
There are three types of methods in Python:
- Instance Methods
- Class Methods
- Static Methods
Let’s explore each one in detail.
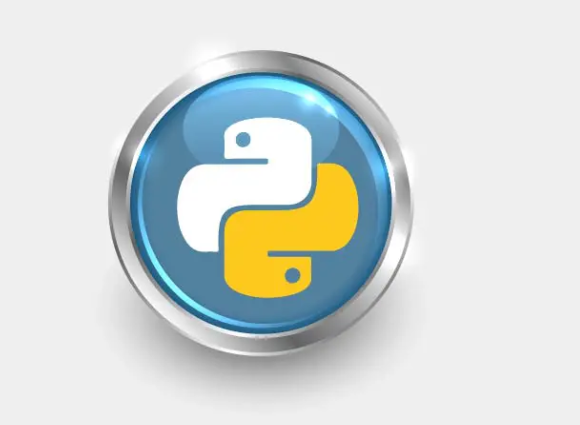
2.1. Instance Methods
Instance methods are the most common type of method in Python. They operate on an instance of the class and can modify the object’s attributes.
The defining characteristic of an instance method is that it takes self as the first parameter, which refers to the instance itself.
Example:
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
def give_raise(self, amount):
self.salary += amount
return f"{self.name} now has a salary of {self.salary}"
# Creating an instance of Employee
emp = Employee("John", 50000)
print(emp.give_raise(5000)) # Output: John now has a salary of 55000
In this example, the method give_raise() is an instance method because it modifies the state of the Employee instance (self.salary).
2.2. Class Methods
Class methods are methods that are bound to the class and not the instance of the class. They take cls (the class itself) as the first parameter instead of self. Class methods can be used to modify class-level data that is shared across all instances.
To define a class method, use the @classmethod decorator.
Example:
class Employee:
company_name = "Tech Corp"
def __init__(self, name):
self.name = name
@classmethod
def change_company_name(cls, new_name):
cls.company_name = new_name
# Change the company name
Employee.change_company_name("Innovate Inc")
# Check the company name for an instance
emp = Employee("Alice")
print(emp.company_name) # Output: Innovate Inc
Here, change_company_name() is a class method that modifies the class-level attribute company_name.
2.3. Static Methods
Static methods are methods that belong to the class but do not operate on class or instance data. They don’t take self or cls as parameters. Static methods are used when the method logic doesn’t depend on the instance or class itself, but the method is conceptually related to the class.
To define a static method, use the @staticmethod decorator.
Example:
class MathOperations:
@staticmethod
def add(a, b):
return a + b
@staticmethod
def subtract(a, b):
return a - b
print(MathOperations.add(10, 5)) # Output: 15
print(MathOperations.subtract(10, 5)) # Output: 5
In this example, the static methods add() and subtract() do not modify or access any class or instance variables.
3. Defining and Calling Methods
3.1. Defining Methods
In Python, methods are defined inside classes. The method’s first parameter distinguishes between instance, class, and static methods.
Syntax:
class ClassName:
def instance_method(self, parameters):
# code for instance method
@classmethod
def class_method(cls, parameters):
# code for class method
@staticmethod
def static_method(parameters):
# code for static method
3.2. Calling Methods
Methods can be called on instances (for instance methods) or directly on the class (for class and static methods).
Example:
# Calling an instance method
emp = Employee("Bob", 40000)
print(emp.give_raise(3000))
# Calling a class method
Employee.change_company_name("FutureTech")
# Calling a static method
print(MathOperations.add(10, 20))
4. Dunder Methods (Special Methods)
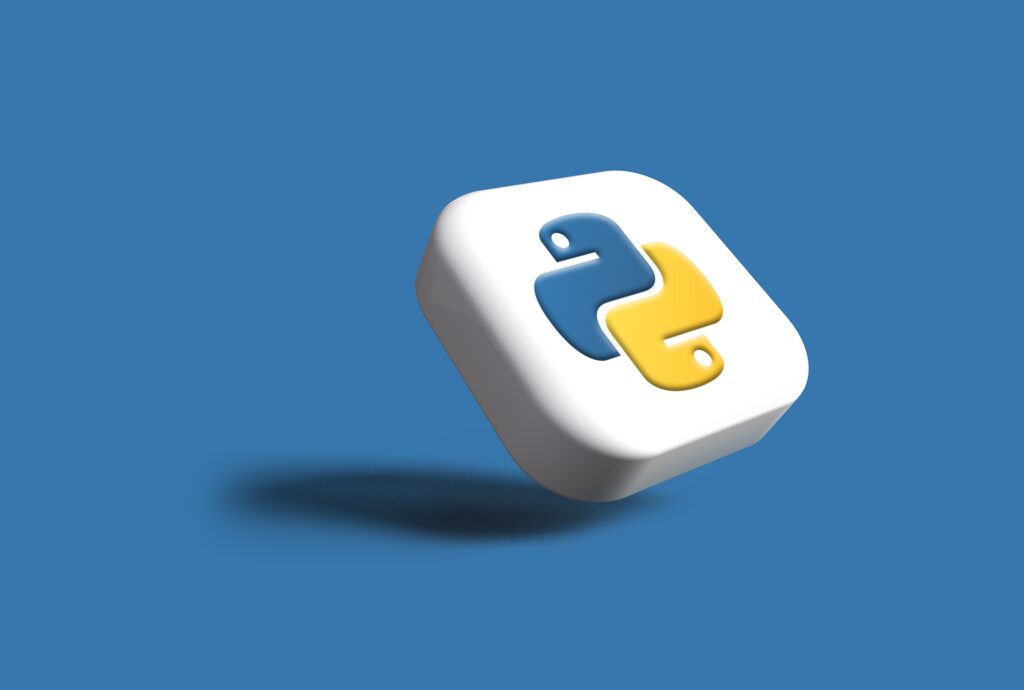
Dunder methods (also known as special methods or magic methods) in Python have double underscores (__) at the beginning and end of their names. These methods allow you to define behaviour’s for built-in Python operations such as addition, subtraction, string representation, and more.
Common Dunder Methods:
- __init__(self, …): The constructor method, called when an object is created.
- __str__(self): Defines the behaviour when str() or print() is called on an object.
- __repr__(self): Defines the behaviour when repr() is called.
- __add__(self, other): Defines the behaviour of the + operator for custom objects.
- __len__(self): Defines the behaviour when len() is called.
Example of Dunder Methods:
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __str__(self):
return f"'{self.title}' by {self.author}"
def __len__(self):
return self.pages
def __add__(self, other):
return self.pages + other.pages
book1 = Book("1984", "George Orwell", 328)
book2 = Book("Animal Farm", "George Orwell", 112)
# Using dunder methods
print(str(book1)) # Output: '1984' by George Orwell
print(len(book1)) # Output: 328
print(book1 + book2) # Output: 440 (sum of pages)
In the above example:
- __str__ defines how the Book object will appear when printed.
- __len__ defines the behavior of the len() function when used with a Book object.
- __add__ allows the use of the + operator between two Book objects to add the number of pages.
5. Real-World Examples of Python Methods
5.1. Instance Methods in a Banking System
Consider a banking system where we manage customer accounts. Each customer has a bank account, and the balance can be modified through deposits or withdrawals.
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self.balance = balance
def deposit(self, amount):
self.balance += amount
return f"Deposited {amount}. New balance is {self.balance}"
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
return f"Withdrew {amount}. New balance is {self.balance}"
else:
return "Insufficient balance."
# Creating an instance of BankAccount
account = BankAccount("123456789", 500)
print(account.deposit(100)) # Output: Deposited 100. New balance is 600
print(account.withdraw(200)) # Output: Withdrew 200. New balance is 400
Here, deposit and withdraw are instance methods operating on the attributes of the BankAccount class.
5.2. Class Methods in a Retail Business
Imagine a retail business where the price of all items can be adjusted for inflation. We can use a class method to modify the base price of items for all instances.
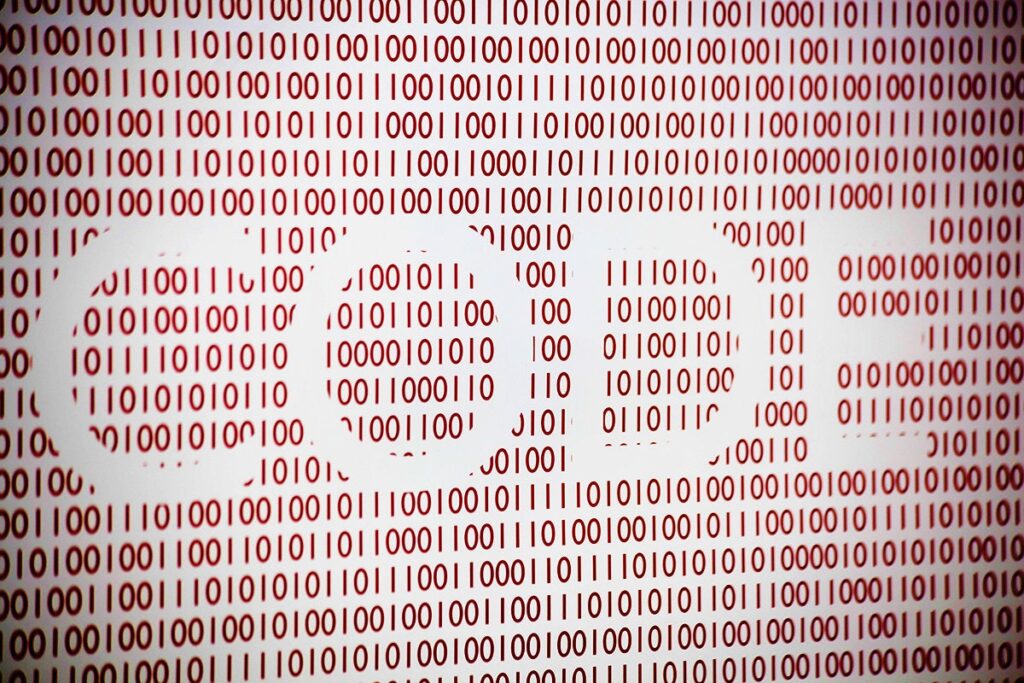
class Item:
inflation_rate = 0.03 # class variable
def __init__(self, name, price):
self.name = name
self.price = price
@classmethod
def adjust_for_inflation(cls):
cls.inflation_rate += 0.02 # increase inflation rate for all items
item1 = Item("Laptop", 1000)
Item.adjust_for_inflation() # Adjust inflation rate globally
print(Item.inflation_rate) # Output: 0.05
In this scenario, adjust_for_inflation() modifies the class-level inflation rate that applies to all Item objects.
5.3. Static Methods in a Logistics Company
A logistics company might need to calculate the distance between two locations based on their coordinates. This can be done using a static method, as it doesn’t depend on any class or instance data.
class Location:
@staticmethod
def calculate_distance(coord1, coord2):
# Dummy implementation for distance calculation
return ((coord2[0] - coord1[0]) ** 2 + (coord2[1] - coord1[1]) ** 2) ** 0.5
distance = Location.calculate_distance((0, 0), (3, 4))
print(distance) # Output: 5.0 (Pythagorean theorem: sqrt(3^2 + 4^2))
Here, calculate_distance is a static method as it doesn’t require access to any instance or class data.
6. Best Practices for Working with Methods in Python
- Keep Methods Simple and Focused: Methods should do one thing and do it well. Large methods with multiple responsibilities can become difficult to debug and maintain.
- Use Meaningful Names: Name your methods based on their behavior. For example, use calculate_salary() rather than process().
- Limit Side Effects: A method should only modify the attributes of the instance if necessary. Minimize methods that change global or external variables unless clearly documented.
- Use Static Methods for Utility Functions: If a method doesn’t need to access any instance or class-specific data, define it as a static method.
- Encapsulate Behavior: Use methods to encapsulate the internal workings of your classes, exposing only the necessary functionality to the outside world.
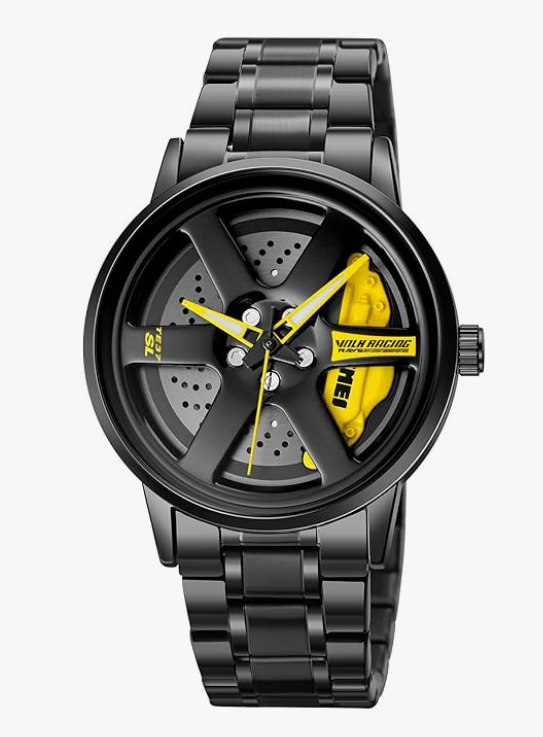
SKMEI Men’s Watch
New Wheels Rolling Creative Fashion Che Youhui League Fans Butterfly Double Snap Gift Wristwatch – 1787
Less Discount -79% ₹1,449
7. Conclusion
In Python, methods are essential for creating structured, modular, and reusable code in object-oriented programming. They allow developers to define and organize the behavior of objects efficiently. Whether it’s instance methods manipulating object state, class methods modifying class-level data, or static methods providing utility functions, methods form the backbone of Python classes.
Furthermore, special dunder methods enable Python objects to interact seamlessly with built-in operations, such as printing, adding, and more. This flexibility, coupled with the simplicity of Python’s syntax, makes methods a powerful tool in building real-world applications across various domains—from banking systems to logistics and retail.
Understanding how to use methods properly is critical for mastering Python and writing clean, maintainable code. By following best practices and leveraging the versatility of methods, you can create scalable and robust Python applications for a wide range of real-world problems.
Curated reads